Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / TrustUi / MS / Internal / documents / RMPermissions.cs / 1 / RMPermissions.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: // RMPermissions. // // History: // 06/20/05 - [....] created // //--------------------------------------------------------------------------- using System; using System.Collections.Generic; using System.ComponentModel; using System.Drawing; using System.Text; using System.Windows.Forms; using System.Collections; using System.Windows.TrustUI; using System.Security; using System.Security.Permissions; using System.Security.RightsManagement; using MS.Internal.Documents.Application; namespace MS.Internal.Documents { ////// Class representing the Rights Management Permissions dialog. /// internal sealed partial class RMPermissionsDialog : DialogBaseForm { //------------------------------------------------------------------------- // Constructors //------------------------------------------------------------------------- #region Constructors ////// Constructor to use for non-owners /// /// The use license of the current user ////// Critical /// 1) A license object that contains critical information is passed /// in as an argument, but it is not saved except as strings to /// display in the UI. /// 2) Critical information about user objects is retrieved, but it is /// not saved except in the UI. /// 3) Calls critical function InitializeReferralInformation with the /// information passed in as a parameter. This function does not /// leak any critical information except again to show in the UI. /// [SecurityCritical] internal RMPermissionsDialog(RightsManagementLicense userLicense) { // Set up labels authenticatedAsLabel.Text = userLicense.LicensedUser.Name; expiresOnLabel.Text = GetUtcDateAsString(userLicense.ValidUntil); InitializeReferralInformation(userLicense); // Set up list of rights AddPermissions(GetRightsFromPermissions(userLicense)); } #endregion Constructors //-------------------------------------------------------------------------- // Private Methods //------------------------------------------------------------------------- #region Private Methods ////// Initialize the referral information (the name and the URI) to /// display in the UI. /// /// The license from which to retrieve the /// information to display ////// Critical /// 1) Sets UI based on object passed in as a parameter /// 2) Accesses critical properties of use license, but doesn't save /// or leak them except as strings to display in the UI or as /// critical member variables /// 3) Sets critical member _referralUri based on object passed in as /// a parameter /// [SecurityCritical] private void InitializeReferralInformation(RightsManagementLicense userLicense) { string referralName = userLicense.ReferralInfoName; Uri referralUri = userLicense.ReferralInfoUri; // The referral information displayed is: // - the referral URI. If that is not available, // - the referral name. If that is not available, // - the default text "Unknown" if (referralUri != null) { requestFromLabel.Text = referralUri.ToString(); } else if (!string.IsNullOrEmpty(referralName)) { requestFromLabel.Text = referralName; } // If the referral URI is a mailto URI, make the LinkLabel clickable if (referralUri != null && AddressUtility.IsMailtoUri(referralUri)) { // LinkLabels have one Link in the Links list by default requestFromLabel.Links[0].Description = referralName; // Set up the click handler; it uses _referralUri _referralUri = referralUri; requestFromLabel.LinkClicked += new LinkLabelLinkClickedEventHandler(requestFromLabel_LinkClicked); } else { // If there is no referral URI or it is not a mailto: link, the // label should not appear clickable. requestFromLabel.Links.Clear(); } } ////// Generates a string for each individual right granted to a user as represented /// in a RightsManagementLicense object. /// /// The license to convert ///An array of strings representing all rights granted private static string[] GetRightsFromPermissions(RightsManagementLicense license) { IListrightsStrings = new List (); if (license.HasPermission(RightsManagementPermissions.AllowOwner)) { rightsStrings.Add(SR.Get(SRID.RMPermissionsOwnerPermission)); } else { if (license.HasPermission(RightsManagementPermissions.AllowView)) { rightsStrings.Add(SR.Get(SRID.RMPermissionsViewPermission)); } if (license.HasPermission(RightsManagementPermissions.AllowPrint)) { rightsStrings.Add(SR.Get(SRID.RMPermissionsPrintPermission)); } if (license.HasPermission(RightsManagementPermissions.AllowCopy)) { rightsStrings.Add(SR.Get(SRID.RMPermissionsCopyPermission)); } if (license.HasPermission(RightsManagementPermissions.AllowSign)) { rightsStrings.Add(SR.Get(SRID.RMPermissionsSignPermission)); } if (license.HasPermission(RightsManagementPermissions.AllowAnnotate)) { rightsStrings.Add(SR.Get(SRID.RMPermissionsAnnotatePermission)); } } string[] stringArray = new string[rightsStrings.Count]; rightsStrings.CopyTo(stringArray, 0); return stringArray; } /// /// Returns a string that is the local representation (in the local /// time zone) of a UTC date /// /// The date to represent ///A string representing the date private static string GetUtcDateAsString(DateTime? date) { if (!date.HasValue || date.Value.Equals(DateTime.MaxValue)) { return SR.Get(SRID.RMPermissionsNoExpiration); } else { DateTime localDate = date.Value.ToLocalTime(); return localDate.ToShortDateString(); } } ////// The handler for when the "request permissions from" link is /// clicked. This navigates to the saved referral URI. /// /// Event sender (not used) /// Event arguments (not used) ///We save and use the cached referral URI for security /// reasons. This function is an event handler, so it cannot be marked /// critical and can be called by anything, so it is probably is not /// safe to read information from the argument. ////// Critical /// 1) Accesses critical data _referralUri /// 2) Calls critical function NavigationHelper.Navigate /// NotSafe /// 1) Could present a risk of a DoS attack because the function /// cannot confirm that this happened on user interaction /// [SecurityCritical] private void requestFromLabel_LinkClicked(object sender, LinkLabelLinkClickedEventArgs e) { // Navigate to the cached referral URI NavigationHelper.Navigate(new SecurityCriticalData(_referralUri)); } /// /// Adds the UI permission strings to the UI. /// /// private void AddPermissions(string[] uiStrings) { foreach (string permission in uiStrings) { if (!string.IsNullOrEmpty(permission)) { // Create a new label and add it to the permissionsFlowPanel Label permissionLabel = new Label(); permissionLabel.AutoSize = true; permissionLabel.Text = permission; permissionLabel.Margin = new Padding(13, 0, 3, 0); permissionsFlowPanel.Controls.Add(permissionLabel); } } } #endregion Private Methods //------------------------------------------------------ // Private Fields //------------------------------------------------------ #region Private Fields ////// The URI to contact for permissions. /// ////// Critical - Personally identifiable information /// [SecurityCritical] private Uri _referralUri; #endregion Private Fields //----------------------------------------------------- // Protected Methods //------------------------------------------------------ #region Protected Methods ////// ApplyResources override. Called to apply dialog resources. /// protected override void ApplyResources() { base.ApplyResources(); Text = SR.Get(SRID.RMPermissionsTitle); authenticatedAsTextLabel.Text = SR.Get(SRID.RMPermissionsAuthenticatedAs); permissionsHeldLabel.Text = SR.Get(SRID.RMPermissionsHavePermissions); requestFromTextLabel.Text = SR.Get(SRID.RMPermissionsRequestFrom); requestFromLabel.Text = SR.Get(SRID.RMPermissionsUnknownOwner); expiresOnTextLabel.Text = SR.Get(SRID.RMPermissionsExpiresOn); closeButton.Text = SR.Get(SRID.RMPermissionsCloseButton); } #endregion Protected Methods } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
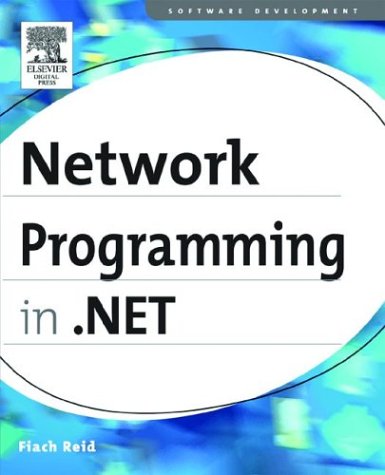
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Error.cs
- XmlWrappingWriter.cs
- SmiEventSink_Default.cs
- PersonalizationProviderHelper.cs
- ISessionStateStore.cs
- ErrorStyle.cs
- RulePatternOps.cs
- TraceSection.cs
- ValidationVisibilityAttribute.cs
- DataControlButton.cs
- Cursors.cs
- WindowInteropHelper.cs
- MobileControlPersister.cs
- Scheduler.cs
- PointHitTestResult.cs
- UnsafeNativeMethods.cs
- FormViewPageEventArgs.cs
- SqlClientPermission.cs
- cookiecollection.cs
- OpenTypeLayoutCache.cs
- CryptoApi.cs
- HelpProvider.cs
- SqlDataSourceStatusEventArgs.cs
- DeferredReference.cs
- CodeEventReferenceExpression.cs
- objectquery_tresulttype.cs
- XmlCustomFormatter.cs
- X509Certificate.cs
- InkCanvasInnerCanvas.cs
- ListItemCollection.cs
- Domain.cs
- XamlStyleSerializer.cs
- TextEndOfLine.cs
- OLEDB_Enum.cs
- BookmarkEventArgs.cs
- PartDesigner.cs
- RepeaterItemCollection.cs
- MailHeaderInfo.cs
- CrossAppDomainChannel.cs
- MethodBuilder.cs
- VirtualPath.cs
- XmlObjectSerializer.cs
- BrowserDefinition.cs
- SqlPersonalizationProvider.cs
- ProcessModelSection.cs
- LocationUpdates.cs
- SqlDataRecord.cs
- DataGridViewCell.cs
- DiscoveryClientProtocol.cs
- ReadOnlyState.cs
- StandardCommands.cs
- SecurityTokenTypes.cs
- CompilerHelpers.cs
- OutputCache.cs
- ArcSegment.cs
- PropertyReference.cs
- HandleCollector.cs
- PageRanges.cs
- InvokeHandlers.cs
- SizeChangedInfo.cs
- GridProviderWrapper.cs
- XmlSchemaType.cs
- CodeThrowExceptionStatement.cs
- PeerApplication.cs
- WmpBitmapEncoder.cs
- Line.cs
- DetailsViewPagerRow.cs
- ConfigXmlWhitespace.cs
- RuntimeConfigurationRecord.cs
- SuppressMergeCheckAttribute.cs
- ColumnHeaderConverter.cs
- WebBaseEventKeyComparer.cs
- SafeNativeMethods.cs
- StringAnimationUsingKeyFrames.cs
- ListCardsInFileRequest.cs
- MeasurementDCInfo.cs
- HttpAsyncResult.cs
- EmptyElement.cs
- BoundColumn.cs
- FormViewPagerRow.cs
- TextDecorationUnitValidation.cs
- DbConnectionClosed.cs
- SimpleHandlerFactory.cs
- _SSPIWrapper.cs
- BamlCollectionHolder.cs
- TrackingAnnotationCollection.cs
- SrgsRulesCollection.cs
- AttributeTable.cs
- XamlVector3DCollectionSerializer.cs
- ToolStripContainerActionList.cs
- SimpleHandlerFactory.cs
- ProcessHostMapPath.cs
- TraceContextEventArgs.cs
- MgmtConfigurationRecord.cs
- SqlException.cs
- ReferenceList.cs
- TypeForwardedFromAttribute.cs
- ScriptReferenceBase.cs
- ColumnMapVisitor.cs
- DesignSurface.cs