Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / ndp / fx / src / DataWeb / Design / system / Data / EntityModel / Emitters / AttributeEmitter.cs / 1 / AttributeEmitter.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.CodeDom; using System.Collections.Generic; using System.Data.Services.Design; using System.Data.Metadata.Edm; using System.Diagnostics; using System.Linq; namespace System.Data.EntityModel.Emitters { ////// Summary description for AttributeEmitter. /// internal sealed class AttributeEmitter { TypeReference _typeReference; internal TypeReference TypeReference { get { return _typeReference; } } internal AttributeEmitter(TypeReference typeReference) { _typeReference = typeReference; } ////// The method to be called to create the type level attributes for the ItemTypeEmitter /// /// The strongly typed emitter /// The type declaration to add the attribues to. public void EmitTypeAttributes(EntityTypeEmitter emitter, CodeTypeDeclaration typeDecl) { Debug.Assert(emitter != null, "emitter should not be null"); Debug.Assert(typeDecl != null, "typeDecl should not be null"); object[] keys = emitter.Item.KeyMembers.Select(km => (object) km.Name).ToArray(); typeDecl.CustomAttributes.Add(EmitSimpleAttribute(Utils.WebFrameworkCommonNamespace + "." + "DataServiceKeyAttribute", keys)); } ////// The method to be called to create the type level attributes for the StructuredTypeEmitter /// /// The strongly typed emitter /// The type declaration to add the attribues to. public void EmitTypeAttributes(StructuredTypeEmitter emitter, CodeTypeDeclaration typeDecl) { Debug.Assert(emitter != null, "emitter should not be null"); Debug.Assert(typeDecl != null, "typeDecl should not be null"); // nothing to do here yet } ////// The method to be called to create the type level attributes for the SchemaTypeEmitter /// /// The strongly typed emitter /// The type declaration to add the attribues to. public void EmitTypeAttributes(SchemaTypeEmitter emitter, CodeTypeDeclaration typeDecl) { Debug.Assert(emitter != null, "emitter should not be null"); Debug.Assert(typeDecl != null, "typeDecl should not be null"); } ////// The method to be called to create the property level attributes for the PropertyEmitter /// /// The strongly typed emitter /// The type declaration to add the attribues to. /// Additional attributes to emit public void EmitPropertyAttributes(PropertyEmitter emitter, CodeMemberProperty propertyDecl, ListadditionalAttributes) { if (additionalAttributes != null && additionalAttributes.Count > 0) { try { propertyDecl.CustomAttributes.AddRange(additionalAttributes.ToArray()); } catch (ArgumentNullException e) { emitter.Generator.AddError(Strings.InvalidAttributeSuppliedForProperty(emitter.Item.Name), ModelBuilderErrorCode.InvalidAttributeSuppliedForProperty, EdmSchemaErrorSeverity.Error, e); } } } /// /// The method to be called to create the type level attributes for the NestedTypeEmitter /// /// The strongly typed emitter /// The type declaration to add the attribues to. public void EmitTypeAttributes(ComplexTypeEmitter emitter, CodeTypeDeclaration typeDecl) { Debug.Assert(emitter != null, "emitter should not be null"); Debug.Assert(typeDecl != null, "typeDecl should not be null"); // not emitting System.Runtime.Serializaton.DataContractAttribute // not emitting System.Serializable } #region Static Methods ////// /// /// /// ///public CodeAttributeDeclaration EmitSimpleAttribute(string attributeType, params object[] arguments) { CodeAttributeDeclaration attribute = new CodeAttributeDeclaration(TypeReference.FromString(attributeType, true)); AddAttributeArguments(attribute, arguments); return attribute; } /// /// /// /// /// public static void AddAttributeArguments(CodeAttributeDeclaration attribute, object[] arguments) { foreach (object argument in arguments) { CodeExpression expression = argument as CodeExpression; if (expression == null) expression = new CodePrimitiveExpression(argument); attribute.Arguments.Add(new CodeAttributeArgument(expression)); } } ////// Adds an XmlIgnore attribute to the given property declaration. This is /// used to explicitly skip certain properties during XML serialization. /// /// the property to mark with XmlIgnore public void AddIgnoreAttributes(CodeMemberProperty propertyDecl) { // not emitting System.Xml.Serialization.XmlIgnoreAttribute // not emitting System.Xml.Serialization.SoapIgnoreAttribute } ////// Adds an Browsable(false) attribute to the given property declaration. /// This is used to explicitly avoid display property in the PropertyGrid. /// /// the property to mark with XmlIgnore public void AddBrowsableAttribute(CodeMemberProperty propertyDecl) { // not emitting System.ComponentModel.BrowsableAttribute } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.CodeDom; using System.Collections.Generic; using System.Data.Services.Design; using System.Data.Metadata.Edm; using System.Diagnostics; using System.Linq; namespace System.Data.EntityModel.Emitters { ////// Summary description for AttributeEmitter. /// internal sealed class AttributeEmitter { TypeReference _typeReference; internal TypeReference TypeReference { get { return _typeReference; } } internal AttributeEmitter(TypeReference typeReference) { _typeReference = typeReference; } ////// The method to be called to create the type level attributes for the ItemTypeEmitter /// /// The strongly typed emitter /// The type declaration to add the attribues to. public void EmitTypeAttributes(EntityTypeEmitter emitter, CodeTypeDeclaration typeDecl) { Debug.Assert(emitter != null, "emitter should not be null"); Debug.Assert(typeDecl != null, "typeDecl should not be null"); object[] keys = emitter.Item.KeyMembers.Select(km => (object) km.Name).ToArray(); typeDecl.CustomAttributes.Add(EmitSimpleAttribute(Utils.WebFrameworkCommonNamespace + "." + "DataServiceKeyAttribute", keys)); } ////// The method to be called to create the type level attributes for the StructuredTypeEmitter /// /// The strongly typed emitter /// The type declaration to add the attribues to. public void EmitTypeAttributes(StructuredTypeEmitter emitter, CodeTypeDeclaration typeDecl) { Debug.Assert(emitter != null, "emitter should not be null"); Debug.Assert(typeDecl != null, "typeDecl should not be null"); // nothing to do here yet } ////// The method to be called to create the type level attributes for the SchemaTypeEmitter /// /// The strongly typed emitter /// The type declaration to add the attribues to. public void EmitTypeAttributes(SchemaTypeEmitter emitter, CodeTypeDeclaration typeDecl) { Debug.Assert(emitter != null, "emitter should not be null"); Debug.Assert(typeDecl != null, "typeDecl should not be null"); } ////// The method to be called to create the property level attributes for the PropertyEmitter /// /// The strongly typed emitter /// The type declaration to add the attribues to. /// Additional attributes to emit public void EmitPropertyAttributes(PropertyEmitter emitter, CodeMemberProperty propertyDecl, ListadditionalAttributes) { if (additionalAttributes != null && additionalAttributes.Count > 0) { try { propertyDecl.CustomAttributes.AddRange(additionalAttributes.ToArray()); } catch (ArgumentNullException e) { emitter.Generator.AddError(Strings.InvalidAttributeSuppliedForProperty(emitter.Item.Name), ModelBuilderErrorCode.InvalidAttributeSuppliedForProperty, EdmSchemaErrorSeverity.Error, e); } } } /// /// The method to be called to create the type level attributes for the NestedTypeEmitter /// /// The strongly typed emitter /// The type declaration to add the attribues to. public void EmitTypeAttributes(ComplexTypeEmitter emitter, CodeTypeDeclaration typeDecl) { Debug.Assert(emitter != null, "emitter should not be null"); Debug.Assert(typeDecl != null, "typeDecl should not be null"); // not emitting System.Runtime.Serializaton.DataContractAttribute // not emitting System.Serializable } #region Static Methods ////// /// /// /// ///public CodeAttributeDeclaration EmitSimpleAttribute(string attributeType, params object[] arguments) { CodeAttributeDeclaration attribute = new CodeAttributeDeclaration(TypeReference.FromString(attributeType, true)); AddAttributeArguments(attribute, arguments); return attribute; } /// /// /// /// /// public static void AddAttributeArguments(CodeAttributeDeclaration attribute, object[] arguments) { foreach (object argument in arguments) { CodeExpression expression = argument as CodeExpression; if (expression == null) expression = new CodePrimitiveExpression(argument); attribute.Arguments.Add(new CodeAttributeArgument(expression)); } } ////// Adds an XmlIgnore attribute to the given property declaration. This is /// used to explicitly skip certain properties during XML serialization. /// /// the property to mark with XmlIgnore public void AddIgnoreAttributes(CodeMemberProperty propertyDecl) { // not emitting System.Xml.Serialization.XmlIgnoreAttribute // not emitting System.Xml.Serialization.SoapIgnoreAttribute } ////// Adds an Browsable(false) attribute to the given property declaration. /// This is used to explicitly avoid display property in the PropertyGrid. /// /// the property to mark with XmlIgnore public void AddBrowsableAttribute(CodeMemberProperty propertyDecl) { // not emitting System.ComponentModel.BrowsableAttribute } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
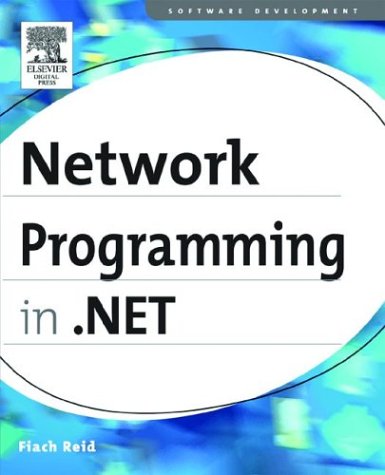
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DesignerOptionService.cs
- EFAssociationProvider.cs
- WindowProviderWrapper.cs
- TemplateDefinition.cs
- WebPartManager.cs
- EncoderReplacementFallback.cs
- MonitoringDescriptionAttribute.cs
- DynamicPropertyHolder.cs
- COM2TypeInfoProcessor.cs
- BrowserCapabilitiesCodeGenerator.cs
- HttpCapabilitiesBase.cs
- SelectionRange.cs
- SHA384Managed.cs
- Debug.cs
- HMACRIPEMD160.cs
- SessionStateSection.cs
- NamespaceListProperty.cs
- BinaryObjectInfo.cs
- TextBox.cs
- ResourceCodeDomSerializer.cs
- DrawListViewColumnHeaderEventArgs.cs
- BaseParser.cs
- ResolveMatches11.cs
- SvcFileManager.cs
- ThreadExceptionEvent.cs
- ProviderCommandInfoUtils.cs
- OleDbReferenceCollection.cs
- AddInController.cs
- XmlDataSourceNodeDescriptor.cs
- XmlWhitespace.cs
- XmlSchemaResource.cs
- TypeFieldSchema.cs
- HelpEvent.cs
- _NtlmClient.cs
- InputBuffer.cs
- DataGridViewColumnCollectionDialog.cs
- XPathNavigator.cs
- ContentDisposition.cs
- BindableAttribute.cs
- SqlClientMetaDataCollectionNames.cs
- ToolStripStatusLabel.cs
- ApplicationBuildProvider.cs
- WindowsRichEdit.cs
- TypedElement.cs
- SafeEventLogWriteHandle.cs
- FormViewUpdateEventArgs.cs
- Label.cs
- RoutedPropertyChangedEventArgs.cs
- GroupBoxAutomationPeer.cs
- CoordinationService.cs
- _OSSOCK.cs
- UdpDuplexChannel.cs
- DateTimeFormatInfo.cs
- TimeoutException.cs
- Deserializer.cs
- XmlSchema.cs
- XhtmlBasicCalendarAdapter.cs
- ListBoxDesigner.cs
- ResumeStoryboard.cs
- DataGridTextColumn.cs
- HttpHandlerActionCollection.cs
- RTTypeWrapper.cs
- DataGridRowClipboardEventArgs.cs
- processwaithandle.cs
- ClientSession.cs
- Gdiplus.cs
- SqlFunctionAttribute.cs
- EventPrivateKey.cs
- TemplateBaseAction.cs
- SkinBuilder.cs
- ArgumentReference.cs
- InvalidAsynchronousStateException.cs
- TextEndOfParagraph.cs
- OutOfProcStateClientManager.cs
- VScrollBar.cs
- MulticastOption.cs
- CodeChecksumPragma.cs
- DbConnectionPoolIdentity.cs
- altserialization.cs
- ImageFormat.cs
- DesigntimeLicenseContextSerializer.cs
- IssuanceLicense.cs
- Attributes.cs
- Style.cs
- SecurityHelper.cs
- ClrPerspective.cs
- ScaleTransform3D.cs
- StyleCollection.cs
- DataControlFieldCell.cs
- GridViewCommandEventArgs.cs
- Trigger.cs
- TextBlockAutomationPeer.cs
- DataReaderContainer.cs
- ProbeRequestResponseAsyncResult.cs
- CompilationUnit.cs
- XamlReaderHelper.cs
- RuntimeEnvironment.cs
- IisTraceWebEventProvider.cs
- HighlightComponent.cs
- Expression.cs