Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Print / Reach / PrintConfig / PTManager.cs / 1 / PTManager.cs
/*++ Copyright (C) 2002 Microsoft Corporation All rights reserved. Module Name: PTManager.cs Abstract: Definition and implementation of the internal PrintTicketManager class. This class should only be internally used by printing LAPI. Application's access to PrintTicket and PrintCapabilities functions should be via printing LAPI. Author: [....] ([....]) 11/22/2002 --*/ using System; using System.IO; using System.Collections.Specialized; using System.Runtime.InteropServices; using System.Globalization; using System.Printing; using System.Printing.Interop; using MS.Internal.Printing.Configuration; using System.Windows.Xps.Serialization; // for Toolbox [assembly:CLSCompliant(true)] //[assembly:ComVisible(false)] namespace System.Printing { #region Public Types ////// Possible cases of conflict resolution result of PrintTicket validation. /// [ComVisible(false)] public enum ConflictStatus { ////// PrintTicket validation hasn't found any conflict. /// NoConflict = 0, ////// PrintTicket validation has found and resolved conflict(s). /// ConflictResolved = 1 }; ////// Struct that contains PrintTicket validation result. /// [ComVisible(false)] public struct ValidationResult { // We don't override Object.Equals and the equality operator here since we don't // expect this value type to be compared with each other by clients. #region Constructors ////// Constructor. It's internal so client won't be able to construct one. /// /// resulting PrintTicket stream of the validation /// conflict resolution result of PrintTicket validation internal ValidationResult(MemoryStream validatedPrintTicketStream, ConflictStatus conflictStatus) { _ptStream = validatedPrintTicketStream; _status = conflictStatus; _printTicket = null; } #endregion Constructors #region Public Properties ////// Resulting PrintTicket of the validation. /// public PrintTicket ValidatedPrintTicket { get { if (_printTicket == null) { _printTicket = new PrintTicket(_ptStream); } return _printTicket; } } ////// Conflict resolution result of the PrintTicket validation. /// public ConflictStatus ConflictStatus { get { return _status; } } #endregion Public Properties #region Private Fields private MemoryStream _ptStream; private ConflictStatus _status; private PrintTicket _printTicket; #endregion Private Fields }; ////// PrintTicket keyword scoping prefix. /// [ComVisible(false)] public enum PrintTicketScope { ////// Scope for keywords with "Page" prefix. /// PageScope = 0, ////// Scope for keywords with "Document" prefix. /// DocumentScope = 1, ////// Scope for keywords with "Job" prefix. /// JobScope = 2, } #endregion Public Types #region PrintTicketManager class ////// PrintTicketManager class that supports PrintTicket and PrintCapabilities functions. /// [MS.Internal.ReachFramework.FriendAccessAllowed] internal class PrintTicketManager : IDisposable { #region Constructors ////// Constructs a new PrintTicketManager instance for the given device. /// /// Name of printer device the PrintTicketManager instance should be bound to. /// Print Schema version requested by client. ////// The ///parameter is null. /// /// The ///parameter value is not greater than 0 /// or is greater than the maximum Print Schema version /// PrintTicketManager can support. /// /// The PrintTicketManager instance failed to bind to the printer specified by public PrintTicketManager(string deviceName, int clientPrintSchemaVersion) { // Check input argument if (deviceName == null) { throw new ArgumentNullException("deviceName"); } // Check if we can support the schema version client has requested if ((clientPrintSchemaVersion > MaxPrintSchemaVersion) || (clientPrintSchemaVersion <= 0)) { throw new ArgumentOutOfRangeException("clientPrintSchemaVersion"); } // Instantiate a new PTProvider instance. PTProvider constructor throws exception if it fails for any reason. _ptProvider = new PTProvider(deviceName, MaxPrintSchemaVersion, clientPrintSchemaVersion); } #endregion Constructors #region Public Properties ///. /// /// Gets the maximum Print Schema version PrintTicketManager can support. /// public static int MaxPrintSchemaVersion { get { return _maxPrintSchemaVersion; } } #endregion Public Properties #region Public Methods ////// Gets the PrintCapabilities relative to the given PrintTicket. /// /// The PrintTicket object based on which PrintCapabilities should be built. ///The PrintCapabilities object. ////// The ///parameter could be null, in which case /// the device's default PrintTicket will be used to construct the PrintCapabilities. /// /// The PrintTicketManager instance has already been disposed. /// ////// The input PrintTicket specified by ///is not well-formed. /// /// The PrintTicketManager instance failed to retrieve the PrintCapabilities. /// public PrintCapabilities GetPrintCapabilities(PrintTicket printTicket) { MemoryStream pcStream = GetPrintCapabilitiesAsXml(printTicket); PrintCapabilities retCap = new PrintCapabilities(pcStream); pcStream.Close(); return retCap; } ////// Gets the PrintCapabilities (in XML form) relative to the given PrintTicket. /// /// The PrintTicket object based on which PrintCapabilities should be built. ///MemoryStream that contains XML PrintCapabilities. ////// The ///parameter could be null, in which case /// the device's default PrintTicket will be used to construct the PrintCapabilities. /// /// The PrintTicketManager instance has already been disposed. /// ////// The input PrintTicket specified by ///is not well-formed. /// /// The PrintTicketManager instance failed to retrieve the PrintCapabilities. /// public MemoryStream GetPrintCapabilitiesAsXml(PrintTicket printTicket) { Toolbox.StartEvent(Toolbox.DRXGETPRINTCAPGUID); if (_disposed) { throw new ObjectDisposedException("PrintTicketManager"); } MemoryStream ptStream = null; if (printTicket != null) { ptStream = printTicket.GetXmlStream(); } MemoryStream pcStream = _ptProvider.GetPrintCapabilities(ptStream); Toolbox.EndEvent(Toolbox.DRXGETPRINTCAPGUID); return pcStream; } ////// Merges delta PrintTicket with base PrintTicket and then validates the merged PrintTicket. /// /// The base PrintTicket. /// The delta PrintTicket. ///Struct that contains PrintTicket validation result info. ////// The ///parameter could be null, in which case /// only validation will be performed on . /// /// The PrintTicketManager instance has already been disposed. /// ////// The ///parameter is null. /// /// The base PrintTicket specified by ///is not well-formed, /// or delta PrintTicket specified by is not well-formed. /// /// The PrintTicketManager instance failed to merge and validate the input PrintTicket(s). /// public ValidationResult MergeAndValidatePrintTicket(PrintTicket basePrintTicket, PrintTicket deltaPrintTicket) { return MergeAndValidatePrintTicket(basePrintTicket, deltaPrintTicket, PrintTicketScope.JobScope); } ////// Merges delta PrintTicket with base PrintTicket and then validates the merged PrintTicket. /// /// The base PrintTicket. /// The delta PrintTicket. /// scope that delta PrintTicket and result PrintTicket will be limited to ///Struct that contains PrintTicket validation result info. ////// The ///parameter could be null, in which case /// only validation will be performed on . /// /// The PrintTicketManager instance has already been disposed. /// ////// The ///parameter is null. /// /// The ///parameter is not one of the standard values. /// /// The base PrintTicket specified by ///is not well-formed, /// or delta PrintTicket specified by is not well-formed. /// /// The PrintTicketManager instance failed to merge and validate the input PrintTicket(s). /// public ValidationResult MergeAndValidatePrintTicket(PrintTicket basePrintTicket, PrintTicket deltaPrintTicket, PrintTicketScope scope) { if (_disposed) { throw new ObjectDisposedException("PrintTicketManager"); } // Base PrintTicket is required. Delta PrintTicket is optional. if (basePrintTicket == null) { throw new ArgumentNullException("basePrintTicket"); } // validate scope value if ((scope != PrintTicketScope.PageScope) && (scope != PrintTicketScope.DocumentScope) && (scope != PrintTicketScope.JobScope)) { throw new ArgumentOutOfRangeException("scope"); } MemoryStream baseStream = null, deltaStream = null, resultStream = null; ConflictStatus status; baseStream = basePrintTicket.GetXmlStream(); if (deltaPrintTicket != null) { deltaStream = deltaPrintTicket.GetXmlStream(); } resultStream = _ptProvider.MergeAndValidatePrintTicket(baseStream, deltaStream, scope, out status); return new ValidationResult(resultStream, status); } ////// Converts the given Win32 DEVMODE into PrintTicket. /// /// Byte buffer containing the Win32 DEVMODE. ///The converted PrintTicket object. public PrintTicket ConvertDevModeToPrintTicket(byte[] devMode) { if (_disposed) { throw new ObjectDisposedException("PrintTicketManager"); } return PrintTicketConverter.InternalConvertDevModeToPrintTicket(_ptProvider, devMode, PrintTicketScope.JobScope); } ////// Converts the given PrintTicket into Win32 DEVMODE. /// /// The PrintTicket to be converted. /// Type of default DEVMODE to use as base of conversion. ///Byte buffer that contains the converted Win32 DEVMODE. public byte[] ConvertPrintTicketToDevMode(PrintTicket printTicket, BaseDevModeType baseType) { if (_disposed) { throw new ObjectDisposedException("PrintTicketManager"); } return PrintTicketConverter.InternalConvertPrintTicketToDevMode(_ptProvider, printTicket, baseType, PrintTicketScope.JobScope); } #endregion Public Methods // No need to implement the finalizer since we are using SafeHandle to wrap the unmanaged resource. #region IDisposable Members ////// Dispose this PrintTicketManager instance. /// public void Dispose() { Dispose(true); } ////// Dispose this instance. /// protected virtual void Dispose(bool disposing) { if (!this._disposed) { if (disposing) { _ptProvider.Dispose(); _ptProvider = null; } this._disposed = true; } } #endregion #region Private Fields ////// max Print Schema version the manager class can support /// private const int _maxPrintSchemaVersion = 1; ////// PrintTicket provider instance the manager instance is using /// private PTProvider _ptProvider; ////// boolean of whether or not this manager instance is disposed /// private bool _disposed; #endregion Private Fields } #endregion PrintTicketManager class } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
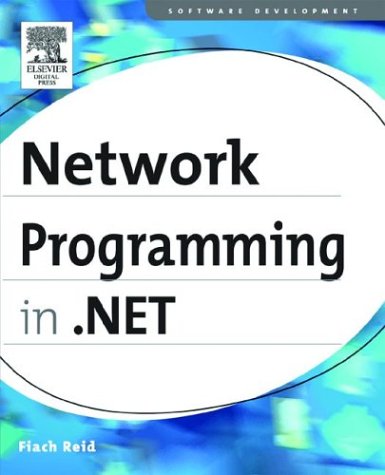
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HashJoinQueryOperatorEnumerator.cs
- EditorBrowsableAttribute.cs
- ListViewItemSelectionChangedEvent.cs
- FamilyTypeface.cs
- InkPresenter.cs
- RealizationDrawingContextWalker.cs
- LogReservationCollection.cs
- ResourceExpression.cs
- SchemaLookupTable.cs
- AttributeCollection.cs
- ServiceMetadataPublishingElement.cs
- ProfileParameter.cs
- autovalidator.cs
- ActivityInterfaces.cs
- validationstate.cs
- TransactionChannel.cs
- MediaSystem.cs
- URL.cs
- ByteStorage.cs
- HtmlCommandAdapter.cs
- EnlistmentTraceIdentifier.cs
- Context.cs
- CorrelationToken.cs
- NamedPipeHostedTransportConfiguration.cs
- NopReturnReader.cs
- TextPointer.cs
- HandlerFactoryCache.cs
- NumericPagerField.cs
- BooleanSwitch.cs
- IPPacketInformation.cs
- SortableBindingList.cs
- StringFunctions.cs
- Storyboard.cs
- FormCollection.cs
- DeflateEmulationStream.cs
- IndentTextWriter.cs
- IriParsingElement.cs
- EntityRecordInfo.cs
- SpecularMaterial.cs
- TreeView.cs
- WizardStepBase.cs
- TimeSpanMinutesOrInfiniteConverter.cs
- DomainUpDown.cs
- UrlPath.cs
- SessionEndingEventArgs.cs
- UInt64Converter.cs
- ToolStripPanelDesigner.cs
- ParentQuery.cs
- LocationUpdates.cs
- TypeConstant.cs
- SweepDirectionValidation.cs
- HttpFormatExtensions.cs
- DataGridItem.cs
- UiaCoreApi.cs
- NativeMethods.cs
- DeadLetterQueue.cs
- WebRequest.cs
- XmlnsCache.cs
- BulletedListDesigner.cs
- DecimalKeyFrameCollection.cs
- MultipleViewProviderWrapper.cs
- ProcessStartInfo.cs
- MemberDescriptor.cs
- EnumerableCollectionView.cs
- PeerApplicationLaunchInfo.cs
- FatalException.cs
- FontStyleConverter.cs
- InfoCardService.cs
- login.cs
- BindStream.cs
- KeysConverter.cs
- EnglishPluralizationService.cs
- DesignTimeVisibleAttribute.cs
- Ops.cs
- GradientStop.cs
- ConvertEvent.cs
- SecureEnvironment.cs
- LinqDataSourceValidationException.cs
- ColorAnimationBase.cs
- Serializer.cs
- assertwrapper.cs
- Privilege.cs
- SoapInteropTypes.cs
- ColorDialog.cs
- XamlPointCollectionSerializer.cs
- EditingScope.cs
- Tuple.cs
- PointAnimationUsingKeyFrames.cs
- DocumentViewer.cs
- NonParentingControl.cs
- MenuCommands.cs
- BrowserDefinition.cs
- ResolveRequestResponseAsyncResult.cs
- AttachedPropertyBrowsableForChildrenAttribute.cs
- PageCatalogPart.cs
- ObjectTypeMapping.cs
- SspiSafeHandles.cs
- FocusTracker.cs
- SignedXml.cs
- GuidelineCollection.cs