Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / CompMod / System / CodeDOM / Compiler / CompilerParameters.cs / 1 / CompilerParameters.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.CodeDom.Compiler { using System; using System.CodeDom; using System.Collections; using System.Collections.Specialized; using Microsoft.Win32; using Microsoft.Win32.SafeHandles; using System.Runtime.InteropServices; using System.Security.Permissions; using System.Security.Policy; using System.Runtime.Serialization; ////// [PermissionSet(SecurityAction.LinkDemand, Name="FullTrust")] [PermissionSet(SecurityAction.InheritanceDemand, Name="FullTrust")] [Serializable] public class CompilerParameters { private StringCollection assemblyNames = new StringCollection(); [OptionalField] private StringCollection embeddedResources = new StringCollection(); [OptionalField] private StringCollection linkedResources = new StringCollection(); private string outputName; private string mainClass; private bool generateInMemory = false; private bool includeDebugInformation = false; private int warningLevel = -1; // -1 means not set (use compiler default) private string compilerOptions; private string win32Resource; private bool treatWarningsAsErrors = false; private bool generateExecutable = false; private TempFileCollection tempFiles; [NonSerializedAttribute] private SafeUserTokenHandle userToken; private Evidence evidence = null; ////// Represents the parameters used in to invoke the compiler. /// ////// public CompilerParameters() : this(null, null) { } ////// Initializes a new instance of ///. /// /// public CompilerParameters(string[] assemblyNames) : this(assemblyNames, null, false) { } ////// Initializes a new instance of ///using the specified /// assembly names. /// /// public CompilerParameters(string[] assemblyNames, string outputName) : this(assemblyNames, outputName, false) { } ////// Initializes a new instance of ///using the specified /// assembly names and output name. /// /// public CompilerParameters(string[] assemblyNames, string outputName, bool includeDebugInformation) { if (assemblyNames != null) { ReferencedAssemblies.AddRange(assemblyNames); } this.outputName = outputName; this.includeDebugInformation = includeDebugInformation; } ////// Initializes a new instance of ///using the specified /// assembly names, output name and a whether to include debug information flag. /// /// public bool GenerateExecutable { get { return generateExecutable; } set { generateExecutable = value; } } ////// Gets or sets whether to generate an executable. /// ////// public bool GenerateInMemory { get { return generateInMemory; } set { generateInMemory = value; } } ////// Gets or sets whether to generate in memory. /// ////// public StringCollection ReferencedAssemblies { get { return assemblyNames; } } ////// Gets or sets the assemblies referenced by the source to compile. /// ////// public string MainClass { get { return mainClass; } set { mainClass = value; } } ////// Gets or sets the main class. /// ////// public string OutputAssembly { get { return outputName; } set { outputName = value; } } ////// Gets or sets the output assembly. /// ////// public TempFileCollection TempFiles { get { if (tempFiles == null) tempFiles = new TempFileCollection(); return tempFiles; } set { tempFiles = value; } } ////// Gets or sets the temp files. /// ////// public bool IncludeDebugInformation { get { return includeDebugInformation; } set { includeDebugInformation = value; } } ////// Gets or sets whether to include debug information in the compiled /// executable. /// ////// public bool TreatWarningsAsErrors { get { return treatWarningsAsErrors; } set { treatWarningsAsErrors = value; } } ///[To be supplied.] ////// public int WarningLevel { get { return warningLevel; } set { warningLevel = value; } } ///[To be supplied.] ////// public string CompilerOptions { get { return compilerOptions; } set { compilerOptions = value; } } ///[To be supplied.] ////// public string Win32Resource { get { return win32Resource; } set { win32Resource = value; } } ///[To be supplied.] ////// [System.Runtime.InteropServices.ComVisible(false)] public StringCollection EmbeddedResources { get { return embeddedResources; } } ////// Gets or sets the resources to be compiled into the target /// ////// [System.Runtime.InteropServices.ComVisible(false)] public StringCollection LinkedResources { get { return linkedResources; } } ////// Gets or sets the linked resources /// ////// public IntPtr UserToken { get { if (userToken != null) return userToken.DangerousGetHandle(); else return IntPtr.Zero; } set { if (userToken != null) userToken.Close(); userToken = new SafeUserTokenHandle(value, false); } } internal SafeUserTokenHandle SafeUserToken { get { return userToken; } } ////// Gets or sets user token to be employed when creating the compiler process. /// ////// public Evidence Evidence { get { Evidence e = null; if (evidence != null) e = CompilerResults.CloneEvidence(evidence); return e; } [SecurityPermissionAttribute( SecurityAction.Demand, ControlEvidence = true )] set { if (value != null) evidence = CompilerResults.CloneEvidence(value); else evidence = null; } } } }/// Set the evidence for partially trusted scenarios. /// ///
Link Menu
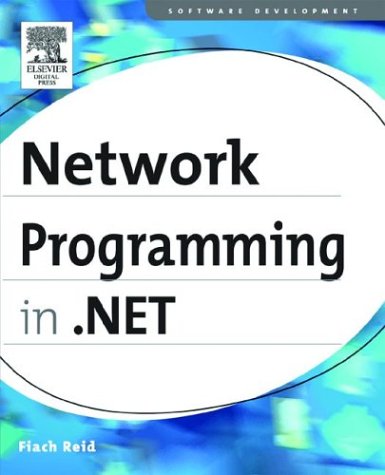
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- IERequestCache.cs
- FrameworkRichTextComposition.cs
- NotifyInputEventArgs.cs
- TrackingProfile.cs
- DoWhileDesigner.xaml.cs
- XmlWriterDelegator.cs
- EnumUnknown.cs
- GregorianCalendar.cs
- TypeDescriptionProvider.cs
- DataGridViewMethods.cs
- InArgument.cs
- DatatypeImplementation.cs
- Thread.cs
- InstalledFontCollection.cs
- DbProviderServices.cs
- SolidBrush.cs
- EntityDataSourceColumn.cs
- RolePrincipal.cs
- TypeUtil.cs
- XPathCompileException.cs
- FormsAuthenticationConfiguration.cs
- CorePropertiesFilter.cs
- ValidationError.cs
- GlyphCollection.cs
- XPathMultyIterator.cs
- RadioButtonFlatAdapter.cs
- InvokePattern.cs
- FatalException.cs
- ActivityContext.cs
- PeerNameResolver.cs
- externdll.cs
- MediaContextNotificationWindow.cs
- AliasGenerator.cs
- DecoderFallback.cs
- SecurityManager.cs
- smtpconnection.cs
- HttpModulesSection.cs
- PropertyInfoSet.cs
- RemoteWebConfigurationHostServer.cs
- ContractListAdapter.cs
- XmlTextReader.cs
- MsmqAppDomainProtocolHandler.cs
- OneToOneMappingSerializer.cs
- FacetDescriptionElement.cs
- BevelBitmapEffect.cs
- ApplicationFileParser.cs
- MasterPageBuildProvider.cs
- RequestBringIntoViewEventArgs.cs
- TcpClientSocketManager.cs
- DataGridViewToolTip.cs
- XmlCDATASection.cs
- IList.cs
- mongolianshape.cs
- AddressUtility.cs
- UpdatePanelControlTrigger.cs
- DataGridViewButtonColumn.cs
- CqlLexerHelpers.cs
- ExtendedProtectionPolicyElement.cs
- SmtpReplyReader.cs
- OpCellTreeNode.cs
- TiffBitmapDecoder.cs
- EmptyControlCollection.cs
- UniqueIdentifierService.cs
- Facet.cs
- PowerModeChangedEventArgs.cs
- ProtocolReflector.cs
- OpenTypeLayout.cs
- securitymgrsite.cs
- DataGrid.cs
- WebPartEditorApplyVerb.cs
- TTSEngineTypes.cs
- UserControlBuildProvider.cs
- _NTAuthentication.cs
- SchemaHelper.cs
- KerberosTokenFactoryCredential.cs
- TraceSwitch.cs
- XmlValidatingReader.cs
- TransformerInfo.cs
- IdentitySection.cs
- AddInIpcChannel.cs
- XmlProcessingInstruction.cs
- SamlNameIdentifierClaimResource.cs
- _HeaderInfo.cs
- Compiler.cs
- TriggerAction.cs
- ConfigXmlComment.cs
- SafeCoTaskMem.cs
- OrderedDictionary.cs
- LocationFactory.cs
- MouseBinding.cs
- DocumentViewerHelper.cs
- httpapplicationstate.cs
- Int16AnimationBase.cs
- CompatibleComparer.cs
- SchemaTableColumn.cs
- WebPartVerbCollection.cs
- SiteMembershipCondition.cs
- Dictionary.cs
- ServiceReference.cs
- shaperfactory.cs