Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Core / CSharp / System / Windows / Input / MouseButtonEventArgs.cs / 1 / MouseButtonEventArgs.cs
using System; namespace System.Windows.Input { ////// The MouseButtonEventArgs describes the state of a Mouse button. /// public class MouseButtonEventArgs : MouseEventArgs { ////// Initializes a new instance of the MouseButtonEventArgs class. /// /// /// The logical Mouse device associated with this event. /// /// /// The time when the input occured. /// /// /// The mouse button whose state is being described. /// public MouseButtonEventArgs(MouseDevice mouse, int timestamp, MouseButton button) : base(mouse, timestamp) { MouseButtonUtilities.Validate(button); _button = button; _count = 1; } ////// Initializes a new instance of the MouseButtonEventArgs class. /// /// /// The logical Mouse device associated with this event. /// /// /// The time when the input occured. /// /// /// The Mouse button whose state is being described. /// /// /// The stylus device that was involved with this event. /// public MouseButtonEventArgs(MouseDevice mouse, int timestamp, MouseButton button, StylusDevice stylusDevice) : base(mouse, timestamp, stylusDevice) { MouseButtonUtilities.Validate(button); _button = button; _count = 1; } ////// Read-only access to the button being described. /// public MouseButton ChangedButton { get {return _button;} } ////// Read-only access to the button state. /// public MouseButtonState ButtonState { get { MouseButtonState state = MouseButtonState.Released; switch(_button) { case MouseButton.Left: state = this.MouseDevice.LeftButton; break; case MouseButton.Right: state = this.MouseDevice.RightButton; break; case MouseButton.Middle: state = this.MouseDevice.MiddleButton; break; case MouseButton.XButton1: state = this.MouseDevice.XButton1; break; case MouseButton.XButton2: state = this.MouseDevice.XButton2; break; } return state; } } ////// Read access to the button click count. /// public int ClickCount { get {return _count;} internal set { _count = value;} } ////// The mechanism used to call the type-specific handler on the /// target. /// /// /// The generic handler to call in a type-specific way. /// /// /// The target to call the handler on. /// protected override void InvokeEventHandler(Delegate genericHandler, object genericTarget) { MouseButtonEventHandler handler = (MouseButtonEventHandler) genericHandler; handler(genericTarget, this); } private MouseButton _button; private int _count; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System; namespace System.Windows.Input { ////// The MouseButtonEventArgs describes the state of a Mouse button. /// public class MouseButtonEventArgs : MouseEventArgs { ////// Initializes a new instance of the MouseButtonEventArgs class. /// /// /// The logical Mouse device associated with this event. /// /// /// The time when the input occured. /// /// /// The mouse button whose state is being described. /// public MouseButtonEventArgs(MouseDevice mouse, int timestamp, MouseButton button) : base(mouse, timestamp) { MouseButtonUtilities.Validate(button); _button = button; _count = 1; } ////// Initializes a new instance of the MouseButtonEventArgs class. /// /// /// The logical Mouse device associated with this event. /// /// /// The time when the input occured. /// /// /// The Mouse button whose state is being described. /// /// /// The stylus device that was involved with this event. /// public MouseButtonEventArgs(MouseDevice mouse, int timestamp, MouseButton button, StylusDevice stylusDevice) : base(mouse, timestamp, stylusDevice) { MouseButtonUtilities.Validate(button); _button = button; _count = 1; } ////// Read-only access to the button being described. /// public MouseButton ChangedButton { get {return _button;} } ////// Read-only access to the button state. /// public MouseButtonState ButtonState { get { MouseButtonState state = MouseButtonState.Released; switch(_button) { case MouseButton.Left: state = this.MouseDevice.LeftButton; break; case MouseButton.Right: state = this.MouseDevice.RightButton; break; case MouseButton.Middle: state = this.MouseDevice.MiddleButton; break; case MouseButton.XButton1: state = this.MouseDevice.XButton1; break; case MouseButton.XButton2: state = this.MouseDevice.XButton2; break; } return state; } } ////// Read access to the button click count. /// public int ClickCount { get {return _count;} internal set { _count = value;} } ////// The mechanism used to call the type-specific handler on the /// target. /// /// /// The generic handler to call in a type-specific way. /// /// /// The target to call the handler on. /// protected override void InvokeEventHandler(Delegate genericHandler, object genericTarget) { MouseButtonEventHandler handler = (MouseButtonEventHandler) genericHandler; handler(genericTarget, this); } private MouseButton _button; private int _count; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
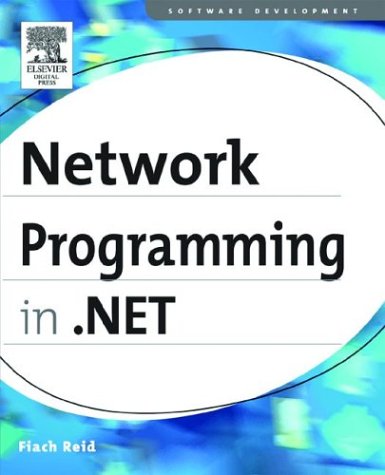
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MetadataSource.cs
- SqlCommand.cs
- EntityRecordInfo.cs
- ManagementNamedValueCollection.cs
- DefaultMemberAttribute.cs
- BitStack.cs
- SchemaManager.cs
- ThicknessAnimationUsingKeyFrames.cs
- NetSectionGroup.cs
- InputLanguageSource.cs
- EncryptedKey.cs
- XmlSignatureManifest.cs
- MarshalByValueComponent.cs
- BlockCollection.cs
- XpsDigitalSignature.cs
- SafeThemeHandle.cs
- SingleStorage.cs
- ProviderBase.cs
- MultipleViewProviderWrapper.cs
- ZipIOZip64EndOfCentralDirectoryBlock.cs
- TypeListConverter.cs
- PeerNameRecord.cs
- RawTextInputReport.cs
- ByteAnimationUsingKeyFrames.cs
- QilInvokeEarlyBound.cs
- XmlSchemaElement.cs
- XmlAttributeProperties.cs
- StringValueSerializer.cs
- BamlResourceDeserializer.cs
- SqlDataSource.cs
- EnumUnknown.cs
- InputMethod.cs
- DetailsViewPagerRow.cs
- IOThreadTimer.cs
- InputLanguageSource.cs
- CredentialCache.cs
- WebBrowserContainer.cs
- WindowInteractionStateTracker.cs
- Parameter.cs
- WindowCollection.cs
- SqlHelper.cs
- EventPropertyMap.cs
- BaseDataBoundControl.cs
- TagNameToTypeMapper.cs
- EnumerableCollectionView.cs
- AtomServiceDocumentSerializer.cs
- GridPatternIdentifiers.cs
- InlinedAggregationOperator.cs
- ImportContext.cs
- AutoResizedEvent.cs
- CheckBox.cs
- DirectionalLight.cs
- ToolStripProgressBar.cs
- SoapSchemaExporter.cs
- RIPEMD160Managed.cs
- Setter.cs
- QilValidationVisitor.cs
- BuildProviderInstallComponent.cs
- ISAPIRuntime.cs
- WebPartZoneDesigner.cs
- ActiveXMessageFormatter.cs
- versioninfo.cs
- EventSource.cs
- ScrollProperties.cs
- DataKeyArray.cs
- Pool.cs
- FileUtil.cs
- FrameworkContentElement.cs
- EventLogPermissionHolder.cs
- NamedObjectList.cs
- BitVector32.cs
- DriveInfo.cs
- PropertyItemInternal.cs
- Empty.cs
- NativeMethodsCLR.cs
- _SslState.cs
- SQLResource.cs
- CreateUserWizardStep.cs
- HttpRequest.cs
- PackWebRequest.cs
- AssemblyInfo.cs
- ChineseLunisolarCalendar.cs
- ClientUtils.cs
- Line.cs
- ToolBarOverflowPanel.cs
- ResXResourceReader.cs
- DocumentPageTextView.cs
- Point.cs
- GridViewRowPresenter.cs
- TableLayoutStyle.cs
- ValuePatternIdentifiers.cs
- ExplicitDiscriminatorMap.cs
- TableAdapterManagerHelper.cs
- _ServiceNameStore.cs
- IChannel.cs
- SymLanguageVendor.cs
- CFStream.cs
- SecurityElement.cs
- CompositeFontParser.cs
- ParallelQuery.cs