Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / xsp / System / Web / UI / AttributeCollection.cs / 1 / AttributeCollection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * AttributeCollection.cs * * Copyright (c) 2000 Microsoft Corporation */ namespace System.Web.UI { using System.IO; using System.Collections; using System.Reflection; using System.Web.UI; using System.Globalization; using System.Security.Permissions; using System.Web.Util; /* * The AttributeCollection represents Attributes on an Html control. */ ////// [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class AttributeCollection { private StateBag _bag; private CssStyleCollection _styleColl; /* * Constructs an AttributeCollection given a StateBag. */ ////// The ///class provides object-model access /// to all attributes declared on an HTML server control element. /// /// public AttributeCollection(StateBag bag) { _bag = bag; } /* * Automatically adds new keys. */ ////// public string this[string key] { get { if (_styleColl != null && StringUtil.EqualsIgnoreCase(key, "style")) return _styleColl.Value; else return _bag[key] as string; } set { Add(key, value); } } /* * Returns a collection of keys. */ ////// Gets or sets a specified attribute value. /// ////// public ICollection Keys { get { return _bag.Keys; } } ////// Gets a collection of keys to all the attributes in the /// ///. /// /// public int Count { get { return _bag.Count; } } ////// Gets the number of items in the ///. /// /// public CssStyleCollection CssStyle { get { if (_styleColl == null) { _styleColl = new CssStyleCollection(_bag); } return _styleColl; } } ////// public void Add(string key, string value) { if (_styleColl != null && StringUtil.EqualsIgnoreCase(key, "style")) _styleColl.Value = value; else _bag[key] = value; } public override bool Equals(object o) { // This implementation of Equals relies on mutable properties and is therefore broken, // but we shipped it this way in V1 so it will be a breaking change to fix it. AttributeCollection attrs = o as AttributeCollection; if (attrs != null) { if (attrs.Count != _bag.Count) { return false; } foreach (DictionaryEntry attr in _bag) { if (this[(string)attr.Key] != attrs[(string)attr.Key]) { return false; } } return true; } return false; } public override int GetHashCode() { // This implementation of GetHashCode uses mutable properties but matches the V1 implementation // of Equals. HashCodeCombiner hashCodeCombiner = new HashCodeCombiner(); foreach (DictionaryEntry attr in _bag) { hashCodeCombiner.AddObject(attr.Key); hashCodeCombiner.AddObject(attr.Value); } return hashCodeCombiner.CombinedHash32; } ////// Adds an item to the ///. /// /// public void Remove(string key) { if (_styleColl != null && StringUtil.EqualsIgnoreCase(key, "style")) _styleColl.Clear(); else _bag.Remove(key); } ////// Removes an attribute from the ///. /// /// public void Clear() { _bag.Clear(); if (_styleColl != null) _styleColl.Clear(); } ////// Removes all attributes from the ///. /// /// public void Render(HtmlTextWriter writer) { if (_bag.Count > 0) { IDictionaryEnumerator e = _bag.GetEnumerator(); while (e.MoveNext()) { StateItem item = e.Value as StateItem; if (item != null) { string value = item.Value as string; string key = e.Key as string; if (key != null && value != null) { writer.WriteAttribute(key, value, true /*fEncode*/); } } } } } ///[To be supplied.] ////// public void AddAttributes(HtmlTextWriter writer) { if (_bag.Count > 0) { IDictionaryEnumerator e = _bag.GetEnumerator(); while (e.MoveNext()) { StateItem item = e.Value as StateItem; if (item != null) { string value = item.Value as string; string key = e.Key as string; if (key != null && value != null) { writer.AddAttribute(key, value, true /*fEncode*/); } } } } } } }[To be supplied.] ///
Link Menu
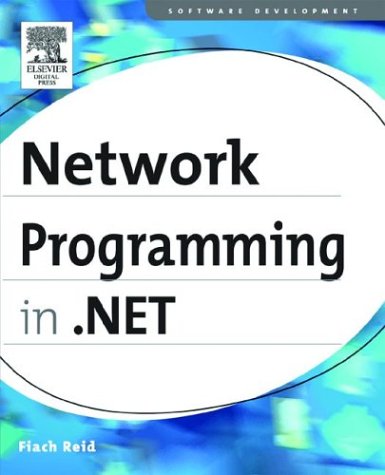
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- EmbeddedMailObject.cs
- AutomationProperty.cs
- ReverseInheritProperty.cs
- Schema.cs
- AsyncContentLoadedEventArgs.cs
- UnknownWrapper.cs
- DetailsViewUpdatedEventArgs.cs
- FlowDocumentPaginator.cs
- CommunicationObjectManager.cs
- LocalsItemDescription.cs
- PropVariant.cs
- RectConverter.cs
- SymmetricAlgorithm.cs
- CompareInfo.cs
- selecteditemcollection.cs
- Privilege.cs
- TimeBoundedCache.cs
- BlockExpression.cs
- NameTable.cs
- HandledEventArgs.cs
- SymmetricCryptoHandle.cs
- XmlSignificantWhitespace.cs
- ScrollChrome.cs
- ServiceHostingEnvironment.cs
- RelationHandler.cs
- XPathPatternBuilder.cs
- QueryResponse.cs
- PageThemeBuildProvider.cs
- IOException.cs
- SettingsContext.cs
- CellQuery.cs
- Attachment.cs
- ServiceReference.cs
- CodeAttributeDeclarationCollection.cs
- SqlCacheDependencyDatabaseCollection.cs
- ResourceManager.cs
- EntityKeyElement.cs
- TypeHelpers.cs
- RawStylusInputCustomData.cs
- ActivityTrace.cs
- EntityDataReader.cs
- SQLGuid.cs
- ErrorProvider.cs
- PackageDigitalSignature.cs
- StructuredType.cs
- TransformCryptoHandle.cs
- FontNamesConverter.cs
- SamlAttribute.cs
- DataGridViewCellFormattingEventArgs.cs
- NumericUpDown.cs
- TraceEventCache.cs
- TypeUtil.cs
- EmptyReadOnlyDictionaryInternal.cs
- StorageEntitySetMapping.cs
- StandardBindingCollectionElement.cs
- OleAutBinder.cs
- XmlSchemaObjectTable.cs
- XPathException.cs
- ExtensionSimplifierMarkupObject.cs
- ClientFormsIdentity.cs
- ListBase.cs
- _ConnectOverlappedAsyncResult.cs
- ToolStripRenderer.cs
- ServiceDesigner.xaml.cs
- SafeEventLogWriteHandle.cs
- NativeMethods.cs
- Guid.cs
- QueryableDataSourceEditData.cs
- DataShape.cs
- DelayedRegex.cs
- FlowDocumentReaderAutomationPeer.cs
- LinqDataSourceStatusEventArgs.cs
- CollectionViewGroup.cs
- XamlTypeMapperSchemaContext.cs
- ResourceReferenceKeyNotFoundException.cs
- SortQueryOperator.cs
- Permission.cs
- LogicalTreeHelper.cs
- ColumnReorderedEventArgs.cs
- TabItem.cs
- XmlQueryType.cs
- TextModifier.cs
- validationstate.cs
- LastQueryOperator.cs
- ExtensionWindowResizeGrip.cs
- Vector3D.cs
- HtmlDocument.cs
- BindingMAnagerBase.cs
- ScheduleChanges.cs
- DictionaryMarkupSerializer.cs
- SecurityTokenProviderContainer.cs
- DataColumnPropertyDescriptor.cs
- HttpCacheParams.cs
- FaultContext.cs
- TabOrder.cs
- SQLDecimalStorage.cs
- ToolStripButton.cs
- shaper.cs
- LinkedList.cs
- DynamicDataResources.Designer.cs