Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / CompMod / System / ComponentModel / Design / Serialization / PrimitiveCodeDomSerializer.cs / 1 / PrimitiveCodeDomSerializer.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.ComponentModel.Design.Serialization { using System; using System.CodeDom; using System.CodeDom.Compiler; using System.Collections; using System.ComponentModel; using System.ComponentModel.Design; using System.Diagnostics; using System.Reflection; ////// /// Code model serializer for primitive types. /// internal class PrimitiveCodeDomSerializer : CodeDomSerializer { private static readonly string JSharpFileExtension = ".jsl"; private static PrimitiveCodeDomSerializer defaultSerializer; ////// /// Retrieves a default static instance of this serializer. /// internal new static PrimitiveCodeDomSerializer Default { get { if (defaultSerializer == null) { defaultSerializer = new PrimitiveCodeDomSerializer(); } return defaultSerializer; } } ////// /// Serializes the given object into a CodeDom object. /// public override object Serialize(IDesignerSerializationManager manager, object value) { using (TraceScope("PrimitiveCodeDomSerializer::Serialize")) { Trace("Value: {0}", (value == null ? "(null)" : value.ToString())); } CodeExpression expression = new CodePrimitiveExpression(value); if (value != null) { if (value is bool || value is char || value is int || value is float || value is double) { // Hack for J#, since they don't support auto-boxing of value types yet. CodeDomProvider codeProvider = manager.GetService(typeof(CodeDomProvider)) as CodeDomProvider; if (codeProvider != null && String.Equals(codeProvider.FileExtension, JSharpFileExtension)) { // See if we are boxing - if so, insert a cast. ExpressionContext cxt = manager.Context[typeof(ExpressionContext)] as ExpressionContext; Debug.Assert(cxt != null, "No expression context on stack - J# boxing cast will not be inserted"); if (cxt != null) { if (cxt.ExpressionType == typeof(object)) { expression = new CodeCastExpression(value.GetType(), expression); expression.UserData.Add("CastIsBoxing", true); } } } } else if (value is string) { string stringValue = value as string; if (stringValue != null && stringValue.Length > 200) { expression = SerializeToResourceExpression(manager, stringValue); } } else { // Generate a cast for all other types because we won't parse them properly otherwise // because we won't know to convert them to the narrow form. expression = new CodeCastExpression(new CodeTypeReference(value.GetType()), expression); } } return expression; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
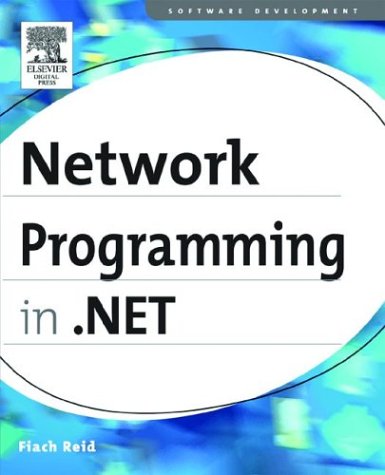
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SAPIEngineTypes.cs
- WebBrowserHelper.cs
- MSAANativeProvider.cs
- QilInvokeLateBound.cs
- Stacktrace.cs
- EventManager.cs
- AuthenticationManager.cs
- RadioButton.cs
- IntegerCollectionEditor.cs
- NegotiateStream.cs
- OptimalBreakSession.cs
- OperationContext.cs
- Deflater.cs
- ProfileSection.cs
- CheckBox.cs
- PerformanceCounterCategory.cs
- HandlerFactoryCache.cs
- RSAPKCS1KeyExchangeFormatter.cs
- SqlCacheDependencySection.cs
- Pointer.cs
- Label.cs
- TrackingStringDictionary.cs
- CmsInterop.cs
- SqlDataReader.cs
- XPathPatternBuilder.cs
- InputScopeManager.cs
- PerformanceCounterManager.cs
- WindowsTokenRoleProvider.cs
- CollectionTraceRecord.cs
- CreateRefExpr.cs
- ColumnPropertiesGroup.cs
- MyContact.cs
- TransformerConfigurationWizardBase.cs
- LoadWorkflowByInstanceKeyCommand.cs
- GridViewSortEventArgs.cs
- PropertyInformation.cs
- COM2IVsPerPropertyBrowsingHandler.cs
- regiisutil.cs
- Header.cs
- sqlnorm.cs
- ProgressBarHighlightConverter.cs
- MemberMaps.cs
- PagesSection.cs
- CodeParameterDeclarationExpression.cs
- DecoderExceptionFallback.cs
- ObjectKeyFrameCollection.cs
- MessageContractExporter.cs
- ErrorCodes.cs
- ProcessHostServerConfig.cs
- SystemColors.cs
- FullTextState.cs
- _StreamFramer.cs
- Gdiplus.cs
- SizeKeyFrameCollection.cs
- Rect3D.cs
- ProfileEventArgs.cs
- IgnoreFileBuildProvider.cs
- SafeSecurityHelper.cs
- BaseDataListComponentEditor.cs
- TemplatePartAttribute.cs
- FlowPanelDesigner.cs
- ClientSideProviderDescription.cs
- StatusBar.cs
- WebPartMovingEventArgs.cs
- MD5CryptoServiceProvider.cs
- DataGridViewCellStyleConverter.cs
- Annotation.cs
- ProcessRequestArgs.cs
- DesignerUtility.cs
- SQLInt32.cs
- GeometryGroup.cs
- DataGridViewColumnEventArgs.cs
- PasswordBox.cs
- InArgumentConverter.cs
- NavigationEventArgs.cs
- ClientSettingsStore.cs
- TargetPerspective.cs
- GridViewAutomationPeer.cs
- WindowsPen.cs
- ProfileGroupSettings.cs
- AttachmentCollection.cs
- DefaultValueAttribute.cs
- BasicExpressionVisitor.cs
- LineServicesCallbacks.cs
- ZipIOExtraFieldPaddingElement.cs
- Frame.cs
- XPathArrayIterator.cs
- ItemAutomationPeer.cs
- InnerItemCollectionView.cs
- GetTokenRequest.cs
- GlobalItem.cs
- XmlTypeAttribute.cs
- ClassicBorderDecorator.cs
- OptimizedTemplateContentHelper.cs
- BitmapDecoder.cs
- FlowNode.cs
- MinimizableAttributeTypeConverter.cs
- DefaultTextStore.cs
- OutputCacheProfileCollection.cs
- DurationConverter.cs