Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / WinForms / Managed / System / WinForms / ToolStripItemCollection.cs / 1 / ToolStripItemCollection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System; using System.Collections; using System.ComponentModel; using System.ComponentModel.Design; using System.Drawing.Design; using System.Diagnostics; using System.Windows.Forms.Layout; using System.Drawing; using System.Security; using System.Security.Permissions; ////// /// Summary description for ToolStripItemCollection. /// [ Editor("System.Windows.Forms.Design.ToolStripCollectionEditor, " + AssemblyRef.SystemDesign, typeof(UITypeEditor)), ListBindable(false), UIPermission(SecurityAction.InheritanceDemand, Window=UIPermissionWindow.AllWindows) ] public class ToolStripItemCollection : ArrangedElementCollection, IList { private ToolStrip owner; private bool itemsCollection; private bool isReadOnly = false; /// A caching mechanism for key accessor /// We use an index here rather than control so that we don't have lifetime /// issues by holding on to extra references. /// Note this is not Thread Safe - but Winforms has to be run in a STA anyways. private int lastAccessedIndex = -1; internal ToolStripItemCollection(ToolStrip owner, bool itemsCollection) : this(owner, itemsCollection, /*isReadOnly=*/false){ } ////// internal ToolStripItemCollection(ToolStrip owner, bool itemsCollection, bool isReadOnly) { this.owner = owner; this.itemsCollection = itemsCollection; this.isReadOnly = isReadOnly; } ///[To be supplied.] ////// /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA2214:DoNotCallOverridableMethodsInConstructors")] public ToolStripItemCollection(ToolStrip owner, ToolStripItem[] value) { if (owner == null) { throw new ArgumentNullException("owner"); } this.owner = owner; AddRange(value); } ///[To be supplied.] ////// /// public new virtual ToolStripItem this[int index] { get { return (ToolStripItem)(InnerList[index]); } } ////// /// /// public virtual ToolStripItem this[string key] { get { // We do not support null and empty string as valid keys. if ((key == null) || (key.Length == 0)){ return null; } // Search for the key in our collection int index = IndexOfKey(key); if (IsValidIndex(index)) { return (ToolStripItem)InnerList[index]; } else { return null; } } } public ToolStripItem Add(string text) { return Add(text,null,null); } public ToolStripItem Add(Image image) { return Add(null,image,null); } public ToolStripItem Add(string text, Image image) { return Add(text,image,null); } public ToolStripItem Add(string text, Image image, EventHandler onClick) { ToolStripItem item = owner.CreateDefaultItem(text,image,onClick); Add(item); return item; } ///Retrieves the child control with the specified key. ////// /// public int Add(ToolStripItem value) { CheckCanAddOrInsertItem(value); SetOwner(value); int retVal = InnerList.Add(value); if (itemsCollection && owner != null) { owner.OnItemAdded(new ToolStripItemEventArgs(value)); } return retVal; } ///[To be supplied.] ////// /// public void AddRange(ToolStripItem[] toolStripItems) { if (toolStripItems == null) { throw new ArgumentNullException("toolStripItems"); } if (IsReadOnly) { throw new NotSupportedException(SR.GetString(SR.ToolStripItemCollectionIsReadOnly)); } // ToolStripDropDown will look for PropertyNames.Items to determine if it needs // to resize itself. using (new LayoutTransaction(this.owner, this.owner, PropertyNames.Items)) { for (int i = 0; i < toolStripItems.Length; i++) { this.Add(toolStripItems[i]); } } } ///[To be supplied.] ////// /// public void AddRange(ToolStripItemCollection toolStripItems) { if (toolStripItems == null) { throw new ArgumentNullException("toolStripItems"); } if (IsReadOnly) { throw new NotSupportedException(SR.GetString(SR.ToolStripItemCollectionIsReadOnly)); } // ToolStripDropDown will look for PropertyNames.Items to determine if it needs // to resize itself. using (new LayoutTransaction(this.owner, this.owner, PropertyNames.Items)) { int currentCount = toolStripItems.Count; for (int i = 0; i < currentCount; i++) { this.Add(toolStripItems[i]); } } } ///[To be supplied.] ////// /// public bool Contains(ToolStripItem value) { return InnerList.Contains(value); } ///[To be supplied.] ////// /// public virtual void Clear() { if (IsReadOnly) { throw new NotSupportedException(SR.GetString(SR.ToolStripItemCollectionIsReadOnly)); } if (Count == 0) { return; } ToolStripOverflow overflow = null; if (owner != null && !owner.IsDisposingItems) { owner.SuspendLayout(); overflow = owner.GetOverflow(); if (overflow != null) { overflow.SuspendLayout(); } } try { while (Count != 0) { RemoveAt(Count - 1); } } finally { if (overflow != null) { overflow.ResumeLayout(false); } if (owner != null && !owner.IsDisposingItems) { owner.ResumeLayout(); } } } ///[To be supplied.] ////// /// public virtual bool ContainsKey(string key) { return IsValidIndex(IndexOfKey(key)); } private void CheckCanAddOrInsertItem(ToolStripItem value) { if (value == null) { throw new ArgumentNullException("value"); } if (IsReadOnly) { throw new NotSupportedException(SR.GetString(SR.ToolStripItemCollectionIsReadOnly)); } ToolStripDropDown dropDown = owner as ToolStripDropDown; if (dropDown != null) { // VSWhidbey 436973: if we're on a dropdown, we can only add non-control host items // as we dont want anything on a dropdown to get keyboard messages in the Internet. if (dropDown.OwnerItem == value) { throw new NotSupportedException(SR.GetString(SR.ToolStripItemCircularReference)); } // VSWhidbey 496526: ScrollButton is the only allowed control host as it correctly eats key messages. if (value is ToolStripControlHost && !(value is System.Windows.Forms.ToolStripScrollButton)) { if (dropDown.IsRestrictedWindow) { IntSecurity.AllWindows.Demand(); } } } } ///Returns true if the collection contains an item with the specified key, false otherwise. ////// /// public ToolStripItem[] Find(string key, bool searchAllChildren) { if ((key == null) || (key.Length == 0)) { throw new System.ArgumentNullException("key", SR.GetString(SR.FindKeyMayNotBeEmptyOrNull)); } ArrayList foundItems = FindInternal(key, searchAllChildren, this, new ArrayList()); // Make this a stongly typed collection. ToolStripItem[] stronglyTypedFoundItems = new ToolStripItem[foundItems.Count]; foundItems.CopyTo(stronglyTypedFoundItems, 0); return stronglyTypedFoundItems; } ///Searches for Items by their Name property, builds up an array /// of all the controls that match. /// ////// ///Searches for Items by their Name property, builds up an array list /// of all the items that match. /// ///private ArrayList FindInternal(string key, bool searchAllChildren, ToolStripItemCollection itemsToLookIn, ArrayList foundItems) { if ((itemsToLookIn == null) || (foundItems == null)) { return null; // } try { for (int i = 0; i < itemsToLookIn.Count; i++) { if (itemsToLookIn[i] == null) { continue; } if (WindowsFormsUtils.SafeCompareStrings(itemsToLookIn[i].Name, key, /* ignoreCase = */ true)) { foundItems.Add(itemsToLookIn[i]); } } // Optional recurive search for controls in child collections. if (searchAllChildren) { for (int j = 0; j < itemsToLookIn.Count; j++) { ToolStripDropDownItem item = itemsToLookIn[j] as ToolStripDropDownItem; if (item == null) { continue; } if (item.HasDropDownItems) { // if it has a valid child collecion, append those results to our collection foundItems = FindInternal(key, searchAllChildren, item.DropDownItems, foundItems); } } } } catch (Exception e) { // VSWHIDBEY 80122 make sure we deal with non-critical failures gracefully. if (ClientUtils.IsCriticalException(e)) { throw; } } return foundItems; } public override bool IsReadOnly { get { return this.isReadOnly; }} void IList.Clear() { Clear(); } bool IList.IsFixedSize { get { return InnerList.IsFixedSize; }} bool IList.Contains(object value) { return InnerList.Contains(value); } void IList.RemoveAt(int index) { RemoveAt(index); } void IList.Remove(object value) { Remove(value as ToolStripItem); } int IList.Add(object value) { return Add(value as ToolStripItem); } int IList.IndexOf(object value) { return IndexOf(value as ToolStripItem); } void IList.Insert(int index, object value) { Insert(index, value as ToolStripItem); } /// /// object IList.this[int index] { get { return InnerList[index]; } set { throw new NotSupportedException(SR.GetString(SR.ToolStripCollectionMustInsertAndRemove)); /* InnerList[index] = value; */ } } /// /// /// public void Insert(int index, ToolStripItem value) { CheckCanAddOrInsertItem(value); SetOwner(value); InnerList.Insert(index, value); if (itemsCollection && owner != null) { if (owner.IsHandleCreated){ LayoutTransaction.DoLayout(owner, value, PropertyNames.Parent); } else { // next time we fetch the preferred size, recalc it. CommonProperties.xClearPreferredSizeCache(owner); } owner.OnItemAdded(new ToolStripItemEventArgs(value)); } } ///[To be supplied.] ////// /// public int IndexOf(ToolStripItem value) { return InnerList.IndexOf(value); } ///[To be supplied.] ////// /// public virtual int IndexOfKey(String key) { // Step 0 - Arg validation if ((key == null) || (key.Length == 0)){ return -1; // we dont support empty or null keys. } // step 1 - check the last cached item if (IsValidIndex(lastAccessedIndex)) { if (WindowsFormsUtils.SafeCompareStrings(this[lastAccessedIndex].Name, key, /* ignoreCase = */ true)) { return lastAccessedIndex; } } // step 2 - search for the item for (int i = 0; i < this.Count; i ++) { if (WindowsFormsUtils.SafeCompareStrings(this[i].Name, key, /* ignoreCase = */ true)) { lastAccessedIndex = i; return i; } } // step 3 - we didn't find it. Invalidate the last accessed index and return -1. lastAccessedIndex = -1; return -1; } ///The zero-based index of the first occurrence of value within the entire CollectionBase, if found; otherwise, -1. ////// ///Determines if the index is valid for the collection. ///private bool IsValidIndex(int index) { return ((index >= 0) && (index < this.Count)); } /// /// Do proper cleanup of ownership, etc. /// private void OnAfterRemove(ToolStripItem item) { if (itemsCollection) { ToolStrip parent = null; if (item != null) { parent = item.ParentInternal; item.SetOwner(null); } if (owner != null) { if (!owner.IsDisposingItems) { ToolStripItemEventArgs e = new ToolStripItemEventArgs(item); owner.OnItemRemoved(e); // VSWhidbey 505129 // dont fire the ItemRemoved event for Overflow // it would fire constantly.... instead clear any state if the item // is really being removed from the master collection. if (parent != null && parent != owner) { parent.OnItemVisibleChanged(e, /*performLayout*/false); } } } } } ////// /// public void Remove(ToolStripItem value) { if (IsReadOnly) { throw new NotSupportedException(SR.GetString(SR.ToolStripItemCollectionIsReadOnly)); } InnerList.Remove(value); OnAfterRemove(value); } ///[To be supplied.] ////// /// public void RemoveAt(int index) { if (IsReadOnly) { throw new NotSupportedException(SR.GetString(SR.ToolStripItemCollectionIsReadOnly)); } ToolStripItem item = null; if (index < Count && index >= 0) { item = (ToolStripItem)(InnerList[index]); } InnerList.RemoveAt(index); OnAfterRemove(item); } ///[To be supplied.] ////// /// public virtual void RemoveByKey(string key) { if (IsReadOnly) { throw new NotSupportedException(SR.GetString(SR.ToolStripItemCollectionIsReadOnly)); } int index = IndexOfKey(key); if (IsValidIndex(index)) { RemoveAt(index); } } ///Removes the child item with the specified key. ////// /// public void CopyTo(ToolStripItem[] array, int index) { InnerList.CopyTo(array, index); } // internal void MoveItem(ToolStripItem value) { if (value.ParentInternal != null) { int indexOfItem = value.ParentInternal.Items.IndexOf(value); if (indexOfItem >= 0) { value.ParentInternal.Items.RemoveAt(indexOfItem); } } Add(value); } internal void MoveItem(int index, ToolStripItem value) { // if moving to the end - call add instead. if (index == this.Count) { MoveItem(value); return; } if (value.ParentInternal != null) { int indexOfItem = value.ParentInternal.Items.IndexOf(value); if (indexOfItem >= 0) { value.ParentInternal.Items.RemoveAt(indexOfItem); if ((value.ParentInternal == owner) && (index > indexOfItem)) { index--; } } } Insert(index,value); } private void SetOwner(ToolStripItem item) { if (itemsCollection) { if (item != null) { if (item.Owner != null) { item.Owner.Items.Remove(item); } item.SetOwner(owner); if (item.Renderer != null) { item.Renderer.InitializeItem(item); } } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //[To be supplied.] ///// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System; using System.Collections; using System.ComponentModel; using System.ComponentModel.Design; using System.Drawing.Design; using System.Diagnostics; using System.Windows.Forms.Layout; using System.Drawing; using System.Security; using System.Security.Permissions; ////// /// Summary description for ToolStripItemCollection. /// [ Editor("System.Windows.Forms.Design.ToolStripCollectionEditor, " + AssemblyRef.SystemDesign, typeof(UITypeEditor)), ListBindable(false), UIPermission(SecurityAction.InheritanceDemand, Window=UIPermissionWindow.AllWindows) ] public class ToolStripItemCollection : ArrangedElementCollection, IList { private ToolStrip owner; private bool itemsCollection; private bool isReadOnly = false; /// A caching mechanism for key accessor /// We use an index here rather than control so that we don't have lifetime /// issues by holding on to extra references. /// Note this is not Thread Safe - but Winforms has to be run in a STA anyways. private int lastAccessedIndex = -1; internal ToolStripItemCollection(ToolStrip owner, bool itemsCollection) : this(owner, itemsCollection, /*isReadOnly=*/false){ } ////// internal ToolStripItemCollection(ToolStrip owner, bool itemsCollection, bool isReadOnly) { this.owner = owner; this.itemsCollection = itemsCollection; this.isReadOnly = isReadOnly; } ///[To be supplied.] ////// /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA2214:DoNotCallOverridableMethodsInConstructors")] public ToolStripItemCollection(ToolStrip owner, ToolStripItem[] value) { if (owner == null) { throw new ArgumentNullException("owner"); } this.owner = owner; AddRange(value); } ///[To be supplied.] ////// /// public new virtual ToolStripItem this[int index] { get { return (ToolStripItem)(InnerList[index]); } } ////// /// /// public virtual ToolStripItem this[string key] { get { // We do not support null and empty string as valid keys. if ((key == null) || (key.Length == 0)){ return null; } // Search for the key in our collection int index = IndexOfKey(key); if (IsValidIndex(index)) { return (ToolStripItem)InnerList[index]; } else { return null; } } } public ToolStripItem Add(string text) { return Add(text,null,null); } public ToolStripItem Add(Image image) { return Add(null,image,null); } public ToolStripItem Add(string text, Image image) { return Add(text,image,null); } public ToolStripItem Add(string text, Image image, EventHandler onClick) { ToolStripItem item = owner.CreateDefaultItem(text,image,onClick); Add(item); return item; } ///Retrieves the child control with the specified key. ////// /// public int Add(ToolStripItem value) { CheckCanAddOrInsertItem(value); SetOwner(value); int retVal = InnerList.Add(value); if (itemsCollection && owner != null) { owner.OnItemAdded(new ToolStripItemEventArgs(value)); } return retVal; } ///[To be supplied.] ////// /// public void AddRange(ToolStripItem[] toolStripItems) { if (toolStripItems == null) { throw new ArgumentNullException("toolStripItems"); } if (IsReadOnly) { throw new NotSupportedException(SR.GetString(SR.ToolStripItemCollectionIsReadOnly)); } // ToolStripDropDown will look for PropertyNames.Items to determine if it needs // to resize itself. using (new LayoutTransaction(this.owner, this.owner, PropertyNames.Items)) { for (int i = 0; i < toolStripItems.Length; i++) { this.Add(toolStripItems[i]); } } } ///[To be supplied.] ////// /// public void AddRange(ToolStripItemCollection toolStripItems) { if (toolStripItems == null) { throw new ArgumentNullException("toolStripItems"); } if (IsReadOnly) { throw new NotSupportedException(SR.GetString(SR.ToolStripItemCollectionIsReadOnly)); } // ToolStripDropDown will look for PropertyNames.Items to determine if it needs // to resize itself. using (new LayoutTransaction(this.owner, this.owner, PropertyNames.Items)) { int currentCount = toolStripItems.Count; for (int i = 0; i < currentCount; i++) { this.Add(toolStripItems[i]); } } } ///[To be supplied.] ////// /// public bool Contains(ToolStripItem value) { return InnerList.Contains(value); } ///[To be supplied.] ////// /// public virtual void Clear() { if (IsReadOnly) { throw new NotSupportedException(SR.GetString(SR.ToolStripItemCollectionIsReadOnly)); } if (Count == 0) { return; } ToolStripOverflow overflow = null; if (owner != null && !owner.IsDisposingItems) { owner.SuspendLayout(); overflow = owner.GetOverflow(); if (overflow != null) { overflow.SuspendLayout(); } } try { while (Count != 0) { RemoveAt(Count - 1); } } finally { if (overflow != null) { overflow.ResumeLayout(false); } if (owner != null && !owner.IsDisposingItems) { owner.ResumeLayout(); } } } ///[To be supplied.] ////// /// public virtual bool ContainsKey(string key) { return IsValidIndex(IndexOfKey(key)); } private void CheckCanAddOrInsertItem(ToolStripItem value) { if (value == null) { throw new ArgumentNullException("value"); } if (IsReadOnly) { throw new NotSupportedException(SR.GetString(SR.ToolStripItemCollectionIsReadOnly)); } ToolStripDropDown dropDown = owner as ToolStripDropDown; if (dropDown != null) { // VSWhidbey 436973: if we're on a dropdown, we can only add non-control host items // as we dont want anything on a dropdown to get keyboard messages in the Internet. if (dropDown.OwnerItem == value) { throw new NotSupportedException(SR.GetString(SR.ToolStripItemCircularReference)); } // VSWhidbey 496526: ScrollButton is the only allowed control host as it correctly eats key messages. if (value is ToolStripControlHost && !(value is System.Windows.Forms.ToolStripScrollButton)) { if (dropDown.IsRestrictedWindow) { IntSecurity.AllWindows.Demand(); } } } } ///Returns true if the collection contains an item with the specified key, false otherwise. ////// /// public ToolStripItem[] Find(string key, bool searchAllChildren) { if ((key == null) || (key.Length == 0)) { throw new System.ArgumentNullException("key", SR.GetString(SR.FindKeyMayNotBeEmptyOrNull)); } ArrayList foundItems = FindInternal(key, searchAllChildren, this, new ArrayList()); // Make this a stongly typed collection. ToolStripItem[] stronglyTypedFoundItems = new ToolStripItem[foundItems.Count]; foundItems.CopyTo(stronglyTypedFoundItems, 0); return stronglyTypedFoundItems; } ///Searches for Items by their Name property, builds up an array /// of all the controls that match. /// ////// ///Searches for Items by their Name property, builds up an array list /// of all the items that match. /// ///private ArrayList FindInternal(string key, bool searchAllChildren, ToolStripItemCollection itemsToLookIn, ArrayList foundItems) { if ((itemsToLookIn == null) || (foundItems == null)) { return null; // } try { for (int i = 0; i < itemsToLookIn.Count; i++) { if (itemsToLookIn[i] == null) { continue; } if (WindowsFormsUtils.SafeCompareStrings(itemsToLookIn[i].Name, key, /* ignoreCase = */ true)) { foundItems.Add(itemsToLookIn[i]); } } // Optional recurive search for controls in child collections. if (searchAllChildren) { for (int j = 0; j < itemsToLookIn.Count; j++) { ToolStripDropDownItem item = itemsToLookIn[j] as ToolStripDropDownItem; if (item == null) { continue; } if (item.HasDropDownItems) { // if it has a valid child collecion, append those results to our collection foundItems = FindInternal(key, searchAllChildren, item.DropDownItems, foundItems); } } } } catch (Exception e) { // VSWHIDBEY 80122 make sure we deal with non-critical failures gracefully. if (ClientUtils.IsCriticalException(e)) { throw; } } return foundItems; } public override bool IsReadOnly { get { return this.isReadOnly; }} void IList.Clear() { Clear(); } bool IList.IsFixedSize { get { return InnerList.IsFixedSize; }} bool IList.Contains(object value) { return InnerList.Contains(value); } void IList.RemoveAt(int index) { RemoveAt(index); } void IList.Remove(object value) { Remove(value as ToolStripItem); } int IList.Add(object value) { return Add(value as ToolStripItem); } int IList.IndexOf(object value) { return IndexOf(value as ToolStripItem); } void IList.Insert(int index, object value) { Insert(index, value as ToolStripItem); } /// /// object IList.this[int index] { get { return InnerList[index]; } set { throw new NotSupportedException(SR.GetString(SR.ToolStripCollectionMustInsertAndRemove)); /* InnerList[index] = value; */ } } /// /// /// public void Insert(int index, ToolStripItem value) { CheckCanAddOrInsertItem(value); SetOwner(value); InnerList.Insert(index, value); if (itemsCollection && owner != null) { if (owner.IsHandleCreated){ LayoutTransaction.DoLayout(owner, value, PropertyNames.Parent); } else { // next time we fetch the preferred size, recalc it. CommonProperties.xClearPreferredSizeCache(owner); } owner.OnItemAdded(new ToolStripItemEventArgs(value)); } } ///[To be supplied.] ////// /// public int IndexOf(ToolStripItem value) { return InnerList.IndexOf(value); } ///[To be supplied.] ////// /// public virtual int IndexOfKey(String key) { // Step 0 - Arg validation if ((key == null) || (key.Length == 0)){ return -1; // we dont support empty or null keys. } // step 1 - check the last cached item if (IsValidIndex(lastAccessedIndex)) { if (WindowsFormsUtils.SafeCompareStrings(this[lastAccessedIndex].Name, key, /* ignoreCase = */ true)) { return lastAccessedIndex; } } // step 2 - search for the item for (int i = 0; i < this.Count; i ++) { if (WindowsFormsUtils.SafeCompareStrings(this[i].Name, key, /* ignoreCase = */ true)) { lastAccessedIndex = i; return i; } } // step 3 - we didn't find it. Invalidate the last accessed index and return -1. lastAccessedIndex = -1; return -1; } ///The zero-based index of the first occurrence of value within the entire CollectionBase, if found; otherwise, -1. ////// ///Determines if the index is valid for the collection. ///private bool IsValidIndex(int index) { return ((index >= 0) && (index < this.Count)); } /// /// Do proper cleanup of ownership, etc. /// private void OnAfterRemove(ToolStripItem item) { if (itemsCollection) { ToolStrip parent = null; if (item != null) { parent = item.ParentInternal; item.SetOwner(null); } if (owner != null) { if (!owner.IsDisposingItems) { ToolStripItemEventArgs e = new ToolStripItemEventArgs(item); owner.OnItemRemoved(e); // VSWhidbey 505129 // dont fire the ItemRemoved event for Overflow // it would fire constantly.... instead clear any state if the item // is really being removed from the master collection. if (parent != null && parent != owner) { parent.OnItemVisibleChanged(e, /*performLayout*/false); } } } } } ////// /// public void Remove(ToolStripItem value) { if (IsReadOnly) { throw new NotSupportedException(SR.GetString(SR.ToolStripItemCollectionIsReadOnly)); } InnerList.Remove(value); OnAfterRemove(value); } ///[To be supplied.] ////// /// public void RemoveAt(int index) { if (IsReadOnly) { throw new NotSupportedException(SR.GetString(SR.ToolStripItemCollectionIsReadOnly)); } ToolStripItem item = null; if (index < Count && index >= 0) { item = (ToolStripItem)(InnerList[index]); } InnerList.RemoveAt(index); OnAfterRemove(item); } ///[To be supplied.] ////// /// public virtual void RemoveByKey(string key) { if (IsReadOnly) { throw new NotSupportedException(SR.GetString(SR.ToolStripItemCollectionIsReadOnly)); } int index = IndexOfKey(key); if (IsValidIndex(index)) { RemoveAt(index); } } ///Removes the child item with the specified key. ////// /// public void CopyTo(ToolStripItem[] array, int index) { InnerList.CopyTo(array, index); } // internal void MoveItem(ToolStripItem value) { if (value.ParentInternal != null) { int indexOfItem = value.ParentInternal.Items.IndexOf(value); if (indexOfItem >= 0) { value.ParentInternal.Items.RemoveAt(indexOfItem); } } Add(value); } internal void MoveItem(int index, ToolStripItem value) { // if moving to the end - call add instead. if (index == this.Count) { MoveItem(value); return; } if (value.ParentInternal != null) { int indexOfItem = value.ParentInternal.Items.IndexOf(value); if (indexOfItem >= 0) { value.ParentInternal.Items.RemoveAt(indexOfItem); if ((value.ParentInternal == owner) && (index > indexOfItem)) { index--; } } } Insert(index,value); } private void SetOwner(ToolStripItem item) { if (itemsCollection) { if (item != null) { if (item.Owner != null) { item.Owner.Items.Remove(item); } item.SetOwner(owner); if (item.Renderer != null) { item.Renderer.InitializeItem(item); } } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.[To be supplied.] ///
Link Menu
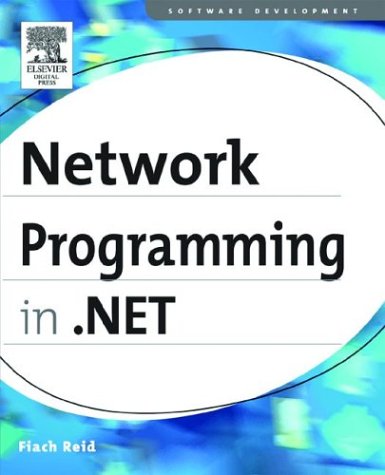
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- EditorPartDesigner.cs
- AuthenticateEventArgs.cs
- CodeTypeConstructor.cs
- ConstructorBuilder.cs
- ExpressionPrefixAttribute.cs
- ParserExtension.cs
- InfoCardSchemas.cs
- CodeConditionStatement.cs
- SHA384Managed.cs
- StreamResourceInfo.cs
- DbParameterHelper.cs
- SqlEnums.cs
- RulePatternOps.cs
- StackOverflowException.cs
- Win32SafeHandles.cs
- StreamAsIStream.cs
- TileBrush.cs
- RegionInfo.cs
- HwndKeyboardInputProvider.cs
- OledbConnectionStringbuilder.cs
- ProcessProtocolHandler.cs
- BamlRecords.cs
- MailSettingsSection.cs
- OutOfProcStateClientManager.cs
- CustomLineCap.cs
- dbenumerator.cs
- DrawingAttributesDefaultValueFactory.cs
- WsdlParser.cs
- LiteralTextContainerControlBuilder.cs
- StorageEntityTypeMapping.cs
- Pkcs7Recipient.cs
- NullableDecimalSumAggregationOperator.cs
- SurrogateSelector.cs
- Vector3D.cs
- FormViewUpdateEventArgs.cs
- COM2IPerPropertyBrowsingHandler.cs
- DispatcherEventArgs.cs
- PathTooLongException.cs
- WindowsToolbar.cs
- PageAsyncTaskManager.cs
- DSACryptoServiceProvider.cs
- StringDictionary.cs
- IntellisenseTextBox.designer.cs
- _IPv6Address.cs
- Utility.cs
- DynamicMethod.cs
- PolyQuadraticBezierSegment.cs
- ExpressionWriter.cs
- DiagnosticsConfigurationHandler.cs
- SchemaSetCompiler.cs
- QilCloneVisitor.cs
- RankException.cs
- DataGridViewCellCollection.cs
- DocumentScope.cs
- SqlProfileProvider.cs
- TextTreeUndoUnit.cs
- StringBuilder.cs
- PassportAuthenticationEventArgs.cs
- AsymmetricKeyExchangeFormatter.cs
- SharedStatics.cs
- SafeRightsManagementPubHandle.cs
- ACL.cs
- ConnectionManagementElement.cs
- CodeTypeMember.cs
- ComponentRenameEvent.cs
- Parameter.cs
- Cursors.cs
- HtmlDocument.cs
- NamespaceMapping.cs
- SmiRecordBuffer.cs
- Invariant.cs
- OnOperation.cs
- wgx_sdk_version.cs
- RowUpdatingEventArgs.cs
- CodeMethodInvokeExpression.cs
- RowParagraph.cs
- TypeInitializationException.cs
- PageSetupDialog.cs
- LinqDataSourceDisposeEventArgs.cs
- RemotingServices.cs
- InfoCardKeyedHashAlgorithm.cs
- SchemaTableColumn.cs
- BitmapEffectInputData.cs
- XmlWriter.cs
- DataBindEngine.cs
- DependencyPropertyHelper.cs
- MatchingStyle.cs
- QueryRewriter.cs
- glyphs.cs
- ToolStrip.cs
- GlyphRunDrawing.cs
- ControlTemplate.cs
- HtmlTitle.cs
- OdbcParameter.cs
- BooleanKeyFrameCollection.cs
- StreamMarshaler.cs
- MessageDesigner.cs
- MouseGesture.cs
- TableItemProviderWrapper.cs
- Converter.cs