Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / ndp / fx / src / DataEntity / System / Data / Metadata / Edm / EdmProperty.cs / 2 / EdmProperty.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....], [....] //--------------------------------------------------------------------- using System.Data.Common; using System.Threading; namespace System.Data.Metadata.Edm { ////// Represent the edm property class /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly", MessageId = "Edm")] public sealed class EdmProperty : EdmMember { #region Constructors ////// Initializes a new instance of the property class /// /// name of the property /// TypeUsage object containing the property type and its facets ///Thrown if name or typeUsage arguments are null ///Thrown if name argument is empty string internal EdmProperty(string name, TypeUsage typeUsage) : base(name, typeUsage) { EntityUtil.CheckStringArgument(name, "name"); EntityUtil.GenericCheckArgumentNull(typeUsage, "typeUsage"); } #endregion #region Fields ///Store the handle, allowing the PropertyInfo/MethodInfo/Type references to be GC'd internal readonly System.RuntimeMethodHandle PropertyGetterHandle; ///Store the handle, allowing the PropertyInfo/MethodInfo/Type references to be GC'd internal readonly System.RuntimeMethodHandle PropertySetterHandle; ///cached dynamic method to get the property value from a CLR instance private Func
Link Menu
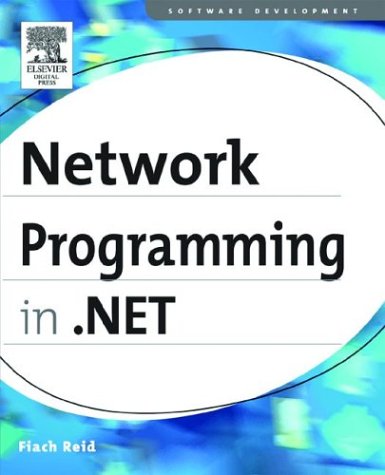
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ChannelServices.cs
- XmlRawWriter.cs
- SerializableTypeCodeDomSerializer.cs
- CopyOnWriteList.cs
- PeerEndPoint.cs
- DocumentEventArgs.cs
- ReflectionHelper.cs
- AssemblyResourceLoader.cs
- QuaternionValueSerializer.cs
- Semaphore.cs
- RelatedView.cs
- EnumerableCollectionView.cs
- PropertyRecord.cs
- FlowDocumentPageViewerAutomationPeer.cs
- TextTreeNode.cs
- DelimitedListTraceListener.cs
- HtmlEmptyTagControlBuilder.cs
- DecimalKeyFrameCollection.cs
- SerialErrors.cs
- CultureTable.cs
- ShapingWorkspace.cs
- HtmlInputCheckBox.cs
- WindowsFormsEditorServiceHelper.cs
- PropagatorResult.cs
- PageCodeDomTreeGenerator.cs
- BinaryConverter.cs
- CodeIndexerExpression.cs
- SharedStatics.cs
- CustomSignedXml.cs
- XmlUtf8RawTextWriter.cs
- DataColumnPropertyDescriptor.cs
- CompilationSection.cs
- ResolvedKeyFrameEntry.cs
- EventDescriptor.cs
- GZipDecoder.cs
- QueuePathEditor.cs
- PaintValueEventArgs.cs
- FilteredAttributeCollection.cs
- AtomEntry.cs
- DataGridViewToolTip.cs
- WebPartVerbCollection.cs
- SaveFileDialog.cs
- NavigateEvent.cs
- OracleCommand.cs
- PasswordValidationException.cs
- TypedServiceChannelBuilder.cs
- SiteMapHierarchicalDataSourceView.cs
- OperationCanceledException.cs
- TextElementEnumerator.cs
- DesignBinding.cs
- ScaleTransform3D.cs
- KnownBoxes.cs
- WinEventHandler.cs
- TabControlCancelEvent.cs
- UseAttributeSetsAction.cs
- DoubleLinkList.cs
- UnsafeNativeMethods.cs
- SqlReferenceCollection.cs
- XPathException.cs
- DbProviderFactoriesConfigurationHandler.cs
- MetadataCollection.cs
- EventMappingSettingsCollection.cs
- DataGridViewComboBoxColumn.cs
- WebPartTransformerAttribute.cs
- counter.cs
- PermissionSetEnumerator.cs
- XmlObjectSerializerWriteContextComplex.cs
- TypeHelper.cs
- BigInt.cs
- EventDescriptor.cs
- CodeDOMProvider.cs
- ActivityExecutorDelegateInfo.cs
- GeneratedCodeAttribute.cs
- MarshalByRefObject.cs
- AssociationTypeEmitter.cs
- DesignerAttribute.cs
- BaseCodePageEncoding.cs
- ClientConfigPaths.cs
- OdbcUtils.cs
- DefaultParameterValueAttribute.cs
- IItemContainerGenerator.cs
- Shape.cs
- TextTrailingCharacterEllipsis.cs
- CompositionTarget.cs
- FrameworkElementFactory.cs
- _PooledStream.cs
- UncommonField.cs
- WindowPattern.cs
- AliasedSlot.cs
- TransformCollection.cs
- Operators.cs
- ProtocolsSection.cs
- XmlParser.cs
- HtmlFormWrapper.cs
- CheckedListBox.cs
- MetaChildrenColumn.cs
- CatalogPart.cs
- JpegBitmapDecoder.cs
- CatalogZone.cs
- TaskExceptionHolder.cs