Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / WinForms / Managed / System / WinForms / ComponentModel / COM2Interop / BaseCAMarshaler.cs / 1 / BaseCAMarshaler.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms.ComponentModel.Com2Interop { using System.Runtime.Remoting; using System.Runtime.InteropServices; using System.ComponentModel; using System.Diagnostics; using System; using Microsoft.Win32; using System.Globalization; ////// /// This class performs basic operation for marshaling data passed /// in from native in one of the CA*** structs (CADWORD, CAUUID, etc), /// which are structs in which the first word is the number of elements /// and the second is a pointer to an array of such elements. /// /// internal abstract class BaseCAMarshaler { private static TraceSwitch CAMarshalSwitch = new TraceSwitch("CAMarshal", "BaseCAMarshaler: Debug CA* struct marshaling"); private IntPtr caArrayAddress; private int count; private object[] itemArray; ////// /// Base ctor /// protected BaseCAMarshaler(NativeMethods.CA_STRUCT caStruct) : base() { if (caStruct == null) { count = 0; Debug.WriteLineIf(CAMarshalSwitch.TraceVerbose, "BaseCAMarshaler: null passed in!"); } // first 4 bytes is the count count = caStruct.cElems; caArrayAddress = caStruct.pElems; Debug.WriteLineIf(CAMarshalSwitch.TraceVerbose, "Marshaling " + count.ToString(CultureInfo.InvariantCulture) + " items of type " + ItemType.Name); } ~BaseCAMarshaler() { try { if (itemArray == null && caArrayAddress != IntPtr.Zero) { object[] items = Items; } } catch { } } protected abstract Array CreateArray(); ////// /// Returns the type of item this marshaler will /// return in the items array. /// public abstract Type ItemType { get; } ////// /// Returns the count of items that will be or have been /// marshaled. /// public int Count { get { return count; } } ////// /// The marshaled items. /// public virtual object[] Items { get { try { if (itemArray == null) { itemArray = Get_Items(); } } catch (Exception ex) { Debug.WriteLineIf(CAMarshalSwitch.TraceVerbose, "Marshaling failed: " + ex.GetType().Name + ", " + ex.Message); } #if DEBUG if (itemArray != null) { Debug.WriteLineIf(CAMarshalSwitch.TraceVerbose, "Marshaled: " + itemArray.Length.ToString(CultureInfo.InvariantCulture) + " items, array type=" + itemArray.GetType().Name); } #endif return itemArray; } } ////// /// Override this member to perform marshalling of a single item /// given it's native address. /// protected abstract object GetItemFromAddress(IntPtr addr); // Retrieve the items private object[] Get_Items() { // cycle through the addresses and get an item for each addr IntPtr addr; Array items = new object[Count]; //cpb vs38262 System.Array.CreateInstance(this.ItemType,count); object curItem; for (int i = 0; i < count; i++) { try { addr = Marshal.ReadIntPtr(caArrayAddress, i * IntPtr.Size); curItem = GetItemFromAddress(addr); if (curItem != null && ItemType.IsInstanceOfType(curItem)) { items.SetValue(curItem, i); } Debug.WriteLineIf(CAMarshalSwitch.TraceVerbose, "Marshaled " + ItemType.Name + " item, value=" + (curItem == null ? "(null)" : curItem.ToString())); } catch (Exception ex) { Debug.WriteLineIf(CAMarshalSwitch.TraceVerbose, "Failed to marshal " + ItemType.Name + " item, exception=" + ex.GetType().Name +", " +ex.Message); } } // free the array Marshal.FreeCoTaskMem(caArrayAddress); caArrayAddress = IntPtr.Zero; return(object[])items; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms.ComponentModel.Com2Interop { using System.Runtime.Remoting; using System.Runtime.InteropServices; using System.ComponentModel; using System.Diagnostics; using System; using Microsoft.Win32; using System.Globalization; ////// /// This class performs basic operation for marshaling data passed /// in from native in one of the CA*** structs (CADWORD, CAUUID, etc), /// which are structs in which the first word is the number of elements /// and the second is a pointer to an array of such elements. /// /// internal abstract class BaseCAMarshaler { private static TraceSwitch CAMarshalSwitch = new TraceSwitch("CAMarshal", "BaseCAMarshaler: Debug CA* struct marshaling"); private IntPtr caArrayAddress; private int count; private object[] itemArray; ////// /// Base ctor /// protected BaseCAMarshaler(NativeMethods.CA_STRUCT caStruct) : base() { if (caStruct == null) { count = 0; Debug.WriteLineIf(CAMarshalSwitch.TraceVerbose, "BaseCAMarshaler: null passed in!"); } // first 4 bytes is the count count = caStruct.cElems; caArrayAddress = caStruct.pElems; Debug.WriteLineIf(CAMarshalSwitch.TraceVerbose, "Marshaling " + count.ToString(CultureInfo.InvariantCulture) + " items of type " + ItemType.Name); } ~BaseCAMarshaler() { try { if (itemArray == null && caArrayAddress != IntPtr.Zero) { object[] items = Items; } } catch { } } protected abstract Array CreateArray(); ////// /// Returns the type of item this marshaler will /// return in the items array. /// public abstract Type ItemType { get; } ////// /// Returns the count of items that will be or have been /// marshaled. /// public int Count { get { return count; } } ////// /// The marshaled items. /// public virtual object[] Items { get { try { if (itemArray == null) { itemArray = Get_Items(); } } catch (Exception ex) { Debug.WriteLineIf(CAMarshalSwitch.TraceVerbose, "Marshaling failed: " + ex.GetType().Name + ", " + ex.Message); } #if DEBUG if (itemArray != null) { Debug.WriteLineIf(CAMarshalSwitch.TraceVerbose, "Marshaled: " + itemArray.Length.ToString(CultureInfo.InvariantCulture) + " items, array type=" + itemArray.GetType().Name); } #endif return itemArray; } } ////// /// Override this member to perform marshalling of a single item /// given it's native address. /// protected abstract object GetItemFromAddress(IntPtr addr); // Retrieve the items private object[] Get_Items() { // cycle through the addresses and get an item for each addr IntPtr addr; Array items = new object[Count]; //cpb vs38262 System.Array.CreateInstance(this.ItemType,count); object curItem; for (int i = 0; i < count; i++) { try { addr = Marshal.ReadIntPtr(caArrayAddress, i * IntPtr.Size); curItem = GetItemFromAddress(addr); if (curItem != null && ItemType.IsInstanceOfType(curItem)) { items.SetValue(curItem, i); } Debug.WriteLineIf(CAMarshalSwitch.TraceVerbose, "Marshaled " + ItemType.Name + " item, value=" + (curItem == null ? "(null)" : curItem.ToString())); } catch (Exception ex) { Debug.WriteLineIf(CAMarshalSwitch.TraceVerbose, "Failed to marshal " + ItemType.Name + " item, exception=" + ex.GetType().Name +", " +ex.Message); } } // free the array Marshal.FreeCoTaskMem(caArrayAddress); caArrayAddress = IntPtr.Zero; return(object[])items; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
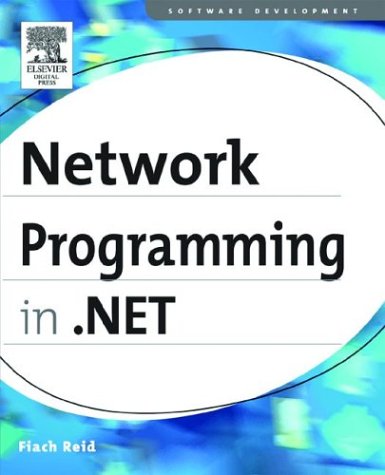
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WebBrowserUriTypeConverter.cs
- TextStore.cs
- ObjectParameter.cs
- SourceElementsCollection.cs
- NonBatchDirectoryCompiler.cs
- DataGridViewRowsRemovedEventArgs.cs
- CriticalHandle.cs
- ToggleButtonAutomationPeer.cs
- DataGridRow.cs
- WindowCollection.cs
- BaseDataList.cs
- WrappingXamlSchemaContext.cs
- WebPartAddingEventArgs.cs
- ServiceProviders.cs
- ConfigurationValidatorAttribute.cs
- GenericNameHandler.cs
- webproxy.cs
- PiiTraceSource.cs
- _ContextAwareResult.cs
- RowsCopiedEventArgs.cs
- WebPartZone.cs
- RepeatButtonAutomationPeer.cs
- CounterCreationData.cs
- DataGridViewCellValidatingEventArgs.cs
- UIPermission.cs
- XsdCachingReader.cs
- SecurityTokenSerializer.cs
- IOThreadTimer.cs
- UnknownBitmapEncoder.cs
- TextRangeEditLists.cs
- MissingSatelliteAssemblyException.cs
- CheckedListBox.cs
- SemanticAnalyzer.cs
- WebBrowserPermission.cs
- ListViewEditEventArgs.cs
- OrderedEnumerableRowCollection.cs
- SimpleWorkerRequest.cs
- UInt64Storage.cs
- AdornedElementPlaceholder.cs
- SystemPens.cs
- ControlType.cs
- CodeEntryPointMethod.cs
- WinEventWrap.cs
- ISO2022Encoding.cs
- TextTreePropertyUndoUnit.cs
- StrokeIntersection.cs
- LassoHelper.cs
- WriteableBitmap.cs
- RemoteHelper.cs
- NullExtension.cs
- SQLUtility.cs
- HandleExceptionArgs.cs
- DeflateEmulationStream.cs
- ListViewUpdateEventArgs.cs
- DataGridViewRowConverter.cs
- WebPartConnectionsEventArgs.cs
- Dump.cs
- InstallerTypeAttribute.cs
- IPipelineRuntime.cs
- ConfigurationLocation.cs
- ActivityWithResultValueSerializer.cs
- CodeDesigner.cs
- FrameworkElement.cs
- ResXResourceSet.cs
- Trace.cs
- GenericPrincipal.cs
- GeneralTransform.cs
- MultiView.cs
- PartialList.cs
- ThreadAbortException.cs
- ServicePoint.cs
- SslSecurityTokenParameters.cs
- MulticastIPAddressInformationCollection.cs
- NetTcpSecurity.cs
- TTSEngineTypes.cs
- OpCodes.cs
- SqlColumnizer.cs
- EditingCommands.cs
- State.cs
- FilteredAttributeCollection.cs
- EndOfStreamException.cs
- ChildChangedEventArgs.cs
- HandleCollector.cs
- DataGridTablesFactory.cs
- ResourceManagerWrapper.cs
- OdbcConnectionPoolProviderInfo.cs
- ConfigurationElement.cs
- Geometry3D.cs
- Literal.cs
- itemelement.cs
- PropertyChange.cs
- PrimitiveCodeDomSerializer.cs
- DataSetMappper.cs
- LineGeometry.cs
- DockPatternIdentifiers.cs
- DbSourceCommand.cs
- CodeConditionStatement.cs
- SafeLibraryHandle.cs
- ProfileInfo.cs
- CodeLinePragma.cs