Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Speech / Src / Synthesis / TTSEngine / TTSEngineTypes.cs / 1 / TTSEngineTypes.cs
//------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------- using System; using System.Collections.Generic; using System.Collections.ObjectModel; using System.ComponentModel; using System.IO; using System.Runtime.InteropServices; using System.Speech.Internal; #if VSCOMPILE using System.Diagnostics; #endif #pragma warning disable 1634, 1691 // Allows suppression of certain PreSharp messages. #pragma warning disable 56504 // The public API is not that public so remove all the parameter validation. namespace System.Speech.Synthesis.TtsEngine { //******************************************************************* // // Public Interface // //******************************************************************* #region Public Interface ////// TODOC /// #if !SPEECHSERVER public #else internal #endif abstract class TtsEngineSsml { ////// Constructor for the TTS engine /// /// Voice token registry entry /// from where this engine was created from protected TtsEngineSsml (string registryKey) { } ////// Queries the engine about the output format it supports. /// /// Wave or Text /// Wave format header ///Returns the closest format that it supports abstract public IntPtr GetOutputFormat (SpeakOutputFormat speakOutputFormat, IntPtr targetWaveFormat); ////// Add a lexicon for this engine /// /// uri /// media type /// Engine site abstract public void AddLexicon (Uri uri, string mediaType, ITtsEngineSite site); ////// Removes a lexicon for this engine /// /// uri /// Engine site abstract public void RemoveLexicon (Uri uri, ITtsEngineSite site); ////// Renders the specified text fragments array in the /// specified output format. /// /// Text fragment with SSML /// attributes information /// Wave format header /// Engine site abstract public void Speak (TextFragment [] fragment, IntPtr waveHeader, ITtsEngineSite site); } ////// TODOC /// [StructLayout (LayoutKind.Sequential)] [ImmutableObject (true)] #if !SPEECHSERVER public #else internal #endif struct SpeechEventInfo : IEquatable{ /// /// TODOC /// public Int16 EventId { get { return _eventId; } internal set { _eventId = value; } } ////// TODOC /// public Int16 ParameterType { get { return _parameterType; } internal set { _parameterType = value; } } ////// Always just a numeric type - contains no unmanaged resources so does not need special clean-up. /// public int Param1 { get { return _param1; } internal set { _param1 = value; } } // ////// Can be a numeric type, or pointer to string. /// public IntPtr Param2 { get { return _param2; } internal set { _param2 = value; } } /// TODOC public SpeechEventInfo (Int16 eventId, Int16 parameterType, int param1, IntPtr param2) { _eventId = eventId; _parameterType = parameterType; _param1 = param1; _param2 = param2; } /// TODOC public static bool operator == (SpeechEventInfo event1, SpeechEventInfo event2) { return event1.EventId == event2.EventId && event1.ParameterType == event2.ParameterType && event1.Param1 == event2.Param1 && event1.Param2 == event2.Param2; } /// TODOC public static bool operator != (SpeechEventInfo event1, SpeechEventInfo event2) { return !(event1 == event2); } /// TODOC public bool Equals (SpeechEventInfo other) { return this == other; } /// TODOC public override bool Equals (object obj) { if (!(obj is SpeechEventInfo)) { return false; } return Equals ((SpeechEventInfo) obj); } /// TODOC public override int GetHashCode () { return base.GetHashCode (); } private Int16 _eventId; private Int16 _parameterType; private int _param1; // Always just a numeric type - contains no unmanaged resources so does not need special clean-up. private IntPtr _param2; // Can be a numeric type, or pointer to string or object. Use SafeSapiLParamHandle to cleanup. } ////// TODOC /// #if !SPEECHSERVER public #else internal #endif interface ITtsEngineSite { ////// TODOC /// void AddEvents (SpeechEventInfo [] events, int count); ////// TODOC /// int Write (IntPtr data, int count); ////// TODOC /// SkipInfo GetSkipInfo (); ////// TODOC /// void CompleteSkip (int skipped); ////// TODOC /// Stream LoadResource (Uri uri, string mediaType); ////// TODOC /// Int32 EventInterest { get; } ////// TODOC /// int Actions { get; } ////// TODOC /// int Rate { get; } ////// TODOC /// int Volume { get; } } ////// TODOC /// #if !SPEECHSERVER public #else internal #endif class SkipInfo { internal SkipInfo (int type, int count) { _type = type; _count = count; } ////// TODOC /// public int Type { set { _type = value; } get { return _type; } } ////// TODOC /// public int Count { set { _count = value; } get { return _count; } } ////// TODOC /// public SkipInfo () { } private int _type; private int _count; } #endregion //******************************************************************** // // Public Types // //******************************************************************* #region Public Types ////// TODOC /// [StructLayout (LayoutKind.Sequential)] #if VSCOMPILE [DebuggerDisplay ("{State.Action} {TextToSpeak!=null?TextToSpeak:\"\"}")] #endif #if !SPEECHSERVER public #else internal #endif class TextFragment { ////// TODOC /// public TextFragment () { } ////// TODOC /// public FragmentState State { get { return _state; } set { _state = value; } } ////// TODOC /// public string TextToSpeak { get { return _textToSpeak; } set { Helpers.ThrowIfEmptyOrNull (value, "value"); _textToSpeak = value; } } ////// TODOC /// public int TextOffset { get { return _textOffset; } set { _textOffset = value; } } ////// TODOC /// public int TextLength { get { return _textLength; } set { _textLength = value; } } internal TextFragment (FragmentState fragState) : this (fragState, null, null, 0, 0) { } internal TextFragment (FragmentState fragState, string textToSpeak) : this (fragState, textToSpeak, textToSpeak, 0, textToSpeak.Length) { } internal TextFragment (FragmentState fragState, string textToSpeak, string textFrag, int offset, int length) { #if SPEECHSERVER || PROMPT_ENGINE if (fragState.Action == TtsEngineAction.PromptEngineSpeak || fragState.Action == TtsEngineAction.Speak || fragState.Action == TtsEngineAction.Pronounce) #else if (fragState.Action == TtsEngineAction.Speak || fragState.Action == TtsEngineAction.Pronounce) #endif { textFrag = textToSpeak; } if (!string.IsNullOrEmpty (textFrag)) { TextToSpeak = textFrag; } State = fragState; TextOffset = offset; TextLength = length; } private FragmentState _state; [MarshalAs (UnmanagedType.LPWStr)] private string _textToSpeak = string.Empty; private int _textOffset; private int _textLength; } ////// TODOC /// [ImmutableObject (true)] #if !SPEECHSERVER public #else internal #endif struct FragmentState : IEquatable{ /// /// TODOC /// public TtsEngineAction Action { get { return _action; } internal set { _action = value; } } ////// TODOC /// public int LangId { get { return _langId; } internal set { _langId = value; } } ////// TODOC /// public int Emphasis { get { return _emphasis; } internal set { _emphasis = value; } } ////// TODOC /// public int Duration { get { return _duration; } internal set { _duration = value; } } ////// TODOC /// public SayAs SayAs { get { return _sayAs; } internal set { Helpers.ThrowIfNull (value, "value"); _sayAs = value; } } ////// TODOC /// public Prosody Prosody { get { return _prosody; } internal set { Helpers.ThrowIfNull (value, "value"); _prosody = value; } } ////// TODOC /// public char [] Phoneme { get { return _phoneme; } internal set { Helpers.ThrowIfNull (value, "value"); _phoneme = value; } } /// TODOC public FragmentState (TtsEngineAction action, int langId, int emphasis, int duration, SayAs sayAs, Prosody prosody, char [] phonemes) { _action = action; _langId = langId; _emphasis = emphasis; _duration = duration; _sayAs = sayAs; _prosody = prosody; _phoneme = phonemes; } /// TODOC public static bool operator == (FragmentState state1, FragmentState state2) { return state1.Action == state2.Action && state1.LangId == state2.LangId && state1.Emphasis == state2.Emphasis && state1.Duration == state2.Duration && state1.SayAs == state2.SayAs && state1.Prosody == state2.Prosody && Array.Equals (state1.Phoneme, state2.Phoneme); } /// TODOC public static bool operator != (FragmentState state1, FragmentState state2) { return !(state1 == state2); } /// TODOC public bool Equals (FragmentState other) { return this == other; } /// TODOC public override bool Equals (object obj) { if (!(obj is FragmentState)) { return false; } return Equals ((FragmentState) obj); } /// TODOC public override int GetHashCode () { return base.GetHashCode (); } private TtsEngineAction _action; private int _langId; private int _emphasis; private int _duration; private SayAs _sayAs; private Prosody _prosody; private char [] _phoneme; } ////// TODOC /// [StructLayout (LayoutKind.Sequential)] #if !SPEECHSERVER public #else internal #endif class Prosody { ////// TODOC /// public ProsodyNumber Pitch { get { return _pitch; } set { _pitch = value; } } ////// TODOC /// public ProsodyNumber Range { get { return _range; } set { _range = value; } } ////// TODOC /// public ProsodyNumber Rate { get { return _rate; } set { _rate = value; } } ////// TODOC /// public int Duration { get { return _duration; } set { _duration = value; } } ////// TODOC /// public ProsodyNumber Volume { get { return _volume; } set { _volume = value; } } ////// TODOC /// public ContourPoint [] GetContourPoints () { return _contourPoints; } ////// TODOC /// public void SetContourPoints (ContourPoint [] points) { Helpers.ThrowIfNull (points, "points"); _contourPoints = (ContourPoint []) points.Clone (); } ////// TODOC /// public Prosody () { Pitch = new ProsodyNumber ((int) ProsodyPitch.Default); ; Range = new ProsodyNumber ((int) ProsodyRange.Default); ; Rate = new ProsodyNumber ((int) ProsodyRate.Default); Volume = new ProsodyNumber ((int) ProsodyVolume.Default); ; } internal Prosody Clone () { Prosody cloned = new Prosody (); cloned._pitch = _pitch; cloned._range = _range; cloned._rate = _rate; cloned._duration = _duration; cloned._volume = _volume; return cloned; } internal ProsodyNumber _pitch; internal ProsodyNumber _range; internal ProsodyNumber _rate; // can be casted to a Prosody Rate internal int _duration; internal ProsodyNumber _volume; internal ContourPoint [] _contourPoints; } ////// TODOC /// [ImmutableObject (true)] #if !SPEECHSERVER public #else internal #endif struct ContourPoint : IEquatable{ /// /// TODOC /// public float Start { get { return _start; } /* internal set { _start = value; } */} ////// TODOC /// public float Change { get { return _change; } /* internal set { _change = value; } */ } ////// TODOC /// public ContourPointChangeType ChangeType { get { return _changeType; } /* internal set { _changeType = value; } */ } ////// TODOC /// public ContourPoint (float start, float change, ContourPointChangeType changeType) { _start = start; _change = change; _changeType = changeType; } /// TODOC public static bool operator == (ContourPoint point1, ContourPoint point2) { return point1.Start.Equals (point2.Start) && point1.Change.Equals (point2.Change) && point1.ChangeType.Equals (point2.ChangeType); } /// TODOC public static bool operator != (ContourPoint point1, ContourPoint point2) { return !(point1 == point2); } /// TODOC public bool Equals (ContourPoint other) { return this == other; } /// TODOC public override bool Equals (object obj) { if (!(obj is ContourPoint)) { return false; } return Equals ((ContourPoint) obj); } /// TODOC public override int GetHashCode () { return base.GetHashCode (); } private float _start; private float _change; private ContourPointChangeType _changeType; } ////// TODOC /// [ImmutableObject (true)] #if !SPEECHSERVER public #else internal #endif struct ProsodyNumber : IEquatable{ /// /// TODOC /// public int SsmlAttributeId { get { return _ssmlAttributeId; } internal set { _ssmlAttributeId = value; } } ////// TODOC /// public bool IsNumberPercent { get { return _isPercent; } internal set { _isPercent = value; } } ////// TODOC /// public float Number { get { return _number; } internal set { _number = value; } } ////// TODOC /// public ProsodyUnit Unit { get { return _unit; } internal set { _unit = value; } } ////// TODOC /// public const int AbsoluteNumber = int.MaxValue; ////// TODOC /// public ProsodyNumber (int ssmlAttributeId) { _ssmlAttributeId = ssmlAttributeId; _number = 1.0f; _isPercent = true; _unit = ProsodyUnit.Default; } ////// TODOC /// public ProsodyNumber (float number) { _ssmlAttributeId = int.MaxValue; _number = number; _isPercent = false; _unit = ProsodyUnit.Default; } /// TODOC public static bool operator == (ProsodyNumber prosodyNumber1, ProsodyNumber prosodyNumber2) { return prosodyNumber1._ssmlAttributeId == prosodyNumber2._ssmlAttributeId && prosodyNumber1.Number.Equals (prosodyNumber2.Number) && prosodyNumber1.IsNumberPercent == prosodyNumber2.IsNumberPercent && prosodyNumber1.Unit == prosodyNumber2.Unit; } /// TODOC public static bool operator != (ProsodyNumber prosodyNumber1, ProsodyNumber prosodyNumber2) { return !(prosodyNumber1 == prosodyNumber2); } /// TODOC public bool Equals (ProsodyNumber other) { return this == other; } /// TODOC public override bool Equals (object obj) { if (!(obj is ProsodyNumber)) { return false; } return Equals ((ProsodyNumber) obj); } /// TODOC public override int GetHashCode () { return base.GetHashCode (); } private int _ssmlAttributeId; private bool _isPercent; private float _number; private ProsodyUnit _unit; } ////// TODOC /// [StructLayout (LayoutKind.Sequential)] #if !SPEECHSERVER public #else internal #endif class SayAs { ////// TODOC /// public string InterpretAs { get { return _interpretAs; } set { Helpers.ThrowIfEmptyOrNull (value, "value"); _interpretAs = value; } } ////// TODOC /// public string Format { get { return _format; } set { Helpers.ThrowIfEmptyOrNull (value, "value"); _format = value; } } ////// TODOC /// public string Detail { get { return _detail; } set { Helpers.ThrowIfEmptyOrNull (value, "value"); _detail = value; } } [MarshalAs (UnmanagedType.LPWStr)] private string _interpretAs; [MarshalAs (UnmanagedType.LPWStr)] private string _format; [MarshalAs (UnmanagedType.LPWStr)] private string _detail; } #endregion //******************************************************************** // // Public Enums // //******************************************************************** #region Public Enums ////// TODOC /// #if !SPEECHSERVER public #else internal #endif enum TtsEngineAction { ////// TODOC /// Speak, ////// TODOC /// Silence, ////// TODOC /// Pronounce, ////// TODOC /// Bookmark, ////// TODOC /// SpellOut, ////// TODOC /// StartSentence, ////// TODOC /// StartParagraph, ////// TODOC /// ParseUnknownTag, #if SPEECHSERVER || PROMPT_ENGINE ////// TODOC /// BeginPromptEngineOutput, ////// TODOC /// EndPromptEngineOutput, ////// TODOC /// PromptEngineDatabase, ////// TODOC /// PromptEngineSpeak, ////// TODOC /// PromptEngineId, ////// TODOC /// BeginPromptEngineWithTag, ////// TODOC /// EndPromptEngineWithTag, ////// TODOC /// BeginPromptEngineRule, ////// TODOC /// EndPromptEngineRule, ////// TODOC /// PromptEngineAudio, #endif } ////// TODOC /// #if !SPEECHSERVER public #else internal #endif enum EmphasisWord : int { ////// TODOC /// Default, ////// TODOC /// Strong, ////// TODOC /// Moderate, ////// TODOC /// None, ////// TODOC /// Reduced } ////// TODOC /// #if !SPEECHSERVER public #else internal #endif enum EmphasisBreak : int { ////// TODOC /// None = -1, ////// TODOC /// ExtraWeak = -2, ////// TODOC /// Weak = -3, ////// TODOC /// Medium = -4, ////// TODOC /// Strong = -5, ////// TODOC /// ExtraStrong = -6, ////// Equivalent to the empty Default = -7, } ////// /// TODOC /// #if !SPEECHSERVER public #else internal #endif enum ProsodyPitch { ////// TODOC /// Default, ////// TODOC /// ExtraLow, ////// TODOC /// Low, ////// TODOC /// Medium, ////// TODOC /// High, ////// TODOC /// ExtraHigh } ////// TODOC /// #if !SPEECHSERVER public #else internal #endif enum ProsodyRange { ////// TODOC /// Default, ////// TODOC /// ExtraLow, ////// TODOC /// Low, ////// TODOC /// Medium, ////// TODOC /// High, ////// TODOC /// ExtraHigh } ////// TODOC /// #if !SPEECHSERVER public #else internal #endif enum ProsodyRate { ////// TODOC /// Default, ////// TODOC /// ExtraSlow, ////// TODOC /// Slow, ////// TODOC /// Medium, ////// TODOC /// Fast, ////// TODOC /// ExtraFast } ////// TODOC /// #if !SPEECHSERVER public #else internal #endif enum ProsodyVolume : int { ////// TODOC /// Default = -1, ////// TODOC /// Silent = -2, ////// TODOC /// ExtraSoft = -3, ////// TODOC /// Soft = -4, ////// TODOC /// Medium = -5, ////// TODOC /// Loud = -6, ////// TODOC /// ExtraLoud = -7 } ////// TODOC /// #if !SPEECHSERVER public #else internal #endif enum ProsodyUnit : int { ////// TODOC /// Default, ////// TODOC /// Hz, ////// TODOC /// Semitone } ////// TODOC /// #if !SPEECHSERVER public #else internal #endif enum TtsEventId { ////// TODOC /// StartInputStream = 1, ////// TODOC /// EndInputStream = 2, ////// TODOC /// VoiceChange = 3, // lparam_is_token ////// TODOC /// Bookmark = 4, // lparam_is_string ////// TODOC /// WordBoundary = 5, ////// TODOC /// Phoneme = 6, ////// TODOC /// SentenceBoundary = 7, ////// TODOC /// Viseme = 8, ////// TODOC /// AudioLevel = 9, // wparam contains current output audio level #if SPEECHSERVER || PROMPT_ENGINE ////// TODOC /// Private = 15 #endif } ////// TODOC /// #if !SPEECHSERVER public #else internal #endif enum EventParameterType { ////// TODOC /// Undefined = 0x0000, ////// TODOC /// Token = 0x0001, ////// TODOC /// Object = 0x0002, ////// TODOC /// Pointer = 0x0003, ////// TODOC /// String = 0x0004 } ////// TODOC /// #if !SPEECHSERVER public #else internal #endif enum SpeakOutputFormat { ////// TODOC /// WaveFormat = 0, ////// TODOC /// Text = 1 } ////// TODOC /// #if !SPEECHSERVER public #else internal #endif enum ContourPointChangeType { ////// TODOC /// Hz = 0, ////// TODOC /// Percentage = 1 } #endregion //******************************************************************* // // Internal Interface // //******************************************************************** #region Internal Interface ////// TODOC /// [ComImport, Guid ("2D0FA0DB-AEA2-4AE2-9F8A-7AFC7794E56B"), InterfaceType (ComInterfaceType.InterfaceIsIUnknown)] #if PROMPT_ENGINE public interface ITtsEngineSsml #else internal interface ITtsEngineSsml #endif { ////// Queries the engine about a specific output format. /// The engine should examine the requested output format, /// and return the closest format that it supports. /// /// Wave or Text /// Expected format - null if no preferences /// Closest wave format header void GetOutputFormat (SpeakOutputFormat speakOutputFormat, IntPtr targetWaveFormat, out IntPtr waveHeader); ////// Add a lexicon to the engine collection of lexicons /// /// A path or a Uri /// media type /// Engine site (ITtsEngineSite) void AddLexicon (string location, string mediaType, IntPtr site); ////// Removes a lexicon to the engine collection of lexicons /// /// A path or a Uri /// Engine site (ITtsEngineSite) void RemoveLexicon (string location, IntPtr site); ////// Renders the specified text fragments array in the /// specified output format. /// /// Text fragment with SSML /// attributes information /// Number of elements in fragment /// Wave format header /// Engine site (ITtsEngineSite) void Speak (IntPtr fragments, int count, IntPtr waveHeader, IntPtr site); } ////// TODOC /// [StructLayout (LayoutKind.Sequential)] internal struct TextFragmentInterop { internal FragmentStateInterop _state; [MarshalAs (UnmanagedType.LPWStr)] internal string _textToSpeak; internal int _textOffset; internal int _textLength; internal static IntPtr FragmentToPtr (ListtextFragments, Collection memoryBlocks) { TextFragmentInterop fragInterop = new TextFragmentInterop (); int len = textFragments.Count; int sizeOfFrag = Marshal.SizeOf (fragInterop); IntPtr ret = Marshal.AllocCoTaskMem (sizeOfFrag * len); memoryBlocks.Add (ret); for (int i = 0; i < len; i++) { fragInterop._state.FragmentStateToPtr (textFragments [i].State, memoryBlocks); fragInterop._textToSpeak = textFragments [i].TextToSpeak; fragInterop._textOffset = textFragments [i].TextOffset; fragInterop._textLength = textFragments [i].TextLength; Marshal.StructureToPtr (fragInterop, (IntPtr) ((ulong) ret + (ulong) (i * sizeOfFrag)), false); } return ret; } } /// /// TODOC /// internal struct FragmentStateInterop { internal TtsEngineAction _action; internal int _langId; internal int _emphasis; internal int _duration; internal IntPtr _sayAs; internal IntPtr _prosody; internal IntPtr _phoneme; internal void FragmentStateToPtr (FragmentState state, CollectionmemoryBlocks) { _action = state.Action; _langId = state.LangId; _emphasis = state.Emphasis; _duration = state.Duration; if (state.SayAs != null) { _sayAs = Marshal.AllocCoTaskMem (Marshal.SizeOf (state.SayAs)); memoryBlocks.Add (_sayAs); Marshal.StructureToPtr (state.SayAs, _sayAs, false); } else { _sayAs = IntPtr.Zero; } if (state.Phoneme != null) { short [] phonemes = new short [state.Phoneme.Length + 1]; for (uint i = 0; i < state.Phoneme.Length; i++) { phonemes [i] = unchecked ((short) state.Phoneme [i]); } phonemes [state.Phoneme.Length] = 0; int sizeOfShort = Marshal.SizeOf (phonemes [0]); _phoneme = Marshal.AllocCoTaskMem (sizeOfShort * phonemes.Length); memoryBlocks.Add (_phoneme); for (uint i = 0; i < phonemes.Length; i++) { Marshal.Copy (phonemes, 0, _phoneme, phonemes.Length); } } else { _phoneme = IntPtr.Zero; } _prosody = ProsodyInterop.ProsodyToPtr (state.Prosody, memoryBlocks); } } /// /// TODOC /// [StructLayout (LayoutKind.Sequential)] internal struct ProsodyInterop { internal ProsodyNumber _pitch; internal ProsodyNumber _range; internal ProsodyNumber _rate; // can be casted to a Prosody Rate internal int _duration; internal ProsodyNumber _volume; internal IntPtr _contourPoints; internal static IntPtr ProsodyToPtr (Prosody prosody, CollectionmemoryBlocks) { if (prosody == null) { return IntPtr.Zero; } ProsodyInterop prosodyInterop = new ProsodyInterop (); prosodyInterop._pitch = prosody.Pitch; prosodyInterop._range = prosody.Range; prosodyInterop._rate = prosody.Rate; prosodyInterop._duration = prosody.Duration; prosodyInterop._volume = prosody.Volume; ContourPoint [] points = prosody.GetContourPoints (); if (points != null) { int sizeOfPoint = Marshal.SizeOf (points [0]); prosodyInterop._contourPoints = Marshal.AllocCoTaskMem (points.Length * sizeOfPoint); memoryBlocks.Add (prosodyInterop._contourPoints); for (uint i = 0; i < points.Length; i++) { Marshal.StructureToPtr (points [i], (IntPtr) ((ulong) prosodyInterop._contourPoints + (ulong) sizeOfPoint * i), false); } } else { prosodyInterop._contourPoints = IntPtr.Zero; } IntPtr ret = Marshal.AllocCoTaskMem (Marshal.SizeOf (prosodyInterop)); memoryBlocks.Add (ret); Marshal.StructureToPtr (prosodyInterop, ret, false); return ret; } } #endregion } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
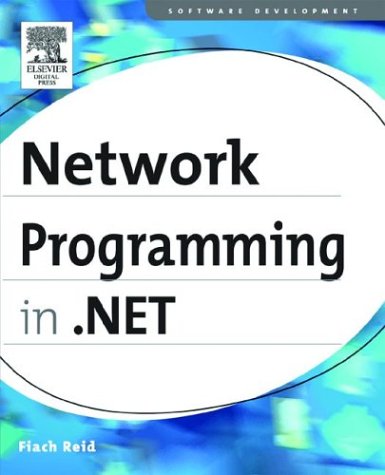
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WebChannelFactory.cs
- BamlLocalizableResourceKey.cs
- _LocalDataStore.cs
- TextUtf8RawTextWriter.cs
- AuthorizationRule.cs
- DisableDpiAwarenessAttribute.cs
- OleDbConnection.cs
- XamlInterfaces.cs
- Model3D.cs
- RuleSettings.cs
- IsolatedStorageException.cs
- MemberCollection.cs
- XmlSchemaImport.cs
- WebBrowser.cs
- ChannelServices.cs
- StringStorage.cs
- _LazyAsyncResult.cs
- ServiceNotStartedException.cs
- CalendarBlackoutDatesCollection.cs
- SmuggledIUnknown.cs
- BaseResourcesBuildProvider.cs
- ProxyFragment.cs
- SchemaTableColumn.cs
- SqlEnums.cs
- WorkflowCompensationBehavior.cs
- ObjectConverter.cs
- CqlLexer.cs
- ToolStripArrowRenderEventArgs.cs
- ToolboxComponentsCreatingEventArgs.cs
- PtsCache.cs
- ClientScriptManagerWrapper.cs
- WorkflowQueuingService.cs
- GifBitmapDecoder.cs
- PriorityQueue.cs
- ColumnReorderedEventArgs.cs
- TagPrefixInfo.cs
- TextPatternIdentifiers.cs
- ZoneIdentityPermission.cs
- BuildProviderAppliesToAttribute.cs
- ResourceSet.cs
- HasCopySemanticsAttribute.cs
- DataControlFieldsEditor.cs
- NetDataContractSerializer.cs
- System.Data_BID.cs
- CategoryNameCollection.cs
- DetailsViewPagerRow.cs
- HtmlWindow.cs
- XmlSchemaComplexContentRestriction.cs
- HyperLinkStyle.cs
- UIAgentAsyncBeginRequest.cs
- SchemaCollectionCompiler.cs
- FunctionUpdateCommand.cs
- SqlExpander.cs
- NameSpaceEvent.cs
- _LazyAsyncResult.cs
- CodeCommentStatementCollection.cs
- CounterNameConverter.cs
- EventMappingSettings.cs
- ImageConverter.cs
- X509CertificateCollection.cs
- TransportConfigurationTypeElementCollection.cs
- HttpAsyncResult.cs
- FontSourceCollection.cs
- Path.cs
- CaseStatementSlot.cs
- OptimalBreakSession.cs
- WindowsTreeView.cs
- DataPagerFieldCollection.cs
- Documentation.cs
- SrgsElement.cs
- EditingScopeUndoUnit.cs
- httpserverutility.cs
- WebBrowserEvent.cs
- CompiledAction.cs
- CodeSnippetTypeMember.cs
- XPathNode.cs
- SemanticBasicElement.cs
- SingleObjectCollection.cs
- ContentPlaceHolder.cs
- EastAsianLunisolarCalendar.cs
- ResourceManager.cs
- UnsafeNativeMethodsTablet.cs
- UpdateException.cs
- XXXInfos.cs
- SqlCacheDependencyDatabase.cs
- SessionPageStateSection.cs
- TextEndOfSegment.cs
- XAMLParseException.cs
- SessionPageStatePersister.cs
- ToolStripDropTargetManager.cs
- GraphicsPathIterator.cs
- Calendar.cs
- LayoutUtils.cs
- Version.cs
- ProvidersHelper.cs
- StyleCollectionEditor.cs
- InstallerTypeAttribute.cs
- ListSortDescriptionCollection.cs
- ConfigXmlReader.cs
- WaitHandle.cs