Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Core / CSharp / System / Windows / Media / Generated / SolidColorBrush.cs / 1 / SolidColorBrush.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.KnownBoxes; using MS.Internal.Collections; using MS.Internal.PresentationCore; using MS.Utility; using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Runtime.InteropServices; using System.ComponentModel.Design.Serialization; using System.Text; using System.Windows; using System.Windows.Media; using System.Windows.Media.Effects; using System.Windows.Media.Media3D; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Windows.Media.Imaging; using System.Windows.Markup; using System.Windows.Media.Converters; using System.Security; using System.Security.Permissions; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; // These types are aliased to match the unamanaged names used in interop using BOOL = System.UInt32; using WORD = System.UInt16; using Float = System.Single; namespace System.Windows.Media { sealed partial class SolidColorBrush : Brush { //----------------------------------------------------- // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Shadows inherited Clone() with a strongly typed /// version for convenience. /// public new SolidColorBrush Clone() { return (SolidColorBrush)base.Clone(); } ////// Shadows inherited CloneCurrentValue() with a strongly typed /// version for convenience. /// public new SolidColorBrush CloneCurrentValue() { return (SolidColorBrush)base.CloneCurrentValue(); } #endregion Public Methods //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- private static void ColorPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { SolidColorBrush target = ((SolidColorBrush) d); target.PropertyChanged(ColorProperty); } #region Public Properties ////// Color - Color. Default value is Colors.Transparent. /// public Color Color { get { return (Color) GetValue(ColorProperty); } set { SetValueInternal(ColorProperty, value); } } #endregion Public Properties //------------------------------------------------------ // // Protected Methods // //------------------------------------------------------ #region Protected Methods ////// Implementation of ///Freezable.CreateInstanceCore . ///The new Freezable. protected override Freezable CreateInstanceCore() { return new SolidColorBrush(); } #endregion ProtectedMethods //----------------------------------------------------- // // Internal Methods // //------------------------------------------------------ #region Internal Methods ////// Critical: This code calls into an unsafe code block /// TreatAsSafe: This code does not return any critical data.It is ok to expose /// Channels are safe to call into and do not go cross domain and cross process /// [SecurityCritical,SecurityTreatAsSafe] internal override void UpdateResource(DUCE.Channel channel, bool skipOnChannelCheck) { // If we're told we can skip the channel check, then we must be on channel Debug.Assert(!skipOnChannelCheck || _duceResource.IsOnChannel(channel)); if (skipOnChannelCheck || _duceResource.IsOnChannel(channel)) { base.UpdateResource(channel, skipOnChannelCheck); // Read values of properties into local variables Transform vTransform = Transform; Transform vRelativeTransform = RelativeTransform; // Obtain handles for properties that implement DUCE.IResource DUCE.ResourceHandle hTransform; if (vTransform == null || Object.ReferenceEquals(vTransform, Transform.Identity) ) { hTransform = DUCE.ResourceHandle.Null; } else { hTransform = ((DUCE.IResource)vTransform).GetHandle(channel); } DUCE.ResourceHandle hRelativeTransform; if (vRelativeTransform == null || Object.ReferenceEquals(vRelativeTransform, Transform.Identity) ) { hRelativeTransform = DUCE.ResourceHandle.Null; } else { hRelativeTransform = ((DUCE.IResource)vRelativeTransform).GetHandle(channel); } // Obtain handles for animated properties DUCE.ResourceHandle hOpacityAnimations = GetAnimationResourceHandle(OpacityProperty, channel); DUCE.ResourceHandle hColorAnimations = GetAnimationResourceHandle(ColorProperty, channel); // Pack & send command packet DUCE.MILCMD_SOLIDCOLORBRUSH data; unsafe { data.Type = MILCMD.MilCmdSolidColorBrush; data.Handle = _duceResource.GetHandle(channel); if (hOpacityAnimations.IsNull) { data.Opacity = Opacity; } data.hOpacityAnimations = hOpacityAnimations; data.hTransform = hTransform; data.hRelativeTransform = hRelativeTransform; if (hColorAnimations.IsNull) { data.Color = CompositionResourceManager.ColorToMilColorF(Color); } data.hColorAnimations = hColorAnimations; // Send packed command structure channel.SendCommand( (byte*)&data, sizeof(DUCE.MILCMD_SOLIDCOLORBRUSH)); } } } internal override DUCE.ResourceHandle AddRefOnChannelCore(DUCE.Channel channel) { if (_duceResource.CreateOrAddRefOnChannel(channel, System.Windows.Media.Composition.DUCE.ResourceType.TYPE_SOLIDCOLORBRUSH)) { Transform vTransform = Transform; if (vTransform != null) ((DUCE.IResource)vTransform).AddRefOnChannel(channel); Transform vRelativeTransform = RelativeTransform; if (vRelativeTransform != null) ((DUCE.IResource)vRelativeTransform).AddRefOnChannel(channel); AddRefOnChannelAnimations(channel); UpdateResource(channel, true /* skip "on channel" check - we already know that we're on channel */ ); } return _duceResource.GetHandle(channel); } internal override void ReleaseOnChannelCore(DUCE.Channel channel) { Debug.Assert(_duceResource.IsOnChannel(channel)); if (_duceResource.ReleaseOnChannel(channel)) { Transform vTransform = Transform; if (vTransform != null) ((DUCE.IResource)vTransform).ReleaseOnChannel(channel); Transform vRelativeTransform = RelativeTransform; if (vRelativeTransform != null) ((DUCE.IResource)vRelativeTransform).ReleaseOnChannel(channel); ReleaseOnChannelAnimations(channel); } } internal override DUCE.ResourceHandle GetHandleCore(DUCE.Channel channel) { // Note that we are in a lock here already. return _duceResource.GetHandle(channel); } internal override int GetChannelCountCore() { // must already be in composition lock here return _duceResource.GetChannelCount(); } internal override DUCE.Channel GetChannelCore(int index) { // Note that we are in a lock here already. return _duceResource.GetChannel(index); } #endregion Internal Methods //----------------------------------------------------- // // Internal Properties // //----------------------------------------------------- #region Internal Properties // // This property finds the correct initial size for the _effectiveValues store on the // current DependencyObject as a performance optimization // // This includes: // Color // internal override int EffectiveValuesInitialSize { get { return 1; } } #endregion Internal Properties //----------------------------------------------------- // // Dependency Properties // //------------------------------------------------------ #region Dependency Properties ////// The DependencyProperty for the SolidColorBrush.Color property. /// public static readonly DependencyProperty ColorProperty; #endregion Dependency Properties //----------------------------------------------------- // // Internal Fields // //------------------------------------------------------ #region Internal Fields internal System.Windows.Media.Composition.DUCE.MultiChannelResource _duceResource = new System.Windows.Media.Composition.DUCE.MultiChannelResource(); internal static Color s_Color = Colors.Transparent; #endregion Internal Fields #region Constructors //------------------------------------------------------ // // Constructors // //----------------------------------------------------- static SolidColorBrush() { // We check our static default fields which are of type Freezable // to make sure that they are not mutable, otherwise we will throw // if these get touched by more than one thread in the lifetime // of your app. (Windows OS Bug #947272) // // Initializations Type typeofThis = typeof(SolidColorBrush); ColorProperty = RegisterProperty("Color", typeof(Color), typeofThis, Colors.Transparent, new PropertyChangedCallback(ColorPropertyChanged), null, /* isIndependentlyAnimated = */ true, /* coerceValueCallback */ null); } #endregion Constructors } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.KnownBoxes; using MS.Internal.Collections; using MS.Internal.PresentationCore; using MS.Utility; using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Runtime.InteropServices; using System.ComponentModel.Design.Serialization; using System.Text; using System.Windows; using System.Windows.Media; using System.Windows.Media.Effects; using System.Windows.Media.Media3D; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Windows.Media.Imaging; using System.Windows.Markup; using System.Windows.Media.Converters; using System.Security; using System.Security.Permissions; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; // These types are aliased to match the unamanaged names used in interop using BOOL = System.UInt32; using WORD = System.UInt16; using Float = System.Single; namespace System.Windows.Media { sealed partial class SolidColorBrush : Brush { //----------------------------------------------------- // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Shadows inherited Clone() with a strongly typed /// version for convenience. /// public new SolidColorBrush Clone() { return (SolidColorBrush)base.Clone(); } ////// Shadows inherited CloneCurrentValue() with a strongly typed /// version for convenience. /// public new SolidColorBrush CloneCurrentValue() { return (SolidColorBrush)base.CloneCurrentValue(); } #endregion Public Methods //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- private static void ColorPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { SolidColorBrush target = ((SolidColorBrush) d); target.PropertyChanged(ColorProperty); } #region Public Properties ////// Color - Color. Default value is Colors.Transparent. /// public Color Color { get { return (Color) GetValue(ColorProperty); } set { SetValueInternal(ColorProperty, value); } } #endregion Public Properties //------------------------------------------------------ // // Protected Methods // //------------------------------------------------------ #region Protected Methods ////// Implementation of ///Freezable.CreateInstanceCore . ///The new Freezable. protected override Freezable CreateInstanceCore() { return new SolidColorBrush(); } #endregion ProtectedMethods //----------------------------------------------------- // // Internal Methods // //------------------------------------------------------ #region Internal Methods ////// Critical: This code calls into an unsafe code block /// TreatAsSafe: This code does not return any critical data.It is ok to expose /// Channels are safe to call into and do not go cross domain and cross process /// [SecurityCritical,SecurityTreatAsSafe] internal override void UpdateResource(DUCE.Channel channel, bool skipOnChannelCheck) { // If we're told we can skip the channel check, then we must be on channel Debug.Assert(!skipOnChannelCheck || _duceResource.IsOnChannel(channel)); if (skipOnChannelCheck || _duceResource.IsOnChannel(channel)) { base.UpdateResource(channel, skipOnChannelCheck); // Read values of properties into local variables Transform vTransform = Transform; Transform vRelativeTransform = RelativeTransform; // Obtain handles for properties that implement DUCE.IResource DUCE.ResourceHandle hTransform; if (vTransform == null || Object.ReferenceEquals(vTransform, Transform.Identity) ) { hTransform = DUCE.ResourceHandle.Null; } else { hTransform = ((DUCE.IResource)vTransform).GetHandle(channel); } DUCE.ResourceHandle hRelativeTransform; if (vRelativeTransform == null || Object.ReferenceEquals(vRelativeTransform, Transform.Identity) ) { hRelativeTransform = DUCE.ResourceHandle.Null; } else { hRelativeTransform = ((DUCE.IResource)vRelativeTransform).GetHandle(channel); } // Obtain handles for animated properties DUCE.ResourceHandle hOpacityAnimations = GetAnimationResourceHandle(OpacityProperty, channel); DUCE.ResourceHandle hColorAnimations = GetAnimationResourceHandle(ColorProperty, channel); // Pack & send command packet DUCE.MILCMD_SOLIDCOLORBRUSH data; unsafe { data.Type = MILCMD.MilCmdSolidColorBrush; data.Handle = _duceResource.GetHandle(channel); if (hOpacityAnimations.IsNull) { data.Opacity = Opacity; } data.hOpacityAnimations = hOpacityAnimations; data.hTransform = hTransform; data.hRelativeTransform = hRelativeTransform; if (hColorAnimations.IsNull) { data.Color = CompositionResourceManager.ColorToMilColorF(Color); } data.hColorAnimations = hColorAnimations; // Send packed command structure channel.SendCommand( (byte*)&data, sizeof(DUCE.MILCMD_SOLIDCOLORBRUSH)); } } } internal override DUCE.ResourceHandle AddRefOnChannelCore(DUCE.Channel channel) { if (_duceResource.CreateOrAddRefOnChannel(channel, System.Windows.Media.Composition.DUCE.ResourceType.TYPE_SOLIDCOLORBRUSH)) { Transform vTransform = Transform; if (vTransform != null) ((DUCE.IResource)vTransform).AddRefOnChannel(channel); Transform vRelativeTransform = RelativeTransform; if (vRelativeTransform != null) ((DUCE.IResource)vRelativeTransform).AddRefOnChannel(channel); AddRefOnChannelAnimations(channel); UpdateResource(channel, true /* skip "on channel" check - we already know that we're on channel */ ); } return _duceResource.GetHandle(channel); } internal override void ReleaseOnChannelCore(DUCE.Channel channel) { Debug.Assert(_duceResource.IsOnChannel(channel)); if (_duceResource.ReleaseOnChannel(channel)) { Transform vTransform = Transform; if (vTransform != null) ((DUCE.IResource)vTransform).ReleaseOnChannel(channel); Transform vRelativeTransform = RelativeTransform; if (vRelativeTransform != null) ((DUCE.IResource)vRelativeTransform).ReleaseOnChannel(channel); ReleaseOnChannelAnimations(channel); } } internal override DUCE.ResourceHandle GetHandleCore(DUCE.Channel channel) { // Note that we are in a lock here already. return _duceResource.GetHandle(channel); } internal override int GetChannelCountCore() { // must already be in composition lock here return _duceResource.GetChannelCount(); } internal override DUCE.Channel GetChannelCore(int index) { // Note that we are in a lock here already. return _duceResource.GetChannel(index); } #endregion Internal Methods //----------------------------------------------------- // // Internal Properties // //----------------------------------------------------- #region Internal Properties // // This property finds the correct initial size for the _effectiveValues store on the // current DependencyObject as a performance optimization // // This includes: // Color // internal override int EffectiveValuesInitialSize { get { return 1; } } #endregion Internal Properties //----------------------------------------------------- // // Dependency Properties // //------------------------------------------------------ #region Dependency Properties ////// The DependencyProperty for the SolidColorBrush.Color property. /// public static readonly DependencyProperty ColorProperty; #endregion Dependency Properties //----------------------------------------------------- // // Internal Fields // //------------------------------------------------------ #region Internal Fields internal System.Windows.Media.Composition.DUCE.MultiChannelResource _duceResource = new System.Windows.Media.Composition.DUCE.MultiChannelResource(); internal static Color s_Color = Colors.Transparent; #endregion Internal Fields #region Constructors //------------------------------------------------------ // // Constructors // //----------------------------------------------------- static SolidColorBrush() { // We check our static default fields which are of type Freezable // to make sure that they are not mutable, otherwise we will throw // if these get touched by more than one thread in the lifetime // of your app. (Windows OS Bug #947272) // // Initializations Type typeofThis = typeof(SolidColorBrush); ColorProperty = RegisterProperty("Color", typeof(Color), typeofThis, Colors.Transparent, new PropertyChangedCallback(ColorPropertyChanged), null, /* isIndependentlyAnimated = */ true, /* coerceValueCallback */ null); } #endregion Constructors } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
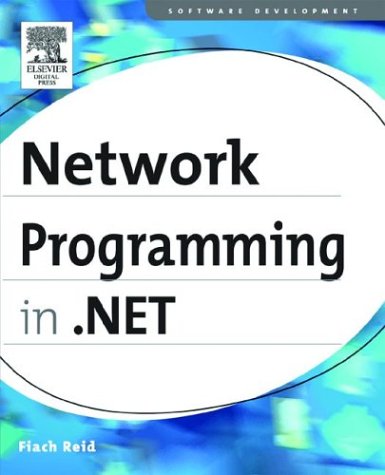
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- GenericTextProperties.cs
- TextParagraphView.cs
- Storyboard.cs
- TypeToken.cs
- DbConnectionPoolCounters.cs
- ObservableCollectionDefaultValueFactory.cs
- TextBox.cs
- EntitySetDataBindingList.cs
- TableLayoutPanelCellPosition.cs
- PermissionSetTriple.cs
- behaviorssection.cs
- VisualBasicImportReference.cs
- ApplicationHost.cs
- AttachInfo.cs
- arabicshape.cs
- Regex.cs
- DataSourceControlBuilder.cs
- QilStrConcat.cs
- EntityDataReader.cs
- Clock.cs
- DataGridViewButtonCell.cs
- WCFBuildProvider.cs
- DelegatingTypeDescriptionProvider.cs
- VirtualPathProvider.cs
- RichTextBoxDesigner.cs
- XmlILAnnotation.cs
- SortDescription.cs
- OdbcConnectionOpen.cs
- NonClientArea.cs
- _HeaderInfoTable.cs
- RunClient.cs
- WeakHashtable.cs
- MultiView.cs
- ObjectToken.cs
- PeerNameResolver.cs
- SqlException.cs
- TextSelectionHelper.cs
- AsyncOperation.cs
- EncryptRequest.cs
- Brush.cs
- SplayTreeNode.cs
- SettingsBase.cs
- NodeLabelEditEvent.cs
- DependencyPropertyKind.cs
- itemelement.cs
- CustomTypeDescriptor.cs
- PeerPresenceInfo.cs
- ResourceSet.cs
- CustomActivityDesigner.cs
- RtfControlWordInfo.cs
- RbTree.cs
- IPCCacheManager.cs
- WebHttpDispatchOperationSelector.cs
- ContextDataSourceContextData.cs
- SynchronizationContextHelper.cs
- SortExpressionBuilder.cs
- FusionWrap.cs
- GeneratedView.cs
- DataRowComparer.cs
- XmlUtf8RawTextWriter.cs
- FactoryGenerator.cs
- TrackPoint.cs
- DataViewListener.cs
- EventDescriptorCollection.cs
- LogSwitch.cs
- DataGridCaption.cs
- hresults.cs
- ApplicationSecurityManager.cs
- ToolStripSettings.cs
- RelationshipFixer.cs
- ProcessHostServerConfig.cs
- CreateDataSourceDialog.cs
- XmlWriter.cs
- HttpChannelBindingToken.cs
- TcpClientChannel.cs
- FontStretch.cs
- ButtonColumn.cs
- XPathItem.cs
- CmsInterop.cs
- IssuedTokenParametersElement.cs
- EventPropertyMap.cs
- AttributedMetaModel.cs
- DropDownButton.cs
- LogAppendAsyncResult.cs
- DependencyPropertyValueSerializer.cs
- ConfigXmlCDataSection.cs
- StyleCollectionEditor.cs
- XmlSerializerAssemblyAttribute.cs
- ListItemParagraph.cs
- BasicCellRelation.cs
- RawStylusInputCustomDataList.cs
- FixedHighlight.cs
- InvalidComObjectException.cs
- ReferentialConstraint.cs
- PathGeometry.cs
- EventToken.cs
- Point3DAnimationBase.cs
- SoapAttributes.cs
- SqlRowUpdatingEvent.cs
- TableRowCollection.cs