Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Core / System / Windows / Media / Generated / RectangleGeometry.cs / 2 / RectangleGeometry.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see [....]/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.KnownBoxes; using MS.Internal.Collections; using MS.Internal.PresentationCore; using MS.Utility; using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Runtime.InteropServices; using System.ComponentModel.Design.Serialization; using System.Text; using System.Windows; using System.Windows.Media; using System.Windows.Media.Effects; using System.Windows.Media.Media3D; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Windows.Media.Imaging; using System.Windows.Markup; using System.Windows.Media.Converters; using System.Security; using System.Security.Permissions; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; // These types are aliased to match the unamanaged names used in interop using BOOL = System.UInt32; using WORD = System.UInt16; using Float = System.Single; namespace System.Windows.Media { sealed partial class RectangleGeometry : Geometry { #region Constructors //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #endregion Constructors //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Shadows inherited Clone() with a strongly typed /// version for convenience. /// public new RectangleGeometry Clone() { return (RectangleGeometry)base.Clone(); } ////// Shadows inherited CloneCurrentValue() with a strongly typed /// version for convenience. /// public new RectangleGeometry CloneCurrentValue() { return (RectangleGeometry)base.CloneCurrentValue(); } #endregion Public Methods //------------------------------------------------------ // // Public Properties // //------------------------------------------------------ private static void RadiusXPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { RectangleGeometry target = ((RectangleGeometry) d); target.PropertyChanged(RadiusXProperty); } private static void RadiusYPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { RectangleGeometry target = ((RectangleGeometry) d); target.PropertyChanged(RadiusYProperty); } private static void RectPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { RectangleGeometry target = ((RectangleGeometry) d); target.PropertyChanged(RectProperty); } #region Public Properties ////// RadiusX - double. Default value is 0.0. /// public double RadiusX { get { return (double) GetValue(RadiusXProperty); } set { SetValueInternal(RadiusXProperty, value); } } ////// RadiusY - double. Default value is 0.0. /// public double RadiusY { get { return (double) GetValue(RadiusYProperty); } set { SetValueInternal(RadiusYProperty, value); } } ////// Rect - Rect. Default value is Rect.Empty. /// public Rect Rect { get { return (Rect) GetValue(RectProperty); } set { SetValueInternal(RectProperty, value); } } #endregion Public Properties //----------------------------------------------------- // // Protected Methods // //------------------------------------------------------ #region Protected Methods ////// Implementation of ///Freezable.CreateInstanceCore . ///The new Freezable. protected override Freezable CreateInstanceCore() { return new RectangleGeometry(); } #endregion ProtectedMethods //----------------------------------------------------- // // Internal Methods // //----------------------------------------------------- #region Internal Methods ////// Critical: This code calls into an unsafe code block /// TreatAsSafe: This code does not return any critical data.It is ok to expose /// Channels are safe to call into and do not go cross domain and cross process /// [SecurityCritical,SecurityTreatAsSafe] internal override void UpdateResource(DUCE.Channel channel, bool skipOnChannelCheck) { // If we're told we can skip the channel check, then we must be on channel Debug.Assert(!skipOnChannelCheck || _duceResource.IsOnChannel(channel)); if (skipOnChannelCheck || _duceResource.IsOnChannel(channel)) { base.UpdateResource(channel, skipOnChannelCheck); // Read values of properties into local variables Transform vTransform = Transform; // Obtain handles for properties that implement DUCE.IResource DUCE.ResourceHandle hTransform; if (vTransform == null || Object.ReferenceEquals(vTransform, Transform.Identity) ) { hTransform = DUCE.ResourceHandle.Null; } else { hTransform = ((DUCE.IResource)vTransform).GetHandle(channel); } // Obtain handles for animated properties DUCE.ResourceHandle hRadiusXAnimations = GetAnimationResourceHandle(RadiusXProperty, channel); DUCE.ResourceHandle hRadiusYAnimations = GetAnimationResourceHandle(RadiusYProperty, channel); DUCE.ResourceHandle hRectAnimations = GetAnimationResourceHandle(RectProperty, channel); // Pack & send command packet DUCE.MILCMD_RECTANGLEGEOMETRY data; unsafe { data.Type = MILCMD.MilCmdRectangleGeometry; data.Handle = _duceResource.GetHandle(channel); data.hTransform = hTransform; if (hRadiusXAnimations.IsNull) { data.RadiusX = RadiusX; } data.hRadiusXAnimations = hRadiusXAnimations; if (hRadiusYAnimations.IsNull) { data.RadiusY = RadiusY; } data.hRadiusYAnimations = hRadiusYAnimations; if (hRectAnimations.IsNull) { data.Rect = Rect; } data.hRectAnimations = hRectAnimations; // Send packed command structure channel.SendCommand( (byte*)&data, sizeof(DUCE.MILCMD_RECTANGLEGEOMETRY)); } } } internal override DUCE.ResourceHandle AddRefOnChannelCore(DUCE.Channel channel) { if (_duceResource.CreateOrAddRefOnChannel(channel, System.Windows.Media.Composition.DUCE.ResourceType.TYPE_RECTANGLEGEOMETRY)) { Transform vTransform = Transform; if (vTransform != null) ((DUCE.IResource)vTransform).AddRefOnChannel(channel); AddRefOnChannelAnimations(channel); UpdateResource(channel, true /* skip "on channel" check - we already know that we're on channel */ ); } return _duceResource.GetHandle(channel); } internal override void ReleaseOnChannelCore(DUCE.Channel channel) { Debug.Assert(_duceResource.IsOnChannel(channel)); if (_duceResource.ReleaseOnChannel(channel)) { Transform vTransform = Transform; if (vTransform != null) ((DUCE.IResource)vTransform).ReleaseOnChannel(channel); ReleaseOnChannelAnimations(channel); } } internal override DUCE.ResourceHandle GetHandleCore(DUCE.Channel channel) { // Note that we are in a lock here already. return _duceResource.GetHandle(channel); } internal override int GetChannelCountCore() { // must already be in composition lock here return _duceResource.GetChannelCount(); } internal override DUCE.Channel GetChannelCore(int index) { // Note that we are in a lock here already. return _duceResource.GetChannel(index); } #endregion Internal Methods //----------------------------------------------------- // // Internal Properties // //------------------------------------------------------ #region Internal Properties // // This property finds the correct initial size for the _effectiveValues store on the // current DependencyObject as a performance optimization // // This includes: // Rect // internal override int EffectiveValuesInitialSize { get { return 1; } } #endregion Internal Properties //----------------------------------------------------- // // Dependency Properties // //------------------------------------------------------ #region Dependency Properties ////// The DependencyProperty for the RectangleGeometry.RadiusX property. /// public static readonly DependencyProperty RadiusXProperty = RegisterProperty("RadiusX", typeof(double), typeof(RectangleGeometry), 0.0, new PropertyChangedCallback(RadiusXPropertyChanged), null, /* isIndependentlyAnimated = */ true, /* coerceValueCallback */ null); ////// The DependencyProperty for the RectangleGeometry.RadiusY property. /// public static readonly DependencyProperty RadiusYProperty = RegisterProperty("RadiusY", typeof(double), typeof(RectangleGeometry), 0.0, new PropertyChangedCallback(RadiusYPropertyChanged), null, /* isIndependentlyAnimated = */ true, /* coerceValueCallback */ null); ////// The DependencyProperty for the RectangleGeometry.Rect property. /// public static readonly DependencyProperty RectProperty = RegisterProperty("Rect", typeof(Rect), typeof(RectangleGeometry), Rect.Empty, new PropertyChangedCallback(RectPropertyChanged), null, /* isIndependentlyAnimated = */ true, /* coerceValueCallback */ null); #endregion Dependency Properties //------------------------------------------------------ // // Internal Fields // //----------------------------------------------------- #region Internal Fields internal System.Windows.Media.Composition.DUCE.MultiChannelResource _duceResource = new System.Windows.Media.Composition.DUCE.MultiChannelResource(); internal const double c_RadiusX = 0.0; internal const double c_RadiusY = 0.0; internal static Rect s_Rect = Rect.Empty; #endregion Internal Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
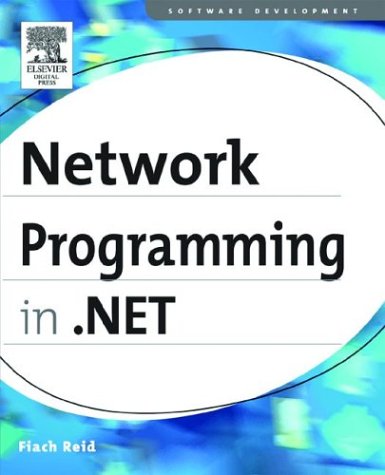
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- NativeCompoundFileAPIs.cs
- SafeNativeMethods.cs
- RegionInfo.cs
- BaseTreeIterator.cs
- OleDbConnectionInternal.cs
- StringConcat.cs
- SafePointer.cs
- DataGridViewCellStyleConverter.cs
- ShaderEffect.cs
- WindowsAuthenticationEventArgs.cs
- SafeArrayRankMismatchException.cs
- DataSourceView.cs
- HandlerBase.cs
- CriticalExceptions.cs
- X509Certificate.cs
- SecondaryIndexDefinition.cs
- Expressions.cs
- SpotLight.cs
- SafeLibraryHandle.cs
- SiteMapHierarchicalDataSourceView.cs
- _NestedSingleAsyncResult.cs
- OrderByBuilder.cs
- TypeLibConverter.cs
- TableStyle.cs
- DataGridViewColumnEventArgs.cs
- _AutoWebProxyScriptWrapper.cs
- PinnedBufferMemoryStream.cs
- AlternateView.cs
- ModelPropertyCollectionImpl.cs
- Visitors.cs
- TypedTableBaseExtensions.cs
- ReachBasicContext.cs
- ScriptControl.cs
- ThrowHelper.cs
- XhtmlConformanceSection.cs
- AudioException.cs
- SystemWebSectionGroup.cs
- EntityCommand.cs
- DataGridViewAutoSizeColumnsModeEventArgs.cs
- SqlFunctionAttribute.cs
- DoubleLinkList.cs
- DragStartedEventArgs.cs
- StrongBox.cs
- FileUpload.cs
- SmtpMail.cs
- OperationAbortedException.cs
- TabControlAutomationPeer.cs
- ImageCollectionEditor.cs
- EditCommandColumn.cs
- DynamicMetaObject.cs
- Processor.cs
- AudioException.cs
- TypeNameConverter.cs
- DateRangeEvent.cs
- HttpContext.cs
- SafeFileMapViewHandle.cs
- SiteMapNodeItemEventArgs.cs
- ModelPropertyCollectionImpl.cs
- ValidationHelper.cs
- PtsHost.cs
- PageRanges.cs
- DbConnectionPoolCounters.cs
- GeneralTransformGroup.cs
- SoapSchemaMember.cs
- PackageStore.cs
- CancellationState.cs
- WindowsImpersonationContext.cs
- ProfileGroupSettingsCollection.cs
- AssemblyAttributes.cs
- SelectionRange.cs
- TextEditorCharacters.cs
- UserControlAutomationPeer.cs
- ThreadAttributes.cs
- ObjectDataSourceChooseMethodsPanel.cs
- Tablet.cs
- FixedStringLookup.cs
- ToolStripItemCollection.cs
- TypeExtensionConverter.cs
- ProgressBarHighlightConverter.cs
- ProvidersHelper.cs
- BuiltInExpr.cs
- SqlDuplicator.cs
- BindingCompleteEventArgs.cs
- RepeaterDataBoundAdapter.cs
- SQLInt16.cs
- DesignSurfaceEvent.cs
- MappingModelBuildProvider.cs
- Misc.cs
- DbConnectionFactory.cs
- SafeProcessHandle.cs
- InfoCardTrace.cs
- DataGridViewSortCompareEventArgs.cs
- CollectionCodeDomSerializer.cs
- WorkflowEventArgs.cs
- DataGridTable.cs
- PropertyTabAttribute.cs
- KeyInstance.cs
- DbProviderManifest.cs
- InternalConfigEventArgs.cs
- EventTrigger.cs