Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / xsp / System / Web / UI / WebControls / TableRow.cs / 2 / TableRow.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls { using System; using System.Collections; using System.ComponentModel; using System.ComponentModel.Design; using System.Globalization; using System.Web; using System.Web.UI; using System.Security.Permissions; ////// [ Bindable(false), DefaultProperty("Cells"), ParseChildren(true, "Cells"), ToolboxItem(false) ] [Designer("System.Web.UI.Design.WebControls.PreviewControlDesigner, " + AssemblyRef.SystemDesign)] [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public class TableRow : WebControl { TableCellCollection cells; ///Encapsulates a row /// within a table. ////// public TableRow() : base(HtmlTextWriterTag.Tr) { PreventAutoID(); } ////// Initializes a new instance of the ///class. /// /// [ MergableProperty(false), WebSysDescription(SR.TableRow_Cells), PersistenceMode(PersistenceMode.InnerDefaultProperty) ] public virtual TableCellCollection Cells { get { if (cells == null) cells = new TableCellCollection(this); return cells; } } ///Indicates the table cell collection of the table /// row. This property is read-only. ////// [ WebCategory("Layout"), DefaultValue(HorizontalAlign.NotSet), WebSysDescription(SR.TableItem_HorizontalAlign) ] public virtual HorizontalAlign HorizontalAlign { get { if (ControlStyleCreated == false) { return HorizontalAlign.NotSet; } return ((TableItemStyle)ControlStyle).HorizontalAlign; } set { ((TableItemStyle)ControlStyle).HorizontalAlign = value; } } [ WebCategory("Accessibility"), DefaultValue(TableRowSection.TableBody), WebSysDescription(SR.TableRow_TableSection) ] public virtual TableRowSection TableSection { get { object o = ViewState["TableSection"]; return((o == null) ? TableRowSection.TableBody : (TableRowSection)o); } set { if (value < TableRowSection.TableHeader || value > TableRowSection.TableFooter) { throw new ArgumentOutOfRangeException("value"); } ViewState["TableSection"] = value; if (value != TableRowSection.TableBody) { Control parent = Parent; if (parent != null) { Table parentTable = parent as Table; if (parentTable != null) { parentTable.HasRowSections = true; } } } } } ///Indicates the horizontal alignment of the content within the table cells. ////// [ WebCategory("Layout"), DefaultValue(VerticalAlign.NotSet), WebSysDescription(SR.TableItem_VerticalAlign) ] public virtual VerticalAlign VerticalAlign { get { if (ControlStyleCreated == false) { return VerticalAlign.NotSet; } return ((TableItemStyle)ControlStyle).VerticalAlign; } set { ((TableItemStyle)ControlStyle).VerticalAlign = value; } } ///Indicates the vertical alignment of the content within the cell. ////// /// protected override Style CreateControlStyle() { return new TableItemStyle(ViewState); } ///A protected method. Creates a table item control style. ////// protected override ControlCollection CreateControlCollection() { return new CellControlCollection(this); } ///[To be supplied.] ////// protected class CellControlCollection : ControlCollection { internal CellControlCollection (Control owner) : base(owner) { } ///[To be supplied.] ////// public override void Add(Control child) { if (child is TableCell) base.Add(child); else throw new ArgumentException(SR.GetString(SR.Cannot_Have_Children_Of_Type, "TableRow", child.GetType().Name.ToString(CultureInfo.InvariantCulture))); // throw an exception here } ///Adds the specified ///object to the collection. The new control is added /// to the end of the array. /// public override void AddAt(int index, Control child) { if (child is TableCell) base.AddAt(index, child); else throw new ArgumentException(SR.GetString(SR.Cannot_Have_Children_Of_Type, "TableRow", child.GetType().Name.ToString(CultureInfo.InvariantCulture))); // throw an exception here } } // class CellControlCollection } }Adds the specified ///object to the collection. The new control is added /// to the array at the specified index location.
Link Menu
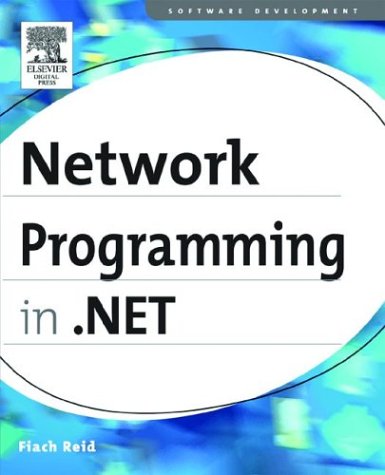
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataGridViewCellFormattingEventArgs.cs
- Enum.cs
- COAUTHINFO.cs
- DeleteMemberBinder.cs
- Policy.cs
- DeferredSelectedIndexReference.cs
- MarkupExtensionParser.cs
- StylusCaptureWithinProperty.cs
- RemotingService.cs
- ElementAction.cs
- ToolStripSeparator.cs
- XmlNodeList.cs
- ListControl.cs
- InkCanvasAutomationPeer.cs
- Buffer.cs
- coordinatorscratchpad.cs
- SHA384.cs
- SchemaInfo.cs
- OleDbStruct.cs
- datacache.cs
- CodeObject.cs
- BrowserDefinition.cs
- WebDescriptionAttribute.cs
- AlignmentYValidation.cs
- DmlSqlGenerator.cs
- PipelineModuleStepContainer.cs
- WebPartConnectionsEventArgs.cs
- KeyManager.cs
- BitmapEffectvisualstate.cs
- KeyValuePair.cs
- IisTraceWebEventProvider.cs
- WindowsListView.cs
- AttributeEmitter.cs
- DataBoundControlAdapter.cs
- Accessible.cs
- AncestorChangedEventArgs.cs
- DataGridItem.cs
- TextElementAutomationPeer.cs
- _BasicClient.cs
- SecUtil.cs
- CommonGetThemePartSize.cs
- TdsParserStateObject.cs
- DataControlButton.cs
- FilteredDataSetHelper.cs
- QueryCacheKey.cs
- RtfToken.cs
- Image.cs
- XamlSerializer.cs
- ProfileService.cs
- TransformedBitmap.cs
- Property.cs
- DataObjectCopyingEventArgs.cs
- Pair.cs
- TextEncodedRawTextWriter.cs
- GridViewUpdatedEventArgs.cs
- HandledEventArgs.cs
- MethodAccessException.cs
- CodeEntryPointMethod.cs
- DependencyPropertyAttribute.cs
- ProtocolsSection.cs
- ISAPIApplicationHost.cs
- Schedule.cs
- WebPartManagerInternals.cs
- DeploymentSection.cs
- TextChangedEventArgs.cs
- TypeUsageBuilder.cs
- ReferenceTypeElement.cs
- WindowsGraphics2.cs
- AuthorizationSection.cs
- SmiRequestExecutor.cs
- InvokePatternIdentifiers.cs
- InnerItemCollectionView.cs
- DataTableMappingCollection.cs
- DbMetaDataColumnNames.cs
- IpcPort.cs
- NameValueSectionHandler.cs
- StoreItemCollection.cs
- KeysConverter.cs
- MobileTemplatedControlDesigner.cs
- XPathMultyIterator.cs
- ModelItem.cs
- Line.cs
- ManagedWndProcTracker.cs
- CharEntityEncoderFallback.cs
- relpropertyhelper.cs
- CryptoProvider.cs
- UserNamePasswordValidator.cs
- BoundsDrawingContextWalker.cs
- DiffuseMaterial.cs
- XmlEventCache.cs
- Camera.cs
- WebPartDisplayModeEventArgs.cs
- PageBuildProvider.cs
- CapabilitiesState.cs
- ScriptComponentDescriptor.cs
- CodeDomSerializerBase.cs
- ReferencedAssembly.cs
- UIAgentAsyncBeginRequest.cs
- cache.cs
- Rijndael.cs