Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / System / data / design / NameService.cs / 2 / NameService.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All Rights Reserved. // Information Contained Herein is Proprietary and Confidential. // //----------------------------------------------------------------------------- namespace System.Data.Design { using System; using System.Collections; using System.Globalization; ////// internal interface INamedObject { string Name { get; set; } } ////// Indicates a collection of objects that support INamedObject. /// internal interface INamedObjectCollection: ICollection { INameService GetNameService(); // Might return null. } ////// A name service can be used for many INamedObjectCollection /// internal interface INameService { // Create UniqueName will always return a valid and unique name // string CreateUniqueName( INamedObjectCollection container, Type type ); string CreateUniqueName( INamedObjectCollection container, string proposed ); string CreateUniqueName( INamedObjectCollection container, string proposedNameRoot, int startSuffix); // ValidateName does not check if the name is unque void ValidateName( string name ); // Should throw NameValidationException when invalid name passed. // Check if the name is unique and valid void ValidateUniqueName( INamedObjectCollection container, string name ); // Should throw NameValidationException when invalid name passed. // Check if the name is unique and valid // This function is useful when renaming an existing object. void ValidateUniqueName(INamedObjectCollection container, INamedObject namedObject, string proposedName); // Should throw NameValidationException when invalid name passed. } ////// internal class NamedObjectUtil { ////// Private contstructor to avoid class being instantiated. /// private NamedObjectUtil() { } public static INamedObject Find( INamedObjectCollection coll, string name ) { return NamedObjectUtil.Find( (ICollection) coll, name, false); } private static INamedObject Find( ICollection coll, string name, bool ignoreCase) { IEnumerator e = coll.GetEnumerator(); while( e.MoveNext() ) { INamedObject n = e.Current as INamedObject; if( n == null ) { throw new InternalException( VSDExceptions.COMMON.NOT_A_NAMED_OBJECT_MSG, VSDExceptions.COMMON.NOT_A_NAMED_OBJECT_CODE ); } if( StringUtil.EqualValue(n.Name, name, ignoreCase)) { return n; } } return null; } } ////// [Serializable] internal sealed class NameValidationException: ApplicationException { public NameValidationException( string message ) : base( message ) {} // No additional fields defined so we do not have to override default ISerializable implementation } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
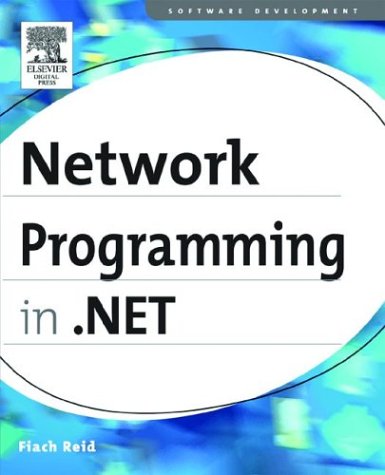
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- FixedSOMElement.cs
- ImageUrlEditor.cs
- RepeatBehaviorConverter.cs
- Pair.cs
- ClientSettings.cs
- WorkflowDebuggerSteppingAttribute.cs
- Single.cs
- SmtpFailedRecipientsException.cs
- StylusButton.cs
- ConcurrentStack.cs
- GAC.cs
- DirectionalLight.cs
- Schedule.cs
- Privilege.cs
- ImageIndexConverter.cs
- ApplicationBuildProvider.cs
- HtmlFormParameterReader.cs
- WpfKnownTypeInvoker.cs
- ExpressionDumper.cs
- SspiSecurityToken.cs
- httpstaticobjectscollection.cs
- XmlSchemaSimpleType.cs
- ImportContext.cs
- MissingSatelliteAssemblyException.cs
- CodeDomLocalizationProvider.cs
- FileLoadException.cs
- TextParaLineResult.cs
- InstanceDataCollectionCollection.cs
- XmlSchemas.cs
- ZipPackagePart.cs
- CollectionEditVerbManager.cs
- RepeaterCommandEventArgs.cs
- SystemWebCachingSectionGroup.cs
- TextBoxAutoCompleteSourceConverter.cs
- ExportOptions.cs
- WebColorConverter.cs
- DataGridViewColumnCollection.cs
- ThreadLocal.cs
- EntityCommandDefinition.cs
- ApplicationException.cs
- AuthenticationConfig.cs
- TransformerConfigurationWizardBase.cs
- DoubleConverter.cs
- PropertyRef.cs
- BaseDataList.cs
- SQLSingleStorage.cs
- ObjectStateEntryDbUpdatableDataRecord.cs
- FontDriver.cs
- UiaCoreTypesApi.cs
- AssemblyName.cs
- BmpBitmapDecoder.cs
- Utility.cs
- ITreeGenerator.cs
- List.cs
- WebRequest.cs
- PropertyConverter.cs
- ToolStripGrip.cs
- ExtendedProtectionPolicyElement.cs
- ContactManager.cs
- Grammar.cs
- OAVariantLib.cs
- FileStream.cs
- CodeArrayIndexerExpression.cs
- SendAgentStatusRequest.cs
- ExpressionVisitorHelpers.cs
- GeneralTransformCollection.cs
- PeerName.cs
- ProcessHostConfigUtils.cs
- XhtmlTextWriter.cs
- XmlCustomFormatter.cs
- ComponentResourceKeyConverter.cs
- TrackPointCollection.cs
- SRGSCompiler.cs
- SqlFacetAttribute.cs
- WizardStepCollectionEditor.cs
- DataBinder.cs
- KeyTime.cs
- ComplexObject.cs
- XmlSchemaObjectTable.cs
- Monitor.cs
- SqlErrorCollection.cs
- StandardBindingElement.cs
- smtppermission.cs
- CmsInterop.cs
- ProfileSettingsCollection.cs
- OdbcRowUpdatingEvent.cs
- CompressionTransform.cs
- ScopelessEnumAttribute.cs
- ThaiBuddhistCalendar.cs
- DBConnectionString.cs
- DataGridViewAdvancedBorderStyle.cs
- CacheSection.cs
- IBuiltInEvidence.cs
- TextTreeFixupNode.cs
- JsonWriterDelegator.cs
- exports.cs
- SystemIPInterfaceProperties.cs
- ExclusiveTcpListener.cs
- PublishLicense.cs
- XmlDataImplementation.cs