Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Wmi / managed / System / Management / ManagementNamedValueCollection.cs / 1305376 / ManagementNamedValueCollection.cs
using System; using System.Collections; using System.Collections.Specialized; using WbemClient_v1; using System.Runtime.Serialization; namespace System.Management { //CCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCC// ////// //CCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCC// public class ManagementNamedValueCollection : NameObjectCollectionBase { // Notification of when the content of this collection changes internal event IdentifierChangedEventHandler IdentifierChanged; //Fires IdentifierChanged event private void FireIdentifierChanged() { if (IdentifierChanged != null) IdentifierChanged(this, null); } //default constructor ///Represents a collection of named values /// suitable for use as context information to WMI operations. The /// names are case-insensitive. ////// Initializes a new instance /// of the ///class. /// /// public ManagementNamedValueCollection() { } ///Initializes a new instance of the ///class, which is empty. This is /// the default constructor. /// /// TheInitializes a new instance of the ///class that is serializable /// and uses the specified /// and . to populate with data. /// The destination (see ) for this serialization. protected ManagementNamedValueCollection(SerializationInfo info, StreamingContext context) : base(info, context) { } /// /// internal IWbemContext GetContext() { IWbemContext wbemContext = null; // Only build a context if we have something to put in it if (0 < Count) { int status = (int)ManagementStatus.NoError; try { wbemContext = (IWbemContext) new WbemContext (); foreach (string name in this) { object val = base.BaseGet(name); status = wbemContext.SetValue_ (name, 0, ref val); if ((status & 0x80000000) != 0) { break; } } } catch {} // } return wbemContext; } ///Internal method to return an IWbemContext representation /// of the named value collection. ////// /// The name of the new value. /// The value to be associated with the name. public void Add (string name, object value) { // Remove any old entry try { base.BaseRemove (name); } catch {} base.BaseAdd (name, value); FireIdentifierChanged (); } ///Adds a single-named value to the collection. ////// /// The name of the value to be removed. public void Remove (string name) { base.BaseRemove (name); FireIdentifierChanged (); } ///Removes a single-named value from the collection. /// If the collection does not contain an element with the /// specified name, the collection remains unchanged and no /// exception is thrown. ////// public void RemoveAll () { base.BaseClear (); FireIdentifierChanged (); } ///Removes all entries from the collection. ////// ///Creates a clone of the collection. Individual values /// are cloned. If a value does not support cloning, then a ////// is thrown. /// The new copy of the collection. /// public ManagementNamedValueCollection Clone () { ManagementNamedValueCollection nvc = new ManagementNamedValueCollection(); foreach (string name in this) { // If we can clone the value, do so. Otherwise throw. object val = base.BaseGet (name); if (null != val) { Type valueType = val.GetType (); if (valueType.IsByRef) { try { object clonedValue = ((ICloneable)val).Clone (); nvc.Add (name, clonedValue); } catch { throw new NotSupportedException (); } } else { nvc.Add (name, val); } } else nvc.Add (name, null); } return nvc; } ////// /// The name of the value to be returned. ///Returns the value associated with the specified name from this collection. ////// public object this[string name] { get { return base.BaseGet(name); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System; using System.Collections; using System.Collections.Specialized; using WbemClient_v1; using System.Runtime.Serialization; namespace System.Management { //CCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCC// ///An ///containing the /// value of the specified item in this collection. /// //CCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCC// public class ManagementNamedValueCollection : NameObjectCollectionBase { // Notification of when the content of this collection changes internal event IdentifierChangedEventHandler IdentifierChanged; //Fires IdentifierChanged event private void FireIdentifierChanged() { if (IdentifierChanged != null) IdentifierChanged(this, null); } //default constructor ///Represents a collection of named values /// suitable for use as context information to WMI operations. The /// names are case-insensitive. ////// Initializes a new instance /// of the ///class. /// /// public ManagementNamedValueCollection() { } ///Initializes a new instance of the ///class, which is empty. This is /// the default constructor. /// /// TheInitializes a new instance of the ///class that is serializable /// and uses the specified /// and . to populate with data. /// The destination (see ) for this serialization. protected ManagementNamedValueCollection(SerializationInfo info, StreamingContext context) : base(info, context) { } /// /// internal IWbemContext GetContext() { IWbemContext wbemContext = null; // Only build a context if we have something to put in it if (0 < Count) { int status = (int)ManagementStatus.NoError; try { wbemContext = (IWbemContext) new WbemContext (); foreach (string name in this) { object val = base.BaseGet(name); status = wbemContext.SetValue_ (name, 0, ref val); if ((status & 0x80000000) != 0) { break; } } } catch {} // } return wbemContext; } ///Internal method to return an IWbemContext representation /// of the named value collection. ////// /// The name of the new value. /// The value to be associated with the name. public void Add (string name, object value) { // Remove any old entry try { base.BaseRemove (name); } catch {} base.BaseAdd (name, value); FireIdentifierChanged (); } ///Adds a single-named value to the collection. ////// /// The name of the value to be removed. public void Remove (string name) { base.BaseRemove (name); FireIdentifierChanged (); } ///Removes a single-named value from the collection. /// If the collection does not contain an element with the /// specified name, the collection remains unchanged and no /// exception is thrown. ////// public void RemoveAll () { base.BaseClear (); FireIdentifierChanged (); } ///Removes all entries from the collection. ////// ///Creates a clone of the collection. Individual values /// are cloned. If a value does not support cloning, then a ////// is thrown. /// The new copy of the collection. /// public ManagementNamedValueCollection Clone () { ManagementNamedValueCollection nvc = new ManagementNamedValueCollection(); foreach (string name in this) { // If we can clone the value, do so. Otherwise throw. object val = base.BaseGet (name); if (null != val) { Type valueType = val.GetType (); if (valueType.IsByRef) { try { object clonedValue = ((ICloneable)val).Clone (); nvc.Add (name, clonedValue); } catch { throw new NotSupportedException (); } } else { nvc.Add (name, val); } } else nvc.Add (name, null); } return nvc; } ////// /// The name of the value to be returned. ///Returns the value associated with the specified name from this collection. ////// public object this[string name] { get { return base.BaseGet(name); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.An ///containing the /// value of the specified item in this collection.
Link Menu
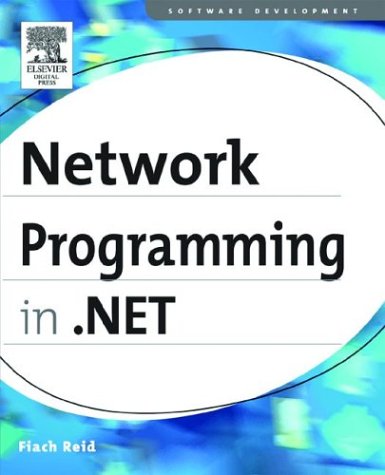
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PreviewPrintController.cs
- HostingEnvironment.cs
- RequestFactory.cs
- InputElement.cs
- GeneralTransform3DTo2D.cs
- ProviderConnectionPointCollection.cs
- RoleGroup.cs
- ChangeNode.cs
- TagNameToTypeMapper.cs
- precedingquery.cs
- StackOverflowException.cs
- DataSourceControl.cs
- InfiniteTimeSpanConverter.cs
- PartManifestEntry.cs
- DLinqColumnProvider.cs
- NamespaceInfo.cs
- PrintPreviewGraphics.cs
- SecurityUtils.cs
- MenuItemAutomationPeer.cs
- Column.cs
- DataGridViewAutoSizeColumnsModeEventArgs.cs
- CustomAssemblyResolver.cs
- ListViewSortEventArgs.cs
- ProxyWebPartConnectionCollection.cs
- ArgumentDesigner.xaml.cs
- CrossSiteScriptingValidation.cs
- ClientConfigPaths.cs
- ThreadExceptionDialog.cs
- CalendarTable.cs
- CallbackException.cs
- DesignerContextDescriptor.cs
- ProxyWebPart.cs
- AutoCompleteStringCollection.cs
- WpfWebRequestHelper.cs
- StrokeSerializer.cs
- SystemUnicastIPAddressInformation.cs
- ExpressionNode.cs
- XmlExpressionDumper.cs
- CrossAppDomainChannel.cs
- SplayTreeNode.cs
- SqlUdtInfo.cs
- ComNativeDescriptor.cs
- FixedSOMLineCollection.cs
- StatusBarItemAutomationPeer.cs
- ConfigurationLocation.cs
- TextWriter.cs
- RootBrowserWindowProxy.cs
- ChangeBlockUndoRecord.cs
- FormsAuthenticationUserCollection.cs
- TextBounds.cs
- Error.cs
- MenuItem.cs
- FileSecurity.cs
- ValueUtilsSmi.cs
- CollaborationHelperFunctions.cs
- DomNameTable.cs
- _ListenerRequestStream.cs
- TextParentUndoUnit.cs
- MultipleViewPattern.cs
- BaseParser.cs
- MulticastIPAddressInformationCollection.cs
- AttributeEmitter.cs
- SQLInt64.cs
- PrintEvent.cs
- SchemaNotation.cs
- FormViewActionList.cs
- CodeGenerator.cs
- XmlSchemaExternal.cs
- LayoutTable.cs
- VectorCollectionConverter.cs
- DirectoryNotFoundException.cs
- PrtTicket_Editor.cs
- SettingsBase.cs
- HwndSource.cs
- ServiceNotStartedException.cs
- AutoGeneratedFieldProperties.cs
- SqlFacetAttribute.cs
- RedirectionProxy.cs
- ReaderWriterLockWrapper.cs
- PenContext.cs
- CodePropertyReferenceExpression.cs
- RolePrincipal.cs
- EarlyBoundInfo.cs
- IISMapPath.cs
- SoapFormatExtensions.cs
- EditCommandColumn.cs
- ObjectViewListener.cs
- Msmq4SubqueuePoisonHandler.cs
- DataRelationCollection.cs
- XPathNodeHelper.cs
- SelectionEditor.cs
- QuotedPrintableStream.cs
- CompatibleIComparer.cs
- HwndHost.cs
- OrderingQueryOperator.cs
- CachedFontFace.cs
- DataMember.cs
- FilteredReadOnlyMetadataCollection.cs
- Terminate.cs
- VectorAnimationBase.cs