Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / xsp / System / Web / UI / DataSourceView.cs / 1 / DataSourceView.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI { using System.Collections; using System.ComponentModel; using System.Security.Permissions; [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public abstract class DataSourceView { private static readonly object EventDataSourceViewChanged = new object(); private EventHandlerList _events; private string _name; protected DataSourceView(IDataSource owner, string viewName) { if (owner == null) { throw new ArgumentNullException("owner"); } if (viewName == null) { throw new ArgumentNullException("viewName"); } _name = viewName; DataSourceControl dataSourceControl = owner as DataSourceControl; if (dataSourceControl != null) { dataSourceControl.DataSourceChangedInternal += new EventHandler(OnDataSourceChangedInternal); } else { owner.DataSourceChanged += new EventHandler(OnDataSourceChangedInternal); } } // CanX properties indicate whether the data source allows each // operation, and if so, whether it's appropriate to do so. // For instance, a control may allow Deletion, but if a required Delete // command isn't set, CanDelete should be false, because a Delete // operation would fail. public virtual bool CanDelete { get { return false; } } public virtual bool CanInsert { get { return false; } } public virtual bool CanPage { get { return false; } } public virtual bool CanRetrieveTotalRowCount { get { return false; } } public virtual bool CanSort { get { return false; } } public virtual bool CanUpdate { get { return false; } } ////// Indicates the list of event handler delegates for the view. This property is read-only. /// protected EventHandlerList Events { get { if (_events == null) { _events = new EventHandlerList(); } return _events; } } public string Name { get { return _name; } } public event EventHandler DataSourceViewChanged { add { Events.AddHandler(EventDataSourceViewChanged, value); } remove { Events.RemoveHandler(EventDataSourceViewChanged, value); } } public virtual void Delete(IDictionary keys, IDictionary oldValues, DataSourceViewOperationCallback callback) { if (callback == null) { throw new ArgumentNullException("callback"); } int affectedRecords = 0; bool performedCallback = false; try { affectedRecords = ExecuteDelete(keys, oldValues); } catch (Exception ex) { performedCallback = true; if (!callback(affectedRecords, ex)) { throw; } } finally { if (!performedCallback) { callback(affectedRecords, null); } } } ////// Performs a delete operation on the specified list. This is only /// supported by a DataSourceControl when CanDelete returns true. /// /// /// The set of name/value pairs used to filter /// the items in the list that should be deleted. /// /// /// The complete set of name/value pairs used to filter /// the items in the list that should be deleted. /// ////// The number of items that were affected by the operation. /// protected virtual int ExecuteDelete(IDictionary keys, IDictionary oldValues) { throw new NotSupportedException(); } ////// Performs an insert operation on the specified list. This is only /// supported by a DataControl when CanInsert is true. /// /// /// The set of name/value pairs to be used to initialize /// a new item in the list. /// ////// The number of items that were affected by the operation. /// protected virtual int ExecuteInsert(IDictionary values) { throw new NotSupportedException(); } ////// protected internal abstract IEnumerable ExecuteSelect(DataSourceSelectArguments arguments); ////// Performs an update operation on the specified list. This is only /// supported by a DataControl when CanUpdate is true. /// /// /// The set of name/value pairs used to filter /// the items in the list that should be updated. /// /// /// The set of name/value pairs to be used to update the /// items in the list. /// /// /// The set of name/value pairs to be used to identify the /// item to be updated. /// ////// The number of items that were affected by the operation. /// protected virtual int ExecuteUpdate(IDictionary keys, IDictionary values, IDictionary oldValues) { throw new NotSupportedException(); } private void OnDataSourceChangedInternal(object sender, EventArgs e) { OnDataSourceViewChanged(e); } protected virtual void OnDataSourceViewChanged(EventArgs e) { EventHandler handler = Events[EventDataSourceViewChanged] as EventHandler; if (handler != null) { handler(this, e); } } public virtual void Insert(IDictionary values, DataSourceViewOperationCallback callback) { if (callback == null) { throw new ArgumentNullException("callback"); } int affectedRecords = 0; bool performedCallback = false; try { affectedRecords = ExecuteInsert(values); } catch (Exception ex) { performedCallback = true; if (!callback(affectedRecords, ex)) { throw; } } finally { if (!performedCallback) { callback(affectedRecords, null); } } } protected internal virtual void RaiseUnsupportedCapabilityError(DataSourceCapabilities capability) { if (!CanPage && ((capability & DataSourceCapabilities.Page) != 0)) { throw new NotSupportedException(SR.GetString(SR.DataSourceView_NoPaging)); } if (!CanSort && ((capability & DataSourceCapabilities.Sort) != 0)) { throw new NotSupportedException(SR.GetString(SR.DataSourceView_NoSorting)); } if (!CanRetrieveTotalRowCount && ((capability & DataSourceCapabilities.RetrieveTotalRowCount) != 0)) { throw new NotSupportedException(SR.GetString(SR.DataSourceView_NoRowCount)); } } public virtual void Select(DataSourceSelectArguments arguments, DataSourceViewSelectCallback callback) { if (callback == null) { throw new ArgumentNullException("callback"); } callback(ExecuteSelect(arguments)); } public virtual void Update(IDictionary keys, IDictionary values, IDictionary oldValues, DataSourceViewOperationCallback callback) { if (callback == null) { throw new ArgumentNullException("callback"); } int affectedRecords = 0; bool performedCallback = false; try { affectedRecords = ExecuteUpdate(keys, values, oldValues); } catch (Exception ex) { performedCallback = true; if (!callback(affectedRecords, ex)) { throw; } } finally { if (!performedCallback) { callback(affectedRecords, null); } } } } }
Link Menu
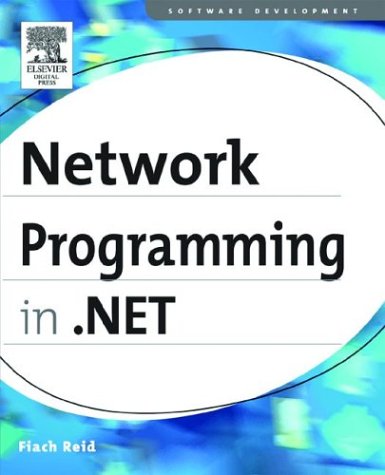
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Typeface.cs
- FrameworkContentElement.cs
- WindowsGraphicsWrapper.cs
- ScriptResourceAttribute.cs
- Geometry3D.cs
- NameValueFileSectionHandler.cs
- DataGridColumnCollection.cs
- ServiceOperationViewControl.cs
- FormViewInsertEventArgs.cs
- WindowsAltTab.cs
- ChildChangedEventArgs.cs
- DataViewManager.cs
- RectAnimationBase.cs
- ArraySubsetEnumerator.cs
- TemplatePropertyEntry.cs
- UInt16Storage.cs
- DrawingGroup.cs
- OracleDateTime.cs
- SqlDataSourceStatusEventArgs.cs
- DBProviderConfigurationHandler.cs
- ButtonFieldBase.cs
- TemplateParser.cs
- EditorPartChrome.cs
- Mapping.cs
- PtsContext.cs
- EmbeddedMailObjectsCollection.cs
- SafeRightsManagementEnvironmentHandle.cs
- StringBuilder.cs
- XmlRootAttribute.cs
- Event.cs
- Codec.cs
- ListParagraph.cs
- ZipIOExtraFieldPaddingElement.cs
- WeakReadOnlyCollection.cs
- ViewStateException.cs
- AppDomainProtocolHandler.cs
- EntityTypeBase.cs
- WpfPayload.cs
- TemplateParser.cs
- SignedXml.cs
- ellipse.cs
- QilVisitor.cs
- GorillaCodec.cs
- ToolBarTray.cs
- SmiXetterAccessMap.cs
- ContentType.cs
- DesignTimeVisibleAttribute.cs
- TogglePattern.cs
- CannotUnloadAppDomainException.cs
- ApplyTemplatesAction.cs
- BindingMemberInfo.cs
- GACIdentityPermission.cs
- DocumentXPathNavigator.cs
- WebPartEditorApplyVerb.cs
- PenContexts.cs
- IndexOutOfRangeException.cs
- EntityFunctions.cs
- Sentence.cs
- Vector3DAnimationUsingKeyFrames.cs
- TextEditorTables.cs
- FileUtil.cs
- SizeAnimationUsingKeyFrames.cs
- Vector3DKeyFrameCollection.cs
- DataBoundControl.cs
- AuthenticationException.cs
- ScriptResourceHandler.cs
- SqlDuplicator.cs
- TransformPattern.cs
- CriticalFinalizerObject.cs
- MonthCalendarDesigner.cs
- DoubleAnimationUsingPath.cs
- validationstate.cs
- FormatVersion.cs
- GeometryValueSerializer.cs
- RecordsAffectedEventArgs.cs
- RoutedCommand.cs
- ProfileSettingsCollection.cs
- ClientSettingsStore.cs
- MissingMethodException.cs
- HMAC.cs
- HostingEnvironment.cs
- SamlAdvice.cs
- TabControl.cs
- DataGridColumn.cs
- HistoryEventArgs.cs
- Int64Storage.cs
- RemotingClientProxy.cs
- AttachedPropertyBrowsableWhenAttributePresentAttribute.cs
- DataGridPageChangedEventArgs.cs
- MarshalByValueComponent.cs
- XPathException.cs
- IncrementalCompileAnalyzer.cs
- WindowsServiceElement.cs
- ExpressionBuilder.cs
- SchemaImporterExtensionElement.cs
- FontCacheUtil.cs
- CompiledELinqQueryState.cs
- HttpWriter.cs
- DocobjHost.cs
- SqlNodeAnnotation.cs