Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / clr / src / BCL / System / __Filters.cs / 1 / __Filters.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // __Filters.cs // // This class defines the delegate methods for the COM+ implemented filters. // // // namespace System { using System; using System.Reflection; using System.Globalization; [Serializable()] internal class __Filters { // Filters... // The following are the built in filters defined for this class. These // should really be defined as static methods. They are used in as delegates // which currently doesn't support static methods. We will change this // once the compiler supports delegates. // FilterAttribute // This method will search for a member based upon the attribute passed in. // filterCriteria -- an Int32 representing the attribute internal virtual bool FilterAttribute(MemberInfo m,Object filterCriteria) { // Check that the criteria object is an Integer object if (filterCriteria == null) throw new InvalidFilterCriteriaException(Environment.GetResourceString("RFLCT.FltCritInt")); switch (m.MemberType) { case MemberTypes.Constructor: case MemberTypes.Method: { MethodAttributes criteria = 0; try { int i = (int) filterCriteria; criteria = (MethodAttributes) i; } catch { throw new InvalidFilterCriteriaException(Environment.GetResourceString("RFLCT.FltCritInt")); } MethodAttributes attr; if (m.MemberType == MemberTypes.Method) attr = ((MethodInfo) m).Attributes; else attr = ((ConstructorInfo) m).Attributes; if (((criteria & MethodAttributes.MemberAccessMask) != 0) && (attr & MethodAttributes.MemberAccessMask) != (criteria & MethodAttributes.MemberAccessMask)) return false; if (((criteria & MethodAttributes.Static) != 0) && (attr & MethodAttributes.Static) == 0) return false; if (((criteria & MethodAttributes.Final) != 0) && (attr & MethodAttributes.Final) == 0) return false; if (((criteria & MethodAttributes.Virtual) != 0) && (attr & MethodAttributes.Virtual) == 0) return false; if (((criteria & MethodAttributes.Abstract) != 0) && (attr & MethodAttributes.Abstract) == 0) return false; if (((criteria & MethodAttributes.SpecialName) != 0) && (attr & MethodAttributes.SpecialName) == 0) return false; return true; } case MemberTypes.Field: { FieldAttributes criteria = 0; try { int i = (int) filterCriteria; criteria = (FieldAttributes) i; } catch { throw new InvalidFilterCriteriaException(Environment.GetResourceString("RFLCT.FltCritInt")); } FieldAttributes attr = ((FieldInfo) m).Attributes; if (((criteria & FieldAttributes.FieldAccessMask) != 0) && (attr & FieldAttributes.FieldAccessMask) != (criteria & FieldAttributes.FieldAccessMask)) return false; if (((criteria & FieldAttributes.Static) != 0) && (attr & FieldAttributes.Static) == 0) return false; if (((criteria & FieldAttributes.InitOnly) != 0) && (attr & FieldAttributes.InitOnly) == 0) return false; if (((criteria & FieldAttributes.Literal) != 0) && (attr & FieldAttributes.Literal) == 0) return false; if (((criteria & FieldAttributes.NotSerialized) != 0) && (attr & FieldAttributes.NotSerialized) == 0) return false; if (((criteria & FieldAttributes.PinvokeImpl) != 0) && (attr & FieldAttributes.PinvokeImpl) == 0) return false; return true; } } return false; } // FilterName // This method will filter based upon the name. A partial wildcard // at the end of the string is supported. // filterCriteria -- This is the string name internal virtual bool FilterName(MemberInfo m,Object filterCriteria) { // Check that the criteria object is a String object if(filterCriteria == null || !(filterCriteria is String)) throw new InvalidFilterCriteriaException(Environment.GetResourceString("RFLCT.FltCritString")); // At the moment this fails if its done on a single line.... String str = ((String) filterCriteria); str = str.Trim(); String name = m.Name; // Get the nested class name only, as opposed to the mangled one if (m.MemberType == MemberTypes.NestedType) name = name.Substring(name.LastIndexOf('+') + 1); // Check to see if this is a prefix or exact match requirement if (str.Length > 0 && str[str.Length - 1] == '*') { str = str.Substring(0, str.Length - 1); return (name.StartsWith(str, StringComparison.Ordinal)); } return (name.Equals(str)); } // FilterIgnoreCase // This delegate will do a name search but does it with the // ignore case specified. internal virtual bool FilterIgnoreCase(MemberInfo m,Object filterCriteria) { // Check that the criteria object is a String object if(filterCriteria == null || !(filterCriteria is String)) throw new InvalidFilterCriteriaException(Environment.GetResourceString("RFLCT.FltCritString")); String str = (String) filterCriteria; str = str.Trim(); String name = m.Name; // Get the nested class name only, as opposed to the mangled one if (m.MemberType == MemberTypes.NestedType) name = name.Substring(name.LastIndexOf('+') + 1); // Check to see if this is a prefix or exact match requirement if (str.Length > 0 && str[str.Length - 1] == '*') { str = str.Substring(0, str.Length - 1); return (String.Compare(name,0,str,0,str.Length,StringComparison.OrdinalIgnoreCase)==0); } return (String.Compare(str,name, StringComparison.OrdinalIgnoreCase) == 0); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // __Filters.cs // // This class defines the delegate methods for the COM+ implemented filters. // // // namespace System { using System; using System.Reflection; using System.Globalization; [Serializable()] internal class __Filters { // Filters... // The following are the built in filters defined for this class. These // should really be defined as static methods. They are used in as delegates // which currently doesn't support static methods. We will change this // once the compiler supports delegates. // FilterAttribute // This method will search for a member based upon the attribute passed in. // filterCriteria -- an Int32 representing the attribute internal virtual bool FilterAttribute(MemberInfo m,Object filterCriteria) { // Check that the criteria object is an Integer object if (filterCriteria == null) throw new InvalidFilterCriteriaException(Environment.GetResourceString("RFLCT.FltCritInt")); switch (m.MemberType) { case MemberTypes.Constructor: case MemberTypes.Method: { MethodAttributes criteria = 0; try { int i = (int) filterCriteria; criteria = (MethodAttributes) i; } catch { throw new InvalidFilterCriteriaException(Environment.GetResourceString("RFLCT.FltCritInt")); } MethodAttributes attr; if (m.MemberType == MemberTypes.Method) attr = ((MethodInfo) m).Attributes; else attr = ((ConstructorInfo) m).Attributes; if (((criteria & MethodAttributes.MemberAccessMask) != 0) && (attr & MethodAttributes.MemberAccessMask) != (criteria & MethodAttributes.MemberAccessMask)) return false; if (((criteria & MethodAttributes.Static) != 0) && (attr & MethodAttributes.Static) == 0) return false; if (((criteria & MethodAttributes.Final) != 0) && (attr & MethodAttributes.Final) == 0) return false; if (((criteria & MethodAttributes.Virtual) != 0) && (attr & MethodAttributes.Virtual) == 0) return false; if (((criteria & MethodAttributes.Abstract) != 0) && (attr & MethodAttributes.Abstract) == 0) return false; if (((criteria & MethodAttributes.SpecialName) != 0) && (attr & MethodAttributes.SpecialName) == 0) return false; return true; } case MemberTypes.Field: { FieldAttributes criteria = 0; try { int i = (int) filterCriteria; criteria = (FieldAttributes) i; } catch { throw new InvalidFilterCriteriaException(Environment.GetResourceString("RFLCT.FltCritInt")); } FieldAttributes attr = ((FieldInfo) m).Attributes; if (((criteria & FieldAttributes.FieldAccessMask) != 0) && (attr & FieldAttributes.FieldAccessMask) != (criteria & FieldAttributes.FieldAccessMask)) return false; if (((criteria & FieldAttributes.Static) != 0) && (attr & FieldAttributes.Static) == 0) return false; if (((criteria & FieldAttributes.InitOnly) != 0) && (attr & FieldAttributes.InitOnly) == 0) return false; if (((criteria & FieldAttributes.Literal) != 0) && (attr & FieldAttributes.Literal) == 0) return false; if (((criteria & FieldAttributes.NotSerialized) != 0) && (attr & FieldAttributes.NotSerialized) == 0) return false; if (((criteria & FieldAttributes.PinvokeImpl) != 0) && (attr & FieldAttributes.PinvokeImpl) == 0) return false; return true; } } return false; } // FilterName // This method will filter based upon the name. A partial wildcard // at the end of the string is supported. // filterCriteria -- This is the string name internal virtual bool FilterName(MemberInfo m,Object filterCriteria) { // Check that the criteria object is a String object if(filterCriteria == null || !(filterCriteria is String)) throw new InvalidFilterCriteriaException(Environment.GetResourceString("RFLCT.FltCritString")); // At the moment this fails if its done on a single line.... String str = ((String) filterCriteria); str = str.Trim(); String name = m.Name; // Get the nested class name only, as opposed to the mangled one if (m.MemberType == MemberTypes.NestedType) name = name.Substring(name.LastIndexOf('+') + 1); // Check to see if this is a prefix or exact match requirement if (str.Length > 0 && str[str.Length - 1] == '*') { str = str.Substring(0, str.Length - 1); return (name.StartsWith(str, StringComparison.Ordinal)); } return (name.Equals(str)); } // FilterIgnoreCase // This delegate will do a name search but does it with the // ignore case specified. internal virtual bool FilterIgnoreCase(MemberInfo m,Object filterCriteria) { // Check that the criteria object is a String object if(filterCriteria == null || !(filterCriteria is String)) throw new InvalidFilterCriteriaException(Environment.GetResourceString("RFLCT.FltCritString")); String str = (String) filterCriteria; str = str.Trim(); String name = m.Name; // Get the nested class name only, as opposed to the mangled one if (m.MemberType == MemberTypes.NestedType) name = name.Substring(name.LastIndexOf('+') + 1); // Check to see if this is a prefix or exact match requirement if (str.Length > 0 && str[str.Length - 1] == '*') { str = str.Substring(0, str.Length - 1); return (String.Compare(name,0,str,0,str.Length,StringComparison.OrdinalIgnoreCase)==0); } return (String.Compare(str,name, StringComparison.OrdinalIgnoreCase) == 0); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
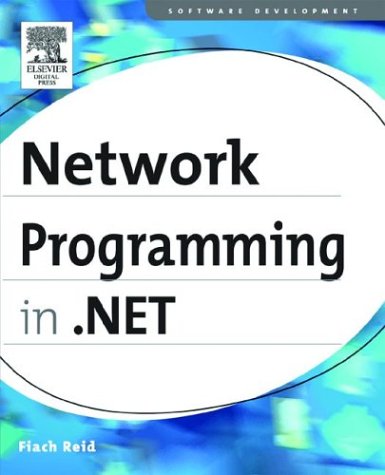
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataGridViewMethods.cs
- ClockController.cs
- AliasedSlot.cs
- LazyTextWriterCreator.cs
- InkPresenter.cs
- PackageDigitalSignature.cs
- BezierSegment.cs
- DrawingAttributesDefaultValueFactory.cs
- SpanIndex.cs
- NoResizeSelectionBorderGlyph.cs
- DecimalAnimation.cs
- String.cs
- DataFieldConverter.cs
- XmlSignatureProperties.cs
- NameTable.cs
- ValidatorAttribute.cs
- InternalCache.cs
- XsltInput.cs
- PixelShader.cs
- Thickness.cs
- HashSetEqualityComparer.cs
- UTF7Encoding.cs
- AuthenticatedStream.cs
- NamespaceCollection.cs
- ActivityScheduledQuery.cs
- StoryFragments.cs
- DefaultProxySection.cs
- InlineObject.cs
- DebugController.cs
- SqlDependencyUtils.cs
- PropertyEmitterBase.cs
- xmlfixedPageInfo.cs
- TrustLevel.cs
- ContainsRowNumberChecker.cs
- OwnerDrawPropertyBag.cs
- TreeNode.cs
- UseLicense.cs
- StylusPointPropertyInfo.cs
- EditorAttributeInfo.cs
- MultitargetUtil.cs
- ToolBarButtonClickEvent.cs
- Classification.cs
- GuidelineSet.cs
- Selection.cs
- SwitchElementsCollection.cs
- FixUpCollection.cs
- CharStorage.cs
- StopStoryboard.cs
- MessageHeader.cs
- SoapIncludeAttribute.cs
- IntPtr.cs
- MethodExpr.cs
- KerberosTicketHashIdentifierClause.cs
- ForwardPositionQuery.cs
- AnnotationObservableCollection.cs
- QueryPageSettingsEventArgs.cs
- WmpBitmapDecoder.cs
- SendKeys.cs
- SerialReceived.cs
- AssemblyAssociatedContentFileAttribute.cs
- Aggregates.cs
- dtdvalidator.cs
- WebPartHeaderCloseVerb.cs
- XmlSerializableWriter.cs
- PenThreadWorker.cs
- ChtmlTextBoxAdapter.cs
- UnmanagedHandle.cs
- InternalBufferManager.cs
- DockPanel.cs
- UriTemplateTableMatchCandidate.cs
- ContentElementAutomationPeer.cs
- FilterQueryOptionExpression.cs
- DeferredTextReference.cs
- ControllableStoryboardAction.cs
- XMLDiffLoader.cs
- CacheMemory.cs
- ClientBuildManager.cs
- UnsafeMethods.cs
- DockingAttribute.cs
- ThrowHelper.cs
- ExpressionBuilder.cs
- JpegBitmapEncoder.cs
- PointIndependentAnimationStorage.cs
- UInt32.cs
- PasswordDeriveBytes.cs
- DiagnosticsConfiguration.cs
- ReverseInheritProperty.cs
- SimpleTextLine.cs
- StringStorage.cs
- PowerModeChangedEventArgs.cs
- SerializerWriterEventHandlers.cs
- SharedUtils.cs
- Utilities.cs
- CapabilitiesAssignment.cs
- ConfigErrorGlyph.cs
- _NtlmClient.cs
- RtfToXamlReader.cs
- ValueProviderWrapper.cs
- translator.cs
- LinkClickEvent.cs