Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / ndp / fx / src / DataEntity / System / Data / Map / Update / Internal / TableChangeProcessor.cs / 1 / TableChangeProcessor.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.Data.Metadata.Edm; using System.Collections.Generic; using System.Data.Common; using System.Data.Common.Utils; using System.Diagnostics; using System.Data.Entity; namespace System.Data.Mapping.Update.Internal { ////// Processes changes applying to a table by merging inserts and deletes into updates /// where appropriate. /// ////// This class is essentially responsible for identifying inserts, deletes /// and updates in a particular table based on the internal class TableChangeProcessor { #region Constructors ////// produced by value propagation w.r.t. the update mapping view for that table. /// Assumes the change node includes at most a single insert and at most a single delete /// for a given key (where we have both, the change is treated as an update). /// /// Constructs processor based on the contents of a change node. /// /// Table for which changes are being processed. internal TableChangeProcessor(EntitySet table) { EntityUtil.CheckArgumentNull(table, "table"); m_table = table; // cache information about table key m_keyOrdinals = InitializeKeyOrdinals(table); } #endregion #region Fields private readonly EntitySet m_table; private readonly int[] m_keyOrdinals; #endregion #region Properties ////// Gets metadata for the table being modified. /// internal EntitySet Table { get { return m_table; } } ////// Gets a map from column ordinal to property descriptions for columns that are components of the table's /// primary key. /// internal int[] KeyOrdinals { get { return m_keyOrdinals; } } #endregion #region Methods // Determines whether the given ordinal position in the property list // for this table is a key value. internal bool IsKeyProperty(int propertyOrdinal) { foreach (int keyOrdinal in m_keyOrdinals) { if (propertyOrdinal == keyOrdinal) { return true; } } return false; } // Determines which column ordinals in the table are part of the key. private static int[] InitializeKeyOrdinals(EntitySet table) { EntityType tableType = table.ElementType; IListkeyMembers = tableType.KeyMembers; IBaseList members = TypeHelpers.GetAllStructuralMembers(tableType); int[] keyOrdinals = new int[keyMembers.Count]; for (int keyMemberIndex = 0; keyMemberIndex < keyMembers.Count; keyMemberIndex++) { EdmMember keyMember = keyMembers[keyMemberIndex]; keyOrdinals[keyMemberIndex] = members.IndexOf(keyMember); Debug.Assert(keyOrdinals[keyMemberIndex] >= 0 && keyOrdinals[keyMemberIndex] < members.Count, "an EntityType key member must also be a member of the entity type"); } return keyOrdinals; } // Processes all insert and delete requests in the table's . Inserts // and deletes with the same key are merged into updates. internal List CompileCommands(ChangeNode changeNode, UpdateCompiler compiler) { Set keys = new Set (compiler.m_translator.KeyComparer); // Retrieve all delete results (original values) and insert results (current values) while // populating a set of all row keys. The set contains a single key per row. Dictionary deleteResults = ProcessKeys(compiler, changeNode.Deleted, keys); Dictionary insertResults = ProcessKeys(compiler, changeNode.Inserted, keys); List commands = new List (deleteResults.Count + insertResults.Count); // Examine each row key to see if the row is being deleted, inserted or updated foreach (CompositeKey key in keys) { PropagatorResult deleteResult; PropagatorResult insertResult; bool hasDelete = deleteResults.TryGetValue(key, out deleteResult); bool hasInsert = insertResults.TryGetValue(key, out insertResult); Debug.Assert(hasDelete || hasInsert, "(update/TableChangeProcessor) m_keys must not contain a value " + "if there is no corresponding insert or delete"); try { if (!hasDelete) { // this is an insert commands.Add(compiler.BuildInsertCommand(insertResult, this)); } else if (!hasInsert) { // this is a delete commands.Add(compiler.BuildDeleteCommand(deleteResult, this)); } else { // this is an update because it has both a delete result and an insert result UpdateCommand updateCommand = compiler.BuildUpdateCommand(deleteResult, insertResult, this); if (null != updateCommand) { // if null is returned, it means it is a no-op update commands.Add(updateCommand); } } } catch (Exception e) { if (UpdateTranslator.RequiresContext(e)) { // collect state entries in scope for the current compilation List stateEntries = new List (); if (null != deleteResult) { stateEntries.AddRange(SourceInterpreter.GetAllStateEntries( deleteResult, compiler.m_translator, m_table)); } if (null != insertResult) { stateEntries.AddRange(SourceInterpreter.GetAllStateEntries( insertResult, compiler.m_translator, m_table)); } throw EntityUtil.Update(System.Data.Entity.Strings.Update_GeneralExecutionException, e, stateEntries); } throw; } } return commands; } // Determines key values for a list of changes. Side effect: populates which // includes an entry for every key involved in a change. private Dictionary ProcessKeys(UpdateCompiler compiler, List changes, Set keys) { Dictionary map = new Dictionary ( compiler.m_translator.KeyComparer); foreach (PropagatorResult change in changes) { // Reassign change to row since we cannot modify iteration variable PropagatorResult row = change; CompositeKey key = new CompositeKey(GetKeyConstants(row)); map.Add(key, row); keys.Add(key); } return map; } // Extracts key constants from the given row. private PropagatorResult[] GetKeyConstants(PropagatorResult row) { PropagatorResult[] keyConstants = new PropagatorResult[m_keyOrdinals.Length]; for (int i = 0; i < m_keyOrdinals.Length; i++) { PropagatorResult constant = row.GetMemberValue(m_keyOrdinals[i]); keyConstants[i] = constant; } return keyConstants; } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.Data.Metadata.Edm; using System.Collections.Generic; using System.Data.Common; using System.Data.Common.Utils; using System.Diagnostics; using System.Data.Entity; namespace System.Data.Mapping.Update.Internal { ////// Processes changes applying to a table by merging inserts and deletes into updates /// where appropriate. /// ////// This class is essentially responsible for identifying inserts, deletes /// and updates in a particular table based on the internal class TableChangeProcessor { #region Constructors ////// produced by value propagation w.r.t. the update mapping view for that table. /// Assumes the change node includes at most a single insert and at most a single delete /// for a given key (where we have both, the change is treated as an update). /// /// Constructs processor based on the contents of a change node. /// /// Table for which changes are being processed. internal TableChangeProcessor(EntitySet table) { EntityUtil.CheckArgumentNull(table, "table"); m_table = table; // cache information about table key m_keyOrdinals = InitializeKeyOrdinals(table); } #endregion #region Fields private readonly EntitySet m_table; private readonly int[] m_keyOrdinals; #endregion #region Properties ////// Gets metadata for the table being modified. /// internal EntitySet Table { get { return m_table; } } ////// Gets a map from column ordinal to property descriptions for columns that are components of the table's /// primary key. /// internal int[] KeyOrdinals { get { return m_keyOrdinals; } } #endregion #region Methods // Determines whether the given ordinal position in the property list // for this table is a key value. internal bool IsKeyProperty(int propertyOrdinal) { foreach (int keyOrdinal in m_keyOrdinals) { if (propertyOrdinal == keyOrdinal) { return true; } } return false; } // Determines which column ordinals in the table are part of the key. private static int[] InitializeKeyOrdinals(EntitySet table) { EntityType tableType = table.ElementType; IListkeyMembers = tableType.KeyMembers; IBaseList members = TypeHelpers.GetAllStructuralMembers(tableType); int[] keyOrdinals = new int[keyMembers.Count]; for (int keyMemberIndex = 0; keyMemberIndex < keyMembers.Count; keyMemberIndex++) { EdmMember keyMember = keyMembers[keyMemberIndex]; keyOrdinals[keyMemberIndex] = members.IndexOf(keyMember); Debug.Assert(keyOrdinals[keyMemberIndex] >= 0 && keyOrdinals[keyMemberIndex] < members.Count, "an EntityType key member must also be a member of the entity type"); } return keyOrdinals; } // Processes all insert and delete requests in the table's . Inserts // and deletes with the same key are merged into updates. internal List CompileCommands(ChangeNode changeNode, UpdateCompiler compiler) { Set keys = new Set (compiler.m_translator.KeyComparer); // Retrieve all delete results (original values) and insert results (current values) while // populating a set of all row keys. The set contains a single key per row. Dictionary deleteResults = ProcessKeys(compiler, changeNode.Deleted, keys); Dictionary insertResults = ProcessKeys(compiler, changeNode.Inserted, keys); List commands = new List (deleteResults.Count + insertResults.Count); // Examine each row key to see if the row is being deleted, inserted or updated foreach (CompositeKey key in keys) { PropagatorResult deleteResult; PropagatorResult insertResult; bool hasDelete = deleteResults.TryGetValue(key, out deleteResult); bool hasInsert = insertResults.TryGetValue(key, out insertResult); Debug.Assert(hasDelete || hasInsert, "(update/TableChangeProcessor) m_keys must not contain a value " + "if there is no corresponding insert or delete"); try { if (!hasDelete) { // this is an insert commands.Add(compiler.BuildInsertCommand(insertResult, this)); } else if (!hasInsert) { // this is a delete commands.Add(compiler.BuildDeleteCommand(deleteResult, this)); } else { // this is an update because it has both a delete result and an insert result UpdateCommand updateCommand = compiler.BuildUpdateCommand(deleteResult, insertResult, this); if (null != updateCommand) { // if null is returned, it means it is a no-op update commands.Add(updateCommand); } } } catch (Exception e) { if (UpdateTranslator.RequiresContext(e)) { // collect state entries in scope for the current compilation List stateEntries = new List (); if (null != deleteResult) { stateEntries.AddRange(SourceInterpreter.GetAllStateEntries( deleteResult, compiler.m_translator, m_table)); } if (null != insertResult) { stateEntries.AddRange(SourceInterpreter.GetAllStateEntries( insertResult, compiler.m_translator, m_table)); } throw EntityUtil.Update(System.Data.Entity.Strings.Update_GeneralExecutionException, e, stateEntries); } throw; } } return commands; } // Determines key values for a list of changes. Side effect: populates which // includes an entry for every key involved in a change. private Dictionary ProcessKeys(UpdateCompiler compiler, List changes, Set keys) { Dictionary map = new Dictionary ( compiler.m_translator.KeyComparer); foreach (PropagatorResult change in changes) { // Reassign change to row since we cannot modify iteration variable PropagatorResult row = change; CompositeKey key = new CompositeKey(GetKeyConstants(row)); map.Add(key, row); keys.Add(key); } return map; } // Extracts key constants from the given row. private PropagatorResult[] GetKeyConstants(PropagatorResult row) { PropagatorResult[] keyConstants = new PropagatorResult[m_keyOrdinals.Length]; for (int i = 0; i < m_keyOrdinals.Length; i++) { PropagatorResult constant = row.GetMemberValue(m_keyOrdinals[i]); keyConstants[i] = constant; } return keyConstants; } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
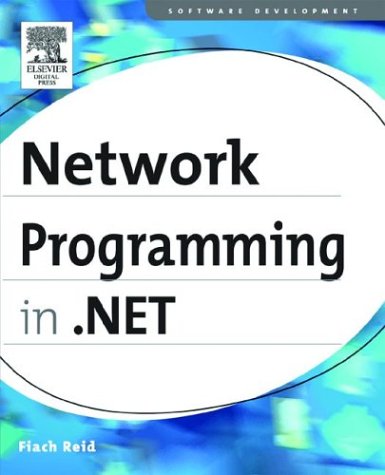
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ProvidePropertyAttribute.cs
- CompiledXpathExpr.cs
- ConstraintEnumerator.cs
- RightsManagementErrorHandler.cs
- TemplatePropertyEntry.cs
- TileBrush.cs
- ImageBrush.cs
- TreeNodeSelectionProcessor.cs
- TextEndOfLine.cs
- EditingCommands.cs
- AnnotationAdorner.cs
- XslTransform.cs
- DeploymentExceptionMapper.cs
- TeredoHelper.cs
- SmtpDigestAuthenticationModule.cs
- DispatchProxy.cs
- XmlSchemaFacet.cs
- CheckBoxField.cs
- TrackingMemoryStream.cs
- ScriptComponentDescriptor.cs
- HttpResponseWrapper.cs
- ProfileGroupSettingsCollection.cs
- RegisteredHiddenField.cs
- DynamicPropertyHolder.cs
- ExitEventArgs.cs
- StandardOleMarshalObject.cs
- UntrustedRecipientException.cs
- TextShapeableCharacters.cs
- TableCell.cs
- AudioFileOut.cs
- AuditLevel.cs
- COM2EnumConverter.cs
- EndEvent.cs
- ProcessInputEventArgs.cs
- ControlCollection.cs
- WindowManager.cs
- XmlMapping.cs
- AttributeCollection.cs
- ReversePositionQuery.cs
- GridItemCollection.cs
- QilXmlReader.cs
- DataError.cs
- TrackingServices.cs
- CharacterMetrics.cs
- ApplyTemplatesAction.cs
- EmptyStringExpandableObjectConverter.cs
- ViewLoader.cs
- RequestResizeEvent.cs
- TypeSystem.cs
- StructuredTypeEmitter.cs
- ImageListUtils.cs
- XmlSerializer.cs
- ImageAutomationPeer.cs
- DispatcherSynchronizationContext.cs
- StringWriter.cs
- CodeDomConfigurationHandler.cs
- ScrollPatternIdentifiers.cs
- ModifierKeysValueSerializer.cs
- RefreshEventArgs.cs
- PackagePartCollection.cs
- XslNumber.cs
- SurrogateSelector.cs
- CounterCreationData.cs
- RequestCachePolicyConverter.cs
- Vector3dCollection.cs
- DiscoveryMessageSequence.cs
- PersonalizationProvider.cs
- XPathCompileException.cs
- SocketManager.cs
- FixedSOMFixedBlock.cs
- KeyPullup.cs
- CLRBindingWorker.cs
- XmlUtil.cs
- WebControlAdapter.cs
- RecognitionEventArgs.cs
- DuplicateWaitObjectException.cs
- CodeCommentStatementCollection.cs
- ProfileWorkflowElement.cs
- HttpApplication.cs
- SQLInt32.cs
- CompilationLock.cs
- XPathExpr.cs
- SecurityBindingElementImporter.cs
- WebRequestModuleElement.cs
- Compress.cs
- DBCommand.cs
- ObjectRef.cs
- OracleException.cs
- WebPartManagerInternals.cs
- LiteralTextParser.cs
- NameValueFileSectionHandler.cs
- ButtonBaseAutomationPeer.cs
- PropertyToken.cs
- MasterPageParser.cs
- DBSqlParserColumn.cs
- TextEditorCopyPaste.cs
- DataGridViewComboBoxColumnDesigner.cs
- ClientScriptManager.cs
- WebReferencesBuildProvider.cs
- ChtmlSelectionListAdapter.cs