Code:
/ FXUpdate3074 / FXUpdate3074 / 1.1 / DEVDIV / depot / DevDiv / releases / whidbey / QFE / ndp / fx / src / xsp / System / Web / UI / WebControls / PanelStyle.cs / 1 / PanelStyle.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls { using System; using System.Collections; using System.ComponentModel; using System.Globalization; using System.Reflection; using System.Web; using System.Web.UI; using System.Security.Permissions; ////// [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public class PanelStyle : Style { // !! private const int PROP_BACKIMAGEURL = 0x00010000; private const int PROP_DIRECTION = 0x00020000; private const int PROP_HORIZONTALALIGN = 0x00040000; private const int PROP_SCROLLBARS = 0x00080000; private const int PROP_WRAP = 0x00100000; private const string STR_BACKIMAGEURL = "BackImageUrl"; private const string STR_DIRECTION = "Direction"; private const string STR_HORIZONTALALIGN = "HorizontalAlign"; private const string STR_SCROLLBARS = "ScrollBars"; private const string STR_WRAP = "Wrap"; ///Specifies the style of the panel. ////// public PanelStyle(StateBag bag) : base (bag) { } ////// Initializes a new instance of the ////// class with state bag information. /// /// [ WebCategory("Appearance"), DefaultValue(""), UrlProperty(), WebSysDescription(SR.Panel_BackImageUrl) ] public virtual string BackImageUrl { get { if (IsSet(PROP_BACKIMAGEURL)) { return(string)(ViewState[STR_BACKIMAGEURL]); } return String.Empty; } set { if (value == null) { throw new ArgumentNullException("value"); } ViewState[STR_BACKIMAGEURL] = value; SetBit(PROP_BACKIMAGEURL); } } ///Gets or sets the URL of the background image for the panel. ////// [ WebCategory("Appearance"), DefaultValue(""), WebSysDescription(SR.Panel_Direction) ] public virtual ContentDirection Direction { get { if (IsSet(PROP_DIRECTION)) { return (ContentDirection)(ViewState[STR_DIRECTION]); } return ContentDirection.NotSet; } set { if (value < ContentDirection.NotSet || value > ContentDirection.RightToLeft) { throw new ArgumentOutOfRangeException("value"); } ViewState[STR_DIRECTION] = value; SetBit(PROP_DIRECTION); } } ///Gets or sets content direction for the panel. ////// [ WebCategory("Appearance"), DefaultValue(""), WebSysDescription(SR.Panel_HorizontalAlign) ] public virtual HorizontalAlign HorizontalAlign { get { if (IsSet(PROP_HORIZONTALALIGN)) { return (HorizontalAlign)(ViewState[STR_HORIZONTALALIGN]); } return HorizontalAlign.NotSet; } set { if (value < HorizontalAlign.NotSet || value > HorizontalAlign.Justify) { throw new ArgumentOutOfRangeException("value"); } ViewState[STR_HORIZONTALALIGN] = value; SetBit(PROP_HORIZONTALALIGN); } } ///Gets or sets the horizontal alignment of the contents within the panel. ////// [ WebCategory("Appearance"), DefaultValue(""), WebSysDescription(SR.Panel_ScrollBars) ] public virtual ScrollBars ScrollBars { get { if (IsSet(PROP_SCROLLBARS)) { return (ScrollBars)(ViewState[STR_SCROLLBARS]); } return ScrollBars.None; } set { if (value < ScrollBars.None || value > ScrollBars.Auto) { throw new ArgumentOutOfRangeException("value"); } ViewState[STR_SCROLLBARS] = value; SetBit(PROP_SCROLLBARS); } } ///Gets or sets the scrollbar behavior of the panel. ////// [ WebCategory("Appearance"), DefaultValue(""), WebSysDescription(SR.Panel_Wrap) ] public virtual bool Wrap { get { if (IsSet(PROP_WRAP)) { return (bool)(ViewState[STR_WRAP]); } return true; } set { ViewState[STR_WRAP] = value; SetBit(PROP_WRAP); } } ///Gets or sets a value /// indicating whether the content wraps within the panel. ////// /// public override void CopyFrom(Style s) { if (s != null && !s.IsEmpty) { base.CopyFrom(s); if (s is PanelStyle) { PanelStyle ts = (PanelStyle)s; if (s.RegisteredCssClass.Length != 0) { if (ts.IsSet(PROP_BACKIMAGEURL)) { ViewState.Remove(STR_BACKIMAGEURL); ClearBit(PROP_BACKIMAGEURL); } if (ts.IsSet(PROP_SCROLLBARS)) { ViewState.Remove(STR_SCROLLBARS); ClearBit(PROP_SCROLLBARS); } if (ts.IsSet(PROP_WRAP)) { ViewState.Remove(STR_WRAP); ClearBit(PROP_WRAP); } } else { if (ts.IsSet(PROP_BACKIMAGEURL)) this.BackImageUrl = ts.BackImageUrl; if (ts.IsSet(PROP_SCROLLBARS)) this.ScrollBars = ts.ScrollBars; if (ts.IsSet(PROP_WRAP)) this.Wrap = ts.Wrap; } if (ts.IsSet(PROP_DIRECTION)) this.Direction = ts.Direction; if (ts.IsSet(PROP_HORIZONTALALIGN)) this.HorizontalAlign = ts.HorizontalAlign; } } } ///Copies non-blank elements from the specified style, overwriting existing /// style elements if necessary. ////// /// public override void MergeWith(Style s) { if (s != null && !s.IsEmpty) { if (IsEmpty) { // merge into an empty style is equivalent to a copy, // which is more efficient CopyFrom(s); return; } base.MergeWith(s); if (s is PanelStyle) { PanelStyle ts = (PanelStyle)s; // Since we're already copying the registered CSS class in base.MergeWith, we don't // need to any attributes that would be included in that class. if (s.RegisteredCssClass.Length == 0) { if (ts.IsSet(PROP_BACKIMAGEURL) && !this.IsSet(PROP_BACKIMAGEURL)) this.BackImageUrl = ts.BackImageUrl; if (ts.IsSet(PROP_SCROLLBARS) && !this.IsSet(PROP_SCROLLBARS)) this.ScrollBars = ts.ScrollBars; if (ts.IsSet(PROP_WRAP) && !this.IsSet(PROP_WRAP)) this.Wrap = ts.Wrap; } if (ts.IsSet(PROP_DIRECTION) && !this.IsSet(PROP_DIRECTION)) this.Direction = ts.Direction; if (ts.IsSet(PROP_HORIZONTALALIGN) && !this.IsSet(PROP_HORIZONTALALIGN)) this.HorizontalAlign = ts.HorizontalAlign; } } } ///Copies non-blank elements from the specified style, but will not overwrite /// any existing style elements. ////// /// public override void Reset() { if (IsSet(PROP_BACKIMAGEURL)) ViewState.Remove(STR_BACKIMAGEURL); if (IsSet(PROP_DIRECTION)) ViewState.Remove(STR_DIRECTION); if (IsSet(PROP_HORIZONTALALIGN)) ViewState.Remove(STR_HORIZONTALALIGN); if (IsSet(PROP_SCROLLBARS)) ViewState.Remove(STR_SCROLLBARS); if (IsSet(PROP_WRAP)) ViewState.Remove(STR_WRAP); base.Reset(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ //Clears out any defined style elements from the state bag. ///// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls { using System; using System.Collections; using System.ComponentModel; using System.Globalization; using System.Reflection; using System.Web; using System.Web.UI; using System.Security.Permissions; ////// [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public class PanelStyle : Style { // !! private const int PROP_BACKIMAGEURL = 0x00010000; private const int PROP_DIRECTION = 0x00020000; private const int PROP_HORIZONTALALIGN = 0x00040000; private const int PROP_SCROLLBARS = 0x00080000; private const int PROP_WRAP = 0x00100000; private const string STR_BACKIMAGEURL = "BackImageUrl"; private const string STR_DIRECTION = "Direction"; private const string STR_HORIZONTALALIGN = "HorizontalAlign"; private const string STR_SCROLLBARS = "ScrollBars"; private const string STR_WRAP = "Wrap"; ///Specifies the style of the panel. ////// public PanelStyle(StateBag bag) : base (bag) { } ////// Initializes a new instance of the ////// class with state bag information. /// /// [ WebCategory("Appearance"), DefaultValue(""), UrlProperty(), WebSysDescription(SR.Panel_BackImageUrl) ] public virtual string BackImageUrl { get { if (IsSet(PROP_BACKIMAGEURL)) { return(string)(ViewState[STR_BACKIMAGEURL]); } return String.Empty; } set { if (value == null) { throw new ArgumentNullException("value"); } ViewState[STR_BACKIMAGEURL] = value; SetBit(PROP_BACKIMAGEURL); } } ///Gets or sets the URL of the background image for the panel. ////// [ WebCategory("Appearance"), DefaultValue(""), WebSysDescription(SR.Panel_Direction) ] public virtual ContentDirection Direction { get { if (IsSet(PROP_DIRECTION)) { return (ContentDirection)(ViewState[STR_DIRECTION]); } return ContentDirection.NotSet; } set { if (value < ContentDirection.NotSet || value > ContentDirection.RightToLeft) { throw new ArgumentOutOfRangeException("value"); } ViewState[STR_DIRECTION] = value; SetBit(PROP_DIRECTION); } } ///Gets or sets content direction for the panel. ////// [ WebCategory("Appearance"), DefaultValue(""), WebSysDescription(SR.Panel_HorizontalAlign) ] public virtual HorizontalAlign HorizontalAlign { get { if (IsSet(PROP_HORIZONTALALIGN)) { return (HorizontalAlign)(ViewState[STR_HORIZONTALALIGN]); } return HorizontalAlign.NotSet; } set { if (value < HorizontalAlign.NotSet || value > HorizontalAlign.Justify) { throw new ArgumentOutOfRangeException("value"); } ViewState[STR_HORIZONTALALIGN] = value; SetBit(PROP_HORIZONTALALIGN); } } ///Gets or sets the horizontal alignment of the contents within the panel. ////// [ WebCategory("Appearance"), DefaultValue(""), WebSysDescription(SR.Panel_ScrollBars) ] public virtual ScrollBars ScrollBars { get { if (IsSet(PROP_SCROLLBARS)) { return (ScrollBars)(ViewState[STR_SCROLLBARS]); } return ScrollBars.None; } set { if (value < ScrollBars.None || value > ScrollBars.Auto) { throw new ArgumentOutOfRangeException("value"); } ViewState[STR_SCROLLBARS] = value; SetBit(PROP_SCROLLBARS); } } ///Gets or sets the scrollbar behavior of the panel. ////// [ WebCategory("Appearance"), DefaultValue(""), WebSysDescription(SR.Panel_Wrap) ] public virtual bool Wrap { get { if (IsSet(PROP_WRAP)) { return (bool)(ViewState[STR_WRAP]); } return true; } set { ViewState[STR_WRAP] = value; SetBit(PROP_WRAP); } } ///Gets or sets a value /// indicating whether the content wraps within the panel. ////// /// public override void CopyFrom(Style s) { if (s != null && !s.IsEmpty) { base.CopyFrom(s); if (s is PanelStyle) { PanelStyle ts = (PanelStyle)s; if (s.RegisteredCssClass.Length != 0) { if (ts.IsSet(PROP_BACKIMAGEURL)) { ViewState.Remove(STR_BACKIMAGEURL); ClearBit(PROP_BACKIMAGEURL); } if (ts.IsSet(PROP_SCROLLBARS)) { ViewState.Remove(STR_SCROLLBARS); ClearBit(PROP_SCROLLBARS); } if (ts.IsSet(PROP_WRAP)) { ViewState.Remove(STR_WRAP); ClearBit(PROP_WRAP); } } else { if (ts.IsSet(PROP_BACKIMAGEURL)) this.BackImageUrl = ts.BackImageUrl; if (ts.IsSet(PROP_SCROLLBARS)) this.ScrollBars = ts.ScrollBars; if (ts.IsSet(PROP_WRAP)) this.Wrap = ts.Wrap; } if (ts.IsSet(PROP_DIRECTION)) this.Direction = ts.Direction; if (ts.IsSet(PROP_HORIZONTALALIGN)) this.HorizontalAlign = ts.HorizontalAlign; } } } ///Copies non-blank elements from the specified style, overwriting existing /// style elements if necessary. ////// /// public override void MergeWith(Style s) { if (s != null && !s.IsEmpty) { if (IsEmpty) { // merge into an empty style is equivalent to a copy, // which is more efficient CopyFrom(s); return; } base.MergeWith(s); if (s is PanelStyle) { PanelStyle ts = (PanelStyle)s; // Since we're already copying the registered CSS class in base.MergeWith, we don't // need to any attributes that would be included in that class. if (s.RegisteredCssClass.Length == 0) { if (ts.IsSet(PROP_BACKIMAGEURL) && !this.IsSet(PROP_BACKIMAGEURL)) this.BackImageUrl = ts.BackImageUrl; if (ts.IsSet(PROP_SCROLLBARS) && !this.IsSet(PROP_SCROLLBARS)) this.ScrollBars = ts.ScrollBars; if (ts.IsSet(PROP_WRAP) && !this.IsSet(PROP_WRAP)) this.Wrap = ts.Wrap; } if (ts.IsSet(PROP_DIRECTION) && !this.IsSet(PROP_DIRECTION)) this.Direction = ts.Direction; if (ts.IsSet(PROP_HORIZONTALALIGN) && !this.IsSet(PROP_HORIZONTALALIGN)) this.HorizontalAlign = ts.HorizontalAlign; } } } ///Copies non-blank elements from the specified style, but will not overwrite /// any existing style elements. ////// /// public override void Reset() { if (IsSet(PROP_BACKIMAGEURL)) ViewState.Remove(STR_BACKIMAGEURL); if (IsSet(PROP_DIRECTION)) ViewState.Remove(STR_DIRECTION); if (IsSet(PROP_HORIZONTALALIGN)) ViewState.Remove(STR_HORIZONTALALIGN); if (IsSet(PROP_SCROLLBARS)) ViewState.Remove(STR_SCROLLBARS); if (IsSet(PROP_WRAP)) ViewState.Remove(STR_WRAP); base.Reset(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.Clears out any defined style elements from the state bag. ///
Link Menu
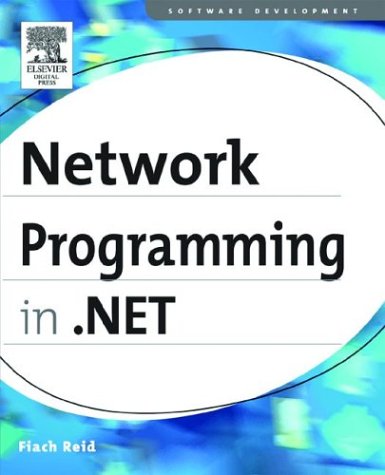
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- OleDbWrapper.cs
- ToolStripDropDownClosingEventArgs.cs
- errorpatternmatcher.cs
- MinimizableAttributeTypeConverter.cs
- SingleAnimationBase.cs
- WCFServiceClientProxyGenerator.cs
- RightsManagementEncryptionTransform.cs
- SystemIPGlobalProperties.cs
- RegexCompilationInfo.cs
- InputLanguageManager.cs
- WebPartsPersonalization.cs
- PersonalizationStateInfo.cs
- XmlNodeReader.cs
- DataBindingsDialog.cs
- MergeFailedEvent.cs
- WebContentFormatHelper.cs
- WebPartVerbCollection.cs
- UriTemplateClientFormatter.cs
- OutputBuffer.cs
- VirtualPathData.cs
- PrivilegeNotHeldException.cs
- SafeRightsManagementEnvironmentHandle.cs
- BamlVersionHeader.cs
- HttpServerChannel.cs
- RSAPKCS1KeyExchangeFormatter.cs
- FormViewInsertEventArgs.cs
- RecognizerInfo.cs
- SystemUnicastIPAddressInformation.cs
- diagnosticsswitches.cs
- EventWaitHandleSecurity.cs
- ListManagerBindingsCollection.cs
- DataGridViewColumnCollection.cs
- FilterUserControlBase.cs
- ObjectCloneHelper.cs
- SafeArrayTypeMismatchException.cs
- ListSortDescription.cs
- TransactionTable.cs
- BCLDebug.cs
- XmlSchemaRedefine.cs
- Rect.cs
- StructuredProperty.cs
- FastEncoder.cs
- XmlNamespaceDeclarationsAttribute.cs
- Normalization.cs
- ConstructorBuilder.cs
- CommonBehaviorsSection.cs
- FrugalMap.cs
- SQLConvert.cs
- ManualWorkflowSchedulerService.cs
- View.cs
- EpmSourcePathSegment.cs
- SQLDateTime.cs
- Cell.cs
- ListBoxItemWrapperAutomationPeer.cs
- ClientSession.cs
- TextTreePropertyUndoUnit.cs
- ArgumentNullException.cs
- MethodCallExpression.cs
- DrawItemEvent.cs
- GeneralTransform3DGroup.cs
- LinqDataSource.cs
- Pkcs7Recipient.cs
- FieldDescriptor.cs
- DataGridViewRowPostPaintEventArgs.cs
- TokenizerHelper.cs
- IdentityReference.cs
- Parameter.cs
- Material.cs
- SerializationException.cs
- TraceSection.cs
- StorageEntitySetMapping.cs
- ScrollBarAutomationPeer.cs
- DbFunctionCommandTree.cs
- TypeInformation.cs
- InvalidFilterCriteriaException.cs
- ComplexBindingPropertiesAttribute.cs
- TextEffect.cs
- XmlComplianceUtil.cs
- Input.cs
- InputScopeAttribute.cs
- StringBuilder.cs
- SqlDataSourceConfigureFilterForm.cs
- PolyBezierSegmentFigureLogic.cs
- FirstMatchCodeGroup.cs
- ScriptingProfileServiceSection.cs
- ListViewTableCell.cs
- OverrideMode.cs
- EmptyImpersonationContext.cs
- DocumentPaginator.cs
- SerializerWriterEventHandlers.cs
- CategoryAttribute.cs
- ProvidersHelper.cs
- NullableIntAverageAggregationOperator.cs
- DataControlFieldCollection.cs
- SpeechAudioFormatInfo.cs
- InheritanceAttribute.cs
- WindowsRegion.cs
- OleDbConnectionInternal.cs
- DocumentPaginator.cs
- WebPageTraceListener.cs