Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / WinForms / Managed / System / Resources / ResXFileRef.cs / 1 / ResXFileRef.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- #if SYSTEM_WEB // See DevDiv 9030 namespace System.PrivateResources { #else namespace System.Resources { #endif using System.Diagnostics; using System.Diagnostics.CodeAnalysis; using System; using System.Windows.Forms; using System.Reflection; using Microsoft.Win32; using System.Drawing; using System.IO; using System.ComponentModel; using System.Collections; using System.Resources; using System.Text; using System.Globalization; using System.Runtime.Serialization; ////// /// ResX File Reference class. This allows the developer to represent /// a link to an external resource. When the resource manager asks /// for the value of the resource item, the external resource is loaded. /// [TypeConverterAttribute(typeof(ResXFileRef.Converter)), Serializable] [System.Security.Permissions.PermissionSetAttribute(System.Security.Permissions.SecurityAction.InheritanceDemand, Name="FullTrust")] [System.Security.Permissions.PermissionSetAttribute(System.Security.Permissions.SecurityAction.LinkDemand, Name="FullTrust")] #if SYSTEM_WEB internal class ResXFileRef { #else public class ResXFileRef { #endif private string fileName; private string typeName; [OptionalField(VersionAdded = 2)] private Encoding textFileEncoding; ////// /// Creates a new ResXFileRef that points to the specified file. /// The type refered to by typeName must support a constructor /// that accepts a System.IO.Stream as a parameter. /// public ResXFileRef(string fileName, string typeName) { if(fileName == null) { throw (new ArgumentNullException("fileName")); } if(typeName == null) { throw (new ArgumentNullException("typeName")); } this.fileName = fileName; this.typeName = typeName; } [OnDeserializing] private void OnDeserializing(StreamingContext ctx) { textFileEncoding = null; } [SuppressMessage("Microsoft.Performance", "CA1822:MarkMethodsAsStatic")] [OnDeserialized] private void OnDeserialized(StreamingContext ctx) { } ////// /// Creates a new ResXFileRef that points to the specified file. /// The type refered to by typeName must support a constructor /// that accepts a System.IO.Stream as a parameter. /// public ResXFileRef(string fileName, string typeName, Encoding textFileEncoding) : this(fileName, typeName) { this.textFileEncoding = textFileEncoding; } internal ResXFileRef Clone() { return new ResXFileRef(fileName, typeName, textFileEncoding); } ////// /// public string FileName { get { return fileName; } } ///[To be supplied.] ////// /// public string TypeName { get { return typeName; } } ///[To be supplied.] ////// /// public Encoding TextFileEncoding { get { return textFileEncoding; } } ///[To be supplied.] ////// /// path1+result = path2 /// A string which is the relative path difference between path1 and /// path2 such that if path1 and the calculated difference are used /// as arguments to Combine(), path2 is returned /// private static string PathDifference(string path1, string path2, bool compareCase) { int i; int si = -1; for (i = 0; (i < path1.Length) && (i < path2.Length); ++i) { if ((path1[i] != path2[i]) && (compareCase || (Char.ToLower(path1[i], CultureInfo.InvariantCulture) != Char.ToLower(path2[i], CultureInfo.InvariantCulture)))) { break; } else if (path1[i] == Path.DirectorySeparatorChar) { si = i; } } if (i == 0) { return path2; } if ((i == path1.Length) && (i == path2.Length)) { return String.Empty; } StringBuilder relPath = new StringBuilder(); for (; i < path1.Length; ++i) { if (path1[i] == Path.DirectorySeparatorChar) { relPath.Append(".."+Path.DirectorySeparatorChar); } } return relPath.ToString() + path2.Substring(si + 1); } internal void MakeFilePathRelative(string basePath) { if(basePath==null || basePath.Length == 0) { return; } fileName = PathDifference(basePath, fileName, false); } ////// /// public override string ToString() { string result = ""; if(fileName.IndexOf(";") != -1 || fileName.IndexOf("\"") != -1) { result += ("\""+ fileName + "\";"); } else { result += (fileName + ";"); } result += typeName; if(textFileEncoding != null) { result += (";" + textFileEncoding.WebName); } return result; } ///[To be supplied.] ////// /// [System.Security.Permissions.PermissionSetAttribute(System.Security.Permissions.SecurityAction.InheritanceDemand, Name="FullTrust")] [System.Security.Permissions.PermissionSetAttribute(System.Security.Permissions.SecurityAction.LinkDemand, Name="FullTrust")] public class Converter : TypeConverter { ///[To be supplied.] ////// /// public override bool CanConvertFrom(ITypeDescriptorContext context, Type sourceType) { if (sourceType == typeof(string)) { return true; } return false; } ///[To be supplied.] ////// /// public override bool CanConvertTo(ITypeDescriptorContext context, Type destinationType) { if (destinationType == typeof(string)) { return true; } return false; } ///[To be supplied.] ////// /// public override Object ConvertTo(ITypeDescriptorContext context, CultureInfo culture, Object value, Type destinationType) { Object created = null; if (destinationType == typeof(string)) { created = ((ResXFileRef)value).ToString(); } return created; } // "value" is the parameter name of ConvertFrom, which calls this method. [SuppressMessage("Microsoft.Globalization", "CA1303:DoNotPassLiteralsAsLocalizedParameters")] internal static string[] ParseResxFileRefString(string stringValue) { string[] result = null; if(stringValue != null ) { stringValue = stringValue.Trim(); string fileName; string remainingString; if(stringValue.StartsWith("\"")) { int lastIndexOfQuote = stringValue.LastIndexOf("\""); if (lastIndexOfQuote-1<0) throw new ArgumentException("value"); fileName = stringValue.Substring(1, lastIndexOfQuote-1); // remove the quotes in" ..... " if(lastIndexOfQuote+2>stringValue.Length) throw new ArgumentException("value"); remainingString = stringValue.Substring(lastIndexOfQuote+2); } else { int nextSemiColumn = stringValue.IndexOf(";"); if(nextSemiColumn == -1) throw new ArgumentException("value"); fileName = stringValue.Substring(0,nextSemiColumn); if(nextSemiColumn+1>stringValue.Length) throw new ArgumentException("value"); remainingString = stringValue.Substring(nextSemiColumn+1); } string[] parts = remainingString.Split(new char[] {';'}); if(parts.Length > 1) { result = new string[] { fileName, parts[0], parts[1] }; } else if(parts.Length > 0) { result = new string[] { fileName, parts[0] }; } else { result = new string[] { fileName }; } } return result; } ///[To be supplied.] ////// /// public override Object ConvertFrom(ITypeDescriptorContext context, CultureInfo culture, Object value) { Object created = null; string stringValue = value as string; if (stringValue != null) { string[] parts = ResXFileRef.Converter.ParseResxFileRefString(stringValue); string fileName = parts[0]; Type toCreate = Type.GetType(parts[1], true); // special case string and byte[] if(toCreate.Equals(typeof(string))) { // we have a string, now we need to check the encoding Encoding textFileEncoding = Encoding.Default; if(parts.Length > 2) { textFileEncoding = Encoding.GetEncoding(parts[2]); } using (StreamReader sr = new StreamReader(fileName, textFileEncoding)) { created = sr.ReadToEnd(); } } else { // this is a regular file, we call it's constructor with a stream as a parameter // or if it's a byte array we just return that byte[] temp = null; using (FileStream s = new FileStream(fileName, FileMode.Open, FileAccess.Read, FileShare.Read)) { Debug.Assert(s != null, "Couldn't open " + fileName); temp = new byte[s.Length]; s.Read(temp, 0, (int)s.Length); } if(toCreate.Equals(typeof(byte[]))) { created = temp; } else { MemoryStream memStream = new MemoryStream(temp); if(toCreate.Equals(typeof(MemoryStream))) { return memStream; } else if(toCreate.Equals(typeof(System.Drawing.Bitmap)) && fileName.EndsWith(".ico")) { // we special case the .ico bitmaps because GDI+ destroy the alpha channel component and // we don't want that to happen Icon ico = new Icon(memStream); created = ico.ToBitmap(); } else { created = Activator.CreateInstance(toCreate, BindingFlags.Instance | BindingFlags.Public | BindingFlags.CreateInstance, null, new Object[] {memStream}, null); } } } } return created; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //[To be supplied.] ///// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- #if SYSTEM_WEB // See DevDiv 9030 namespace System.PrivateResources { #else namespace System.Resources { #endif using System.Diagnostics; using System.Diagnostics.CodeAnalysis; using System; using System.Windows.Forms; using System.Reflection; using Microsoft.Win32; using System.Drawing; using System.IO; using System.ComponentModel; using System.Collections; using System.Resources; using System.Text; using System.Globalization; using System.Runtime.Serialization; ////// /// ResX File Reference class. This allows the developer to represent /// a link to an external resource. When the resource manager asks /// for the value of the resource item, the external resource is loaded. /// [TypeConverterAttribute(typeof(ResXFileRef.Converter)), Serializable] [System.Security.Permissions.PermissionSetAttribute(System.Security.Permissions.SecurityAction.InheritanceDemand, Name="FullTrust")] [System.Security.Permissions.PermissionSetAttribute(System.Security.Permissions.SecurityAction.LinkDemand, Name="FullTrust")] #if SYSTEM_WEB internal class ResXFileRef { #else public class ResXFileRef { #endif private string fileName; private string typeName; [OptionalField(VersionAdded = 2)] private Encoding textFileEncoding; ////// /// Creates a new ResXFileRef that points to the specified file. /// The type refered to by typeName must support a constructor /// that accepts a System.IO.Stream as a parameter. /// public ResXFileRef(string fileName, string typeName) { if(fileName == null) { throw (new ArgumentNullException("fileName")); } if(typeName == null) { throw (new ArgumentNullException("typeName")); } this.fileName = fileName; this.typeName = typeName; } [OnDeserializing] private void OnDeserializing(StreamingContext ctx) { textFileEncoding = null; } [SuppressMessage("Microsoft.Performance", "CA1822:MarkMethodsAsStatic")] [OnDeserialized] private void OnDeserialized(StreamingContext ctx) { } ////// /// Creates a new ResXFileRef that points to the specified file. /// The type refered to by typeName must support a constructor /// that accepts a System.IO.Stream as a parameter. /// public ResXFileRef(string fileName, string typeName, Encoding textFileEncoding) : this(fileName, typeName) { this.textFileEncoding = textFileEncoding; } internal ResXFileRef Clone() { return new ResXFileRef(fileName, typeName, textFileEncoding); } ////// /// public string FileName { get { return fileName; } } ///[To be supplied.] ////// /// public string TypeName { get { return typeName; } } ///[To be supplied.] ////// /// public Encoding TextFileEncoding { get { return textFileEncoding; } } ///[To be supplied.] ////// /// path1+result = path2 /// A string which is the relative path difference between path1 and /// path2 such that if path1 and the calculated difference are used /// as arguments to Combine(), path2 is returned /// private static string PathDifference(string path1, string path2, bool compareCase) { int i; int si = -1; for (i = 0; (i < path1.Length) && (i < path2.Length); ++i) { if ((path1[i] != path2[i]) && (compareCase || (Char.ToLower(path1[i], CultureInfo.InvariantCulture) != Char.ToLower(path2[i], CultureInfo.InvariantCulture)))) { break; } else if (path1[i] == Path.DirectorySeparatorChar) { si = i; } } if (i == 0) { return path2; } if ((i == path1.Length) && (i == path2.Length)) { return String.Empty; } StringBuilder relPath = new StringBuilder(); for (; i < path1.Length; ++i) { if (path1[i] == Path.DirectorySeparatorChar) { relPath.Append(".."+Path.DirectorySeparatorChar); } } return relPath.ToString() + path2.Substring(si + 1); } internal void MakeFilePathRelative(string basePath) { if(basePath==null || basePath.Length == 0) { return; } fileName = PathDifference(basePath, fileName, false); } ////// /// public override string ToString() { string result = ""; if(fileName.IndexOf(";") != -1 || fileName.IndexOf("\"") != -1) { result += ("\""+ fileName + "\";"); } else { result += (fileName + ";"); } result += typeName; if(textFileEncoding != null) { result += (";" + textFileEncoding.WebName); } return result; } ///[To be supplied.] ////// /// [System.Security.Permissions.PermissionSetAttribute(System.Security.Permissions.SecurityAction.InheritanceDemand, Name="FullTrust")] [System.Security.Permissions.PermissionSetAttribute(System.Security.Permissions.SecurityAction.LinkDemand, Name="FullTrust")] public class Converter : TypeConverter { ///[To be supplied.] ////// /// public override bool CanConvertFrom(ITypeDescriptorContext context, Type sourceType) { if (sourceType == typeof(string)) { return true; } return false; } ///[To be supplied.] ////// /// public override bool CanConvertTo(ITypeDescriptorContext context, Type destinationType) { if (destinationType == typeof(string)) { return true; } return false; } ///[To be supplied.] ////// /// public override Object ConvertTo(ITypeDescriptorContext context, CultureInfo culture, Object value, Type destinationType) { Object created = null; if (destinationType == typeof(string)) { created = ((ResXFileRef)value).ToString(); } return created; } // "value" is the parameter name of ConvertFrom, which calls this method. [SuppressMessage("Microsoft.Globalization", "CA1303:DoNotPassLiteralsAsLocalizedParameters")] internal static string[] ParseResxFileRefString(string stringValue) { string[] result = null; if(stringValue != null ) { stringValue = stringValue.Trim(); string fileName; string remainingString; if(stringValue.StartsWith("\"")) { int lastIndexOfQuote = stringValue.LastIndexOf("\""); if (lastIndexOfQuote-1<0) throw new ArgumentException("value"); fileName = stringValue.Substring(1, lastIndexOfQuote-1); // remove the quotes in" ..... " if(lastIndexOfQuote+2>stringValue.Length) throw new ArgumentException("value"); remainingString = stringValue.Substring(lastIndexOfQuote+2); } else { int nextSemiColumn = stringValue.IndexOf(";"); if(nextSemiColumn == -1) throw new ArgumentException("value"); fileName = stringValue.Substring(0,nextSemiColumn); if(nextSemiColumn+1>stringValue.Length) throw new ArgumentException("value"); remainingString = stringValue.Substring(nextSemiColumn+1); } string[] parts = remainingString.Split(new char[] {';'}); if(parts.Length > 1) { result = new string[] { fileName, parts[0], parts[1] }; } else if(parts.Length > 0) { result = new string[] { fileName, parts[0] }; } else { result = new string[] { fileName }; } } return result; } ///[To be supplied.] ////// /// public override Object ConvertFrom(ITypeDescriptorContext context, CultureInfo culture, Object value) { Object created = null; string stringValue = value as string; if (stringValue != null) { string[] parts = ResXFileRef.Converter.ParseResxFileRefString(stringValue); string fileName = parts[0]; Type toCreate = Type.GetType(parts[1], true); // special case string and byte[] if(toCreate.Equals(typeof(string))) { // we have a string, now we need to check the encoding Encoding textFileEncoding = Encoding.Default; if(parts.Length > 2) { textFileEncoding = Encoding.GetEncoding(parts[2]); } using (StreamReader sr = new StreamReader(fileName, textFileEncoding)) { created = sr.ReadToEnd(); } } else { // this is a regular file, we call it's constructor with a stream as a parameter // or if it's a byte array we just return that byte[] temp = null; using (FileStream s = new FileStream(fileName, FileMode.Open, FileAccess.Read, FileShare.Read)) { Debug.Assert(s != null, "Couldn't open " + fileName); temp = new byte[s.Length]; s.Read(temp, 0, (int)s.Length); } if(toCreate.Equals(typeof(byte[]))) { created = temp; } else { MemoryStream memStream = new MemoryStream(temp); if(toCreate.Equals(typeof(MemoryStream))) { return memStream; } else if(toCreate.Equals(typeof(System.Drawing.Bitmap)) && fileName.EndsWith(".ico")) { // we special case the .ico bitmaps because GDI+ destroy the alpha channel component and // we don't want that to happen Icon ico = new Icon(memStream); created = ico.ToBitmap(); } else { created = Activator.CreateInstance(toCreate, BindingFlags.Instance | BindingFlags.Public | BindingFlags.CreateInstance, null, new Object[] {memStream}, null); } } } } return created; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.[To be supplied.] ///
Link Menu
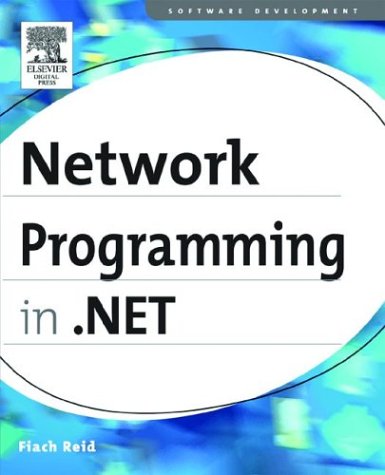
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SystemFonts.cs
- XmlComplianceUtil.cs
- FreezableDefaultValueFactory.cs
- RtfToXamlReader.cs
- Int32Converter.cs
- XmlDataSource.cs
- ExternalCalls.cs
- ExpressionBuilderContext.cs
- AsyncOperationContext.cs
- Blend.cs
- LoginUtil.cs
- DifferencingCollection.cs
- ObjectRef.cs
- _RequestCacheProtocol.cs
- DataServiceQueryOfT.cs
- GeometryCombineModeValidation.cs
- ProofTokenCryptoHandle.cs
- _OSSOCK.cs
- ComponentCache.cs
- DataSetUtil.cs
- Processor.cs
- SqlInternalConnectionTds.cs
- ObjectTag.cs
- Baml2006KeyRecord.cs
- QueryStringParameter.cs
- DeploymentSection.cs
- ThicknessAnimation.cs
- DtdParser.cs
- XmlWriterDelegator.cs
- TypeConstant.cs
- DataList.cs
- ArrayHelper.cs
- GPPOINT.cs
- BulletChrome.cs
- GrabHandleGlyph.cs
- WebPartZoneDesigner.cs
- WebCategoryAttribute.cs
- ExtenderProviderService.cs
- ActiveDesignSurfaceEvent.cs
- PathFigure.cs
- UnsafeNativeMethods.cs
- PlanCompiler.cs
- PropVariant.cs
- TransportationConfigurationTypeInstallComponent.cs
- Section.cs
- WebSysDisplayNameAttribute.cs
- InputScope.cs
- FlowDocumentView.cs
- WeakEventManager.cs
- AuthorizationSection.cs
- TreeWalkHelper.cs
- StrokeSerializer.cs
- ModifierKeysValueSerializer.cs
- NotSupportedException.cs
- WebServicesSection.cs
- OleDbPropertySetGuid.cs
- HwndStylusInputProvider.cs
- FileSystemWatcher.cs
- ForeignKeyConstraint.cs
- Command.cs
- TransformerInfo.cs
- Ipv6Element.cs
- ListViewGroupConverter.cs
- ErrorWrapper.cs
- SqlDataReader.cs
- SqlConnectionPoolGroupProviderInfo.cs
- TypeSource.cs
- HttpListenerContext.cs
- CorePropertiesFilter.cs
- StringSource.cs
- StylusSystemGestureEventArgs.cs
- EventTask.cs
- MatrixCamera.cs
- FlagsAttribute.cs
- EditableRegion.cs
- BaseAsyncResult.cs
- HierarchicalDataBoundControl.cs
- EditorZoneAutoFormat.cs
- WindowsIPAddress.cs
- ChainedAsyncResult.cs
- TypeElement.cs
- ManagementOptions.cs
- DirectionalLight.cs
- FormatterServices.cs
- SerialErrors.cs
- Cursors.cs
- AllMembershipCondition.cs
- ExceptionUtil.cs
- DateTimeSerializationSection.cs
- Types.cs
- ListenerElementsCollection.cs
- TextSchema.cs
- SecurityDocument.cs
- HeaderPanel.cs
- SimpleWorkerRequest.cs
- GiveFeedbackEvent.cs
- HttpDateParse.cs
- EventsTab.cs
- DesignerLoader.cs
- XsltOutput.cs