Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Print / Reach / Serialization / manager / NGCSerializationManager.cs / 2 / NGCSerializationManager.cs
/*++ Copyright (C) 2004- 2005 Microsoft Corporation All rights reserved. Module Name: ReachSerializationManager.cs Abstract: Author: [....] ([....]) 10-Jan-2005 Revision History: --*/ using System; using System.Collections; using System.Collections.Specialized; using System.ComponentModel; using System.Diagnostics; using System.Reflection; using System.Xml; using System.IO; using System.Security; using System.Security.Permissions; using System.ComponentModel.Design.Serialization; using System.Windows.Xps.Packaging; using System.Windows.Documents; using System.Windows.Media; using System.Windows.Markup; using System.Printing; using System.Drawing.Printing; using Microsoft.Internal.AlphaFlattener; // // Ngc = Next Generation Converter. It means to convert the avalon element tree // to the downlevel GDI primitives. // namespace System.Windows.Xps.Serialization { ////// NgcSerializationManager is a class to help print avalon content (element tree) to traditional printer /// //[CLSCompliant(false)] internal sealed class NgcSerializationManager : PackageSerializationManager { #region Constructor ////// This constructor take PrintQueue parameter /// ///queue is NULL. public NgcSerializationManager( PrintQueue queue, bool isBatchMode ): base() { if (queue == null) { throw new ArgumentNullException("queue"); } _printQueue = queue; this._isBatchMode = isBatchMode; this._isSimulating = false; this._printTicketManager = new NgcPrintTicketManager(_printQueue); } #endregion Construtor #region PackageSerializationManager override ////// The function will serializer the avalon content to the printer spool file. /// SaveAsXaml is not a propriate name. Maybe it should be "Print" /// ///serializedObject is NULL. ///serializedObject is not a supported type. public override void SaveAsXaml( Object serializedObject ) { Toolbox.StartEvent(MS.Utility.EventTraceGuidId.DRXSAVEXPSGUID); if (serializedObject == null) { throw new ArgumentNullException("serializedObject"); } if(!IsSerializedObjectTypeSupported(serializedObject)) { throw new XpsSerializationException(ReachSR.Get(ReachSRID.ReachSerialization_NotSupported)); } if(_isBatchMode && !_isSimulating) { XpsSerializationPrintTicketRequiredEventArgs printTicketEvent = new XpsSerializationPrintTicketRequiredEventArgs(PrintTicketLevel.FixedDocumentSequencePrintTicket, 0); OnNGCSerializationPrintTicketRequired(printTicketEvent); StartDocument(null,true); _isSimulating = true; } if(_isBatchMode) { StartPage(); } if(!_isBatchMode && IsDocumentSequencePrintTicketRequired(serializedObject)) { XpsSerializationPrintTicketRequiredEventArgs printTicketEvent = new XpsSerializationPrintTicketRequiredEventArgs(PrintTicketLevel.FixedDocumentSequencePrintTicket, 0); OnNGCSerializationPrintTicketRequired(printTicketEvent); } ReachSerializer reachSerializer = GetSerializer(serializedObject); if(reachSerializer != null) { // // Call top-level type serializer, it will walk through the contents and // all CLR and DP properties of the object and invoke the proper serializer // and typeconverter respectively // reachSerializer.SerializeObject(serializedObject); if(_isBatchMode) { EndPage(); } } else { throw new XpsSerializationException(ReachSR.Get(ReachSRID.ReachSerialization_NoSerializer)); } Toolbox.EndEvent(MS.Utility.EventTraceGuidId.DRXSAVEXPSGUID); } ////// /// public void Cancel( ) { if(_startDocCnt != 0 ) { _device.AbortDocument(); _startDocCnt = 0; } } ////// /// public void Commit( ) { if(_isBatchMode && _isSimulating) { EndDocument(); } else { // // throw the appropariate exception // } } ////// /// public event XpsSerializationPrintTicketRequiredEventHandler XpsSerializationPrintTicketRequired; ////// /// public event XpsSerializationProgressChangedEventHandler XpsSerializationProgressChanged; ////// Todo: This function GetXmlNSForType and the other four XML writer functiosn (like AcquireXmlWriter) /// and the two stream function (like ReleaseResourceStream) should be removed from /// the base PackageSerializationManager class. /// internal override String GetXmlNSForType( Type objectType ) { return null; } ////// /// internal override ReachSerializer GetSerializer( Object serializedObject ) { ReachSerializer reachSerializer = null; if((reachSerializer = base.GetSerializer(serializedObject)) == null) { reachSerializer = this.SerializersCacheManager.GetSerializer(serializedObject); } return reachSerializer; } ////// /// internal override Type GetSerializerType( Type objectType ) { Type serializerType = null; if((serializerType = base.GetSerializerType(objectType)) == null) { if (typeof(System.Windows.Documents.FixedDocumentSequence).IsAssignableFrom(objectType)) { serializerType = typeof(NgcDocumentSequenceSerializer); } else if (typeof(System.Windows.Documents.DocumentReferenceCollection).IsAssignableFrom(objectType)) { serializerType = typeof(NgcDocumentReferenceCollectionSerializer); } else if (typeof(System.Windows.Documents.FixedDocument).IsAssignableFrom(objectType)) { serializerType = typeof(NgcFixedDocumentSerializer); } else if(typeof(System.Windows.Documents.PageContentCollection).IsAssignableFrom(objectType)) { serializerType = typeof(NGCReachPageContentCollectionSerializer); } else if(typeof(System.Windows.Documents.PageContent).IsAssignableFrom(objectType)) { serializerType = typeof(NGCReachPageContentSerializer); } else if(typeof(System.Windows.Controls.UIElementCollection).IsAssignableFrom(objectType)) { serializerType = typeof(NGCReachUIElementCollectionSerializer); } else if(typeof(System.Windows.Documents.FixedPage).IsAssignableFrom(objectType)) { serializerType = typeof(NgcFixedPageSerializer); } else if (typeof(System.Windows.Documents.DocumentPaginator).IsAssignableFrom(objectType)) { serializerType = typeof(NgcDocumentPaginatorSerializer); } else if (typeof(System.Windows.Documents.DocumentPage).IsAssignableFrom(objectType)) { serializerType = typeof(NgcDocumentPageSerializer); } else if (typeof(System.Windows.Media.Visual).IsAssignableFrom(objectType)) { serializerType = typeof(NgcReachVisualSerializer); } } return serializerType; } internal override XmlWriter AcquireXmlWriter( Type writerType ) { return null; } internal override void ReleaseXmlWriter( Type writerType ) { return; } internal override XpsResourceStream AcquireResourceStream( Type resourceType ) { return null; } internal override XpsResourceStream AcquireResourceStream( Type resourceType, String resourceID ) { return null; } internal override void ReleaseResourceStream( Type resourceType ) { return; } internal override void ReleaseResourceStream( Type resourceType, String resourceID ) { return; } internal override void AddRelationshipToCurrentPage( Uri targetUri, string relationshipName ) { return; } internal override XpsResourcePolicy ResourcePolicy { get { return null; } } internal override BasePackagingPolicy PackagingPolicy { get { return null; } } #endregion PackageSerializationManager override #region Internal Properties ////// /// internal PrintQueue PrintQueue { get { return _printQueue; } } internal String JobName { set { if (_jobName == null) { _jobName = value; } } get { return _jobName; } } internal Size PageSize { set { _pageSize = value; } get { return _pageSize; } } #endregion Internal Properties #region Internal Methods ////// Critical : Elevates to be able to set the print job id, which demands DefaultPrinting /// TreatAsSafe: Partial Trust caller needs DefaultPrinting to be able to read the JobIdentifier property /// See JobIdentifier property in base class PackageSerializationManager (MetroSerializationManager.cs) /// [SecurityCritical, SecurityTreatAsSafe] internal void StartDocument( Object o, bool documentPrintTicketRequired ) { if(documentPrintTicketRequired) { XpsSerializationPrintTicketRequiredEventArgs e = new XpsSerializationPrintTicketRequiredEventArgs(PrintTicketLevel.FixedDocumentPrintTicket, 0); OnNGCSerializationPrintTicketRequired(e); } if( _startDocCnt == 0 ) { JobName = _printQueue.CurrentJobSettings.Description; if(JobName == null) { JobName = NgcSerializerUtil.InferJobName(o); } _device = new MetroToGdiConverter(PrintQueue); if (!_isSimulating) { (new PrintingPermission(PrintingPermissionLevel.DefaultPrinting)).Assert(); try { JobIdentifier = _device.StartDocument(_jobName, _printTicketManager.ConsumeActivePrintTicket(true)); } finally { CodeAccessPermission.RevertAssert(); } } } _startDocCnt++; } ////// /// /// internal void EndDocument() { if( _startDocCnt == 1 ) { _device.EndDocument(); // // Inform any potential listeners that the doucment has been printed // XpsSerializationProgressChangedEventArgs e = new XpsSerializationProgressChangedEventArgs(XpsWritingProgressChangeLevel.FixedDocumentWritingProgress, 0, 0, null); OnNGCSerializationProgressChagned(e); } _startDocCnt--; } ////// /// /// internal bool StartPage() { bool bManulStartDoc = false; if (_startDocCnt == 0) { StartDocument(null,true); bManulStartDoc = true; } if(!_isStartPage) { if (_isPrintTicketMerged == false) { XpsSerializationPrintTicketRequiredEventArgs e = new XpsSerializationPrintTicketRequiredEventArgs(PrintTicketLevel.FixedPagePrintTicket, 0); OnNGCSerializationPrintTicketRequired(e); } _device.StartPage(_printTicketManager.ConsumeActivePrintTicket(true)); _isStartPage = true; } return bManulStartDoc; } ////// /// /// internal void EndPage() { if (_isStartPage) { try { _device.FlushPage(); } catch (PrintingCanceledException ) { _device.EndDocument(); throw; } } _isStartPage = false; _isPrintTicketMerged = false; // // Inform any potential listeners that the page has been printed // XpsSerializationProgressChangedEventArgs e = new XpsSerializationProgressChangedEventArgs(XpsWritingProgressChangeLevel.FixedPageWritingProgress, 0, 0, null); OnNGCSerializationProgressChagned(e); } internal void OnNGCSerializationPrintTicketRequired( object operationState ) { XpsSerializationPrintTicketRequiredEventArgs e = operationState as XpsSerializationPrintTicketRequiredEventArgs; if(XpsSerializationPrintTicketRequired != null) { e.Modified = true; if (e.PrintTicketLevel == PrintTicketLevel.FixedPagePrintTicket) { _isPrintTicketMerged = true; } XpsSerializationPrintTicketRequired(this,e); _printTicketManager.ConstructPrintTicketTree(e); } } internal void OnNGCSerializationProgressChagned( object operationState ) { XpsSerializationProgressChangedEventArgs e = operationState as XpsSerializationProgressChangedEventArgs; if(XpsSerializationProgressChanged != null) { XpsSerializationProgressChanged(this,e); } } ////// /// /// internal void WalkVisual( Visual visual ) { bool bManulStartDoc = false; bool bManulStartpage = false; if (_startDocCnt == 0) { StartDocument(visual,true); bManulStartDoc = true; } if (!_isStartPage) { StartPage(); bManulStartpage = true; } // // Call VisualTreeFlattener to flatten the visual on IMetroDrawingContext // Toolbox.StartEvent(MS.Utility.EventTraceGuidId.DRXLOADPRIMITIVEGUID); VisualTreeFlattener.Walk(visual, _device, PageSize); Toolbox.EndEvent(MS.Utility.EventTraceGuidId.DRXLOADPRIMITIVEGUID); if (bManulStartpage) { EndPage(); } if (bManulStartDoc) { EndDocument(); } } internal PrintTicket GetActivePrintTicket() { return _printTicketManager.ActivePrintTicket; } internal bool IsPrintTicketEventHandlerEnabled { get { if(XpsSerializationPrintTicketRequired!=null) { return true; } else { return false; } } } private bool IsSerializedObjectTypeSupported( Object serializedObject ) { bool isSupported = false; Type serializedObjectType = serializedObject.GetType(); if(_isBatchMode) { if((typeof(System.Windows.Media.Visual).IsAssignableFrom(serializedObjectType)) && (serializedObjectType != typeof(System.Windows.Documents.FixedPage))) { isSupported = true; } } else { if ( (serializedObjectType == typeof(System.Windows.Documents.FixedDocumentSequence)) || (serializedObjectType == typeof(System.Windows.Documents.FixedDocument)) || (serializedObjectType == typeof(System.Windows.Documents.FixedPage)) || (typeof(System.Windows.Documents.DocumentPaginator).IsAssignableFrom(serializedObjectType)) || (typeof(System.Windows.Media.Visual).IsAssignableFrom(serializedObjectType)) ) { isSupported = true; } } return isSupported; } private bool IsDocumentSequencePrintTicketRequired( Object serializedObject ) { bool isRequired = false; Type serializedObjectType = serializedObject.GetType(); if ((serializedObjectType == typeof(System.Windows.Documents.FixedDocument)) || (serializedObjectType == typeof(System.Windows.Documents.FixedPage)) || (typeof(System.Windows.Documents.DocumentPaginator).IsAssignableFrom(serializedObjectType)) || (typeof(System.Windows.Media.Visual).IsAssignableFrom(serializedObjectType)) ) { isRequired = true; } return isRequired; } #endregion Internal Methods #region Private Member private PrintQueue _printQueue; private int _startDocCnt; private bool _isStartPage; private MetroToGdiConverter _device; private String _jobName; private bool _isBatchMode; private bool _isSimulating; private NgcPrintTicketManager _printTicketManager; private bool _isPrintTicketMerged; private Size _pageSize = new Size(816,1056); #endregion Private Member }; internal sealed class NgcPrintTicketManager { #region constructor public NgcPrintTicketManager( PrintQueue printQueue ) { this._printQueue = printQueue; this._fdsPrintTicket = null; this._fdPrintTicket = null; this._fpPrintTicket = null; this._rootPrintTicket = null; this._activePrintTicket = null; this._isActiveUpdated = false; } #endregion constructor #region Public Methods public void ConstructPrintTicketTree( XpsSerializationPrintTicketRequiredEventArgs args ) { switch(args.PrintTicketLevel) { case PrintTicketLevel.FixedDocumentSequencePrintTicket: { if(args.PrintTicket != null) { _fdsPrintTicket = args.PrintTicket; _rootPrintTicket = _fdsPrintTicket; } else { _rootPrintTicket = new PrintTicket(_printQueue.UserPrintTicket.GetXmlStream()); } _activePrintTicket = _rootPrintTicket; _isActiveUpdated = true; break; } case PrintTicketLevel.FixedDocumentPrintTicket: { if(args.PrintTicket != null) { _fdPrintTicket = args.PrintTicket; if(_fdsPrintTicket!=null) { // // we have to merge the 2 print tickets // _rootPrintTicket = _printQueue. MergeAndValidatePrintTicket(_fdsPrintTicket, _fdPrintTicket). ValidatedPrintTicket; } else { _rootPrintTicket = _fdPrintTicket; } } else { _fdPrintTicket = null; _rootPrintTicket = _fdsPrintTicket; } if(_rootPrintTicket == null) { _rootPrintTicket = new PrintTicket(_printQueue.UserPrintTicket.GetXmlStream()); } _activePrintTicket = _rootPrintTicket; _isActiveUpdated = true; break; } case PrintTicketLevel.FixedPagePrintTicket: { if(args.PrintTicket != null) { _fpPrintTicket = args.PrintTicket; // // we have to merge the 2 print tickets // _activePrintTicket = _printQueue. MergeAndValidatePrintTicket(_rootPrintTicket, _fpPrintTicket). ValidatedPrintTicket; _isActiveUpdated = true; } else { if(_fpPrintTicket != null) { _fpPrintTicket = null; _activePrintTicket = _rootPrintTicket; _isActiveUpdated = true; } } break; } default: { break; } } } public PrintTicket ConsumeActivePrintTicket( bool consumePrintTicket ) { PrintTicket printTicket = null; if (_activePrintTicket == null && _rootPrintTicket == null) { _activePrintTicket = new PrintTicket(_printQueue.UserPrintTicket.GetXmlStream()); _rootPrintTicket = _activePrintTicket; printTicket = _activePrintTicket; } else if (_isActiveUpdated) { printTicket = _activePrintTicket; if (consumePrintTicket) { _isActiveUpdated = false; } } return printTicket; } #endregion Public Methods #region Public Properties public PrintTicket ActivePrintTicket { get { //Call Consume Active Print Ticket with false flag, to get the current print ticket but do not consume it //so it can be retrieved again return ConsumeActivePrintTicket(false); } } #endregion Public Properties #region Private Members private PrintQueue _printQueue; private PrintTicket _fdsPrintTicket; private PrintTicket _fdPrintTicket; private PrintTicket _fpPrintTicket; private PrintTicket _rootPrintTicket; private PrintTicket _activePrintTicket; private bool _isActiveUpdated; #endregion Private Members } internal sealed class MXDWSerializationManager { #region constructor ////// Critical - Sets up the gdiDevice which is critical /// Safe - Initiliazed only in this method. /// [SecurityCritical, SecurityTreatAsSafe] public MXDWSerializationManager( PrintQueue queue ) { this._jobName = null; this._gdiDevice = null; this._mxdwFileName = null; _printQueue = queue; _jobName = _printQueue.CurrentJobSettings.Description; if(_jobName == null) { _jobName = NgcSerializerUtil.InferJobName(null); } _gdiDevice = new MetroToGdiConverter(_printQueue); GdiDevice.CreateDeviceContext(_jobName, InferPrintTicket()); _isPassThruSupported = GdiDevice.ExtEscMXDWPassThru(); GdiDevice.DeleteDeviceContext(); } #endregion constructor ////// Critical - Initilaizes critial fieled _mxdwFileName /// Safe - field is kept local /// [SecurityCritical, SecurityTreatAsSafe] public void EnablePassThru( ) { GdiDevice.CreateDeviceContext(_jobName, InferPrintTicket()); GdiDevice.ExtEscMXDWPassThru(); GdiDevice.StartDocumentWithoutCreatingDC(_jobName); _mxdwFileName = GdiDevice.ExtEscGetName(); } ////// Critical - Exposes user selected file path /// public String MxdwFileName { [SecurityCritical] get { return _mxdwFileName; } } public bool IsPassThruSupported { get { return _isPassThruSupported; } } public void Commit( ) { GdiDevice.EndDocument(); } private PrintTicket InferPrintTicket( ) { PrintTicket printTicket = new PrintTicket(_printQueue.UserPrintTicket.GetXmlStream()); return printTicket; } ////// Critical - access _gdiDivice /// Safe - Read access is safe /// private MetroToGdiConverter GdiDevice { [SecurityCritical, SecurityTreatAsSafe] get { return _gdiDevice; } } private PrintQueue _printQueue; ////// Critical - device is used to aquire mxdw file. Set is only valid in constructor /// [SecurityCritical] private MetroToGdiConverter _gdiDevice; private String _jobName; ////// Critical - User selected file path recieved from mxdw driver. Path information is critical /// [SecurityCritical] private String _mxdwFileName; private bool _isPassThruSupported; }; } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
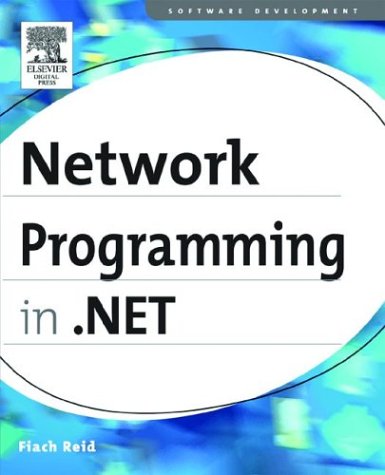
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- BaseValidator.cs
- SQLStringStorage.cs
- CopyCodeAction.cs
- EmptyEnumerable.cs
- NeutralResourcesLanguageAttribute.cs
- ZipPackagePart.cs
- FixedDocumentSequencePaginator.cs
- ServicePointManager.cs
- StringComparer.cs
- TextOnlyOutput.cs
- WindowsListViewGroup.cs
- SiteMapDataSource.cs
- SoapMessage.cs
- WebBrowserDocumentCompletedEventHandler.cs
- DataGridClipboardCellContent.cs
- ServiceProviders.cs
- GraphicsContainer.cs
- XsltContext.cs
- ConnectionManagementElementCollection.cs
- UnionExpr.cs
- WebHttpDispatchOperationSelectorData.cs
- PersonalizationProviderHelper.cs
- SqlTriggerContext.cs
- DispatchChannelSink.cs
- SystemWebSectionGroup.cs
- odbcmetadatafactory.cs
- BindingOperations.cs
- PauseStoryboard.cs
- TriState.cs
- CommandHelpers.cs
- MessageHeaders.cs
- mediaeventshelper.cs
- Object.cs
- XmlLoader.cs
- SqlOuterApplyReducer.cs
- LazyTextWriterCreator.cs
- GridViewUpdatedEventArgs.cs
- TaiwanCalendar.cs
- ParenthesizePropertyNameAttribute.cs
- ClockGroup.cs
- TrailingSpaceComparer.cs
- TraceSection.cs
- TypeToArgumentTypeConverter.cs
- ColorKeyFrameCollection.cs
- CombinedGeometry.cs
- MetabaseReader.cs
- MemberAccessException.cs
- URL.cs
- HotSpotCollection.cs
- MetadataAssemblyHelper.cs
- ColorEditor.cs
- HtmlTable.cs
- PictureBox.cs
- Activity.cs
- SimpleWorkerRequest.cs
- ComponentCollection.cs
- NamespaceExpr.cs
- InkCanvasAutomationPeer.cs
- SignatureResourcePool.cs
- IDQuery.cs
- GridErrorDlg.cs
- XPathDocumentBuilder.cs
- ContainerVisual.cs
- ThreadStaticAttribute.cs
- ImageIndexConverter.cs
- SQLDouble.cs
- ObjectPropertyMapping.cs
- TdsParserStaticMethods.cs
- WindowsAuthenticationEventArgs.cs
- XmlElementAttributes.cs
- InkPresenterAutomationPeer.cs
- Mappings.cs
- Operand.cs
- RayHitTestParameters.cs
- InboundActivityHelper.cs
- JapaneseLunisolarCalendar.cs
- VolatileEnlistmentState.cs
- ListDictionaryInternal.cs
- ValueSerializer.cs
- StatusBarDrawItemEvent.cs
- DBCommandBuilder.cs
- TableColumn.cs
- CacheHelper.cs
- StatusBarDrawItemEvent.cs
- WebControlParameterProxy.cs
- WpfMemberInvoker.cs
- EvidenceTypeDescriptor.cs
- XPathDocument.cs
- HtmlInputFile.cs
- ObjectDataSource.cs
- AsymmetricSignatureFormatter.cs
- ListItemParagraph.cs
- WindowsGraphics.cs
- AuthenticationModuleElementCollection.cs
- TemplatePartAttribute.cs
- WebPartPersonalization.cs
- SystemUdpStatistics.cs
- FormView.cs
- TaskHelper.cs
- ServiceOperationHelpers.cs