Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / ndp / fx / src / DataEntity / System / Data / Objects / ELinq / BindingContext.cs / 2 / BindingContext.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] //--------------------------------------------------------------------- using CqtExpression = System.Data.Common.CommandTrees.DbExpression; using LinqExpression = System.Linq.Expressions.Expression; using System.Linq.Expressions; using System.Collections.ObjectModel; using System.Linq; using System.Collections.Generic; using System.Data.Common.CommandTrees; using System.Data.Metadata.Edm; using System.Reflection; using System.Data.Common.EntitySql; using System.Diagnostics; using System.Data.Common; using System.Globalization; namespace System.Data.Objects.ELinq { ////// Class containing binding information for an expression converter (associating CQT bindings /// with LINQ lambda parameter) /// ////// Usage pattern: /// internal sealed class BindingContext { private readonly Binding _rootContextBinding; private readonly List/// BindingContext context = ...; /// /// // translate a "Where" lamba expression input.Where(i => i.X > 2); /// LambdaExpression whereLambda = ...; /// CqtExpression inputCqt = Translate(whereLambda.Arguments[1]); /// CqtExpression inputBinding = CreateExpressionBinding(inputCqt).Var; /// /// // push the scope defined by the parameter /// context.PushBindingScope(new KeyValuePair{ParameterExpression, CqtExpression}(whereLambda.Parameters[0], inputBinding)); /// /// // translate the expression in this context /// CqtExpression result = Translate(whereLambda.Expression); /// /// // pop the scope /// context.PopBindingScope(); ///
///_scopes; internal readonly ObjectContext ObjectContext; /// /// Initialize a new binding context /// internal BindingContext(ParameterExpression rootContextParameter, ObjectContext objectContext, CompiledQueryParameter [] compiledQueryParameters ) : this() { Debug.Assert(null != rootContextParameter && null != objectContext, "missing required args to BindingContext"); _rootContextBinding = new Binding(rootContextParameter, null); this.ObjectContext = objectContext; PushBindingScope(_rootContextBinding); foreach (var parameter in compiledQueryParameters) { Binding binding = new Binding(parameter.Expression, parameter.ParameterReference); PushBindingScope(binding); } } ////// Initialize a new binding context /// internal BindingContext() { _scopes = new List(); } /// /// Set up a new binding scope where parameter expressions map to their paired CQT expressions. /// /// Parameter/Expression bindings internal void PushBindingScope(params Binding[] bindings) { _scopes.Add(bindings); } ////// Removes a scope when leaving a particular sub-expression. /// ///Scope. internal void PopBindingScope() { _scopes.RemoveAt(_scopes.Count - 1); } internal bool TryGetBoundExpression(Expression linqExpression, out CqtExpression cqtExpression) { Binding binding; if (TryGetBinding(linqExpression, out binding)) { if (binding != _rootContextBinding) { cqtExpression = binding.CqtExpression; return true; } } cqtExpression = null; return false; } internal bool IsRootContextParameter(ParameterExpression parameter) { Binding binding; if (TryGetBinding(parameter, out binding)) { return _rootContextBinding == binding; } return false; } // Try to find a CQT expression that is the translation of a LINQ parameter. private bool TryGetBinding(Expression parameter, out Binding binding) { binding = null; // if there are no binding scopes, their can be no binding if (_scopes.Count == 0) { return false; } for (int i = _scopes.Count - 1; i >= 0; i--) { Binding[] scope = _scopes[i]; foreach (Binding scopeBinding in scope) { if (parameter == scopeBinding.LinqExpression) { binding = scopeBinding; return true; } } } // no match found return false; } } ////// Class describing a LINQ parameter and its bound expression. For instance, in /// /// products.Select(p => p.ID) /// /// the 'products' query is the bound expression, and 'p' is the parameter. /// internal sealed class Binding { internal Binding(Expression linqExpression, CqtExpression cqtExpression) { LinqExpression = linqExpression; CqtExpression = cqtExpression; } internal readonly Expression LinqExpression; internal readonly CqtExpression CqtExpression; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] //--------------------------------------------------------------------- using CqtExpression = System.Data.Common.CommandTrees.DbExpression; using LinqExpression = System.Linq.Expressions.Expression; using System.Linq.Expressions; using System.Collections.ObjectModel; using System.Linq; using System.Collections.Generic; using System.Data.Common.CommandTrees; using System.Data.Metadata.Edm; using System.Reflection; using System.Data.Common.EntitySql; using System.Diagnostics; using System.Data.Common; using System.Globalization; namespace System.Data.Objects.ELinq { ////// Class containing binding information for an expression converter (associating CQT bindings /// with LINQ lambda parameter) /// ////// Usage pattern: /// internal sealed class BindingContext { private readonly Binding _rootContextBinding; private readonly List/// BindingContext context = ...; /// /// // translate a "Where" lamba expression input.Where(i => i.X > 2); /// LambdaExpression whereLambda = ...; /// CqtExpression inputCqt = Translate(whereLambda.Arguments[1]); /// CqtExpression inputBinding = CreateExpressionBinding(inputCqt).Var; /// /// // push the scope defined by the parameter /// context.PushBindingScope(new KeyValuePair{ParameterExpression, CqtExpression}(whereLambda.Parameters[0], inputBinding)); /// /// // translate the expression in this context /// CqtExpression result = Translate(whereLambda.Expression); /// /// // pop the scope /// context.PopBindingScope(); ///
///_scopes; internal readonly ObjectContext ObjectContext; /// /// Initialize a new binding context /// internal BindingContext(ParameterExpression rootContextParameter, ObjectContext objectContext, CompiledQueryParameter [] compiledQueryParameters ) : this() { Debug.Assert(null != rootContextParameter && null != objectContext, "missing required args to BindingContext"); _rootContextBinding = new Binding(rootContextParameter, null); this.ObjectContext = objectContext; PushBindingScope(_rootContextBinding); foreach (var parameter in compiledQueryParameters) { Binding binding = new Binding(parameter.Expression, parameter.ParameterReference); PushBindingScope(binding); } } ////// Initialize a new binding context /// internal BindingContext() { _scopes = new List(); } /// /// Set up a new binding scope where parameter expressions map to their paired CQT expressions. /// /// Parameter/Expression bindings internal void PushBindingScope(params Binding[] bindings) { _scopes.Add(bindings); } ////// Removes a scope when leaving a particular sub-expression. /// ///Scope. internal void PopBindingScope() { _scopes.RemoveAt(_scopes.Count - 1); } internal bool TryGetBoundExpression(Expression linqExpression, out CqtExpression cqtExpression) { Binding binding; if (TryGetBinding(linqExpression, out binding)) { if (binding != _rootContextBinding) { cqtExpression = binding.CqtExpression; return true; } } cqtExpression = null; return false; } internal bool IsRootContextParameter(ParameterExpression parameter) { Binding binding; if (TryGetBinding(parameter, out binding)) { return _rootContextBinding == binding; } return false; } // Try to find a CQT expression that is the translation of a LINQ parameter. private bool TryGetBinding(Expression parameter, out Binding binding) { binding = null; // if there are no binding scopes, their can be no binding if (_scopes.Count == 0) { return false; } for (int i = _scopes.Count - 1; i >= 0; i--) { Binding[] scope = _scopes[i]; foreach (Binding scopeBinding in scope) { if (parameter == scopeBinding.LinqExpression) { binding = scopeBinding; return true; } } } // no match found return false; } } ////// Class describing a LINQ parameter and its bound expression. For instance, in /// /// products.Select(p => p.ID) /// /// the 'products' query is the bound expression, and 'p' is the parameter. /// internal sealed class Binding { internal Binding(Expression linqExpression, CqtExpression cqtExpression) { LinqExpression = linqExpression; CqtExpression = cqtExpression; } internal readonly Expression LinqExpression; internal readonly CqtExpression CqtExpression; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
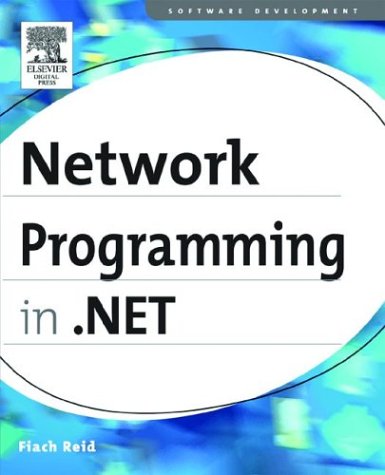
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MetadataException.cs
- DataReceivedEventArgs.cs
- ExpressionVisitor.cs
- CapabilitiesState.cs
- MutexSecurity.cs
- TraceRecord.cs
- MouseActionConverter.cs
- EditorZoneBase.cs
- AttachedPropertyBrowsableWhenAttributePresentAttribute.cs
- LinqDataSourceView.cs
- DataErrorValidationRule.cs
- CodeNamespaceImportCollection.cs
- AuthenticatingEventArgs.cs
- HexParser.cs
- Matrix.cs
- SqlInternalConnectionTds.cs
- DeflateStream.cs
- SubstitutionList.cs
- VisualStyleTypesAndProperties.cs
- RectangleGeometry.cs
- SqlNotificationEventArgs.cs
- WindowsImpersonationContext.cs
- SyntaxCheck.cs
- MetadataCollection.cs
- InternalRelationshipCollection.cs
- HandleCollector.cs
- SectionVisual.cs
- AccessKeyManager.cs
- RuntimeResourceSet.cs
- _SSPISessionCache.cs
- StubHelpers.cs
- DescendantBaseQuery.cs
- DtrList.cs
- FixedElement.cs
- RSAPKCS1KeyExchangeFormatter.cs
- SQLBytesStorage.cs
- HtmlTableRowCollection.cs
- PreviewKeyDownEventArgs.cs
- GridViewSelectEventArgs.cs
- IPAddressCollection.cs
- LinkedList.cs
- DesignTimeResourceProviderFactoryAttribute.cs
- ImplicitInputBrush.cs
- CodeSnippetExpression.cs
- DelegatingConfigHost.cs
- FontFamilyValueSerializer.cs
- AsymmetricAlgorithm.cs
- SuppressMergeCheckAttribute.cs
- DataControlFieldHeaderCell.cs
- ISAPIRuntime.cs
- XPathAxisIterator.cs
- SigningCredentials.cs
- PathSegment.cs
- MeshGeometry3D.cs
- relpropertyhelper.cs
- ExpressionBinding.cs
- RowBinding.cs
- XslAst.cs
- InputLanguageSource.cs
- CodeIdentifier.cs
- WhitespaceRuleLookup.cs
- PermissionRequestEvidence.cs
- RequestResizeEvent.cs
- ExecutedRoutedEventArgs.cs
- LambdaCompiler.Expressions.cs
- RemotingConfigParser.cs
- PEFileReader.cs
- IncrementalCompileAnalyzer.cs
- LogStore.cs
- WebEvents.cs
- OutputCacheProfileCollection.cs
- FontUnitConverter.cs
- XsltContext.cs
- SatelliteContractVersionAttribute.cs
- DataServiceEntityAttribute.cs
- WaitHandle.cs
- ClientData.cs
- FreezableOperations.cs
- MexNamedPipeBindingElement.cs
- EventPrivateKey.cs
- ContentElement.cs
- LinqDataView.cs
- DataRowExtensions.cs
- SqlConnectionString.cs
- MetabaseServerConfig.cs
- Activator.cs
- WebBrowserProgressChangedEventHandler.cs
- PieceNameHelper.cs
- XmlAttributeCollection.cs
- ServiceModelInstallComponent.cs
- PersonalizationProvider.cs
- FocusChangedEventArgs.cs
- Debug.cs
- BitmapDecoder.cs
- SchemaCollectionPreprocessor.cs
- WindowsComboBox.cs
- Screen.cs
- InfiniteTimeSpanConverter.cs
- TriggerCollection.cs
- CodeGen.cs