Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / Media / Animation / EasingFunctionBase.cs / 1305600 / EasingFunctionBase.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation, 2008 // // File: EasingFunctionBase.cs //----------------------------------------------------------------------------- namespace System.Windows.Media.Animation { ////// This class is the base class for many easing functions. /// public abstract class EasingFunctionBase : Freezable, IEasingFunction { ////// EasingMode Property /// public static readonly DependencyProperty EasingModeProperty = DependencyProperty.Register( "EasingMode", typeof(EasingMode), typeof(EasingFunctionBase), new PropertyMetadata(EasingMode.EaseOut)); ////// Specifies the easing behavior. /// public EasingMode EasingMode { get { return (EasingMode)GetValue(EasingModeProperty); } set { SetValueInternal(EasingModeProperty, value); } } ////// Transforms normalized time to control the pace of an animation. /// /// normalized time (progress) of the animation ///transformed progress ///Uses EasingMode in conjunction with EaseInCore to evaluate the easing function. public double Ease(double normalizedTime) { switch (EasingMode) { case EasingMode.EaseIn: return EaseInCore(normalizedTime); case EasingMode.EaseOut: // EaseOut is the same as EaseIn, except time is reversed & the result is flipped. return 1.0 - EaseInCore(1.0 - normalizedTime); case EasingMode.EaseInOut: default: // EaseInOut is a combination of EaseIn & EaseOut fit to the 0-1, 0-1 range. return (normalizedTime < 0.5) ? EaseInCore( normalizedTime * 2.0 ) * 0.5 : (1.0 - EaseInCore((1.0 - normalizedTime) * 2.0)) * 0.5 + 0.5; } } ////// Transforms normalized time to control the pace of an animation for the EaseIn EasingMode /// /// normalized time (progress) of the animation ///transformed progress ////// You only have to specifiy your easing function for the 'EaseIn' case because the implementation /// of Ease will handle transforming normalizedTime & the result of this method to handle 'EaseOut' & 'EaseInOut'. /// protected abstract double EaseInCore(double normalizedTime); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
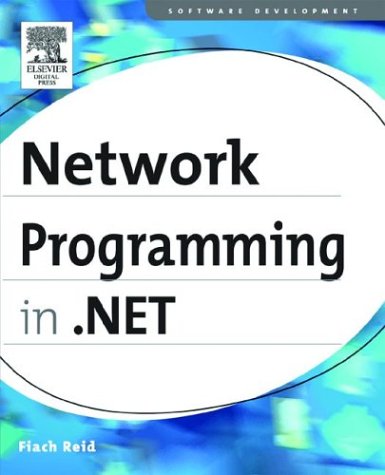
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- StorageEntityTypeMapping.cs
- NoneExcludedImageIndexConverter.cs
- RootBuilder.cs
- MonthChangedEventArgs.cs
- RefreshResponseInfo.cs
- RuntimeConfigurationRecord.cs
- HandoffBehavior.cs
- Decoder.cs
- SmiGettersStream.cs
- WebPartDisplayModeCollection.cs
- RecordConverter.cs
- UriExt.cs
- SerialPort.cs
- HeaderElement.cs
- TimelineClockCollection.cs
- GroupLabel.cs
- WaitHandleCannotBeOpenedException.cs
- TraceInternal.cs
- ExceptionHandlers.cs
- Int32.cs
- XsdDataContractExporter.cs
- PlaceHolder.cs
- QilStrConcatenator.cs
- SymDocumentType.cs
- MethodInfo.cs
- ObjectDataSource.cs
- ContentElement.cs
- CodeComment.cs
- TextParaLineResult.cs
- ControlBuilderAttribute.cs
- AbstractSvcMapFileLoader.cs
- CancellationToken.cs
- SymbolDocumentGenerator.cs
- MsmqEncryptionAlgorithm.cs
- XmlSignificantWhitespace.cs
- LocationUpdates.cs
- ConcatQueryOperator.cs
- SessionSymmetricMessageSecurityProtocolFactory.cs
- HealthMonitoringSectionHelper.cs
- Comparer.cs
- FormatException.cs
- WebResourceAttribute.cs
- GetWinFXPath.cs
- Splitter.cs
- UserValidatedEventArgs.cs
- XmlnsCache.cs
- SQLInt16Storage.cs
- DecimalKeyFrameCollection.cs
- ExceptionUtil.cs
- SQLUtility.cs
- MethodInfo.cs
- FreezableCollection.cs
- Error.cs
- MimeWriter.cs
- DocumentPageViewAutomationPeer.cs
- EntityContainerEmitter.cs
- TextBoxLine.cs
- uribuilder.cs
- LogLogRecordHeader.cs
- MailMessageEventArgs.cs
- TemplateKey.cs
- EntityViewGenerator.cs
- DelegatingConfigHost.cs
- StrokeNode.cs
- SystemKeyConverter.cs
- TerminateWorkflow.cs
- GenericAuthenticationEventArgs.cs
- SecurityRuntime.cs
- ConnectionDemuxer.cs
- LightweightEntityWrapper.cs
- NonVisualControlAttribute.cs
- ClassData.cs
- Rotation3D.cs
- FormParameter.cs
- EventWaitHandleSecurity.cs
- HttpsHostedTransportConfiguration.cs
- RectKeyFrameCollection.cs
- PrefixHandle.cs
- TableCellAutomationPeer.cs
- Interlocked.cs
- DateTimeConverter2.cs
- SqlDataSourceFilteringEventArgs.cs
- RegexReplacement.cs
- PocoPropertyAccessorStrategy.cs
- SHA1Managed.cs
- WCFBuildProvider.cs
- HeaderFilter.cs
- GridViewDeletedEventArgs.cs
- SpecularMaterial.cs
- ListViewGroup.cs
- SettingsBase.cs
- ListBoxItemWrapperAutomationPeer.cs
- VisualStyleInformation.cs
- ConfigXmlElement.cs
- FixedPage.cs
- PropertyOverridesTypeEditor.cs
- DesignerExtenders.cs
- WebPartCatalogCloseVerb.cs
- ContentType.cs
- PictureBox.cs