Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataEntity / System / Data / EntityModel / SchemaObjectModel / StructuredProperty.cs / 1305376 / StructuredProperty.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Collections.Specialized; using System.Data.Metadata.Edm; using System.Diagnostics; using System.Xml; using System.Data; using System.Reflection; using System.IO; using System.Globalization; namespace System.Data.EntityModel.SchemaObjectModel { ////// Summary description for StructuredProperty. /// internal class StructuredProperty : Property { #region Instance Fields private SchemaType _type = null; private string _unresolvedType = null; // Facets private TypeUsageBuilder _typeUsageBuilder; //Type of the Collection. By Default Single, and in case of Collections, will be either Bag or List private CollectionKind _collectionKind = CollectionKind.None; #endregion #region Static Fields //private static System.Text.RegularExpressions.Regex _binaryValueValidator = new System.Text.RegularExpressions.Regex("0[xX][0-9a-fA-F]+"); #endregion #region Public Methods ////// /// /// internal StructuredProperty(StructuredType parentElement) : base(parentElement) { _typeUsageBuilder = new TypeUsageBuilder(this); } #endregion #region Public Properties ////// /// public override SchemaType Type { get { return _type; } } ////// Returns a TypeUsage that represent this property. /// public TypeUsage TypeUsage { get { return _typeUsageBuilder.TypeUsage; } } ////// The nullablity of this property. /// public bool Nullable { get { return _typeUsageBuilder.Nullable; } } ////// /// public string Default { get { return _typeUsageBuilder.Default; } } ////// /// public object DefaultAsObject { get { return _typeUsageBuilder.DefaultAsObject; } } ////// Specifies the type of the Collection. /// By Default this is Single( i.e. not a Collection. /// And in case of Collections, will be either Bag or List /// public CollectionKind CollectionKind { get { return _collectionKind; } } #endregion #region Internal Methods ////// /// internal override void ResolveTopLevelNames() { base.ResolveTopLevelNames(); if (_type != null) { return; } _type = ResolveType(UnresolvedType); _typeUsageBuilder.ValidateDefaultValue(_type); ScalarType scalar = _type as ScalarType; if (scalar != null) { _typeUsageBuilder.ValidateAndSetTypeUsage(scalar, true); } } ////// Resolve the type string to a SchemaType object /// /// ///protected virtual SchemaType ResolveType(string typeName) { SchemaType element; if (!Schema.ResolveTypeName(this, typeName, out element)) { return null; } if (!(element is SchemaComplexType) && !(element is ScalarType)) { AddError(ErrorCode.InvalidPropertyType, EdmSchemaErrorSeverity.Error, System.Data.Entity.Strings.InvalidPropertyType(UnresolvedType)); return null; } SchemaType structuredType = element as SchemaType; return element; } #endregion #region Internal Properties /// /// /// ///internal string UnresolvedType { get { return _unresolvedType; } set { _unresolvedType = value; } } #endregion #region Protected Methods /// /// /// ///internal override void Validate() { base.Validate(); //Non Complex Collections are not supported if ((_collectionKind == CollectionKind.Bag) || (_collectionKind == CollectionKind.List)) { Debug.Assert(Schema.SchemaVersion != XmlConstants.EdmVersionForV1, "CollctionKind Attribute is not supported in EDM V1"); } //Nullable Complex Types are not supported in V 1.0 and V 2.0 if (Nullable && (this.Schema.SchemaVersion == XmlConstants.EdmVersionForV1 || this.Schema.SchemaVersion == XmlConstants.EdmVersionForV2) && (this._type is SchemaComplexType)) { AddError(ErrorCode.NullableComplexType, EdmSchemaErrorSeverity.Error, System.Data.Entity.Strings.NullableComplexType(this.FQName)); } } #endregion #region Protected Properties protected override bool HandleAttribute(XmlReader reader) { if (base.HandleAttribute(reader)) { return true; } else if (CanHandleAttribute(reader, XmlConstants.TypeElement)) { HandleTypeAttribute(reader); return true; } else if (CanHandleAttribute(reader, XmlConstants.CollectionKind)) { HandleCollectionKindAttribute(reader); return true; } else if (_typeUsageBuilder.HandleAttribute(reader)) { return true; } return false; } #endregion #region Private Methods /// /// /// /// private void HandleTypeAttribute(XmlReader reader) { if (UnresolvedType != null) { AddError(ErrorCode.AlreadyDefined, EdmSchemaErrorSeverity.Error, reader, System.Data.Entity.Strings.PropertyTypeAlreadyDefined(reader.Name)); return; } string type; if (!Utils.GetDottedName(Schema, reader, out type)) return; UnresolvedType = type; } ////// Handles the Multiplicity attribute on the property. /// /// private void HandleCollectionKindAttribute(XmlReader reader) { string value = reader.Value; if (value == XmlConstants.CollectionKind_None) { _collectionKind = CollectionKind.None; } else { if (value == XmlConstants.CollectionKind_List) { _collectionKind = CollectionKind.List; } else if (value == XmlConstants.CollectionKind_Bag) { _collectionKind = CollectionKind.Bag; } else { Debug.Fail("Xsd should have changed", "XSD validation should have ensured that" + " Multiplicity attribute has only 'None' or 'Bag' or 'List' as the values"); return; } } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
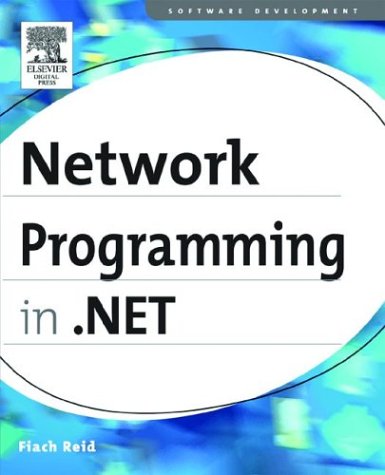
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ServiceDescriptionSerializer.cs
- CoreSwitches.cs
- SqlBulkCopyColumnMappingCollection.cs
- SynchronizingStream.cs
- PropertyItem.cs
- TreeNodeBindingCollection.cs
- StringHelper.cs
- RuleProcessor.cs
- LineUtil.cs
- RewritingSimplifier.cs
- XmlCharCheckingReader.cs
- UrlAuthFailureHandler.cs
- ExpressionVisitorHelpers.cs
- XmlSubtreeReader.cs
- SamlAttributeStatement.cs
- XmlParserContext.cs
- SuppressIldasmAttribute.cs
- BrowserCapabilitiesFactoryBase.cs
- HttpProfileGroupBase.cs
- TaskForm.cs
- DynamicExpression.cs
- SiteMapNodeCollection.cs
- CurrentChangedEventManager.cs
- DateTimeSerializationSection.cs
- SafeLibraryHandle.cs
- EvidenceBase.cs
- InputManager.cs
- Task.cs
- ToolStripItemTextRenderEventArgs.cs
- GlobalizationSection.cs
- ServerIdentity.cs
- DeferredRunTextReference.cs
- CalendarDateRange.cs
- ListItemConverter.cs
- EntityContainerAssociationSet.cs
- PackWebRequest.cs
- ConnectionPool.cs
- SqlMethodAttribute.cs
- DateTimeConverter2.cs
- ComPlusContractBehavior.cs
- RenderTargetBitmap.cs
- ColumnResizeAdorner.cs
- ArrangedElementCollection.cs
- Globals.cs
- BlurBitmapEffect.cs
- Parser.cs
- ParamArrayAttribute.cs
- DBSchemaTable.cs
- Type.cs
- TemplateKeyConverter.cs
- MetadataSource.cs
- BamlLocalizerErrorNotifyEventArgs.cs
- ConnectionPoint.cs
- CommandLineParser.cs
- BuildManager.cs
- ClientSettingsSection.cs
- ClickablePoint.cs
- SmiMetaData.cs
- _RequestLifetimeSetter.cs
- CodeDomConfigurationHandler.cs
- PagesChangedEventArgs.cs
- ConnectionOrientedTransportElement.cs
- DesignerActionItemCollection.cs
- Utils.cs
- CharacterMetrics.cs
- AddInIpcChannel.cs
- HttpContextWrapper.cs
- PanelDesigner.cs
- XPathEmptyIterator.cs
- Win32.cs
- SimpleRecyclingCache.cs
- WebSysDefaultValueAttribute.cs
- loginstatus.cs
- XmlSchemaType.cs
- EventProviderWriter.cs
- Serializer.cs
- DllNotFoundException.cs
- XmlSchemaObject.cs
- WebPartDisplayModeEventArgs.cs
- XmlQueryContext.cs
- HyperlinkAutomationPeer.cs
- DataGridCell.cs
- ListViewGroupItemCollection.cs
- Win32SafeHandles.cs
- AliasExpr.cs
- List.cs
- CharKeyFrameCollection.cs
- AuthenticationManager.cs
- TransformerInfo.cs
- DateTimePicker.cs
- FontSizeConverter.cs
- XXXInfos.cs
- CLRBindingWorker.cs
- RadioButton.cs
- ControlTemplate.cs
- AutomationElementIdentifiers.cs
- StringConverter.cs
- MDIControlStrip.cs
- AsyncPostBackTrigger.cs
- DataSysAttribute.cs