Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Core / CSharp / System / Windows / Media / Effects / BitmapEffectrendercontext.cs / 1 / BitmapEffectrendercontext.cs
//------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, 2005 // // File: BitmapEffectRenderContext.cs //----------------------------------------------------------------------------- using MS.Internal; using System; using System.IO; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Diagnostics; using System.Reflection; using System.Runtime.InteropServices; using System.Windows; using System.Windows.Media; using System.Windows.Markup; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Security; using MS.Internal.PresentationCore; namespace System.Windows.Media.Effects { ////// BitmapEffectRenderContext /// internal class BitmapEffectRenderContext { SafeMILHandle /*IMILBitmapEffectRenderContext*/ context; ////// BitmapEffectRenderContext /// public BitmapEffectRenderContext() { Create(); } ////// NativeContext /// internal SafeMILHandle NativeContextSafeHandle { get { return context; } } ////// Sets the output pixel format /// public PixelFormat OutputPixelFormat { #if never get { Guid guid; HRESULT.Check(MS.Win32.PresentationCore.UnsafeNativeMethods.IMILBitmapEffectRenderContext.GetOutputPixelFormat(NativeContextSafeHandle, out guid)); return PixelFormat.GetPixelFormat(guid); } #endif set { Guid guid = value.Guid; HRESULT.Check(MS.Win32.PresentationCore.UnsafeNativeMethods.IMILBitmapEffectRenderContext.SetOutputPixelFormat(NativeContextSafeHandle, ref guid)); } } #if never ////// /// public bool SoftwareRenderer { set { HRESULT.Check(MS.Win32.PresentationCore.UnsafeNativeMethods.IMILBitmapEffectRenderContext.SetUseSoftwareRenderer(context, value)); } } #endif ////// Gets or sets the transform for the effects pipeline /// public Matrix Transform { get { double m11, m12, m21, m22, m31, m32; HRESULT.Check(MS.Win32.PresentationCore.UnsafeNativeMethods.IMILBitmapEffectRenderContext.GetFinalTransform(NativeContextSafeHandle, out m11, out m12, out m21, out m22, out m31, out m32)); return new Matrix(m11, m12, m21, m22, m31, m32); } set { HRESULT.Check(MS.Win32.PresentationCore.UnsafeNativeMethods.IMILBitmapEffectRenderContext.SetInitialTransform(NativeContextSafeHandle, value.M11, value.M12, value.M21, value.M22, value.OffsetX, value.OffsetY)); } } ////// Sets the output DPI /// public Point OutputDPI { #if never get { double dpiX, dpiY; HRESULT.Check(MS.Win32.PresentationCore.UnsafeNativeMethods.IMILBitmapEffectRenderContext.GetOutputDPI(NativeContextSafeHandle, out dpiX, out dpiY)); return new Point(dpiX, dpiY); } #endif set { HRESULT.Check(MS.Win32.PresentationCore.UnsafeNativeMethods.IMILBitmapEffectRenderContext.SetOutputDPI(NativeContextSafeHandle, value.X, value.Y)); } } ////// Sets the region of interest /// public Rect RenderRect { set { HRESULT.Check(MS.Win32.PresentationCore.UnsafeNativeMethods.IMILBitmapEffectRenderContext.SetRegionOfInterest(context, ref value)); } } ////// Create context /// ////// Critical - calls critical code method FactoryMaker.FactoryMaker /// TreatAsSafe - as there is a demand /// [SecurityCritical, SecurityTreatAsSafe] internal void Create() { SecurityHelper.DemandUIWindowPermission(); using (FactoryMaker myFactory = new FactoryMaker()) { HRESULT.Check(MS.Win32.PresentationCore.UnsafeNativeMethods.MILFactoryEffects.CreateBitmapEffectContext(myFactory.FactoryPtr, out context)); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, 2005 // // File: BitmapEffectRenderContext.cs //----------------------------------------------------------------------------- using MS.Internal; using System; using System.IO; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Diagnostics; using System.Reflection; using System.Runtime.InteropServices; using System.Windows; using System.Windows.Media; using System.Windows.Markup; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Security; using MS.Internal.PresentationCore; namespace System.Windows.Media.Effects { ////// BitmapEffectRenderContext /// internal class BitmapEffectRenderContext { SafeMILHandle /*IMILBitmapEffectRenderContext*/ context; ////// BitmapEffectRenderContext /// public BitmapEffectRenderContext() { Create(); } ////// NativeContext /// internal SafeMILHandle NativeContextSafeHandle { get { return context; } } ////// Sets the output pixel format /// public PixelFormat OutputPixelFormat { #if never get { Guid guid; HRESULT.Check(MS.Win32.PresentationCore.UnsafeNativeMethods.IMILBitmapEffectRenderContext.GetOutputPixelFormat(NativeContextSafeHandle, out guid)); return PixelFormat.GetPixelFormat(guid); } #endif set { Guid guid = value.Guid; HRESULT.Check(MS.Win32.PresentationCore.UnsafeNativeMethods.IMILBitmapEffectRenderContext.SetOutputPixelFormat(NativeContextSafeHandle, ref guid)); } } #if never ////// /// public bool SoftwareRenderer { set { HRESULT.Check(MS.Win32.PresentationCore.UnsafeNativeMethods.IMILBitmapEffectRenderContext.SetUseSoftwareRenderer(context, value)); } } #endif ////// Gets or sets the transform for the effects pipeline /// public Matrix Transform { get { double m11, m12, m21, m22, m31, m32; HRESULT.Check(MS.Win32.PresentationCore.UnsafeNativeMethods.IMILBitmapEffectRenderContext.GetFinalTransform(NativeContextSafeHandle, out m11, out m12, out m21, out m22, out m31, out m32)); return new Matrix(m11, m12, m21, m22, m31, m32); } set { HRESULT.Check(MS.Win32.PresentationCore.UnsafeNativeMethods.IMILBitmapEffectRenderContext.SetInitialTransform(NativeContextSafeHandle, value.M11, value.M12, value.M21, value.M22, value.OffsetX, value.OffsetY)); } } ////// Sets the output DPI /// public Point OutputDPI { #if never get { double dpiX, dpiY; HRESULT.Check(MS.Win32.PresentationCore.UnsafeNativeMethods.IMILBitmapEffectRenderContext.GetOutputDPI(NativeContextSafeHandle, out dpiX, out dpiY)); return new Point(dpiX, dpiY); } #endif set { HRESULT.Check(MS.Win32.PresentationCore.UnsafeNativeMethods.IMILBitmapEffectRenderContext.SetOutputDPI(NativeContextSafeHandle, value.X, value.Y)); } } ////// Sets the region of interest /// public Rect RenderRect { set { HRESULT.Check(MS.Win32.PresentationCore.UnsafeNativeMethods.IMILBitmapEffectRenderContext.SetRegionOfInterest(context, ref value)); } } ////// Create context /// ////// Critical - calls critical code method FactoryMaker.FactoryMaker /// TreatAsSafe - as there is a demand /// [SecurityCritical, SecurityTreatAsSafe] internal void Create() { SecurityHelper.DemandUIWindowPermission(); using (FactoryMaker myFactory = new FactoryMaker()) { HRESULT.Check(MS.Win32.PresentationCore.UnsafeNativeMethods.MILFactoryEffects.CreateBitmapEffectContext(myFactory.FactoryPtr, out context)); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
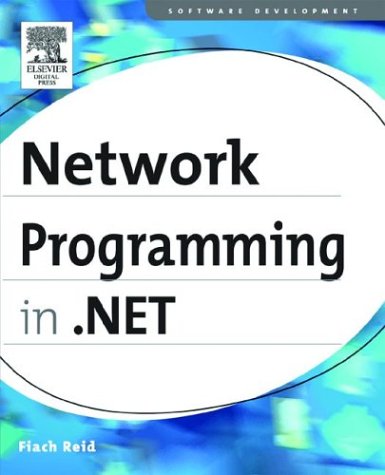
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- AbstractDataSvcMapFileLoader.cs
- EnvelopedSignatureTransform.cs
- WebServicesDescriptionAttribute.cs
- RuntimeCompatibilityAttribute.cs
- DetailsViewDeletedEventArgs.cs
- MSAAEventDispatcher.cs
- X509CertificateEndpointIdentity.cs
- MethodImplAttribute.cs
- METAHEADER.cs
- SuspendDesigner.cs
- DataColumnChangeEvent.cs
- ResourceDisplayNameAttribute.cs
- HtmlTableCellCollection.cs
- HtmlWindowCollection.cs
- ToolStripActionList.cs
- BatchParser.cs
- Base64Encoder.cs
- FlowNode.cs
- StylusPoint.cs
- SoapSchemaExporter.cs
- DataGridViewRow.cs
- ValueTable.cs
- BreakRecordTable.cs
- GridViewAutoFormat.cs
- UiaCoreApi.cs
- OrderPreservingPipeliningMergeHelper.cs
- Tablet.cs
- ParallelTimeline.cs
- XdrBuilder.cs
- HuffModule.cs
- TraceUtils.cs
- SchemaElementDecl.cs
- ApplicationSecurityManager.cs
- IgnoreSectionHandler.cs
- PathParser.cs
- PropertySegmentSerializer.cs
- ValidationError.cs
- ListViewGroupCollectionEditor.cs
- GradientBrush.cs
- MessageParameterAttribute.cs
- StateItem.cs
- WebServiceHandlerFactory.cs
- WindowsListViewScroll.cs
- MulticastNotSupportedException.cs
- VisualTarget.cs
- VisualTreeUtils.cs
- SizeIndependentAnimationStorage.cs
- PartitionedStreamMerger.cs
- TreeIterator.cs
- UnicodeEncoding.cs
- TypeConverters.cs
- ContentOperations.cs
- PageAsyncTask.cs
- AsyncStreamReader.cs
- Brush.cs
- MenuItem.cs
- IndexedString.cs
- BaseDataList.cs
- ToolTip.cs
- XPathAxisIterator.cs
- EdmType.cs
- SelectionHighlightInfo.cs
- XmlDataDocument.cs
- WebPartExportVerb.cs
- ZipIOLocalFileHeader.cs
- AuthorizationRuleCollection.cs
- SimpleType.cs
- SecurityContext.cs
- Soap.cs
- CapabilitiesRule.cs
- Size.cs
- DllHostInitializer.cs
- VectorAnimation.cs
- SqlCacheDependencyDatabaseCollection.cs
- MeshGeometry3D.cs
- XmlEventCache.cs
- WhereQueryOperator.cs
- ObjectDataSource.cs
- XmlAttributeOverrides.cs
- ObjectStateEntryDbDataRecord.cs
- ImageDrawing.cs
- CodeIndexerExpression.cs
- SqlProvider.cs
- SqlRecordBuffer.cs
- SystemIPInterfaceStatistics.cs
- TypeBuilderInstantiation.cs
- HttpApplicationFactory.cs
- EpmTargetTree.cs
- WorkflowInlining.cs
- EntityClassGenerator.cs
- DebugControllerThread.cs
- SQLInt16.cs
- HttpRequestCacheValidator.cs
- BitmapScalingModeValidation.cs
- TargetConverter.cs
- Parser.cs
- WebPartMenu.cs
- OracleConnectionFactory.cs
- LineVisual.cs
- StylusPointProperty.cs