Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataSet / System / Data / DataSetUtil.cs / 1305376 / DataSetUtil.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //[....] //----------------------------------------------------------------------------- using System; using System.Data; using System.Data.DataSetExtensions; using System.Diagnostics; internal static class DataSetUtil { #region CheckArgument internal static void CheckArgumentNull(T argumentValue, string argumentName) where T : class { if (null == argumentValue) { throw ArgumentNull(argumentName); } } #endregion #region Trace private static T TraceException (string trace, T e) { Debug.Assert(null != e, "TraceException: null Exception"); if (null != e) { //Bid.Trace(trace, e.ToString()); // will include callstack if permission is available } return e; } private static T TraceExceptionAsReturnValue (T e) { return TraceException(" '%ls'\n", e); } #endregion #region new Exception internal static ArgumentException Argument(string message) { return TraceExceptionAsReturnValue(new ArgumentException(message)); } internal static ArgumentNullException ArgumentNull(string message) { return TraceExceptionAsReturnValue(new ArgumentNullException(message)); } internal static ArgumentOutOfRangeException ArgumentOutOfRange(string message, string parameterName) { return TraceExceptionAsReturnValue(new ArgumentOutOfRangeException(parameterName, message)); } internal static InvalidCastException InvalidCast(string message) { return TraceExceptionAsReturnValue(new InvalidCastException(message)); } internal static InvalidOperationException InvalidOperation(string message) { return TraceExceptionAsReturnValue(new InvalidOperationException(message)); } internal static NotSupportedException NotSupported(string message) { return TraceExceptionAsReturnValue(new NotSupportedException(message)); } #endregion #region new EnumerationValueNotValid static internal ArgumentOutOfRangeException InvalidEnumerationValue(Type type, int value) { return ArgumentOutOfRange(Strings.DataSetLinq_InvalidEnumerationValue(type.Name, value.ToString(System.Globalization.CultureInfo.InvariantCulture)), type.Name); } static internal ArgumentOutOfRangeException InvalidDataRowState(DataRowState value) { #if DEBUG switch (value) { case DataRowState.Detached: case DataRowState.Unchanged: case DataRowState.Added: case DataRowState.Deleted: case DataRowState.Modified: Debug.Assert(false, "valid DataRowState " + value.ToString()); break; } #endif return InvalidEnumerationValue(typeof(DataRowState), (int)value); } static internal ArgumentOutOfRangeException InvalidLoadOption(LoadOption value) { #if DEBUG switch (value) { case LoadOption.OverwriteChanges: case LoadOption.PreserveChanges: case LoadOption.Upsert: Debug.Assert(false, "valid LoadOption " + value.ToString()); break; } #endif return InvalidEnumerationValue(typeof(LoadOption), (int)value); } #endregion // only StackOverflowException & ThreadAbortException are sealed classes static private readonly Type StackOverflowType = typeof(System.StackOverflowException); static private readonly Type OutOfMemoryType = typeof(System.OutOfMemoryException); static private readonly Type ThreadAbortType = typeof(System.Threading.ThreadAbortException); static private readonly Type NullReferenceType = typeof(System.NullReferenceException); static private readonly Type AccessViolationType = typeof(System.AccessViolationException); static private readonly Type SecurityType = typeof(System.Security.SecurityException); static internal bool IsCatchableExceptionType(Exception e) { // a 'catchable' exception is defined by what it is not. Type type = e.GetType(); return ((type != StackOverflowType) && (type != OutOfMemoryType) && (type != ThreadAbortType) && (type != NullReferenceType) && (type != AccessViolationType) && !SecurityType.IsAssignableFrom(type)); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //[....] //----------------------------------------------------------------------------- using System; using System.Data; using System.Data.DataSetExtensions; using System.Diagnostics; internal static class DataSetUtil { #region CheckArgument internal static void CheckArgumentNull(T argumentValue, string argumentName) where T : class { if (null == argumentValue) { throw ArgumentNull(argumentName); } } #endregion #region Trace private static T TraceException (string trace, T e) { Debug.Assert(null != e, "TraceException: null Exception"); if (null != e) { //Bid.Trace(trace, e.ToString()); // will include callstack if permission is available } return e; } private static T TraceExceptionAsReturnValue (T e) { return TraceException(" '%ls'\n", e); } #endregion #region new Exception internal static ArgumentException Argument(string message) { return TraceExceptionAsReturnValue(new ArgumentException(message)); } internal static ArgumentNullException ArgumentNull(string message) { return TraceExceptionAsReturnValue(new ArgumentNullException(message)); } internal static ArgumentOutOfRangeException ArgumentOutOfRange(string message, string parameterName) { return TraceExceptionAsReturnValue(new ArgumentOutOfRangeException(parameterName, message)); } internal static InvalidCastException InvalidCast(string message) { return TraceExceptionAsReturnValue(new InvalidCastException(message)); } internal static InvalidOperationException InvalidOperation(string message) { return TraceExceptionAsReturnValue(new InvalidOperationException(message)); } internal static NotSupportedException NotSupported(string message) { return TraceExceptionAsReturnValue(new NotSupportedException(message)); } #endregion #region new EnumerationValueNotValid static internal ArgumentOutOfRangeException InvalidEnumerationValue(Type type, int value) { return ArgumentOutOfRange(Strings.DataSetLinq_InvalidEnumerationValue(type.Name, value.ToString(System.Globalization.CultureInfo.InvariantCulture)), type.Name); } static internal ArgumentOutOfRangeException InvalidDataRowState(DataRowState value) { #if DEBUG switch (value) { case DataRowState.Detached: case DataRowState.Unchanged: case DataRowState.Added: case DataRowState.Deleted: case DataRowState.Modified: Debug.Assert(false, "valid DataRowState " + value.ToString()); break; } #endif return InvalidEnumerationValue(typeof(DataRowState), (int)value); } static internal ArgumentOutOfRangeException InvalidLoadOption(LoadOption value) { #if DEBUG switch (value) { case LoadOption.OverwriteChanges: case LoadOption.PreserveChanges: case LoadOption.Upsert: Debug.Assert(false, "valid LoadOption " + value.ToString()); break; } #endif return InvalidEnumerationValue(typeof(LoadOption), (int)value); } #endregion // only StackOverflowException & ThreadAbortException are sealed classes static private readonly Type StackOverflowType = typeof(System.StackOverflowException); static private readonly Type OutOfMemoryType = typeof(System.OutOfMemoryException); static private readonly Type ThreadAbortType = typeof(System.Threading.ThreadAbortException); static private readonly Type NullReferenceType = typeof(System.NullReferenceException); static private readonly Type AccessViolationType = typeof(System.AccessViolationException); static private readonly Type SecurityType = typeof(System.Security.SecurityException); static internal bool IsCatchableExceptionType(Exception e) { // a 'catchable' exception is defined by what it is not. Type type = e.GetType(); return ((type != StackOverflowType) && (type != OutOfMemoryType) && (type != ThreadAbortType) && (type != NullReferenceType) && (type != AccessViolationType) && !SecurityType.IsAssignableFrom(type)); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
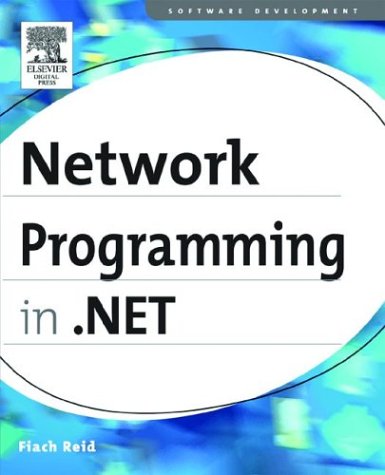
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MimeImporter.cs
- SiteMapSection.cs
- OleDbConnection.cs
- DispatcherSynchronizationContext.cs
- PenThreadPool.cs
- util.cs
- SqlComparer.cs
- LoadGrammarCompletedEventArgs.cs
- EmbeddedMailObjectsCollection.cs
- Transform3D.cs
- EntityDataSourceChangedEventArgs.cs
- DocumentManager.cs
- WebPartVerbCollection.cs
- ConfigXmlWhitespace.cs
- SetMemberBinder.cs
- HitTestParameters.cs
- XmlSchemaComplexContentExtension.cs
- precedingquery.cs
- CanonicalXml.cs
- LocationEnvironment.cs
- MetadataWorkspace.cs
- ArrayTypeMismatchException.cs
- ExpressionBindingsDialog.cs
- URL.cs
- FileLevelControlBuilderAttribute.cs
- DoubleAnimation.cs
- WrappedReader.cs
- DataGridViewCellMouseEventArgs.cs
- TreeIterators.cs
- FormsAuthenticationConfiguration.cs
- METAHEADER.cs
- FileSystemInfo.cs
- PeerUnsafeNativeMethods.cs
- ObjectQueryExecutionPlan.cs
- WmlPanelAdapter.cs
- Expressions.cs
- RealizationDrawingContextWalker.cs
- HostedNamedPipeTransportManager.cs
- DefaultProxySection.cs
- xdrvalidator.cs
- ExpressionBuilder.cs
- login.cs
- OleDbPermission.cs
- WebPartCloseVerb.cs
- XmlJsonWriter.cs
- Int16Converter.cs
- XmlReflectionMember.cs
- BaseTemplateCodeDomTreeGenerator.cs
- RuntimeHelpers.cs
- TreeNodeStyle.cs
- DataGridViewLinkColumn.cs
- PixelFormatConverter.cs
- DocumentProperties.cs
- XmlReflectionImporter.cs
- LinkButton.cs
- SchemaCollectionPreprocessor.cs
- AnonymousIdentificationModule.cs
- ConnectionOrientedTransportBindingElement.cs
- XmlNodeReader.cs
- ToolboxComponentsCreatedEventArgs.cs
- DeferredReference.cs
- ComboBox.cs
- TableLayoutStyleCollection.cs
- PhysicalAddress.cs
- StrokeNode.cs
- FormClosedEvent.cs
- GridViewHeaderRowPresenter.cs
- XmlMembersMapping.cs
- sqlstateclientmanager.cs
- RequestResizeEvent.cs
- GenericTypeParameterBuilder.cs
- MultiDataTrigger.cs
- TimeStampChecker.cs
- NetworkInformationPermission.cs
- RuleProcessor.cs
- CaseCqlBlock.cs
- ApplicationBuildProvider.cs
- StringConverter.cs
- ApplicationSecurityManager.cs
- Sentence.cs
- SafeReversePInvokeHandle.cs
- ListViewHitTestInfo.cs
- XmlDeclaration.cs
- FreezableDefaultValueFactory.cs
- NumberFunctions.cs
- IPHostEntry.cs
- SqlNodeAnnotation.cs
- AsyncCodeActivityContext.cs
- PathHelper.cs
- Baml2006Reader.cs
- Int32AnimationUsingKeyFrames.cs
- CheckedPointers.cs
- DefaultAssemblyResolver.cs
- ScriptingSectionGroup.cs
- SafeArrayRankMismatchException.cs
- RuntimeComponentFilter.cs
- OrderByQueryOptionExpression.cs
- XsltCompileContext.cs
- EventLogException.cs
- TypeResolver.cs