Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / Xml / System / Xml / Serialization / _Events.cs / 1 / _Events.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace System.Xml.Serialization { using System.IO; using System; using System.Collections; using System.ComponentModel; ////// /// public delegate void XmlAttributeEventHandler(object sender, XmlAttributeEventArgs e); ///[To be supplied.] ////// /// public class XmlAttributeEventArgs : EventArgs { object o; XmlAttribute attr; string qnames; int lineNumber; int linePosition; internal XmlAttributeEventArgs(XmlAttribute attr, int lineNumber, int linePosition, object o, string qnames) { this.attr = attr; this.o = o; this.qnames = qnames; this.lineNumber = lineNumber; this.linePosition = linePosition; } ///[To be supplied.] ////// /// public object ObjectBeingDeserialized { get { return o; } } ///[To be supplied.] ////// /// public XmlAttribute Attr { get { return attr; } } ///[To be supplied.] ////// /// public int LineNumber { get { return lineNumber; } } ////// Gets the current line number. /// ////// /// public int LinePosition { get { return linePosition; } } ////// Gets the current line position. /// ////// /// public string ExpectedAttributes { get { return qnames == null ? string.Empty : qnames; } } } ////// List the qnames of attributes expected in the current context. /// ///public delegate void XmlElementEventHandler(object sender, XmlElementEventArgs e); /// public class XmlElementEventArgs : EventArgs { object o; XmlElement elem; string qnames; int lineNumber; int linePosition; internal XmlElementEventArgs(XmlElement elem, int lineNumber, int linePosition, object o, string qnames) { this.elem = elem; this.o = o; this.qnames = qnames; this.lineNumber = lineNumber; this.linePosition = linePosition; } /// public object ObjectBeingDeserialized { get { return o; } } /// public XmlElement Element { get { return elem; } } /// public int LineNumber { get { return lineNumber; } } /// public int LinePosition { get { return linePosition; } } /// /// /// public string ExpectedElements { get { return qnames == null ? string.Empty : qnames; } } } ////// List of qnames of elements expected in the current context. /// ////// /// public delegate void XmlNodeEventHandler(object sender, XmlNodeEventArgs e); ///[To be supplied.] ////// /// public class XmlNodeEventArgs : EventArgs { object o; XmlNode xmlNode; int lineNumber; int linePosition; internal XmlNodeEventArgs(XmlNode xmlNode, int lineNumber, int linePosition, object o) { this.o = o; this.xmlNode = xmlNode; this.lineNumber = lineNumber; this.linePosition = linePosition; } ///[To be supplied.] ////// /// public object ObjectBeingDeserialized { get { return o; } } ///[To be supplied.] ////// /// public XmlNodeType NodeType { get { return xmlNode.NodeType; } } ///[To be supplied.] ////// /// public string Name { get { return xmlNode.Name; } } ///[To be supplied.] ////// /// public string LocalName { get { return xmlNode.LocalName; } } ///[To be supplied.] ////// /// public string NamespaceURI { get { return xmlNode.NamespaceURI; } } ///[To be supplied.] ////// /// public string Text { get { return xmlNode.Value; } } ///[To be supplied.] ////// /// public int LineNumber { get { return lineNumber; } } ////// Gets the current line number. /// ////// /// public int LinePosition { get { return linePosition; } } } ////// Gets the current line position. /// ///public delegate void UnreferencedObjectEventHandler(object sender, UnreferencedObjectEventArgs e); /// public class UnreferencedObjectEventArgs : EventArgs { object o; string id; /// public UnreferencedObjectEventArgs(object o, string id) { this.o = o; this.id = id; } /// public object UnreferencedObject { get { return o; } } /// public string UnreferencedId { get { return id; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace System.Xml.Serialization { using System.IO; using System; using System.Collections; using System.ComponentModel; ////// /// public delegate void XmlAttributeEventHandler(object sender, XmlAttributeEventArgs e); ///[To be supplied.] ////// /// public class XmlAttributeEventArgs : EventArgs { object o; XmlAttribute attr; string qnames; int lineNumber; int linePosition; internal XmlAttributeEventArgs(XmlAttribute attr, int lineNumber, int linePosition, object o, string qnames) { this.attr = attr; this.o = o; this.qnames = qnames; this.lineNumber = lineNumber; this.linePosition = linePosition; } ///[To be supplied.] ////// /// public object ObjectBeingDeserialized { get { return o; } } ///[To be supplied.] ////// /// public XmlAttribute Attr { get { return attr; } } ///[To be supplied.] ////// /// public int LineNumber { get { return lineNumber; } } ////// Gets the current line number. /// ////// /// public int LinePosition { get { return linePosition; } } ////// Gets the current line position. /// ////// /// public string ExpectedAttributes { get { return qnames == null ? string.Empty : qnames; } } } ////// List the qnames of attributes expected in the current context. /// ///public delegate void XmlElementEventHandler(object sender, XmlElementEventArgs e); /// public class XmlElementEventArgs : EventArgs { object o; XmlElement elem; string qnames; int lineNumber; int linePosition; internal XmlElementEventArgs(XmlElement elem, int lineNumber, int linePosition, object o, string qnames) { this.elem = elem; this.o = o; this.qnames = qnames; this.lineNumber = lineNumber; this.linePosition = linePosition; } /// public object ObjectBeingDeserialized { get { return o; } } /// public XmlElement Element { get { return elem; } } /// public int LineNumber { get { return lineNumber; } } /// public int LinePosition { get { return linePosition; } } /// /// /// public string ExpectedElements { get { return qnames == null ? string.Empty : qnames; } } } ////// List of qnames of elements expected in the current context. /// ////// /// public delegate void XmlNodeEventHandler(object sender, XmlNodeEventArgs e); ///[To be supplied.] ////// /// public class XmlNodeEventArgs : EventArgs { object o; XmlNode xmlNode; int lineNumber; int linePosition; internal XmlNodeEventArgs(XmlNode xmlNode, int lineNumber, int linePosition, object o) { this.o = o; this.xmlNode = xmlNode; this.lineNumber = lineNumber; this.linePosition = linePosition; } ///[To be supplied.] ////// /// public object ObjectBeingDeserialized { get { return o; } } ///[To be supplied.] ////// /// public XmlNodeType NodeType { get { return xmlNode.NodeType; } } ///[To be supplied.] ////// /// public string Name { get { return xmlNode.Name; } } ///[To be supplied.] ////// /// public string LocalName { get { return xmlNode.LocalName; } } ///[To be supplied.] ////// /// public string NamespaceURI { get { return xmlNode.NamespaceURI; } } ///[To be supplied.] ////// /// public string Text { get { return xmlNode.Value; } } ///[To be supplied.] ////// /// public int LineNumber { get { return lineNumber; } } ////// Gets the current line number. /// ////// /// public int LinePosition { get { return linePosition; } } } ////// Gets the current line position. /// ///public delegate void UnreferencedObjectEventHandler(object sender, UnreferencedObjectEventArgs e); /// public class UnreferencedObjectEventArgs : EventArgs { object o; string id; /// public UnreferencedObjectEventArgs(object o, string id) { this.o = o; this.id = id; } /// public object UnreferencedObject { get { return o; } } /// public string UnreferencedId { get { return id; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
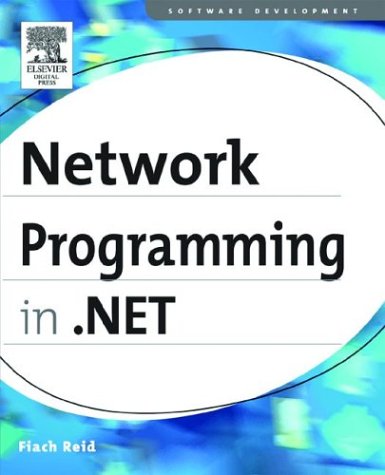
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MetadataStore.cs
- MonitorWrapper.cs
- Compiler.cs
- InfiniteTimeSpanConverter.cs
- SettingsSection.cs
- CategoryGridEntry.cs
- NegotiationTokenAuthenticatorStateCache.cs
- PagesChangedEventArgs.cs
- UnaryNode.cs
- CodeMemberField.cs
- ColorTransform.cs
- DynamicPropertyHolder.cs
- SqlFunctionAttribute.cs
- DNS.cs
- XmlElementElement.cs
- StaticExtension.cs
- MimeParameters.cs
- RelationshipManager.cs
- SpellerStatusTable.cs
- ApplyTemplatesAction.cs
- COM2PictureConverter.cs
- ListParaClient.cs
- HashSetEqualityComparer.cs
- TripleDESCryptoServiceProvider.cs
- PrimitiveDataContract.cs
- ValidationSummary.cs
- GridViewDeletedEventArgs.cs
- Misc.cs
- _IPv4Address.cs
- PersistenceException.cs
- bidPrivateBase.cs
- SoapAttributeAttribute.cs
- HuffmanTree.cs
- SessionStateModule.cs
- StorageComplexTypeMapping.cs
- ArrayTypeMismatchException.cs
- PartitionResolver.cs
- UnmanagedMarshal.cs
- SocketInformation.cs
- IImplicitResourceProvider.cs
- EventManager.cs
- DbConnectionClosed.cs
- FastPropertyAccessor.cs
- SqlDataReader.cs
- IgnoreFileBuildProvider.cs
- RuntimeCompatibilityAttribute.cs
- SubMenuStyle.cs
- CollectionBase.cs
- SolidColorBrush.cs
- ZipPackagePart.cs
- regiisutil.cs
- SQLMoney.cs
- WindowsListViewGroup.cs
- NamespaceList.cs
- UrlMappingsSection.cs
- SharingService.cs
- UpWmlMobileTextWriter.cs
- WebPartConnectionsConnectVerb.cs
- SynchronizationContext.cs
- GridErrorDlg.cs
- FtpCachePolicyElement.cs
- AvTraceDetails.cs
- EndOfStreamException.cs
- UrlPath.cs
- FigureHelper.cs
- GAC.cs
- RulePatternOps.cs
- ExpressionDumper.cs
- Renderer.cs
- ConnectionsZoneAutoFormat.cs
- MetadataStore.cs
- XamlInt32CollectionSerializer.cs
- ConditionalAttribute.cs
- ping.cs
- TreeViewImageIndexConverter.cs
- SoapObjectWriter.cs
- SafeMILHandleMemoryPressure.cs
- HandleTable.cs
- DataListAutoFormat.cs
- QilGenerator.cs
- ComplexLine.cs
- ComponentChangingEvent.cs
- EndpointNotFoundException.cs
- BamlLocalizerErrorNotifyEventArgs.cs
- StringInfo.cs
- ConfigurationStrings.cs
- AuthorizationRule.cs
- HttpCapabilitiesSectionHandler.cs
- FormatSelectingMessageInspector.cs
- WebBrowserUriTypeConverter.cs
- CodeIndexerExpression.cs
- WmfPlaceableFileHeader.cs
- BulletedListEventArgs.cs
- ServiceDescriptionImporter.cs
- XmlDictionaryReaderQuotasElement.cs
- SqlAggregateChecker.cs
- UrlPath.cs
- EnumConverter.cs
- FamilyMap.cs
- CodeGenerator.cs