Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Speech / Src / Recognition / SrgsGrammar / SrgsElementFactory.cs / 1 / SrgsElementFactory.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: // // History: // 5/1/2004 jeanfp Created from the Kurosawa Code //--------------------------------------------------------------------------- #region Using directives using System; using System.Speech.Internal; using System.Speech.Internal.SrgsParser; #endregion namespace System.Speech.Recognition.SrgsGrammar { internal class SrgsElementFactory : IElementFactory { internal SrgsElementFactory (SrgsGrammar grammar) { _grammar = grammar; } ////// Clear all the rules /// void IElementFactory.RemoveAllRules () { } IPropertyTag IElementFactory.CreatePropertyTag (IElement parent) { return (IPropertyTag) new SrgsNameValueTag (); } ISemanticTag IElementFactory.CreateSemanticTag (IElement parent) { return (ISemanticTag) new SrgsSemanticInterpretationTag (); } IElementText IElementFactory.CreateText (IElement parent, string value) { return (IElementText) new SrgsText (value); } IToken IElementFactory.CreateToken (IElement parent, string content, string pronunciation, string display, float reqConfidence) { SrgsToken token = new SrgsToken (content); if (!string.IsNullOrEmpty (pronunciation)) { // Check if the pronunciations are ok string sPron = pronunciation; for (int iCurPron = 0, iDeliminator = 0; iCurPron < sPron.Length; iCurPron = iDeliminator + 1) { // Find semi-colon deliminator and replace with null iDeliminator = pronunciation.IndexOfAny (_pronSeparator, iCurPron); if (iDeliminator == -1) { iDeliminator = sPron.Length; } string sSubPron = sPron.Substring (iCurPron, iDeliminator - iCurPron); // make sure this goes through switch (_grammar.PhoneticAlphabet) { case AlphabetType.Sapi: sSubPron = PhonemeConverter.ConvertPronToId (sSubPron, _grammar.Culture.LCID); break; case AlphabetType.Ipa: PhonemeConverter.ValidateUpsIds (sSubPron); break; case AlphabetType.Ups: sSubPron = PhonemeConverter.UpsConverter.ConvertPronToId (sSubPron); break; } } token.Pronunciation = pronunciation; } if (!string.IsNullOrEmpty (display)) { token.Display = display; } if (reqConfidence >= 0) { throw new NotSupportedException (SR.Get (SRID.ReqConfidenceNotSupported)); } return (IToken) token; } IItem IElementFactory.CreateItem (IElement parent, IRule rule, int minRepeat, int maxRepeat, float repeatProbability, float weight) { SrgsItem item = new SrgsItem (); if (minRepeat != 1 || maxRepeat != 1) { item.SetRepeat (minRepeat, maxRepeat); } item.RepeatProbability = repeatProbability; item.Weight = weight; return (IItem) item; } IRuleRef IElementFactory.CreateRuleRef (IElement parent, Uri srgsUri) { return (IRuleRef) new SrgsRuleRef (srgsUri); } IRuleRef IElementFactory.CreateRuleRef (IElement parent, Uri srgsUri, string semanticKey, string parameters) { return (IRuleRef) new SrgsRuleRef (semanticKey, parameters, srgsUri); } IOneOf IElementFactory.CreateOneOf (IElement parent, IRule rule) { return (IOneOf) new SrgsOneOf (); } ISubset IElementFactory.CreateSubset (IElement parent, string text, MatchMode matchMode) { SubsetMatchingMode matchingMode = SubsetMatchingMode.Subsequence; switch (matchMode) { case MatchMode.OrderedSubset: matchingMode = SubsetMatchingMode.OrderedSubset; break; case MatchMode.OrderedSubsetContentRequired: matchingMode = SubsetMatchingMode.OrderedSubsetContentRequired; break; case MatchMode.Subsequence: matchingMode = SubsetMatchingMode.Subsequence; break; case MatchMode.SubsequenceContentRequired: matchingMode = SubsetMatchingMode.SubsequenceContentRequired; break; } return (ISubset) new SrgsSubset (text, matchingMode); } void IElementFactory.InitSpecialRuleRef (IElement parent, IRuleRef special) { } #if !NO_STG void IElementFactory.AddScript (IGrammar grammar, string sRule, string code) { SrgsGrammar srgsGrammar = (SrgsGrammar) grammar; SrgsRule rule = srgsGrammar.Rules [sRule]; if (rule != null) { rule.Script = rule.Script + code; } else { srgsGrammar.AddScript (sRule, code); } } string IElementFactory.AddScript (IGrammar grammar, string sRule, string code, string filename, int line) { return code; } void IElementFactory.AddScript (IGrammar grammar, string script, string filename, int line) { SrgsGrammar srgsGrammar = (SrgsGrammar) grammar; srgsGrammar.AddScript (null, script); } #endif void IElementFactory.AddItem (IOneOf oneOf, IItem value) { ((SrgsOneOf) oneOf).Add ((SrgsItem) value); } void IElementFactory.AddElement (IRule rule, IElement value) { ((SrgsRule) rule).Elements.Add ((SrgsElement) value); } void IElementFactory.AddElement (IItem item, IElement value) { ((SrgsItem) item).Elements.Add ((SrgsElement) value); } IGrammar IElementFactory.Grammar { get { return _grammar; } } IRuleRef IElementFactory.Null { get { return SrgsRuleRef.Null; } } IRuleRef IElementFactory.Void { get { return SrgsRuleRef.Void; } } IRuleRef IElementFactory.Garbage { get { return SrgsRuleRef.Garbage; } } private SrgsGrammar _grammar; private static readonly char [] _pronSeparator = new char [] { ' ', '\t', '\n', '\r', ';' }; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: // // History: // 5/1/2004 jeanfp Created from the Kurosawa Code //--------------------------------------------------------------------------- #region Using directives using System; using System.Speech.Internal; using System.Speech.Internal.SrgsParser; #endregion namespace System.Speech.Recognition.SrgsGrammar { internal class SrgsElementFactory : IElementFactory { internal SrgsElementFactory (SrgsGrammar grammar) { _grammar = grammar; } ////// Clear all the rules /// void IElementFactory.RemoveAllRules () { } IPropertyTag IElementFactory.CreatePropertyTag (IElement parent) { return (IPropertyTag) new SrgsNameValueTag (); } ISemanticTag IElementFactory.CreateSemanticTag (IElement parent) { return (ISemanticTag) new SrgsSemanticInterpretationTag (); } IElementText IElementFactory.CreateText (IElement parent, string value) { return (IElementText) new SrgsText (value); } IToken IElementFactory.CreateToken (IElement parent, string content, string pronunciation, string display, float reqConfidence) { SrgsToken token = new SrgsToken (content); if (!string.IsNullOrEmpty (pronunciation)) { // Check if the pronunciations are ok string sPron = pronunciation; for (int iCurPron = 0, iDeliminator = 0; iCurPron < sPron.Length; iCurPron = iDeliminator + 1) { // Find semi-colon deliminator and replace with null iDeliminator = pronunciation.IndexOfAny (_pronSeparator, iCurPron); if (iDeliminator == -1) { iDeliminator = sPron.Length; } string sSubPron = sPron.Substring (iCurPron, iDeliminator - iCurPron); // make sure this goes through switch (_grammar.PhoneticAlphabet) { case AlphabetType.Sapi: sSubPron = PhonemeConverter.ConvertPronToId (sSubPron, _grammar.Culture.LCID); break; case AlphabetType.Ipa: PhonemeConverter.ValidateUpsIds (sSubPron); break; case AlphabetType.Ups: sSubPron = PhonemeConverter.UpsConverter.ConvertPronToId (sSubPron); break; } } token.Pronunciation = pronunciation; } if (!string.IsNullOrEmpty (display)) { token.Display = display; } if (reqConfidence >= 0) { throw new NotSupportedException (SR.Get (SRID.ReqConfidenceNotSupported)); } return (IToken) token; } IItem IElementFactory.CreateItem (IElement parent, IRule rule, int minRepeat, int maxRepeat, float repeatProbability, float weight) { SrgsItem item = new SrgsItem (); if (minRepeat != 1 || maxRepeat != 1) { item.SetRepeat (minRepeat, maxRepeat); } item.RepeatProbability = repeatProbability; item.Weight = weight; return (IItem) item; } IRuleRef IElementFactory.CreateRuleRef (IElement parent, Uri srgsUri) { return (IRuleRef) new SrgsRuleRef (srgsUri); } IRuleRef IElementFactory.CreateRuleRef (IElement parent, Uri srgsUri, string semanticKey, string parameters) { return (IRuleRef) new SrgsRuleRef (semanticKey, parameters, srgsUri); } IOneOf IElementFactory.CreateOneOf (IElement parent, IRule rule) { return (IOneOf) new SrgsOneOf (); } ISubset IElementFactory.CreateSubset (IElement parent, string text, MatchMode matchMode) { SubsetMatchingMode matchingMode = SubsetMatchingMode.Subsequence; switch (matchMode) { case MatchMode.OrderedSubset: matchingMode = SubsetMatchingMode.OrderedSubset; break; case MatchMode.OrderedSubsetContentRequired: matchingMode = SubsetMatchingMode.OrderedSubsetContentRequired; break; case MatchMode.Subsequence: matchingMode = SubsetMatchingMode.Subsequence; break; case MatchMode.SubsequenceContentRequired: matchingMode = SubsetMatchingMode.SubsequenceContentRequired; break; } return (ISubset) new SrgsSubset (text, matchingMode); } void IElementFactory.InitSpecialRuleRef (IElement parent, IRuleRef special) { } #if !NO_STG void IElementFactory.AddScript (IGrammar grammar, string sRule, string code) { SrgsGrammar srgsGrammar = (SrgsGrammar) grammar; SrgsRule rule = srgsGrammar.Rules [sRule]; if (rule != null) { rule.Script = rule.Script + code; } else { srgsGrammar.AddScript (sRule, code); } } string IElementFactory.AddScript (IGrammar grammar, string sRule, string code, string filename, int line) { return code; } void IElementFactory.AddScript (IGrammar grammar, string script, string filename, int line) { SrgsGrammar srgsGrammar = (SrgsGrammar) grammar; srgsGrammar.AddScript (null, script); } #endif void IElementFactory.AddItem (IOneOf oneOf, IItem value) { ((SrgsOneOf) oneOf).Add ((SrgsItem) value); } void IElementFactory.AddElement (IRule rule, IElement value) { ((SrgsRule) rule).Elements.Add ((SrgsElement) value); } void IElementFactory.AddElement (IItem item, IElement value) { ((SrgsItem) item).Elements.Add ((SrgsElement) value); } IGrammar IElementFactory.Grammar { get { return _grammar; } } IRuleRef IElementFactory.Null { get { return SrgsRuleRef.Null; } } IRuleRef IElementFactory.Void { get { return SrgsRuleRef.Void; } } IRuleRef IElementFactory.Garbage { get { return SrgsRuleRef.Garbage; } } private SrgsGrammar _grammar; private static readonly char [] _pronSeparator = new char [] { ' ', '\t', '\n', '\r', ';' }; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
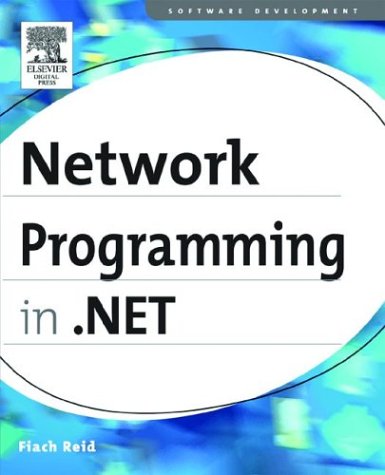
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MarkupObject.cs
- Configuration.cs
- LinqDataSourceUpdateEventArgs.cs
- TargetException.cs
- ObjectTag.cs
- DocobjHost.cs
- OleCmdHelper.cs
- ComponentGuaranteesAttribute.cs
- TimerEventSubscriptionCollection.cs
- BuilderInfo.cs
- mediaeventargs.cs
- WinEventWrap.cs
- TextTabProperties.cs
- XamlVector3DCollectionSerializer.cs
- RequestCache.cs
- GetPageCompletedEventArgs.cs
- cookie.cs
- TypeDefinition.cs
- StructureChangedEventArgs.cs
- DeviceContexts.cs
- unsafenativemethodstextservices.cs
- Globals.cs
- MetadataItemSerializer.cs
- DbModificationClause.cs
- AssemblyUtil.cs
- IPAddress.cs
- ConfigurationStrings.cs
- XmlDigitalSignatureProcessor.cs
- ContainerControl.cs
- SmtpReplyReaderFactory.cs
- AuthenticationException.cs
- TerminatorSinks.cs
- TrackingProfileDeserializationException.cs
- PagesChangedEventArgs.cs
- DataObjectFieldAttribute.cs
- NativeMethods.cs
- FontSourceCollection.cs
- WebConfigurationHost.cs
- SqlDataSourceConfigureSelectPanel.cs
- PeerToPeerException.cs
- DocumentScope.cs
- CreatingCookieEventArgs.cs
- ActivitySurrogateSelector.cs
- InstrumentationTracker.cs
- XmlSchemaImporter.cs
- StringInfo.cs
- SchemaDeclBase.cs
- ArrayEditor.cs
- GridViewCancelEditEventArgs.cs
- PlatformCulture.cs
- OutputCacheSettingsSection.cs
- newinstructionaction.cs
- FilterException.cs
- ColorBuilder.cs
- XmlILConstructAnalyzer.cs
- Activity.cs
- InstanceDataCollectionCollection.cs
- EncodingTable.cs
- TraceProvider.cs
- XsdDuration.cs
- HashAlgorithm.cs
- WindowsListViewScroll.cs
- Propagator.cs
- PackageDigitalSignatureManager.cs
- WindowsTab.cs
- IDictionary.cs
- dbdatarecord.cs
- SmiRecordBuffer.cs
- InputMethodStateTypeInfo.cs
- ResourceDescriptionAttribute.cs
- ReferenceSchema.cs
- SqlDataAdapter.cs
- XmlComment.cs
- diagnosticsswitches.cs
- InternalResources.cs
- WindowHideOrCloseTracker.cs
- OrderedDictionaryStateHelper.cs
- NullableConverter.cs
- SecurityPermission.cs
- HttpRawResponse.cs
- DateTimeConverter2.cs
- BmpBitmapDecoder.cs
- GlyphInfoList.cs
- CodeEventReferenceExpression.cs
- OleDbRowUpdatedEvent.cs
- XmlSchemas.cs
- DrawingContextDrawingContextWalker.cs
- ChangeInterceptorAttribute.cs
- GridViewColumn.cs
- Wizard.cs
- EtwTrackingBehavior.cs
- JoinQueryOperator.cs
- SessionEndingEventArgs.cs
- Decimal.cs
- RequiredAttributeAttribute.cs
- DynamicILGenerator.cs
- SocketException.cs
- ConnectionManagementElement.cs
- InternalSafeNativeMethods.cs
- SafeEventHandle.cs