Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / WinForms / System / WinForms / Design / DataGridViewComboBoxColumnDesigner.cs / 1 / DataGridViewComboBoxColumnDesigner.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms.Design { using System.Design; using System.ComponentModel; using System.Diagnostics; using System; using System.Collections; using System.Windows.Forms; using System.Data; using System.ComponentModel.Design; using System.Drawing; using Microsoft.Win32; using System.Windows.Forms.ComponentModel; ////// /// internal class DataGridViewComboBoxColumnDesigner : DataGridViewColumnDesigner { static BindingContext bc; private string ValueMember { get { DataGridViewComboBoxColumn col = (DataGridViewComboBoxColumn) this.Component; return col.ValueMember; } set { DataGridViewComboBoxColumn col = (DataGridViewComboBoxColumn) this.Component; if (col.DataSource == null) { return; } if (ValidDataMember(col.DataSource, value)) { col.ValueMember = value; } } } private string DisplayMember { get { DataGridViewComboBoxColumn col = (DataGridViewComboBoxColumn) this.Component; return col.DisplayMember; } set { DataGridViewComboBoxColumn col = (DataGridViewComboBoxColumn) this.Component; if (col.DataSource == null) { return; } if (ValidDataMember(col.DataSource, value)) { col.DisplayMember = value; } } } private bool ShouldSerializeDisplayMember() { DataGridViewComboBoxColumn col = (DataGridViewComboBoxColumn) this.Component; return !String.IsNullOrEmpty(col.DisplayMember); } private bool ShouldSerializeValueMember() { DataGridViewComboBoxColumn col = (DataGridViewComboBoxColumn) this.Component; return !String.IsNullOrEmpty(col.ValueMember); } private static bool ValidDataMember(object dataSource, string dataMember) { if (String.IsNullOrEmpty(dataMember)) { // a null string is a valid value return true; } if (bc == null) { bc = new BindingContext(); } // // scrub the hashTable inside the BindingContext every time we access this method. // int count = ((ICollection) bc).Count; BindingMemberInfo bmi = new BindingMemberInfo(dataMember); PropertyDescriptorCollection props = null; BindingManagerBase bmb; try { bmb = bc[dataSource, bmi.BindingPath]; } catch (System.ArgumentException) { return false; } if (bmb == null) { return false; } props = bmb.GetItemProperties(); if (props == null) { return false; } if (props[bmi.BindingField] == null) { return false; } return true; } protected override void PreFilterProperties(IDictionary properties) { base.PreFilterProperties(properties); PropertyDescriptor prop = (PropertyDescriptor) properties["ValueMember"]; if (prop != null) { properties["ValueMember"] = TypeDescriptor.CreateProperty(typeof(DataGridViewComboBoxColumnDesigner), prop, new Attribute[0]); } prop = (PropertyDescriptor) properties["DisplayMember"]; if (prop != null) { properties["DisplayMember"] = TypeDescriptor.CreateProperty(typeof(DataGridViewComboBoxColumnDesigner), prop, new Attribute[0]); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.Provides a base designer for data grid view columns. ///
Link Menu
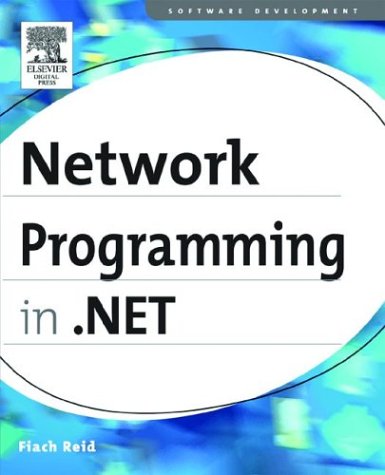
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DragCompletedEventArgs.cs
- FixedSOMPage.cs
- ContentPresenter.cs
- GroupBox.cs
- FocusManager.cs
- _LoggingObject.cs
- ControlBuilder.cs
- Configuration.cs
- BufferedReadStream.cs
- UserInitiatedNavigationPermission.cs
- WCFServiceClientProxyGenerator.cs
- _Win32.cs
- TextTreeObjectNode.cs
- HashCodeCombiner.cs
- HttpDigestClientCredential.cs
- ClientSponsor.cs
- DragSelectionMessageFilter.cs
- DateBoldEvent.cs
- propertyentry.cs
- BaseTemplateBuildProvider.cs
- TransformCryptoHandle.cs
- TickBar.cs
- UrlAuthFailedErrorFormatter.cs
- KnownTypesHelper.cs
- RowsCopiedEventArgs.cs
- BindingCollectionElement.cs
- DateTimeParse.cs
- ProcessThread.cs
- CompilationRelaxations.cs
- MetadataResolver.cs
- DebuggerAttributes.cs
- EventMap.cs
- TdsParameterSetter.cs
- ContractBase.cs
- Rect3D.cs
- SafeProcessHandle.cs
- CorrelationManager.cs
- ASCIIEncoding.cs
- HtmlInputReset.cs
- DynamicDataResources.Designer.cs
- DockingAttribute.cs
- CaseExpr.cs
- ProxyAttribute.cs
- PermissionListSet.cs
- BezierSegment.cs
- ContentValidator.cs
- MetadataCache.cs
- LicFileLicenseProvider.cs
- OdbcEnvironment.cs
- EmulateRecognizeCompletedEventArgs.cs
- ExpressionHelper.cs
- SocketAddress.cs
- CopyOfAction.cs
- ConnectionStringsExpressionEditor.cs
- IODescriptionAttribute.cs
- Thread.cs
- ZipIOCentralDirectoryDigitalSignature.cs
- CompleteWizardStep.cs
- MaxMessageSizeStream.cs
- UpdateException.cs
- TypeDelegator.cs
- OrderToken.cs
- MailBnfHelper.cs
- basenumberconverter.cs
- HttpConfigurationContext.cs
- InstancePersistenceException.cs
- ParserOptions.cs
- MdiWindowListStrip.cs
- ListViewInsertedEventArgs.cs
- ScriptRef.cs
- Form.cs
- CommandDesigner.cs
- FileIOPermission.cs
- WriteFileContext.cs
- DataGridViewRowEventArgs.cs
- ConfigXmlAttribute.cs
- PropertyEmitterBase.cs
- ObjectDataSourceView.cs
- MethodImplAttribute.cs
- CorrelationService.cs
- XmlAggregates.cs
- TransformGroup.cs
- DBConcurrencyException.cs
- BamlTreeUpdater.cs
- Compiler.cs
- Context.cs
- FillRuleValidation.cs
- CollectionViewGroup.cs
- ReferentialConstraintRoleElement.cs
- SuppressMessageAttribute.cs
- NotFiniteNumberException.cs
- PrimaryKeyTypeConverter.cs
- ProgressBarBrushConverter.cs
- JavaScriptObjectDeserializer.cs
- SingleQueryOperator.cs
- DbTypeMap.cs
- WorkflowOwnerAsyncResult.cs
- CssClassPropertyAttribute.cs
- DesignTimeParseData.cs
- XmlSchemaComplexContentExtension.cs