Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Core / CSharp / System / Windows / Media / Effects / BitmapEffectGeneralTransform.cs / 1 / BitmapEffectGeneralTransform.cs
/****************************************************************************\ * * File: BitmapEffectGeneralTransform.cs * * Description: * BitmapEffectGeneralTransform.cs defines the "BitmapEffectGeneralTransform" object * * Copyright (C) 2002 by Microsoft Corporation. All rights reserved. * \***************************************************************************/ using MS.Internal; using System; using System.Collections; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Runtime.InteropServices; using System.Security; using System.Security.Permissions; using System.Windows; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Windows.Markup; using MS.Internal.PresentationCore; using SR = MS.Internal.PresentationCore.SR; using SRID = MS.Internal.PresentationCore.SRID; namespace System.Windows.Media.Effects { ////// This is a general transform wrapper for a bitmap effect /// internal partial class BitmapEffectGeneralTransform : GeneralTransform { private bool _fInverse = false; private Rect _visualBounds = Rect.Empty; ////// Constructor /// public BitmapEffectGeneralTransform() { } ////// Constructor /// /// bitmap effect /// bitmap effect input /// Inverse transform /// The bounds of the visual for public BitmapEffectGeneralTransform(BitmapEffect effect, BitmapEffectInput input, bool fInverse, Rect visualBounds) { if (effect == null) throw new InvalidOperationException(SR.Get(SRID.Transform_No_Effect, null)); this.BitmapEffect = effect.Clone(); this.BitmapEffectInput = (input != null) ? input.Clone() : new BitmapEffectInput(); _fInverse = fInverse; _visualBounds = visualBounds; } ////// Returns true if the transform is an inverse /// internal bool IsInverse { get { return _fInverse; } set { _fInverse = value; } } ////// Implementation of /// protected override void CloneCore(Freezable sourceFreezable) { BitmapEffectGeneralTransform transform = (BitmapEffectGeneralTransform)sourceFreezable; base.CloneCore(sourceFreezable); CopyCommon(transform); } ///Freezable.CloneCore . ////// Implementation of /// protected override void CloneCurrentValueCore(Freezable sourceFreezable) { BitmapEffectGeneralTransform transform = (BitmapEffectGeneralTransform)sourceFreezable; base.CloneCurrentValueCore(sourceFreezable); CopyCommon(transform); } ///Freezable.CloneCurrentValueCore . ////// Implementation of /// protected override void GetAsFrozenCore(Freezable sourceFreezable) { BitmapEffectGeneralTransform transform = (BitmapEffectGeneralTransform)sourceFreezable; base.GetAsFrozenCore(sourceFreezable); CopyCommon(transform); } ///Freezable.GetAsFrozenCore . ////// Implementation of /// protected override void GetCurrentValueAsFrozenCore(Freezable sourceFreezable) { BitmapEffectGeneralTransform transform = (BitmapEffectGeneralTransform)sourceFreezable; base.GetCurrentValueAsFrozenCore(sourceFreezable); CopyCommon(transform); } ///Freezable.GetCurrentValueAsFrozenCore . ////// Transforms a point /// /// input point /// output point ///false if the point cannot be transformed public override bool TryTransform(Point inPoint, out Point result) { if (BitmapEffect == null) throw new InvalidOperationException(SR.Get(SRID.Transform_No_Effect, null)); if (BitmapEffectInput == null) throw new InvalidOperationException(SR.Get(SRID.Transform_No_Effect_Input, null)); BitmapEffect.VisualBounds = _visualBounds; return BitmapEffect.TransformPoint(BitmapEffectInput, inPoint, out result, _fInverse); } ////// Transform the rect bounds into the smallest axis alligned bounding box that /// contains all the point in the original bounds. /// /// ///public override Rect TransformBounds(Rect rect) { if (BitmapEffect == null) throw new InvalidOperationException(SR.Get(SRID.Transform_No_Effect, null)); if (BitmapEffectInput == null) throw new InvalidOperationException(SR.Get(SRID.Transform_No_Effect_Input, null)); return BitmapEffect.TransformRect(BitmapEffectInput, rect, _fInverse); } /// /// Returns the inverse transform if there is one, null otherwise /// public override GeneralTransform Inverse { get { ReadPreamble(); if (!BitmapEffect.IsInverseTransform) return null; BitmapEffectGeneralTransform inverse = Clone(); inverse.IsInverse = !_fInverse; return inverse; } } ////// Returns a best effort affine transform /// internal override Transform AffineTransform { [FriendAccessAllowed] // Built into Core, also used by Framework. get { return null; } } ////// Clones values that do not have corresponding DPs /// /// private void CopyCommon(BitmapEffectGeneralTransform transform) { _fInverse = transform._fInverse; _visualBounds = transform._visualBounds; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. /****************************************************************************\ * * File: BitmapEffectGeneralTransform.cs * * Description: * BitmapEffectGeneralTransform.cs defines the "BitmapEffectGeneralTransform" object * * Copyright (C) 2002 by Microsoft Corporation. All rights reserved. * \***************************************************************************/ using MS.Internal; using System; using System.Collections; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Runtime.InteropServices; using System.Security; using System.Security.Permissions; using System.Windows; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Windows.Markup; using MS.Internal.PresentationCore; using SR = MS.Internal.PresentationCore.SR; using SRID = MS.Internal.PresentationCore.SRID; namespace System.Windows.Media.Effects { ////// This is a general transform wrapper for a bitmap effect /// internal partial class BitmapEffectGeneralTransform : GeneralTransform { private bool _fInverse = false; private Rect _visualBounds = Rect.Empty; ////// Constructor /// public BitmapEffectGeneralTransform() { } ////// Constructor /// /// bitmap effect /// bitmap effect input /// Inverse transform /// The bounds of the visual for public BitmapEffectGeneralTransform(BitmapEffect effect, BitmapEffectInput input, bool fInverse, Rect visualBounds) { if (effect == null) throw new InvalidOperationException(SR.Get(SRID.Transform_No_Effect, null)); this.BitmapEffect = effect.Clone(); this.BitmapEffectInput = (input != null) ? input.Clone() : new BitmapEffectInput(); _fInverse = fInverse; _visualBounds = visualBounds; } ////// Returns true if the transform is an inverse /// internal bool IsInverse { get { return _fInverse; } set { _fInverse = value; } } ////// Implementation of /// protected override void CloneCore(Freezable sourceFreezable) { BitmapEffectGeneralTransform transform = (BitmapEffectGeneralTransform)sourceFreezable; base.CloneCore(sourceFreezable); CopyCommon(transform); } ///Freezable.CloneCore . ////// Implementation of /// protected override void CloneCurrentValueCore(Freezable sourceFreezable) { BitmapEffectGeneralTransform transform = (BitmapEffectGeneralTransform)sourceFreezable; base.CloneCurrentValueCore(sourceFreezable); CopyCommon(transform); } ///Freezable.CloneCurrentValueCore . ////// Implementation of /// protected override void GetAsFrozenCore(Freezable sourceFreezable) { BitmapEffectGeneralTransform transform = (BitmapEffectGeneralTransform)sourceFreezable; base.GetAsFrozenCore(sourceFreezable); CopyCommon(transform); } ///Freezable.GetAsFrozenCore . ////// Implementation of /// protected override void GetCurrentValueAsFrozenCore(Freezable sourceFreezable) { BitmapEffectGeneralTransform transform = (BitmapEffectGeneralTransform)sourceFreezable; base.GetCurrentValueAsFrozenCore(sourceFreezable); CopyCommon(transform); } ///Freezable.GetCurrentValueAsFrozenCore . ////// Transforms a point /// /// input point /// output point ///false if the point cannot be transformed public override bool TryTransform(Point inPoint, out Point result) { if (BitmapEffect == null) throw new InvalidOperationException(SR.Get(SRID.Transform_No_Effect, null)); if (BitmapEffectInput == null) throw new InvalidOperationException(SR.Get(SRID.Transform_No_Effect_Input, null)); BitmapEffect.VisualBounds = _visualBounds; return BitmapEffect.TransformPoint(BitmapEffectInput, inPoint, out result, _fInverse); } ////// Transform the rect bounds into the smallest axis alligned bounding box that /// contains all the point in the original bounds. /// /// ///public override Rect TransformBounds(Rect rect) { if (BitmapEffect == null) throw new InvalidOperationException(SR.Get(SRID.Transform_No_Effect, null)); if (BitmapEffectInput == null) throw new InvalidOperationException(SR.Get(SRID.Transform_No_Effect_Input, null)); return BitmapEffect.TransformRect(BitmapEffectInput, rect, _fInverse); } /// /// Returns the inverse transform if there is one, null otherwise /// public override GeneralTransform Inverse { get { ReadPreamble(); if (!BitmapEffect.IsInverseTransform) return null; BitmapEffectGeneralTransform inverse = Clone(); inverse.IsInverse = !_fInverse; return inverse; } } ////// Returns a best effort affine transform /// internal override Transform AffineTransform { [FriendAccessAllowed] // Built into Core, also used by Framework. get { return null; } } ////// Clones values that do not have corresponding DPs /// /// private void CopyCommon(BitmapEffectGeneralTransform transform) { _fInverse = transform._fInverse; _visualBounds = transform._visualBounds; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
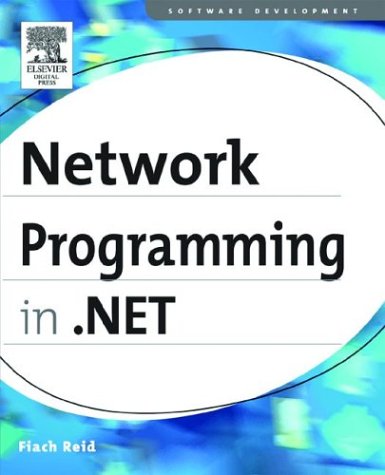
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HttpFormatExtensions.cs
- ResourceReferenceKeyNotFoundException.cs
- Monitor.cs
- XmlReflectionImporter.cs
- FieldTemplateFactory.cs
- CodeNamespace.cs
- RepeatButtonAutomationPeer.cs
- XmlSortKey.cs
- ResponseBodyWriter.cs
- DataControlLinkButton.cs
- TextOptionsInternal.cs
- DataMember.cs
- mediaeventargs.cs
- DefaultDialogButtons.cs
- CalendarAutoFormatDialog.cs
- FixedSOMFixedBlock.cs
- LoginDesigner.cs
- SafeFileMappingHandle.cs
- StubHelpers.cs
- FileInfo.cs
- safesecurityhelperavalon.cs
- TypeTypeConverter.cs
- WebConfigurationManager.cs
- DataGridViewTextBoxEditingControl.cs
- CounterCreationDataConverter.cs
- PrintDialogException.cs
- WindowsScroll.cs
- NavigationCommands.cs
- FilterException.cs
- DbFunctionCommandTree.cs
- safelink.cs
- sqlpipe.cs
- PointAnimationUsingPath.cs
- ServicesUtilities.cs
- QilCloneVisitor.cs
- EventDescriptor.cs
- InputProcessorProfilesLoader.cs
- DataSourceHelper.cs
- ParserHooks.cs
- RowBinding.cs
- ProviderConnectionPointCollection.cs
- WindowsPrincipal.cs
- LocationUpdates.cs
- ResXFileRef.cs
- DecoderFallbackWithFailureFlag.cs
- MainMenu.cs
- Expression.cs
- DragCompletedEventArgs.cs
- HttpResponseHeader.cs
- SByte.cs
- DataViewSetting.cs
- ClientTargetCollection.cs
- ParsedAttributeCollection.cs
- ByteArrayHelperWithString.cs
- SlotInfo.cs
- PersistChildrenAttribute.cs
- Attributes.cs
- SiteOfOriginContainer.cs
- DataGridViewAutoSizeColumnModeEventArgs.cs
- XComponentModel.cs
- SystemIcmpV6Statistics.cs
- IssuedTokenClientElement.cs
- SystemIPv6InterfaceProperties.cs
- EventSinkActivity.cs
- ProfileGroupSettings.cs
- StylusPointDescription.cs
- ListControl.cs
- ExpandCollapseProviderWrapper.cs
- CredentialCache.cs
- ControlUtil.cs
- AffineTransform3D.cs
- PropertyMappingExceptionEventArgs.cs
- XmlSignatureManifest.cs
- NamespaceList.cs
- Rotation3D.cs
- PreviousTrackingServiceAttribute.cs
- Sql8ConformanceChecker.cs
- Type.cs
- Hash.cs
- ClientSettingsSection.cs
- DocumentGrid.cs
- ThicknessKeyFrameCollection.cs
- GeometryDrawing.cs
- CodeConstructor.cs
- NumberFormatter.cs
- TrackPoint.cs
- AvTrace.cs
- KeyTimeConverter.cs
- cookie.cs
- BamlTreeUpdater.cs
- BackStopAuthenticationModule.cs
- __Error.cs
- ValueQuery.cs
- securitymgrsite.cs
- MediaElement.cs
- DocumentXPathNavigator.cs
- ConfigurationStrings.cs
- MDIClient.cs
- AQNBuilder.cs
- EventManager.cs