Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / ndp / fx / src / DataEntity / System / Data / Objects / DataClasses / RelationshipNavigation.cs / 1 / RelationshipNavigation.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Data.Common; using System.Globalization; using System.Text; namespace System.Data.Objects.DataClasses { ////// This class describes a relationship navigation from the /// navigation property on one entity to another entity. It is /// used throughout the collections and refs system to describe a /// relationship and to connect from the navigation property on /// one end of a relationship to the navigation property on the /// other end. /// // devnote: Might be able to change this back to a valuetype given its current purpose [Serializable] internal class RelationshipNavigation { // ------------ // Constructors // ------------ ////// Creates a navigation object with the given relationship /// name, role name for the source and role name for the /// destination. /// /// Canonical-space name of the relationship. /// Name of the role/navigation property which is the source of the navigation. /// Name of the role/navigation property which is the destination of the navigation. internal RelationshipNavigation(string relationshipName, string from, string to) { EntityUtil.CheckStringArgument(relationshipName, "relationshipName"); EntityUtil.CheckStringArgument(from, "from"); EntityUtil.CheckStringArgument(to, "to"); _relationshipName = relationshipName; _from = from; _to = to; } // ------ // Fields // ------ // The following fields are serialized. Adding or removing a serialized field is considered // a breaking change. This includes changing the field type or field name of existing // serialized fields. If you need to make this kind of change, it may be possible, but it // will require some custom serialization/deserialization code. private readonly string _relationshipName; private readonly string _from; private readonly string _to; [NonSerialized] private RelationshipNavigation _reverse; // ---------- // Properties // ---------- ////// Canonical-space relationship name. /// internal string RelationshipName { get { return _relationshipName; } } ////// Role/navigation property name for the source of this navigation. /// internal string From { get { return _from; } } ////// Role/navigation property name for the destination of this navigation. /// internal string To { get { return _to; } } ////// The "reverse" version of this navigation. /// internal RelationshipNavigation Reverse { get { if (_reverse == null) { // the reverse relationship is exactly like this // one but from & to are switched _reverse = new RelationshipNavigation(_relationshipName, _to, _from); } return _reverse; } } ////// Compares this instance to a given Navigation by their values. /// public override bool Equals(object obj) { RelationshipNavigation compareTo = obj as RelationshipNavigation; return ((this == compareTo) || ((null != this) && (null != compareTo) && (this.RelationshipName == compareTo.RelationshipName) && (this.From == compareTo.From) && (this.To == compareTo.To))); } ////// Returns a value-based hash code. /// ///the hash value of this Navigation public override int GetHashCode() { return this.RelationshipName.GetHashCode(); } // ------- // Methods // ------- ////// ToString is provided to simplify debugging, etc. /// public override string ToString() { return String.Format(CultureInfo.InvariantCulture, "RelationshipNavigation: ({0},{1},{2})", _relationshipName, _from, _to); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Data.Common; using System.Globalization; using System.Text; namespace System.Data.Objects.DataClasses { ////// This class describes a relationship navigation from the /// navigation property on one entity to another entity. It is /// used throughout the collections and refs system to describe a /// relationship and to connect from the navigation property on /// one end of a relationship to the navigation property on the /// other end. /// // devnote: Might be able to change this back to a valuetype given its current purpose [Serializable] internal class RelationshipNavigation { // ------------ // Constructors // ------------ ////// Creates a navigation object with the given relationship /// name, role name for the source and role name for the /// destination. /// /// Canonical-space name of the relationship. /// Name of the role/navigation property which is the source of the navigation. /// Name of the role/navigation property which is the destination of the navigation. internal RelationshipNavigation(string relationshipName, string from, string to) { EntityUtil.CheckStringArgument(relationshipName, "relationshipName"); EntityUtil.CheckStringArgument(from, "from"); EntityUtil.CheckStringArgument(to, "to"); _relationshipName = relationshipName; _from = from; _to = to; } // ------ // Fields // ------ // The following fields are serialized. Adding or removing a serialized field is considered // a breaking change. This includes changing the field type or field name of existing // serialized fields. If you need to make this kind of change, it may be possible, but it // will require some custom serialization/deserialization code. private readonly string _relationshipName; private readonly string _from; private readonly string _to; [NonSerialized] private RelationshipNavigation _reverse; // ---------- // Properties // ---------- ////// Canonical-space relationship name. /// internal string RelationshipName { get { return _relationshipName; } } ////// Role/navigation property name for the source of this navigation. /// internal string From { get { return _from; } } ////// Role/navigation property name for the destination of this navigation. /// internal string To { get { return _to; } } ////// The "reverse" version of this navigation. /// internal RelationshipNavigation Reverse { get { if (_reverse == null) { // the reverse relationship is exactly like this // one but from & to are switched _reverse = new RelationshipNavigation(_relationshipName, _to, _from); } return _reverse; } } ////// Compares this instance to a given Navigation by their values. /// public override bool Equals(object obj) { RelationshipNavigation compareTo = obj as RelationshipNavigation; return ((this == compareTo) || ((null != this) && (null != compareTo) && (this.RelationshipName == compareTo.RelationshipName) && (this.From == compareTo.From) && (this.To == compareTo.To))); } ////// Returns a value-based hash code. /// ///the hash value of this Navigation public override int GetHashCode() { return this.RelationshipName.GetHashCode(); } // ------- // Methods // ------- ////// ToString is provided to simplify debugging, etc. /// public override string ToString() { return String.Format(CultureInfo.InvariantCulture, "RelationshipNavigation: ({0},{1},{2})", _relationshipName, _from, _to); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
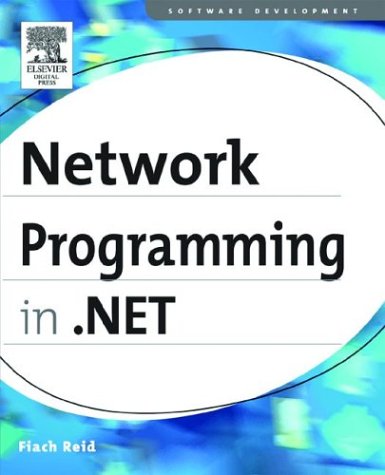
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- _HelperAsyncResults.cs
- DateTime.cs
- oledbconnectionstring.cs
- Model3DCollection.cs
- ListParaClient.cs
- TreeNodeBindingCollection.cs
- SelectiveScrollingGrid.cs
- FormClosedEvent.cs
- DefaultTextStore.cs
- VisualBrush.cs
- XamlDesignerSerializationManager.cs
- PolygonHotSpot.cs
- CookielessHelper.cs
- SHA256Managed.cs
- ConfigurationValidatorAttribute.cs
- ReliabilityContractAttribute.cs
- ClockController.cs
- BasicHttpMessageCredentialType.cs
- XmlUtilWriter.cs
- ListCollectionView.cs
- GetRecipientRequest.cs
- PackageRelationshipSelector.cs
- LinkTarget.cs
- EasingKeyFrames.cs
- Function.cs
- IItemContainerGenerator.cs
- EntityDataSourceChangingEventArgs.cs
- DetailsViewInsertedEventArgs.cs
- DoubleAnimationBase.cs
- XmlSerializerFactory.cs
- IBuiltInEvidence.cs
- TextElementEnumerator.cs
- ObjectDataSourceSelectingEventArgs.cs
- NavigationService.cs
- ConnectionPoolRegistry.cs
- Literal.cs
- ClientSettingsStore.cs
- ListDataBindEventArgs.cs
- TakeOrSkipWhileQueryOperator.cs
- CapabilitiesState.cs
- PublisherIdentityPermission.cs
- ProcessModelSection.cs
- GridViewDeleteEventArgs.cs
- ToolStripManager.cs
- Debug.cs
- MdImport.cs
- MsmqIntegrationProcessProtocolHandler.cs
- SqlDependencyListener.cs
- CompilerInfo.cs
- CodeMemberField.cs
- DataSourceXmlTextReader.cs
- LogRestartAreaEnumerator.cs
- TokenBasedSet.cs
- FileLogRecordHeader.cs
- DataGridPageChangedEventArgs.cs
- PagerSettings.cs
- ResourceExpression.cs
- LockedAssemblyCache.cs
- Pair.cs
- _LocalDataStoreMgr.cs
- GeneratedContractType.cs
- DependencyPropertyConverter.cs
- SiteOfOriginPart.cs
- NonVisualControlAttribute.cs
- DataGridViewColumnCollection.cs
- Int32EqualityComparer.cs
- EnumerableRowCollection.cs
- FileChangeNotifier.cs
- MenuCommands.cs
- CapiSymmetricAlgorithm.cs
- CngProperty.cs
- _NTAuthentication.cs
- InvokeHandlers.cs
- DataFormats.cs
- RelatedPropertyManager.cs
- Delegate.cs
- DesignerCategoryAttribute.cs
- DeferredTextReference.cs
- DiagnosticTrace.cs
- ParameterCollectionEditorForm.cs
- PlaceHolder.cs
- TextAction.cs
- ActivityDesignerResources.cs
- ZeroOpNode.cs
- MessageAction.cs
- RegexWriter.cs
- LoadMessageLogger.cs
- DesignerHost.cs
- JsonEncodingStreamWrapper.cs
- selecteditemcollection.cs
- ItemList.cs
- sqlstateclientmanager.cs
- AspNetSynchronizationContext.cs
- ControlUtil.cs
- Cursor.cs
- SHA512.cs
- DataReceivedEventArgs.cs
- WebScriptMetadataFormatter.cs
- PersonalizableTypeEntry.cs
- SignerInfo.cs