Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Framework / System / Windows / MultiDataTrigger.cs / 1 / MultiDataTrigger.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: Defines MultiDataTrigger object, akin to MultiTrigger except it // gets values from data. // //--------------------------------------------------------------------------- using MS.Utility; using System; using System.Diagnostics; using System.Collections.Specialized; using System.ComponentModel; using System.Windows.Data; using System.Windows.Markup; namespace System.Windows { ////// A multiple Style data conditional dependency driver /// [ContentProperty("Setters")] public sealed class MultiDataTrigger : TriggerBase, IAddChild { ////// Conditions collection /// [DesignerSerializationVisibility(DesignerSerializationVisibility.Content)] public ConditionCollection Conditions { get { // Verify Context Access VerifyAccess(); return _conditions; } } ////// Collection of Setter objects, which describes what to apply /// when this trigger is active. /// [DesignerSerializationVisibility(DesignerSerializationVisibility.Content)] public SetterBaseCollection Setters { get { // Verify Context Access VerifyAccess(); if( _setters == null ) { _setters = new SetterBaseCollection(); } return _setters; } } ////// This method is called to Add a Setter object as a child of the Style. /// /// /// The object to add as a child; it must be a Setter or subclass. /// void IAddChild.AddChild (Object value) { // Verify Context Access VerifyAccess(); Setters.Add(Trigger.CheckChildIsSetter(value)); } ////// This method is called by the parser when text appears under the tag in markup. /// As default Styles do not support text, calling this method has no effect. /// /// /// Text to add as a child. /// void IAddChild.AddText (string text) { // Verify Context Access VerifyAccess(); XamlSerializerUtil.ThrowIfNonWhiteSpaceInAddText(text, this); } internal override void Seal() { if (IsSealed) { return; } // Process the _setters collection: Copy values into PropertyValueList and seal the Setter objects. ProcessSettersCollection(_setters); if (_conditions.Count > 0) { // Seal conditions _conditions.Seal(ValueLookupType.DataTrigger); } // Build conditions array from collection TriggerConditions = new TriggerCondition[_conditions.Count]; for (int i = 0; i < TriggerConditions.Length; ++i) { if (_conditions[i].SourceName != null && _conditions[i].SourceName.Length > 0) { throw new InvalidOperationException(SR.Get(SRID.SourceNameNotSupportedForDataTriggers)); } TriggerConditions[i] = new TriggerCondition( _conditions[i].Binding, LogicalOp.Equals, _conditions[i].Value); } // Set conditions array for all property triggers for (int i = 0; i < PropertyValues.Count; ++i) { PropertyValue propertyValue = PropertyValues[i]; propertyValue.Conditions = TriggerConditions; switch (propertyValue.ValueType) { case PropertyValueType.Trigger: propertyValue.ValueType = PropertyValueType.DataTrigger; break; case PropertyValueType.PropertyTriggerResource: propertyValue.ValueType = PropertyValueType.DataTriggerResource; break; default: throw new InvalidOperationException(SR.Get(SRID.UnexpectedValueTypeForDataTrigger, propertyValue.ValueType)); } // Put back modified struct PropertyValues[i] = propertyValue; } base.Seal(); } // evaluate the current state of the trigger internal override bool GetCurrentState(DependencyObject container, UncommonFielddataField) { bool retVal = (TriggerConditions.Length > 0); for( int i = 0; retVal && i < TriggerConditions.Length; i++ ) { retVal = TriggerConditions[i].ConvertAndMatch(StyleHelper.GetDataTriggerValue(dataField, container, TriggerConditions[i].Binding)); } return retVal; } private ConditionCollection _conditions = new ConditionCollection(); private SetterBaseCollection _setters = null; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // // Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: Defines MultiDataTrigger object, akin to MultiTrigger except it // gets values from data. // //--------------------------------------------------------------------------- using MS.Utility; using System; using System.Diagnostics; using System.Collections.Specialized; using System.ComponentModel; using System.Windows.Data; using System.Windows.Markup; namespace System.Windows { ////// A multiple Style data conditional dependency driver /// [ContentProperty("Setters")] public sealed class MultiDataTrigger : TriggerBase, IAddChild { ////// Conditions collection /// [DesignerSerializationVisibility(DesignerSerializationVisibility.Content)] public ConditionCollection Conditions { get { // Verify Context Access VerifyAccess(); return _conditions; } } ////// Collection of Setter objects, which describes what to apply /// when this trigger is active. /// [DesignerSerializationVisibility(DesignerSerializationVisibility.Content)] public SetterBaseCollection Setters { get { // Verify Context Access VerifyAccess(); if( _setters == null ) { _setters = new SetterBaseCollection(); } return _setters; } } ////// This method is called to Add a Setter object as a child of the Style. /// /// /// The object to add as a child; it must be a Setter or subclass. /// void IAddChild.AddChild (Object value) { // Verify Context Access VerifyAccess(); Setters.Add(Trigger.CheckChildIsSetter(value)); } ////// This method is called by the parser when text appears under the tag in markup. /// As default Styles do not support text, calling this method has no effect. /// /// /// Text to add as a child. /// void IAddChild.AddText (string text) { // Verify Context Access VerifyAccess(); XamlSerializerUtil.ThrowIfNonWhiteSpaceInAddText(text, this); } internal override void Seal() { if (IsSealed) { return; } // Process the _setters collection: Copy values into PropertyValueList and seal the Setter objects. ProcessSettersCollection(_setters); if (_conditions.Count > 0) { // Seal conditions _conditions.Seal(ValueLookupType.DataTrigger); } // Build conditions array from collection TriggerConditions = new TriggerCondition[_conditions.Count]; for (int i = 0; i < TriggerConditions.Length; ++i) { if (_conditions[i].SourceName != null && _conditions[i].SourceName.Length > 0) { throw new InvalidOperationException(SR.Get(SRID.SourceNameNotSupportedForDataTriggers)); } TriggerConditions[i] = new TriggerCondition( _conditions[i].Binding, LogicalOp.Equals, _conditions[i].Value); } // Set conditions array for all property triggers for (int i = 0; i < PropertyValues.Count; ++i) { PropertyValue propertyValue = PropertyValues[i]; propertyValue.Conditions = TriggerConditions; switch (propertyValue.ValueType) { case PropertyValueType.Trigger: propertyValue.ValueType = PropertyValueType.DataTrigger; break; case PropertyValueType.PropertyTriggerResource: propertyValue.ValueType = PropertyValueType.DataTriggerResource; break; default: throw new InvalidOperationException(SR.Get(SRID.UnexpectedValueTypeForDataTrigger, propertyValue.ValueType)); } // Put back modified struct PropertyValues[i] = propertyValue; } base.Seal(); } // evaluate the current state of the trigger internal override bool GetCurrentState(DependencyObject container, UncommonFielddataField) { bool retVal = (TriggerConditions.Length > 0); for( int i = 0; retVal && i < TriggerConditions.Length; i++ ) { retVal = TriggerConditions[i].ConvertAndMatch(StyleHelper.GetDataTriggerValue(dataField, container, TriggerConditions[i].Binding)); } return retVal; } private ConditionCollection _conditions = new ConditionCollection(); private SetterBaseCollection _setters = null; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
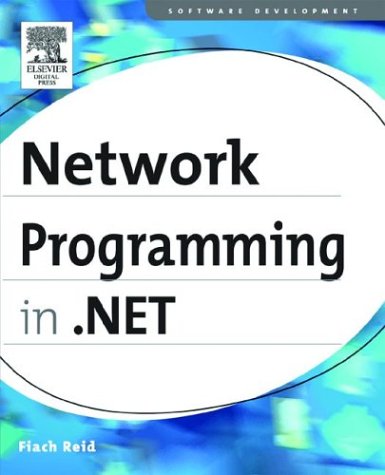
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Config.cs
- FontCollection.cs
- JournalEntry.cs
- WriteTimeStream.cs
- WebPartCatalogCloseVerb.cs
- DbConnectionPoolGroupProviderInfo.cs
- ScopelessEnumAttribute.cs
- WorkflowExecutor.cs
- Trigger.cs
- IBuiltInEvidence.cs
- HtmlToClrEventProxy.cs
- Win32SafeHandles.cs
- StubHelpers.cs
- GridViewDeletedEventArgs.cs
- FixedStringLookup.cs
- TextEditorDragDrop.cs
- XmlSchemaSimpleTypeUnion.cs
- StateMachine.cs
- SplineQuaternionKeyFrame.cs
- BrowserTree.cs
- Baml2006KnownTypes.cs
- SQLMembershipProvider.cs
- DbCommandDefinition.cs
- KeyManager.cs
- SafeEventHandle.cs
- EntityDataSourceSelectedEventArgs.cs
- DetailsViewActionList.cs
- dsa.cs
- CacheOutputQuery.cs
- TargetFrameworkUtil.cs
- COM2ColorConverter.cs
- OleDbInfoMessageEvent.cs
- XmlSchemaAnnotated.cs
- ComponentDispatcherThread.cs
- ReaderContextStackData.cs
- SignatureResourcePool.cs
- SamlAssertionKeyIdentifierClause.cs
- NavigatorInvalidBodyAccessException.cs
- QuaternionConverter.cs
- ImageList.cs
- querybuilder.cs
- sitestring.cs
- MetadataAssemblyHelper.cs
- CodeSnippetCompileUnit.cs
- RouteValueExpressionBuilder.cs
- isolationinterop.cs
- MaterialGroup.cs
- OdbcEnvironmentHandle.cs
- XmlAnyAttributeAttribute.cs
- ControlParser.cs
- SynchronizationLockException.cs
- EntityContainerRelationshipSetEnd.cs
- SmiContext.cs
- DataProviderNameConverter.cs
- CompilationUnit.cs
- ServiceSecurityAuditElement.cs
- GZipUtils.cs
- XomlCompilerError.cs
- SafeNativeMethods.cs
- COM2EnumConverter.cs
- _BufferOffsetSize.cs
- ScrollContentPresenter.cs
- RecognizedWordUnit.cs
- ZipIOCentralDirectoryDigitalSignature.cs
- FocusChangedEventArgs.cs
- TextureBrush.cs
- odbcmetadatacolumnnames.cs
- CollectionViewGroup.cs
- SQLDecimal.cs
- FormatConvertedBitmap.cs
- XPathNodePointer.cs
- DecimalStorage.cs
- SqlUserDefinedTypeAttribute.cs
- ConstraintCollection.cs
- typedescriptorpermissionattribute.cs
- XmlAttribute.cs
- ReaderOutput.cs
- unsafenativemethodsother.cs
- BooleanFunctions.cs
- UpdatePanel.cs
- FacetChecker.cs
- TextSerializer.cs
- cookieexception.cs
- FixedTextBuilder.cs
- ArraySet.cs
- Oid.cs
- WebSysDescriptionAttribute.cs
- UIElementHelper.cs
- WebPartUserCapability.cs
- ImageBrush.cs
- HttpHandlerAction.cs
- RectAnimationClockResource.cs
- ProtectedConfigurationProviderCollection.cs
- JsonSerializer.cs
- HttpResponseHeader.cs
- CodeCatchClause.cs
- ToolStripSeparatorRenderEventArgs.cs
- SymLanguageType.cs
- TextCompositionManager.cs
- SeekStoryboard.cs