Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Shared / MS / Win32 / handlecollector.cs / 1 / handlecollector.cs
using System; using MS.Win32 ; using System.Runtime.InteropServices; namespace MS.Win32 { internal static class HandleCollector { private static HandleType[] handleTypes; private static int handleTypeCount = 0; private static Object handleMutex = new Object(); ////// Adds the given handle to the handle collector. This keeps the /// handle on a "hot list" of objects that may need to be garbage /// collected. /// internal static IntPtr Add(IntPtr handle, int type) { handleTypes[type - 1].Add(); return handle; } internal static SafeHandle Add(SafeHandle handle, int type) { handleTypes[type - 1].Add(); return handle; } ////// Registers a new type of handle with the handle collector. /// internal static int RegisterType(string typeName, int expense, int initialThreshold) { lock (handleMutex) { if (handleTypeCount == 0 || handleTypeCount == handleTypes.Length) { HandleType[] newTypes = new HandleType[handleTypeCount + 10]; if (handleTypes != null) { Array.Copy(handleTypes, 0, newTypes, 0, handleTypeCount); } handleTypes = newTypes; } handleTypes[handleTypeCount++] = new HandleType(typeName, expense, initialThreshold); return handleTypeCount; } } ////// Removes the given handle from the handle collector. Removing a /// handle removes it from our "hot list" of objects that should be /// frequently garbage collected. /// internal static IntPtr Remove(IntPtr handle, int type) { handleTypes[type - 1].Remove(); return handle ; } internal static SafeHandle Remove(SafeHandle handle, int type) { handleTypes[type - 1].Remove(); return handle ; } ////// Represents a specific type of handle. /// private class HandleType { internal readonly string name; private int initialThreshHold; private int threshHold; private int handleCount; private readonly int deltaPercent; ////// Creates a new handle type. /// internal HandleType(string name, int expense, int initialThreshHold) { this.name = name; this.initialThreshHold = initialThreshHold; this.threshHold = initialThreshHold; this.deltaPercent = 100 - expense; } ////// Adds a handle to this handle type for monitoring. /// internal void Add() { bool performCollect = false; lock(this) { handleCount++; performCollect = NeedCollection(); if (!performCollect) { return; } } if (performCollect) { #if DEBUG_HANDLECOLLECTOR Debug.WriteLine("HC> Forcing garbage collect"); Debug.WriteLine("HC> name :" + name); Debug.WriteLine("HC> threshHold :" + (threshHold).ToString()); Debug.WriteLine("HC> handleCount :" + (handleCount).ToString()); Debug.WriteLine("HC> deltaPercent:" + (deltaPercent).ToString()); #endif GC.Collect(); // We just performed a GC. If the main thread is in a tight // loop there is a this will cause us to increase handles forever and prevent handle collector // from doing its job. Yield the thread here. This won't totally cause // a finalization pass but it will effectively elevate the priority // of the finalizer thread just for an instant. But how long should // we sleep? We base it on how expensive the handles are because the // more expensive the handle, the more critical that it be reclaimed. int sleep = (100 - deltaPercent) / 4; System.Threading.Thread.Sleep(sleep); } } ////// Determines if this handle type needs a garbage collection pass. /// internal bool NeedCollection() { if (handleCount > threshHold) { threshHold = handleCount + ((handleCount * deltaPercent) / 100); #if DEBUG_HANDLECOLLECTOR Debug.WriteLine("HC> NeedCollection: increase threshHold to " + threshHold); #endif return true; } // If handle count < threshHold, we don't // need to collect, but if it 10% below the next lowest threshhold we // will bump down a rung. We need to choose a percentage here or else // we will oscillate. // int oldThreshHold = (100 * threshHold) / (100 + deltaPercent); if (oldThreshHold >= initialThreshHold && handleCount < (int)(oldThreshHold * .9F)) { #if DEBUG_HANDLECOLLECTOR Debug.WriteLine("HC> NeedCollection: throttle threshhold " + threshHold + " down to " + oldThreshHold); #endif threshHold = oldThreshHold; } return false; } ////// Removes the given handle from our monitor list. /// internal void Remove() { lock(this) { handleCount--; handleCount = Math.Max(0, handleCount); } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
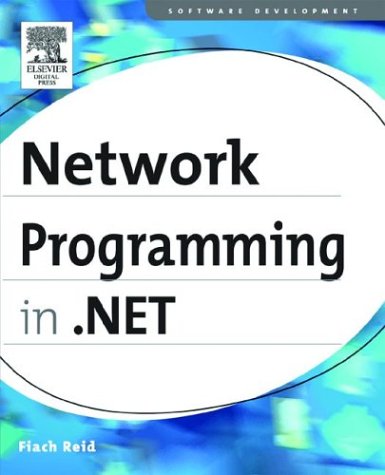
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ServiceModelReg.cs
- GetPageNumberCompletedEventArgs.cs
- RotateTransform3D.cs
- PathStreamGeometryContext.cs
- querybuilder.cs
- CqlBlock.cs
- _LoggingObject.cs
- MessageHeader.cs
- CollectionBase.cs
- XsltException.cs
- AsyncSerializedWorker.cs
- OleDbConnectionInternal.cs
- JoinSymbol.cs
- DataSourceHelper.cs
- HttpProfileBase.cs
- OleDbConnection.cs
- IItemContainerGenerator.cs
- OleDbPropertySetGuid.cs
- TextRangeEdit.cs
- ToolStripItemRenderEventArgs.cs
- WebPartRestoreVerb.cs
- TraceContext.cs
- XPathPatternBuilder.cs
- HtmlTextViewAdapter.cs
- SqlDataSourceParameterParser.cs
- RectAnimationClockResource.cs
- documentsequencetextcontainer.cs
- DataColumn.cs
- LinqDataSourceValidationException.cs
- BindStream.cs
- PreparingEnlistment.cs
- WebScriptClientGenerator.cs
- ReferenceEqualityComparer.cs
- TiffBitmapEncoder.cs
- DependencyObject.cs
- ContextMenu.cs
- XmlNamedNodeMap.cs
- BindingManagerDataErrorEventArgs.cs
- OdbcConnectionFactory.cs
- ZoomPercentageConverter.cs
- IdentityVerifier.cs
- TreeIterator.cs
- ZipIOCentralDirectoryBlock.cs
- PenContext.cs
- TextServicesCompartmentContext.cs
- FilterQueryOptionExpression.cs
- ModelTreeManager.cs
- UIElement3D.cs
- ControlCachePolicy.cs
- RadioButtonFlatAdapter.cs
- Attribute.cs
- ObjectItemLoadingSessionData.cs
- Random.cs
- WebPartManagerInternals.cs
- PathFigureCollectionConverter.cs
- basemetadatamappingvisitor.cs
- SmiXetterAccessMap.cs
- CodeDirectionExpression.cs
- TerminatorSinks.cs
- FileUtil.cs
- odbcmetadatacollectionnames.cs
- DescendantOverDescendantQuery.cs
- RangeValidator.cs
- SubMenuStyle.cs
- RoleService.cs
- QueuePropertyVariants.cs
- ColumnResizeUndoUnit.cs
- XmlEncodedRawTextWriter.cs
- HelpProvider.cs
- WorkflowValidationFailedException.cs
- SwitchAttribute.cs
- MouseBinding.cs
- WebPartTransformer.cs
- GrammarBuilderBase.cs
- RecordsAffectedEventArgs.cs
- InternalPermissions.cs
- HtmlInputButton.cs
- GeometryModel3D.cs
- MimeParameter.cs
- brushes.cs
- IndentedWriter.cs
- ProjectedSlot.cs
- PatternMatcher.cs
- PersonalizationStateInfo.cs
- DPCustomTypeDescriptor.cs
- MsmqIntegrationChannelFactory.cs
- Operators.cs
- DetailsViewModeEventArgs.cs
- precedingsibling.cs
- SyndicationDeserializer.cs
- SiteMapPath.cs
- CollectionType.cs
- PolyLineSegment.cs
- KeyConstraint.cs
- ButtonFlatAdapter.cs
- ListViewTableCell.cs
- JpegBitmapDecoder.cs
- DataSourceXmlElementAttribute.cs
- PageAsyncTask.cs
- DoubleLink.cs