Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / System / Windows / Data / BindingBase.cs / 1305600 / BindingBase.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: Defines BindingBase object, common base class for Binding, // PriorityBinding, MultiBinding. // // See spec at http://avalon/connecteddata/Specs/Data%20Binding.mht // //--------------------------------------------------------------------------- using System; using System.Collections.ObjectModel; // Collectionusing System.ComponentModel; // [DefaultValue] using System.Diagnostics; // Debug.Assert using System.Globalization; // CultureInfo using System.Windows.Markup; // MarkupExtension using System.Windows.Controls; // ValidationRule using MS.Internal; // Helper namespace System.Windows.Data { /// This enum describes how the data flows through a given Binding /// public enum BindingMode { ///Data flows from source to target and vice-versa TwoWay, ///Data flows from source to target, source changes cause data flow OneWay, ///Data flows from source to target once, source changes are ignored OneTime, ///Data flows from target to source, target changes cause data flow OneWayToSource, ///Data flow is obtained from target property default Default } ///This enum describes when updates (target-to-source data flow) /// happen in a given Binding. /// public enum UpdateSourceTrigger { ///Obtain trigger from target property default Default, ///Update whenever the target property changes PropertyChanged, ///Update only when target element loses focus, or when Binding deactivates LostFocus, ///Update only by explicit call to BindingExpression.UpdateSource() Explicit } ////// Base class for Binding, PriorityBinding, and MultiBinding. /// [MarkupExtensionReturnType(typeof(object))] [Localizability(LocalizationCategory.None, Modifiability = Modifiability.Unmodifiable, Readability = Readability.Unreadable)] // Not localizable by-default public abstract class BindingBase: MarkupExtension { #region Constructors //----------------------------------------------------- // // Constructors // //----------------------------------------------------- ///Initialize a new instance of BindingBase. ////// This constructor can only be called by one of the built-in /// derived classes. /// internal BindingBase() { } #endregion Constructors #region Public Properties //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- ///Value to use when source cannot provide a value ////// Initialized to DependencyProperty.UnsetValue; if FallbackValue is not set, BindingExpression /// will return target property's default when Binding cannot get a real value. /// public object FallbackValue { get { return _fallbackValue; } set { CheckSealed(); _fallbackValue = value; } } ////// This method is used by TypeDescriptor to determine if this property should /// be serialized. /// [System.ComponentModel.EditorBrowsable(System.ComponentModel.EditorBrowsableState.Never)] public bool ShouldSerializeFallbackValue() { return _fallbackValue != DependencyProperty.UnsetValue; } ///Format string used to convert the data to type String. /// ////// This property is used when the target of the binding has type /// String and no Converter is declared. It is ignored in all other /// cases. /// [System.ComponentModel.DefaultValue(null)] public string StringFormat { get { return _stringFormat; } set { CheckSealed(); _stringFormat = value; } } ///Value used to represent "null" in the target property. /// public object TargetNullValue { get { return _targetNullValue; } set { CheckSealed(); _targetNullValue = value; } } ////// This method is used by TypeDescriptor to determine if this property should /// be serialized. /// [System.ComponentModel.EditorBrowsable(System.ComponentModel.EditorBrowsableState.Never)] public bool ShouldSerializeTargetNullValue() { return _targetNullValue != DependencyProperty.UnsetValue; } ///Name of the [DefaultValue("")] public string BindingGroupName { get { return _bindingGroupName; } set { CheckSealed(); _bindingGroupName = value; } } #endregion Public Properties #region Public Methods //------------------------------------------------------ // // MarkupExtension overrides // //------------------------------------------------------ ///this binding should join. /// /// Return the value to set on the property for the target for this /// binding. /// public sealed override object ProvideValue(IServiceProvider serviceProvider) { // Binding a property value only works on DependencyObject and DependencyProperties. // For all other cases, just return this Binding object as the value. if (serviceProvider == null) { return this; } IProvideValueTarget provideValueTarget = serviceProvider.GetService( typeof(IProvideValueTarget) ) as IProvideValueTarget; // Bindings are not allowed On CLR props except for Setter,Trigger,Condition (bugs 1183373,1572537) DependencyObject targetDependencyObject; DependencyProperty targetDependencyProperty; Helper.CheckCanReceiveMarkupExtension(this, provideValueTarget, out targetDependencyObject, out targetDependencyProperty); if (targetDependencyObject == null || targetDependencyProperty == null) { return this; } // delegate real work to subclass return CreateBindingExpression(targetDependencyObject, targetDependencyProperty); } //----------------------------------------------------- // // Public Methods // //------------------------------------------------------ #endregion Public Methods #region Protected Enums //----------------------------------------------------- // // Protected Enums // //----------------------------------------------------- ///Flags indicating special properties of a Binding. [Flags] internal enum BindingFlags { ///Data flows from source to target (only) OneWay = BindingExpressionBase.BindingFlags.OneWay, ///Data flows in both directions - source to target and vice-versa TwoWay = BindingExpressionBase.BindingFlags.TwoWay, ///Data flows from target to source (only) OneWayToSource = BindingExpressionBase.BindingFlags.OneWayToSource, ///Target is initialized from the source (only) OneTime = BindingExpressionBase.BindingFlags.OneTime, ///Data flow obtained from target property default PropDefault = BindingExpressionBase.BindingFlags.PropDefault, ///Raise TargetUpdated event whenever a value flows from source to target NotifyOnTargetUpdated = BindingExpressionBase.BindingFlags.NotifyOnTargetUpdated, ///Raise SourceUpdated event whenever a value flows from target to source NotifyOnSourceUpdated = BindingExpressionBase.BindingFlags.NotifyOnSourceUpdated, ///Raise ValidationError event whenever there is a ValidationError on Update NotifyOnValidationError = BindingExpressionBase.BindingFlags.NotifyOnValidationError, ///Obtain trigger from target property default UpdateDefault = BindingExpressionBase.BindingFlags.UpdateDefault, ///Update the source value whenever the target value changes UpdateOnPropertyChanged = BindingExpressionBase.BindingFlags.UpdateOnPropertyChanged, ///Update the source value whenever the target element loses focus UpdateOnLostFocus = BindingExpressionBase.BindingFlags.UpdateOnLostFocus, ///Update the source value only when explicitly told to do so UpdateExplicitly = BindingExpressionBase.BindingFlags.UpdateExplicitly, ////// Used to determine whether the Path was internally Generated (such as the implicit /// /InnerText from an XPath). If it is, then it doesn't need to be serialized. /// PathGeneratedInternally = BindingExpressionBase.BindingFlags.PathGeneratedInternally, ValidatesOnExceptions = BindingExpressionBase.BindingFlags.ValidatesOnExceptions, ValidatesOnDataErrors = BindingExpressionBase.BindingFlags.ValidatesOnDataErrors, ///Flags describing data transfer PropagationMask = OneWay | TwoWay | OneWayToSource | OneTime | PropDefault, ///Flags describing update trigger UpdateMask = UpdateDefault | UpdateOnPropertyChanged | UpdateOnLostFocus | UpdateExplicitly, ///Default value, namely (PropDefault | UpdateDefault) Default = BindingExpressionBase.BindingFlags.Default, ///Error value, returned by FlagsFrom to indicate faulty input IllegalInput = BindingExpressionBase.BindingFlags.IllegalInput, } #endregion Protected Enums #region Protected Methods //----------------------------------------------------- // // Protected Methods // //------------------------------------------------------ ////// Create an appropriate expression for this Binding, to be attached /// to the given DependencyProperty on the given DependencyObject. /// internal abstract BindingExpressionBase CreateBindingExpressionOverride(DependencyObject targetObject, DependencyProperty targetProperty, BindingExpressionBase owner); ///Return true if any of the given flags are set. internal bool TestFlag(BindingFlags flag) { return (_flags & flag) != 0; } ///Set the given flags. internal void SetFlag(BindingFlags flag) { _flags |= flag; } ///Clear the given flags. internal void ClearFlag(BindingFlags flag) { _flags &= ~flag; } ///Change the given flags to have the given value. internal void ChangeFlag(BindingFlags flag, bool value) { if (value) _flags |= flag; else _flags &= ~flag; } ///Get the flags within the given mas. internal BindingFlags GetFlagsWithinMask(BindingFlags mask) { return (_flags & mask); } ///Change the flags within the given mask to have the given value. internal void ChangeFlagsWithinMask(BindingFlags mask, BindingFlags flags) { _flags = (_flags & ~mask) | (flags & mask); } ///Convert the given BindingMode to BindingFlags. internal static BindingFlags FlagsFrom(BindingMode bindingMode) { switch (bindingMode) { case BindingMode.OneWay: return BindingFlags.OneWay; case BindingMode.TwoWay: return BindingFlags.TwoWay; case BindingMode.OneWayToSource: return BindingFlags.OneWayToSource; case BindingMode.OneTime: return BindingFlags.OneTime; case BindingMode.Default: return BindingFlags.PropDefault; } return BindingFlags.IllegalInput; } ///Convert the given UpdateSourceTrigger to BindingFlags. internal static BindingFlags FlagsFrom(UpdateSourceTrigger updateSourceTrigger) { switch (updateSourceTrigger) { case UpdateSourceTrigger.Default: return BindingFlags.UpdateDefault; case UpdateSourceTrigger.PropertyChanged: return BindingFlags.UpdateOnPropertyChanged; case UpdateSourceTrigger.LostFocus: return BindingFlags.UpdateOnLostFocus; case UpdateSourceTrigger.Explicit: return BindingFlags.UpdateExplicitly; } return BindingFlags.IllegalInput; } #endregion Protected Methods #region Internal Properties //----------------------------------------------------- // // Internal Properties // //------------------------------------------------------ internal BindingFlags Flags { get { return _flags; } } internal virtual CultureInfo ConverterCultureInternal { get { return null; } } internal virtual CollectionValidationRulesInternal { get { return null; } } #endregion Internal Properties #region Internal Methods //------------------------------------------------------ // // Internal Methods // //----------------------------------------------------- /// /// Create an appropriate expression for this Binding, to be attached /// to the given DependencyProperty on the given DependencyObject. /// internal BindingExpressionBase CreateBindingExpression(DependencyObject targetObject, DependencyProperty targetProperty) { _isSealed = true; return CreateBindingExpressionOverride(targetObject, targetProperty, null); } ////// Create an appropriate expression for this Binding, to be attached /// to the given DependencyProperty on the given DependencyObject. /// internal BindingExpressionBase CreateBindingExpression(DependencyObject targetObject, DependencyProperty targetProperty, BindingExpressionBase owner) { _isSealed = true; return CreateBindingExpressionOverride(targetObject, targetProperty, owner); } // Throw if the binding is sealed. internal void CheckSealed() { if (_isSealed) throw new InvalidOperationException(SR.Get(SRID.ChangeSealedBinding)); } // Return one of the special ValidationRules internal ValidationRule GetValidationRule(Type type) { if (TestFlag(BindingFlags.ValidatesOnExceptions) && type == typeof(System.Windows.Controls.ExceptionValidationRule)) return System.Windows.Controls.ExceptionValidationRule.Instance; if (TestFlag(BindingFlags.ValidatesOnDataErrors) && type == typeof(System.Windows.Controls.DataErrorValidationRule)) return System.Windows.Controls.DataErrorValidationRule.Instance; return LookupValidationRule(type); } internal virtual ValidationRule LookupValidationRule(Type type) { return null; } internal static ValidationRule LookupValidationRule(Type type, Collectioncollection) { if (collection == null) return null; for (int i=0; i
Link Menu
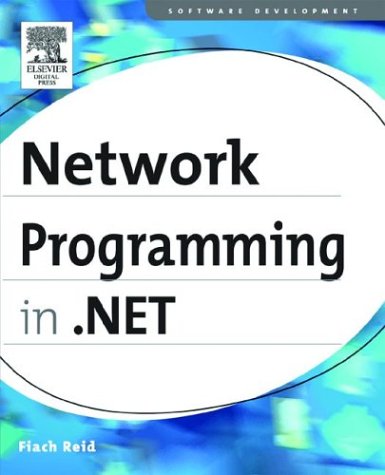
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- BuildProvidersCompiler.cs
- RIPEMD160.cs
- HttpStaticObjectsCollectionWrapper.cs
- StringArrayConverter.cs
- SafeMILHandle.cs
- QueryableDataSourceEditData.cs
- ServiceBusyException.cs
- DispatcherOperation.cs
- InProcStateClientManager.cs
- SubclassTypeValidatorAttribute.cs
- XmlNodeChangedEventArgs.cs
- Effect.cs
- FixedDSBuilder.cs
- AxHost.cs
- SocketException.cs
- StructuredType.cs
- DataBoundControl.cs
- GrammarBuilderRuleRef.cs
- KnownIds.cs
- OrthographicCamera.cs
- DBCommand.cs
- autovalidator.cs
- WebPartConnectionsConfigureVerb.cs
- ConfigurationElementProperty.cs
- BitmapPalettes.cs
- Keyboard.cs
- CodeDirectoryCompiler.cs
- FlowchartStart.xaml.cs
- ExternalException.cs
- FillBehavior.cs
- SymbolTable.cs
- Image.cs
- HtmlFormAdapter.cs
- NonClientArea.cs
- InfoCardRSAPKCS1KeyExchangeFormatter.cs
- SupportingTokenDuplexChannel.cs
- IfJoinedCondition.cs
- DrawingAttributes.cs
- WebDescriptionAttribute.cs
- XmlCustomFormatter.cs
- ScrollViewerAutomationPeer.cs
- ValidatingPropertiesEventArgs.cs
- XamlVector3DCollectionSerializer.cs
- InstanceHandleConflictException.cs
- TypeDescriptor.cs
- WorkflowMarkupSerializerMapping.cs
- QualifierSet.cs
- ServicePointManagerElement.cs
- InfoCardHelper.cs
- SchemaElement.cs
- ADConnectionHelper.cs
- SqlDataSourceSelectingEventArgs.cs
- MultiPageTextView.cs
- LicenseProviderAttribute.cs
- DbMetaDataColumnNames.cs
- SaveRecipientRequest.cs
- MediaElement.cs
- XmlSchemaException.cs
- BooleanFunctions.cs
- BamlLocalizabilityResolver.cs
- ControlBuilderAttribute.cs
- ToolStripContextMenu.cs
- StrongTypingException.cs
- AdapterDictionary.cs
- Pen.cs
- FormViewUpdateEventArgs.cs
- FontCollection.cs
- ConstructorNeedsTagAttribute.cs
- OrderedParallelQuery.cs
- EventDescriptor.cs
- LinearGradientBrush.cs
- IOException.cs
- ToolStripDropDownClosedEventArgs.cs
- ImmutableObjectAttribute.cs
- XmlReflectionImporter.cs
- GB18030Encoding.cs
- TextServicesProperty.cs
- FontStyles.cs
- DemultiplexingClientMessageFormatter.cs
- HtmlElementErrorEventArgs.cs
- DescendantQuery.cs
- InfoCardRSAPKCS1SignatureDeformatter.cs
- DataTemplateSelector.cs
- Identity.cs
- XmlSchemaIdentityConstraint.cs
- TextReader.cs
- CacheAxisQuery.cs
- ToolboxItemAttribute.cs
- RegexMatch.cs
- IfElseDesigner.xaml.cs
- TextFormatterContext.cs
- HostAdapter.cs
- MultiSelector.cs
- HtmlEncodedRawTextWriter.cs
- CurrentChangingEventArgs.cs
- ObjectViewFactory.cs
- DataSourceCache.cs
- DesignUtil.cs
- PassportAuthenticationModule.cs
- SmiSettersStream.cs