Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / WinForms / Managed / System / WinForms / ComponentModel / COM2Interop / COM2PictureConverter.cs / 1 / COM2PictureConverter.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms.ComponentModel.Com2Interop { using System.Runtime.Serialization.Formatters; using System.ComponentModel; using System.Diagnostics; using System; using System.Drawing; using System.Collections; using Microsoft.Win32; ////// /// This class maps an IPicture to a System.Drawing.Image. /// internal class Com2PictureConverter : Com2DataTypeToManagedDataTypeConverter { object lastManaged; IntPtr lastNativeHandle; WeakReference pictureRef; IntPtr lastPalette = IntPtr.Zero; Type pictureType = typeof(Bitmap); public Com2PictureConverter(Com2PropertyDescriptor pd) { if (pd.DISPID == NativeMethods.ActiveX.DISPID_MOUSEICON || pd.Name.IndexOf("Icon") != -1) { pictureType = typeof(Icon); } } ////// /// Returns the managed type that this editor maps the property type to. /// public override Type ManagedType { get { return pictureType; } } ////// /// Converts the native value into a managed value /// public override object ConvertNativeToManaged(object nativeValue, Com2PropertyDescriptor pd) { if (nativeValue == null) { return null; } Debug.Assert(nativeValue is UnsafeNativeMethods.IPicture, "nativevalue is not IPicture"); UnsafeNativeMethods.IPicture nativePicture = (UnsafeNativeMethods.IPicture)nativeValue; IntPtr handle = nativePicture.GetHandle(); if (lastManaged != null && handle == lastNativeHandle) { return lastManaged; } lastNativeHandle = handle; //lastPalette = nativePicture.GetHPal(); if (handle != IntPtr.Zero) { switch (nativePicture.GetPictureType()) { case NativeMethods.Ole.PICTYPE_ICON: pictureType = typeof(Icon); lastManaged = Icon.FromHandle(handle); break; case NativeMethods.Ole.PICTYPE_BITMAP: pictureType = typeof(Bitmap); lastManaged = Image.FromHbitmap(handle); break; default: Debug.Fail("Unknown picture type"); break; } pictureRef = new WeakReference(nativePicture); } else { lastManaged = null; pictureRef = null; } return lastManaged; } ////// /// Converts the managed value into a native value /// public override object ConvertManagedToNative(object managedValue, Com2PropertyDescriptor pd, ref bool cancelSet) { // don't cancel the set cancelSet = false; if (lastManaged != null && lastManaged.Equals(managedValue) && pictureRef != null && pictureRef.IsAlive) { return pictureRef.Target; } // we have to build an IPicture lastManaged = managedValue; if (managedValue != null) { Guid g = typeof(UnsafeNativeMethods.IPicture).GUID; NativeMethods.PICTDESC pictdesc = null; bool own = false; if (lastManaged is Icon) { pictdesc = NativeMethods.PICTDESC.CreateIconPICTDESC(((Icon)lastManaged).Handle); } else if (lastManaged is Bitmap) { pictdesc = NativeMethods.PICTDESC.CreateBitmapPICTDESC(((Bitmap)lastManaged).GetHbitmap(), lastPalette); own = true; } else { Debug.Fail("Unknown Image type: " + managedValue.GetType().Name); } UnsafeNativeMethods.IPicture pict = UnsafeNativeMethods.OleCreatePictureIndirect(pictdesc, ref g, own); lastNativeHandle = pict.GetHandle(); pictureRef = new WeakReference(pict); return pict; } else { lastManaged = null; lastNativeHandle = lastPalette = IntPtr.Zero; pictureRef = null; return null; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
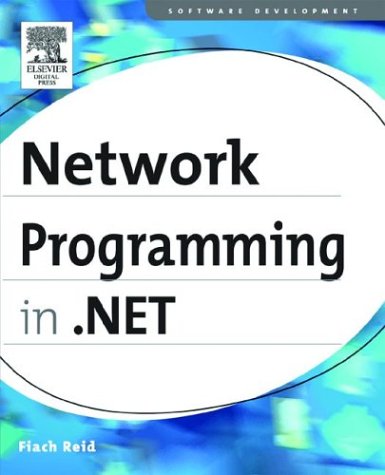
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ProfileGroupSettings.cs
- UInt32.cs
- PropertyTabAttribute.cs
- SqlConnectionString.cs
- BuildManager.cs
- DesignerTransactionCloseEvent.cs
- XmlSchemas.cs
- TrustSection.cs
- DocumentApplicationJournalEntryEventArgs.cs
- LogReservationCollection.cs
- XmlReaderSettings.cs
- RegistryKey.cs
- SecurityContext.cs
- EdmFunction.cs
- BaseComponentEditor.cs
- MinimizableAttributeTypeConverter.cs
- ToolboxComponentsCreatingEventArgs.cs
- SingleBodyParameterMessageFormatter.cs
- BitmapCodecInfoInternal.cs
- XmlSchemaComplexContent.cs
- StrictAndMessageFilter.cs
- FilterException.cs
- WebPartEventArgs.cs
- ConfigurationManagerInternalFactory.cs
- DockPattern.cs
- SignatureDescription.cs
- keycontainerpermission.cs
- HwndTarget.cs
- FreeFormDragDropManager.cs
- SafeMILHandle.cs
- XmlResolver.cs
- InternalConfigHost.cs
- CustomAttributeBuilder.cs
- ActivityTypeResolver.xaml.cs
- Thumb.cs
- DesignerOptions.cs
- TraceEventCache.cs
- GetReadStreamResult.cs
- PolyQuadraticBezierSegment.cs
- AmbientProperties.cs
- DataBoundControlHelper.cs
- BinaryWriter.cs
- DateBoldEvent.cs
- BaseDataListComponentEditor.cs
- Scene3D.cs
- CriticalFinalizerObject.cs
- EncoderReplacementFallback.cs
- GradientBrush.cs
- WebBrowserEvent.cs
- PopupRootAutomationPeer.cs
- TypedTableBase.cs
- HashStream.cs
- SoapDocumentServiceAttribute.cs
- RelatedView.cs
- ToolboxItemSnapLineBehavior.cs
- HashAlgorithm.cs
- CatalogPart.cs
- Walker.cs
- EntityDataSourceEntityTypeFilterItem.cs
- EntityDataSourceEntitySetNameItem.cs
- ProgressBar.cs
- FileNotFoundException.cs
- LinkUtilities.cs
- TransactionValidationBehavior.cs
- FastPropertyAccessor.cs
- Int32RectConverter.cs
- PersonalizationProviderCollection.cs
- Facet.cs
- XmlTextWriter.cs
- AppDomainFactory.cs
- ToolZone.cs
- ContextMenuStripActionList.cs
- xml.cs
- LoginView.cs
- CopyOnWriteList.cs
- XmlDocument.cs
- BoundField.cs
- XmlSiteMapProvider.cs
- SignatureHelper.cs
- ScriptIgnoreAttribute.cs
- ExpressionParser.cs
- LogicalExpr.cs
- QueueProcessor.cs
- ConstraintStruct.cs
- WebPart.cs
- AsyncDataRequest.cs
- SqlDataSourceEnumerator.cs
- RefreshEventArgs.cs
- DataGridViewImageCell.cs
- CapabilitiesAssignment.cs
- _FtpDataStream.cs
- Int32AnimationBase.cs
- PermissionListSet.cs
- UniqueSet.cs
- GlobalItem.cs
- XPathAncestorQuery.cs
- MimeTypeAttribute.cs
- ExpressionParser.cs
- IndexedEnumerable.cs
- XPathChildIterator.cs