Code:
/ FXUpdate3074 / FXUpdate3074 / 1.1 / DEVDIV / depot / DevDiv / releases / whidbey / QFE / ndp / fx / src / xsp / System / Web / UI / WebControls / DataControlField.cs / 1 / DataControlField.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls { using System; using System.Collections; using System.Collections.Specialized; using System.ComponentModel; using System.Drawing.Design; using System.Security.Permissions; ////// Creates a field and is the base class for all [ TypeConverterAttribute(typeof(ExpandableObjectConverter)), DefaultProperty("HeaderText") ] [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public abstract class DataControlField : IStateManager, IDataSourceViewSchemaAccessor { private TableItemStyle _itemStyle; private TableItemStyle _headerStyle; private TableItemStyle _footerStyle; private Style _controlStyle; private StateBag _statebag; private bool _trackViewState; private bool _sortingEnabled; private Control _control; private object _dataSourceViewSchema; internal event EventHandler FieldChanged; ///types. /// /// protected DataControlField() { _statebag = new StateBag(); _dataSourceViewSchema = null; } ///Initializes a new instance of the System.Web.UI.WebControls.Field class. ////// [ Localizable(true), WebCategory("Accessibility"), DefaultValue(""), WebSysDescription(SR.DataControlField_AccessibleHeaderText) ] public virtual string AccessibleHeaderText { get { object o = ViewState["AccessibleHeaderText"]; if (o != null) return(string)o; return String.Empty; } set { if (!String.Equals(value, ViewState["AccessibleHeaderText"])) { ViewState["AccessibleHeaderText"] = value; OnFieldChanged(); } } } ///Gets or sets the text rendered as the AbbreviatedText in some controls. ////// [ WebCategory("Styles"), DefaultValue(null), WebSysDescription(SR.DataControlField_ControlStyle), DesignerSerializationVisibility(DesignerSerializationVisibility.Content), PersistenceMode(PersistenceMode.InnerProperty) ] public Style ControlStyle { get { if (_controlStyle == null) { _controlStyle = new Style(); if (IsTrackingViewState) ((IStateManager)_controlStyle).TrackViewState(); } return _controlStyle; } } internal Style ControlStyleInternal { get { return _controlStyle; } } protected Control Control { get { return _control; } } ///Gets the style properties for the controls inside this field. ////// protected bool DesignMode { get { if (_control != null) { return _control.DesignMode; } return false; } } ///[To be supplied.] ////// [ WebCategory("Styles"), DefaultValue(null), WebSysDescription(SR.DataControlField_FooterStyle), DesignerSerializationVisibility(DesignerSerializationVisibility.Content), PersistenceMode(PersistenceMode.InnerProperty) ] public TableItemStyle FooterStyle { get { if (_footerStyle == null) { _footerStyle = new TableItemStyle(); if (IsTrackingViewState) ((IStateManager)_footerStyle).TrackViewState(); } return _footerStyle; } } ///Gets the style properties for the footer item. ////// internal TableItemStyle FooterStyleInternal { get { return _footerStyle; } } ////// [ Localizable(true), WebCategory("Appearance"), DefaultValue(""), WebSysDescription(SR.DataControlField_FooterText) ] public virtual string FooterText { get { object o = ViewState["FooterText"]; if (o != null) return(string)o; return String.Empty; } set { if (!String.Equals(value, ViewState["FooterText"])) { ViewState["FooterText"] = value; OnFieldChanged(); } } } ///Gets or sets the text displayed in the footer of the /// System.Web.UI.WebControls.Field. ////// [ WebCategory("Appearance"), DefaultValue(""), Editor("System.Web.UI.Design.ImageUrlEditor, " + AssemblyRef.SystemDesign, typeof(UITypeEditor)), UrlProperty(), WebSysDescription(SR.DataControlField_HeaderImageUrl) ] public virtual string HeaderImageUrl { get { object o = ViewState["HeaderImageUrl"]; if (o != null) return(string)o; return String.Empty; } set { if (!String.Equals(value, ViewState["HeaderImageUrl"])) { ViewState["HeaderImageUrl"] = value; OnFieldChanged(); } } } ///Gets or sets the URL reference to an image to display /// instead of text on the header of this System.Web.UI.WebControls.Field /// . ////// [ WebCategory("Styles"), DefaultValue(null), WebSysDescription(SR.DataControlField_HeaderStyle), DesignerSerializationVisibility(DesignerSerializationVisibility.Content), PersistenceMode(PersistenceMode.InnerProperty) ] public TableItemStyle HeaderStyle { get { if (_headerStyle == null) { _headerStyle = new TableItemStyle(); if (IsTrackingViewState) ((IStateManager)_headerStyle).TrackViewState(); } return _headerStyle; } } ///Gets the style properties for the header of the System.Web.UI.WebControls.Field. This property is read-only. ////// internal TableItemStyle HeaderStyleInternal { get { return _headerStyle; } } ////// [ Localizable(true), WebCategory("Appearance"), DefaultValue(""), WebSysDescription(SR.DataControlField_HeaderText) ] public virtual string HeaderText { get { object o = ViewState["HeaderText"]; if (o != null) return(string)o; return String.Empty; } set { if (!String.Equals(value, ViewState["HeaderText"])) { ViewState["HeaderText"] = value; OnFieldChanged(); } } } ///Gets or sets the text displayed in the header of the /// System.Web.UI.WebControls.Field. ////// [ WebCategory("Behavior"), DefaultValue(true), WebSysDescription(SR.DataControlField_InsertVisible) ] public virtual bool InsertVisible { get { object o = ViewState["InsertVisible"]; if (o != null) return (bool)o; return true; } set { object oldValue = ViewState["InsertVisible"]; if (oldValue == null || value != (bool)oldValue) { ViewState["InsertVisible"] = value; OnFieldChanged(); } } } ///Gets or sets whether the field is visible in Insert mode. Turn off for auto-gen'd db fields ////// [ WebCategory("Styles"), DefaultValue(null), WebSysDescription(SR.DataControlField_ItemStyle), DesignerSerializationVisibility(DesignerSerializationVisibility.Content), PersistenceMode(PersistenceMode.InnerProperty) ] public TableItemStyle ItemStyle { get { if (_itemStyle == null) { _itemStyle = new TableItemStyle(); if (IsTrackingViewState) ((IStateManager)_itemStyle).TrackViewState(); } return _itemStyle; } } ///Gets the style properties of an item within the System.Web.UI.WebControls.Field. This property is read-only. ////// internal TableItemStyle ItemStyleInternal { get { return _itemStyle; } } [ WebCategory("Behavior"), DefaultValue(true), WebSysDescription(SR.DataControlField_ShowHeader) ] public virtual bool ShowHeader { get { object o = ViewState["ShowHeader"]; if (o != null) { return (bool)o; } return true; } set { object oldValue = ViewState["ShowHeader"]; if (oldValue == null || (bool)oldValue != value) { ViewState["ShowHeader"] = value; OnFieldChanged(); } } } ////// [ WebCategory("Behavior"), DefaultValue(""), TypeConverter("System.Web.UI.Design.DataSourceViewSchemaConverter, " + AssemblyRef.SystemDesign), WebSysDescription(SR.DataControlField_SortExpression) ] public virtual string SortExpression { get { object o = ViewState["SortExpression"]; if (o != null) return(string)o; return String.Empty; } set { if (!String.Equals(value, ViewState["SortExpression"])) { ViewState["SortExpression"] = value; OnFieldChanged(); } } } ///Gets or sets the expression used when this field is used to sort the data source> by. ////// protected StateBag ViewState { get { return _statebag; } } ///Gets the statebag for the System.Web.UI.WebControls.Field. This property is read-only. ////// [ WebCategory("Behavior"), DefaultValue(true), WebSysDescription(SR.DataControlField_Visible) ] public bool Visible { get { object o = ViewState["Visible"]; if (o != null) return(bool)o; return true; } set { object oldValue = ViewState["Visible"]; if (oldValue == null || value != (bool)oldValue) { ViewState["Visible"] = value; OnFieldChanged(); } } } protected internal DataControlField CloneField() { DataControlField newField = CreateField(); CopyProperties(newField); return newField; } protected virtual void CopyProperties(DataControlField newField) { newField.AccessibleHeaderText = AccessibleHeaderText; newField.ControlStyle.CopyFrom(ControlStyle); newField.FooterStyle.CopyFrom(FooterStyle); newField.HeaderStyle.CopyFrom(HeaderStyle); newField.ItemStyle.CopyFrom(ItemStyle); newField.FooterText = FooterText; newField.HeaderImageUrl = HeaderImageUrl; newField.HeaderText = HeaderText; newField.InsertVisible = InsertVisible; newField.ShowHeader = ShowHeader; newField.SortExpression = SortExpression; newField.Visible = Visible; } protected abstract DataControlField CreateField(); ///Gets or sets a value to indicate whether the System.Web.UI.WebControls.Field is visible. ////// Extracts the value of the databound cell and inserts the value into the given dictionary /// public virtual void ExtractValuesFromCell(IOrderedDictionary dictionary, DataControlFieldCell cell, DataControlRowState rowState, bool includeReadOnly) { return; } ////// public virtual bool Initialize(bool sortingEnabled, Control control) { _sortingEnabled = sortingEnabled; _control = control; return false; } ////// public virtual void InitializeCell(DataControlFieldCell cell, DataControlCellType cellType, DataControlRowState rowState, int rowIndex) { switch (cellType) { case DataControlCellType.Header: { WebControl headerControl = null; string sortExpression = SortExpression; bool sortableHeader = (_sortingEnabled && sortExpression.Length > 0); string headerImageUrl = HeaderImageUrl; string headerText = HeaderText; if (headerImageUrl.Length != 0) { if (sortableHeader) { ImageButton sortButton; IPostBackContainer container = _control as IPostBackContainer; if (container != null) { sortButton = new DataControlImageButton(container); ((DataControlImageButton)sortButton).EnableCallback(null); // no command argument for the callback uses Sort } else { sortButton = new ImageButton(); } sortButton.ImageUrl = HeaderImageUrl; sortButton.CommandName = DataControlCommands.SortCommandName; sortButton.CommandArgument = sortExpression; if (!(sortButton is DataControlImageButton)) { sortButton.CausesValidation = false; } sortButton.AlternateText = headerText; headerControl = sortButton; } else { Image headerImage = new Image(); headerImage.ImageUrl = headerImageUrl; headerControl = headerImage; headerImage.AlternateText = headerText; } } else { if (sortableHeader) { LinkButton sortButton; IPostBackContainer container = _control as IPostBackContainer; if (container != null) { sortButton = new DataControlLinkButton(container); ((DataControlLinkButton)sortButton).EnableCallback(null); // no command argument for the callback uses Sort } else { sortButton = new LinkButton(); } sortButton.Text = headerText; sortButton.CommandName = DataControlCommands.SortCommandName; sortButton.CommandArgument = sortExpression; if (!(sortButton is DataControlLinkButton)) { sortButton.CausesValidation = false; } headerControl = sortButton; } else { if (headerText.Length == 0) { // the browser does not render table borders for cells with nothing // in their content, so we add a non-breaking space. headerText = " "; } cell.Text = headerText; } } if (headerControl != null) { cell.Controls.Add(headerControl); } } break; case DataControlCellType.Footer: { string footerText = FooterText; if (footerText.Length == 0) { // the browser does not render table borders for cells with nothing // in their content, so we add a non-breaking space. footerText = " "; } cell.Text = footerText; } break; } } ///Initializes a cell in the System.Web.UI.WebControls.Field. ////// protected bool IsTrackingViewState { get { return _trackViewState; } } ///Determines if the System.Web.UI.WebControls.Field is marked to save its state. ////// protected virtual void LoadViewState(object savedState) { if (savedState != null) { object[] myState = (object[])savedState; if (myState[0] != null) ((IStateManager)ViewState).LoadViewState(myState[0]); if (myState[1] != null) ((IStateManager)ItemStyle).LoadViewState(myState[1]); if (myState[2] != null) ((IStateManager)HeaderStyle).LoadViewState(myState[2]); if (myState[3] != null) ((IStateManager)FooterStyle).LoadViewState(myState[3]); } } ///Loads the state of the System.Web.UI.WebControls.Field. ////// protected virtual void OnFieldChanged() { if (FieldChanged != null) { FieldChanged(this, EventArgs.Empty); } } ///Raises the FieldChanged event for a System.Web.UI.WebControls.Field. ////// protected virtual object SaveViewState() { object propState = ((IStateManager)ViewState).SaveViewState(); object itemStyleState = (_itemStyle != null) ? ((IStateManager)_itemStyle).SaveViewState() : null; object headerStyleState = (_headerStyle != null) ? ((IStateManager)_headerStyle).SaveViewState() : null; object footerStyleState = (_footerStyle != null) ? ((IStateManager)_footerStyle).SaveViewState() : null; object controlStyleState = (_controlStyle != null) ? ((IStateManager)_controlStyle).SaveViewState() : null; if ((propState != null) || (itemStyleState != null) || (headerStyleState != null) || (footerStyleState != null) || (controlStyleState != null)) { return new object[5] { propState, itemStyleState, headerStyleState, footerStyleState, controlStyleState }; } return null; } internal void SetDirty() { _statebag.SetDirty(true); if (_itemStyle != null) { _itemStyle.SetDirty(); } if (_headerStyle != null) { _headerStyle.SetDirty(); } if (_footerStyle != null) { _footerStyle.SetDirty(); } if (_controlStyle != null) { _controlStyle.SetDirty(); } } ///Saves the current state of the System.Web.UI.WebControls.Field. ////// /// Return a textual representation of the column for UI-display purposes. /// public override string ToString() { string headerText = HeaderText.Trim(); return headerText.Length > 0 ? headerText : GetType().Name; } ////// protected virtual void TrackViewState() { _trackViewState = true; ((IStateManager)ViewState).TrackViewState(); if (_itemStyle != null) ((IStateManager)_itemStyle).TrackViewState(); if (_headerStyle != null) ((IStateManager)_headerStyle).TrackViewState(); if (_footerStyle != null) ((IStateManager)_footerStyle).TrackViewState(); if (_controlStyle != null) ((IStateManager)_controlStyle).TrackViewState(); } ///Marks the starting point to begin tracking and saving changes to the /// control as part of the control viewstate. ////// public virtual void ValidateSupportsCallback() { throw new NotSupportedException(SR.GetString(SR.DataControlField_CallbacksNotSupported, Control.ID)); } ///Override with an empty body if the field's controls all support callback. /// Otherwise, override and throw a useful error message about why the field can't support callbacks. ////// /// Return true if tracking state changes. /// bool IStateManager.IsTrackingViewState { get { return IsTrackingViewState; } } ////// /// Load previously saved state. /// void IStateManager.LoadViewState(object state) { LoadViewState(state); } ////// /// Start tracking state changes. /// void IStateManager.TrackViewState() { TrackViewState(); } ////// /// Return object containing state changes. /// object IStateManager.SaveViewState() { return SaveViewState(); } #region IDataSourceViewSchemaAccessor implementation ///object IDataSourceViewSchemaAccessor.DataSourceViewSchema { get { return _dataSourceViewSchema; } set { _dataSourceViewSchema = value; } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls { using System; using System.Collections; using System.Collections.Specialized; using System.ComponentModel; using System.Drawing.Design; using System.Security.Permissions; ////// Creates a field and is the base class for all [ TypeConverterAttribute(typeof(ExpandableObjectConverter)), DefaultProperty("HeaderText") ] [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public abstract class DataControlField : IStateManager, IDataSourceViewSchemaAccessor { private TableItemStyle _itemStyle; private TableItemStyle _headerStyle; private TableItemStyle _footerStyle; private Style _controlStyle; private StateBag _statebag; private bool _trackViewState; private bool _sortingEnabled; private Control _control; private object _dataSourceViewSchema; internal event EventHandler FieldChanged; ///types. /// /// protected DataControlField() { _statebag = new StateBag(); _dataSourceViewSchema = null; } ///Initializes a new instance of the System.Web.UI.WebControls.Field class. ////// [ Localizable(true), WebCategory("Accessibility"), DefaultValue(""), WebSysDescription(SR.DataControlField_AccessibleHeaderText) ] public virtual string AccessibleHeaderText { get { object o = ViewState["AccessibleHeaderText"]; if (o != null) return(string)o; return String.Empty; } set { if (!String.Equals(value, ViewState["AccessibleHeaderText"])) { ViewState["AccessibleHeaderText"] = value; OnFieldChanged(); } } } ///Gets or sets the text rendered as the AbbreviatedText in some controls. ////// [ WebCategory("Styles"), DefaultValue(null), WebSysDescription(SR.DataControlField_ControlStyle), DesignerSerializationVisibility(DesignerSerializationVisibility.Content), PersistenceMode(PersistenceMode.InnerProperty) ] public Style ControlStyle { get { if (_controlStyle == null) { _controlStyle = new Style(); if (IsTrackingViewState) ((IStateManager)_controlStyle).TrackViewState(); } return _controlStyle; } } internal Style ControlStyleInternal { get { return _controlStyle; } } protected Control Control { get { return _control; } } ///Gets the style properties for the controls inside this field. ////// protected bool DesignMode { get { if (_control != null) { return _control.DesignMode; } return false; } } ///[To be supplied.] ////// [ WebCategory("Styles"), DefaultValue(null), WebSysDescription(SR.DataControlField_FooterStyle), DesignerSerializationVisibility(DesignerSerializationVisibility.Content), PersistenceMode(PersistenceMode.InnerProperty) ] public TableItemStyle FooterStyle { get { if (_footerStyle == null) { _footerStyle = new TableItemStyle(); if (IsTrackingViewState) ((IStateManager)_footerStyle).TrackViewState(); } return _footerStyle; } } ///Gets the style properties for the footer item. ////// internal TableItemStyle FooterStyleInternal { get { return _footerStyle; } } ////// [ Localizable(true), WebCategory("Appearance"), DefaultValue(""), WebSysDescription(SR.DataControlField_FooterText) ] public virtual string FooterText { get { object o = ViewState["FooterText"]; if (o != null) return(string)o; return String.Empty; } set { if (!String.Equals(value, ViewState["FooterText"])) { ViewState["FooterText"] = value; OnFieldChanged(); } } } ///Gets or sets the text displayed in the footer of the /// System.Web.UI.WebControls.Field. ////// [ WebCategory("Appearance"), DefaultValue(""), Editor("System.Web.UI.Design.ImageUrlEditor, " + AssemblyRef.SystemDesign, typeof(UITypeEditor)), UrlProperty(), WebSysDescription(SR.DataControlField_HeaderImageUrl) ] public virtual string HeaderImageUrl { get { object o = ViewState["HeaderImageUrl"]; if (o != null) return(string)o; return String.Empty; } set { if (!String.Equals(value, ViewState["HeaderImageUrl"])) { ViewState["HeaderImageUrl"] = value; OnFieldChanged(); } } } ///Gets or sets the URL reference to an image to display /// instead of text on the header of this System.Web.UI.WebControls.Field /// . ////// [ WebCategory("Styles"), DefaultValue(null), WebSysDescription(SR.DataControlField_HeaderStyle), DesignerSerializationVisibility(DesignerSerializationVisibility.Content), PersistenceMode(PersistenceMode.InnerProperty) ] public TableItemStyle HeaderStyle { get { if (_headerStyle == null) { _headerStyle = new TableItemStyle(); if (IsTrackingViewState) ((IStateManager)_headerStyle).TrackViewState(); } return _headerStyle; } } ///Gets the style properties for the header of the System.Web.UI.WebControls.Field. This property is read-only. ////// internal TableItemStyle HeaderStyleInternal { get { return _headerStyle; } } ////// [ Localizable(true), WebCategory("Appearance"), DefaultValue(""), WebSysDescription(SR.DataControlField_HeaderText) ] public virtual string HeaderText { get { object o = ViewState["HeaderText"]; if (o != null) return(string)o; return String.Empty; } set { if (!String.Equals(value, ViewState["HeaderText"])) { ViewState["HeaderText"] = value; OnFieldChanged(); } } } ///Gets or sets the text displayed in the header of the /// System.Web.UI.WebControls.Field. ////// [ WebCategory("Behavior"), DefaultValue(true), WebSysDescription(SR.DataControlField_InsertVisible) ] public virtual bool InsertVisible { get { object o = ViewState["InsertVisible"]; if (o != null) return (bool)o; return true; } set { object oldValue = ViewState["InsertVisible"]; if (oldValue == null || value != (bool)oldValue) { ViewState["InsertVisible"] = value; OnFieldChanged(); } } } ///Gets or sets whether the field is visible in Insert mode. Turn off for auto-gen'd db fields ////// [ WebCategory("Styles"), DefaultValue(null), WebSysDescription(SR.DataControlField_ItemStyle), DesignerSerializationVisibility(DesignerSerializationVisibility.Content), PersistenceMode(PersistenceMode.InnerProperty) ] public TableItemStyle ItemStyle { get { if (_itemStyle == null) { _itemStyle = new TableItemStyle(); if (IsTrackingViewState) ((IStateManager)_itemStyle).TrackViewState(); } return _itemStyle; } } ///Gets the style properties of an item within the System.Web.UI.WebControls.Field. This property is read-only. ////// internal TableItemStyle ItemStyleInternal { get { return _itemStyle; } } [ WebCategory("Behavior"), DefaultValue(true), WebSysDescription(SR.DataControlField_ShowHeader) ] public virtual bool ShowHeader { get { object o = ViewState["ShowHeader"]; if (o != null) { return (bool)o; } return true; } set { object oldValue = ViewState["ShowHeader"]; if (oldValue == null || (bool)oldValue != value) { ViewState["ShowHeader"] = value; OnFieldChanged(); } } } ////// [ WebCategory("Behavior"), DefaultValue(""), TypeConverter("System.Web.UI.Design.DataSourceViewSchemaConverter, " + AssemblyRef.SystemDesign), WebSysDescription(SR.DataControlField_SortExpression) ] public virtual string SortExpression { get { object o = ViewState["SortExpression"]; if (o != null) return(string)o; return String.Empty; } set { if (!String.Equals(value, ViewState["SortExpression"])) { ViewState["SortExpression"] = value; OnFieldChanged(); } } } ///Gets or sets the expression used when this field is used to sort the data source> by. ////// protected StateBag ViewState { get { return _statebag; } } ///Gets the statebag for the System.Web.UI.WebControls.Field. This property is read-only. ////// [ WebCategory("Behavior"), DefaultValue(true), WebSysDescription(SR.DataControlField_Visible) ] public bool Visible { get { object o = ViewState["Visible"]; if (o != null) return(bool)o; return true; } set { object oldValue = ViewState["Visible"]; if (oldValue == null || value != (bool)oldValue) { ViewState["Visible"] = value; OnFieldChanged(); } } } protected internal DataControlField CloneField() { DataControlField newField = CreateField(); CopyProperties(newField); return newField; } protected virtual void CopyProperties(DataControlField newField) { newField.AccessibleHeaderText = AccessibleHeaderText; newField.ControlStyle.CopyFrom(ControlStyle); newField.FooterStyle.CopyFrom(FooterStyle); newField.HeaderStyle.CopyFrom(HeaderStyle); newField.ItemStyle.CopyFrom(ItemStyle); newField.FooterText = FooterText; newField.HeaderImageUrl = HeaderImageUrl; newField.HeaderText = HeaderText; newField.InsertVisible = InsertVisible; newField.ShowHeader = ShowHeader; newField.SortExpression = SortExpression; newField.Visible = Visible; } protected abstract DataControlField CreateField(); ///Gets or sets a value to indicate whether the System.Web.UI.WebControls.Field is visible. ////// Extracts the value of the databound cell and inserts the value into the given dictionary /// public virtual void ExtractValuesFromCell(IOrderedDictionary dictionary, DataControlFieldCell cell, DataControlRowState rowState, bool includeReadOnly) { return; } ////// public virtual bool Initialize(bool sortingEnabled, Control control) { _sortingEnabled = sortingEnabled; _control = control; return false; } ////// public virtual void InitializeCell(DataControlFieldCell cell, DataControlCellType cellType, DataControlRowState rowState, int rowIndex) { switch (cellType) { case DataControlCellType.Header: { WebControl headerControl = null; string sortExpression = SortExpression; bool sortableHeader = (_sortingEnabled && sortExpression.Length > 0); string headerImageUrl = HeaderImageUrl; string headerText = HeaderText; if (headerImageUrl.Length != 0) { if (sortableHeader) { ImageButton sortButton; IPostBackContainer container = _control as IPostBackContainer; if (container != null) { sortButton = new DataControlImageButton(container); ((DataControlImageButton)sortButton).EnableCallback(null); // no command argument for the callback uses Sort } else { sortButton = new ImageButton(); } sortButton.ImageUrl = HeaderImageUrl; sortButton.CommandName = DataControlCommands.SortCommandName; sortButton.CommandArgument = sortExpression; if (!(sortButton is DataControlImageButton)) { sortButton.CausesValidation = false; } sortButton.AlternateText = headerText; headerControl = sortButton; } else { Image headerImage = new Image(); headerImage.ImageUrl = headerImageUrl; headerControl = headerImage; headerImage.AlternateText = headerText; } } else { if (sortableHeader) { LinkButton sortButton; IPostBackContainer container = _control as IPostBackContainer; if (container != null) { sortButton = new DataControlLinkButton(container); ((DataControlLinkButton)sortButton).EnableCallback(null); // no command argument for the callback uses Sort } else { sortButton = new LinkButton(); } sortButton.Text = headerText; sortButton.CommandName = DataControlCommands.SortCommandName; sortButton.CommandArgument = sortExpression; if (!(sortButton is DataControlLinkButton)) { sortButton.CausesValidation = false; } headerControl = sortButton; } else { if (headerText.Length == 0) { // the browser does not render table borders for cells with nothing // in their content, so we add a non-breaking space. headerText = " "; } cell.Text = headerText; } } if (headerControl != null) { cell.Controls.Add(headerControl); } } break; case DataControlCellType.Footer: { string footerText = FooterText; if (footerText.Length == 0) { // the browser does not render table borders for cells with nothing // in their content, so we add a non-breaking space. footerText = " "; } cell.Text = footerText; } break; } } ///Initializes a cell in the System.Web.UI.WebControls.Field. ////// protected bool IsTrackingViewState { get { return _trackViewState; } } ///Determines if the System.Web.UI.WebControls.Field is marked to save its state. ////// protected virtual void LoadViewState(object savedState) { if (savedState != null) { object[] myState = (object[])savedState; if (myState[0] != null) ((IStateManager)ViewState).LoadViewState(myState[0]); if (myState[1] != null) ((IStateManager)ItemStyle).LoadViewState(myState[1]); if (myState[2] != null) ((IStateManager)HeaderStyle).LoadViewState(myState[2]); if (myState[3] != null) ((IStateManager)FooterStyle).LoadViewState(myState[3]); } } ///Loads the state of the System.Web.UI.WebControls.Field. ////// protected virtual void OnFieldChanged() { if (FieldChanged != null) { FieldChanged(this, EventArgs.Empty); } } ///Raises the FieldChanged event for a System.Web.UI.WebControls.Field. ////// protected virtual object SaveViewState() { object propState = ((IStateManager)ViewState).SaveViewState(); object itemStyleState = (_itemStyle != null) ? ((IStateManager)_itemStyle).SaveViewState() : null; object headerStyleState = (_headerStyle != null) ? ((IStateManager)_headerStyle).SaveViewState() : null; object footerStyleState = (_footerStyle != null) ? ((IStateManager)_footerStyle).SaveViewState() : null; object controlStyleState = (_controlStyle != null) ? ((IStateManager)_controlStyle).SaveViewState() : null; if ((propState != null) || (itemStyleState != null) || (headerStyleState != null) || (footerStyleState != null) || (controlStyleState != null)) { return new object[5] { propState, itemStyleState, headerStyleState, footerStyleState, controlStyleState }; } return null; } internal void SetDirty() { _statebag.SetDirty(true); if (_itemStyle != null) { _itemStyle.SetDirty(); } if (_headerStyle != null) { _headerStyle.SetDirty(); } if (_footerStyle != null) { _footerStyle.SetDirty(); } if (_controlStyle != null) { _controlStyle.SetDirty(); } } ///Saves the current state of the System.Web.UI.WebControls.Field. ////// /// Return a textual representation of the column for UI-display purposes. /// public override string ToString() { string headerText = HeaderText.Trim(); return headerText.Length > 0 ? headerText : GetType().Name; } ////// protected virtual void TrackViewState() { _trackViewState = true; ((IStateManager)ViewState).TrackViewState(); if (_itemStyle != null) ((IStateManager)_itemStyle).TrackViewState(); if (_headerStyle != null) ((IStateManager)_headerStyle).TrackViewState(); if (_footerStyle != null) ((IStateManager)_footerStyle).TrackViewState(); if (_controlStyle != null) ((IStateManager)_controlStyle).TrackViewState(); } ///Marks the starting point to begin tracking and saving changes to the /// control as part of the control viewstate. ////// public virtual void ValidateSupportsCallback() { throw new NotSupportedException(SR.GetString(SR.DataControlField_CallbacksNotSupported, Control.ID)); } ///Override with an empty body if the field's controls all support callback. /// Otherwise, override and throw a useful error message about why the field can't support callbacks. ////// /// Return true if tracking state changes. /// bool IStateManager.IsTrackingViewState { get { return IsTrackingViewState; } } ////// /// Load previously saved state. /// void IStateManager.LoadViewState(object state) { LoadViewState(state); } ////// /// Start tracking state changes. /// void IStateManager.TrackViewState() { TrackViewState(); } ////// /// Return object containing state changes. /// object IStateManager.SaveViewState() { return SaveViewState(); } #region IDataSourceViewSchemaAccessor implementation ///object IDataSourceViewSchemaAccessor.DataSourceViewSchema { get { return _dataSourceViewSchema; } set { _dataSourceViewSchema = value; } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
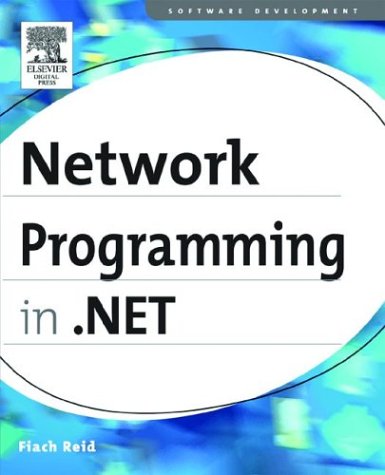
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CacheOutputQuery.cs
- DefaultWorkflowSchedulerService.cs
- DayRenderEvent.cs
- UIElement3D.cs
- RotateTransform.cs
- WmlListAdapter.cs
- InternalRelationshipCollection.cs
- CacheVirtualItemsEvent.cs
- BuildProvider.cs
- UnsafeNativeMethods.cs
- SplineKeyFrames.cs
- StateMachineHelpers.cs
- GradientStopCollection.cs
- ResourceContainer.cs
- TextElementEnumerator.cs
- SchemaImporterExtensionsSection.cs
- VerticalAlignConverter.cs
- RowSpanVector.cs
- AtomicFile.cs
- ExecutionEngineException.cs
- SchemaObjectWriter.cs
- TextRangeAdaptor.cs
- base64Transforms.cs
- SessionStateModule.cs
- WebPartTransformerCollection.cs
- ContentDisposition.cs
- AdvancedBindingPropertyDescriptor.cs
- HttpCapabilitiesBase.cs
- AbandonedMutexException.cs
- ServicesUtilities.cs
- TrackingCondition.cs
- DataGridViewEditingControlShowingEventArgs.cs
- DataSourceXmlSerializationAttribute.cs
- EncodingDataItem.cs
- PasswordTextContainer.cs
- backend.cs
- ContextMarshalException.cs
- Math.cs
- isolationinterop.cs
- ItemList.cs
- _SafeNetHandles.cs
- DiagnosticsConfigurationHandler.cs
- XamlUtilities.cs
- DiagnosticTraceRecords.cs
- TextRangeEditLists.cs
- RotateTransform3D.cs
- Padding.cs
- FormsAuthenticationUserCollection.cs
- Condition.cs
- EnumerableRowCollection.cs
- FixedSOMTableRow.cs
- Errors.cs
- CodeIterationStatement.cs
- TypeUtil.cs
- SchemaDeclBase.cs
- DocumentXPathNavigator.cs
- AnimationStorage.cs
- Byte.cs
- InkCanvasSelectionAdorner.cs
- SHA1CryptoServiceProvider.cs
- RecognizedWordUnit.cs
- XPathConvert.cs
- ParamArrayAttribute.cs
- SupportsPreviewControlAttribute.cs
- BaseValidator.cs
- OdbcConnectionString.cs
- ObjectQuery_EntitySqlExtensions.cs
- DataGridViewColumnDesigner.cs
- indexingfiltermarshaler.cs
- HMACRIPEMD160.cs
- PictureBoxDesigner.cs
- PropertyDescriptorGridEntry.cs
- TextServicesProperty.cs
- IncrementalHitTester.cs
- ListViewItem.cs
- SearchExpression.cs
- DetailsViewUpdateEventArgs.cs
- HtmlTernaryTree.cs
- ComboBoxItem.cs
- Range.cs
- IpcClientManager.cs
- EntityDataSourceDesignerHelper.cs
- BooleanConverter.cs
- RegexInterpreter.cs
- ComponentCollection.cs
- SocketPermission.cs
- Regex.cs
- SectionInput.cs
- DbProviderFactory.cs
- CannotUnloadAppDomainException.cs
- LayoutTableCell.cs
- MemberDomainMap.cs
- Simplifier.cs
- basecomparevalidator.cs
- Rect3D.cs
- ToolboxItemCollection.cs
- IUnknownConstantAttribute.cs
- loginstatus.cs
- HierarchicalDataBoundControlAdapter.cs
- ArrangedElement.cs