Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Speech / Src / Internal / Synthesis / ISSmlParser.cs / 1 / ISSmlParser.cs
//// Copyright (c) Microsoft Corporation. All rights reserved. // // // Description: // // History: // 3/15/2005 [....] Created //---------------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Globalization; using System.IO; using System.Speech.Synthesis; using System.Speech.Synthesis.TtsEngine; using System.Text; using System.Xml; namespace System.Speech.Internal.Synthesis { //******************************************************************* // // Internal Types // //******************************************************************* #region Internal Types internal interface ISsmlParser { object ProcessSpeak (string sVersion, string sBaseUri, CultureInfo culture, ListextraNamespace); void ProcessText (string text, object voice, ref FragmentState fragmentState, int position, bool fIgnore); void ProcessAudio (object voice, string sUri, string baseUri, bool fIgnore); void ProcessBreak (object voice, ref FragmentState fragmentState, EmphasisBreak eBreak, int time, bool fIgnore); void ProcessDesc (CultureInfo culture); void ProcessEmphasis (bool noLevel, EmphasisWord word); void ProcessMark (object voice, ref FragmentState fragmentState, string name, bool fIgnore); object ProcessTextBlock (bool isParagraph, object voice, ref FragmentState fragmentState, CultureInfo culture, bool newCulture, VoiceGender gender, VoiceAge age); void EndProcessTextBlock (bool isParagraph); void ProcessPhoneme (ref FragmentState fragmentState, AlphabetType alphabet, string ph, char [] phoneIds); void ProcessProsody (string pitch, string range, string rate, string volume, string duration, string points); void ProcessSayAs (string interpretAs, string format, string detail); void ProcessSub (string alias, object voice, ref FragmentState fragmentState, int position, bool fIgnore); object ProcessVoice (string name, CultureInfo culture, VoiceGender gender, VoiceAge age, int variant, bool fNewCulture, List extraNamespace); void ProcessLexicon (Uri uri, string type); void EndElement (); void EndSpeakElement (); void ProcessUnknownElement (object voice, ref FragmentState fragmentState, XmlReader reader); void StartProcessUnknownAttributes (object voice, ref FragmentState fragmentState, string element, List extraAttributes); void EndProcessUnknownAttributes (object voice, ref FragmentState fragmentState, string element, List extraAttributes); // Prompt data used void ContainsPexml (string pexmlPrefix); // Prompt Engine tags bool BeginPromptEngineOutput (object voice); void EndPromptEngineOutput (object voice); // global elements bool ProcessPromptEngineDatabase (object voice, string fname, string delta, string idset); bool ProcessPromptEngineDiv (object voice); bool ProcessPromptEngineId (object voice, string id); // scoped elements bool BeginPromptEngineTts (object voice); void EndPromptEngineTts (object voice); bool BeginPromptEngineWithTag (object voice, string tag); void EndPromptEngineWithTag (object voice, string tag); bool BeginPromptEngineRule (object voice, string name); void EndPromptEngineRule (object voice, string name); // Properties string Ssml { get; } } internal class LexiconEntry { internal Uri _uri; internal string _mediaType; internal LexiconEntry (Uri uri, string mediaType) { _uri = uri; _mediaType = mediaType; } /// /// Tests whether two objects are equivalent /// public override bool Equals (object obj) { LexiconEntry entry = obj as LexiconEntry; return entry != null && _uri.Equals (entry._uri); } ////// Overrides Object.GetHashCode() /// public override int GetHashCode () { return _uri.GetHashCode (); } } internal class SsmlXmlAttribute { internal SsmlXmlAttribute (string prefix, string name, string value, string ns) { _prefix = prefix; _name = name; _value = value; _ns = ns; } internal string _prefix; internal string _name; internal string _value; internal string _ns; } #endregion } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
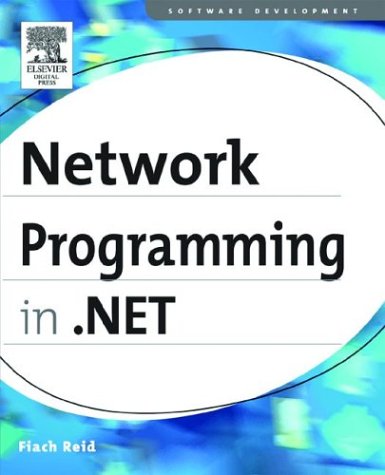
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- wgx_commands.cs
- NumberSubstitution.cs
- ECDiffieHellman.cs
- CompositeTypefaceMetrics.cs
- ToolStripProfessionalLowResolutionRenderer.cs
- SafeIUnknown.cs
- Rect.cs
- AuthenticateEventArgs.cs
- ListBoxChrome.cs
- OperatingSystem.cs
- UserNamePasswordValidationMode.cs
- ApplicationSettingsBase.cs
- DoubleCollectionValueSerializer.cs
- AppendHelper.cs
- XmlArrayAttribute.cs
- WS2007HttpBinding.cs
- CompilerGlobalScopeAttribute.cs
- MetadataArtifactLoaderComposite.cs
- ImageField.cs
- DynamicMetaObject.cs
- PrimitiveXmlSerializers.cs
- TextProperties.cs
- xml.cs
- ExpressionBuilder.cs
- FileLevelControlBuilderAttribute.cs
- QilInvokeEarlyBound.cs
- ScrollItemPattern.cs
- DbMetaDataColumnNames.cs
- StringAttributeCollection.cs
- TextRunTypographyProperties.cs
- MaterialGroup.cs
- IisTraceWebEventProvider.cs
- MimeWriter.cs
- ConnectionManagementSection.cs
- ResourceReader.cs
- DynamicDiscoveryDocument.cs
- WebCategoryAttribute.cs
- DbParameterCollection.cs
- BitmapEffectGeneralTransform.cs
- DependencyStoreSurrogate.cs
- dbenumerator.cs
- TemplatePropertyEntry.cs
- BoolExpr.cs
- QueryOpeningEnumerator.cs
- ExpressionBuilder.cs
- XPathItem.cs
- EventHandlerService.cs
- MailFileEditor.cs
- XamlWriter.cs
- ContentElement.cs
- PriorityQueue.cs
- FlowDocumentReaderAutomationPeer.cs
- IsolatedStorageSecurityState.cs
- ExpressionBuilder.cs
- DbParameterCollectionHelper.cs
- RoleService.cs
- Mouse.cs
- CultureMapper.cs
- TranslateTransform.cs
- RemoteWebConfigurationHost.cs
- HealthMonitoringSectionHelper.cs
- ZoneIdentityPermission.cs
- DbFunctionCommandTree.cs
- ZipIOLocalFileHeader.cs
- RowVisual.cs
- X509CertificateStore.cs
- RegexMatch.cs
- AlphabeticalEnumConverter.cs
- Action.cs
- ProxyGenerator.cs
- GraphicsContext.cs
- BooleanFunctions.cs
- XmlReaderSettings.cs
- ManualResetEvent.cs
- LayoutExceptionEventArgs.cs
- DataGridViewRowCollection.cs
- DocumentViewerConstants.cs
- AuthStoreRoleProvider.cs
- TdsRecordBufferSetter.cs
- SemanticBasicElement.cs
- TextPointerBase.cs
- PropertyGridView.cs
- AggregatePushdown.cs
- HostVisual.cs
- EpmContentDeSerializer.cs
- AdCreatedEventArgs.cs
- WebPartVerb.cs
- WindowsUpDown.cs
- ReadOnlyDataSource.cs
- Compilation.cs
- ScriptResourceAttribute.cs
- Main.cs
- SubMenuStyle.cs
- AssemblyAttributes.cs
- XmlEventCache.cs
- MessageEncodingBindingElement.cs
- NameValueConfigurationElement.cs
- TemplateBaseAction.cs
- DataGridViewSelectedCellCollection.cs
- EmptyTextWriter.cs