Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / ndp / fx / src / DLinq / Dlinq / SqlClient / Common / SqlNodeTypeOperators.cs / 1 / SqlNodeTypeOperators.cs
using System; using System.Collections.Generic; using System.Text; using System.Diagnostics.CodeAnalysis; namespace System.Data.Linq.SqlClient { internal static class SqlNodeTypeOperators { ////// Determines whether the given unary operator node type returns a value that /// is predicate. /// [SuppressMessage("Microsoft.Maintainability", "CA1502:AvoidExcessiveComplexity", Justification="These issues are related to our use of if-then and case statements for node types, which adds to the complexity count however when reviewed they are easy to navigate and understand.")] internal static bool IsPredicateUnaryOperator(this SqlNodeType nodeType) { switch (nodeType) { case SqlNodeType.Not: case SqlNodeType.Not2V: case SqlNodeType.IsNull: case SqlNodeType.IsNotNull: return true; case SqlNodeType.Negate: case SqlNodeType.BitNot: case SqlNodeType.Count: case SqlNodeType.LongCount: case SqlNodeType.Max: case SqlNodeType.Min: case SqlNodeType.Sum: case SqlNodeType.Avg: case SqlNodeType.Stddev: case SqlNodeType.Covar: case SqlNodeType.Convert: case SqlNodeType.Cast: case SqlNodeType.ValueOf: case SqlNodeType.OuterJoinedValue: case SqlNodeType.ClrLength: return false; default: throw Error.UnexpectedNode(nodeType); } } ////// Determines whether the given unary operator expects a predicate as input. /// [SuppressMessage("Microsoft.Maintainability", "CA1502:AvoidExcessiveComplexity", Justification="These issues are related to our use of if-then and case statements for node types, which adds to the complexity count however when reviewed they are easy to navigate and understand.")] internal static bool IsUnaryOperatorExpectingPredicateOperand(this SqlNodeType nodeType) { switch (nodeType) { case SqlNodeType.Not: case SqlNodeType.Not2V: return true; case SqlNodeType.IsNull: case SqlNodeType.IsNotNull: case SqlNodeType.Negate: case SqlNodeType.BitNot: case SqlNodeType.Count: case SqlNodeType.LongCount: case SqlNodeType.Max: case SqlNodeType.Min: case SqlNodeType.Sum: case SqlNodeType.Avg: case SqlNodeType.Stddev: case SqlNodeType.Covar: case SqlNodeType.Convert: case SqlNodeType.Cast: case SqlNodeType.ValueOf: case SqlNodeType.OuterJoinedValue: case SqlNodeType.ClrLength: return false; default: throw Error.UnexpectedNode(nodeType); } } ////// Determines whether the given binary operator node type returns a value that /// is a predicate boolean. /// [SuppressMessage("Microsoft.Maintainability", "CA1502:AvoidExcessiveComplexity", Justification="These issues are related to our use of if-then and case statements for node types, which adds to the complexity count however when reviewed they are easy to navigate and understand.")] internal static bool IsPredicateBinaryOperator(this SqlNodeType nodeType) { switch (nodeType) { case SqlNodeType.GE: case SqlNodeType.GT: case SqlNodeType.LE: case SqlNodeType.LT: case SqlNodeType.EQ: case SqlNodeType.NE: case SqlNodeType.EQ2V: case SqlNodeType.NE2V: case SqlNodeType.And: case SqlNodeType.Or: return true; case SqlNodeType.Add: case SqlNodeType.Sub: case SqlNodeType.Mul: case SqlNodeType.Div: case SqlNodeType.Mod: case SqlNodeType.BitAnd: case SqlNodeType.BitOr: case SqlNodeType.BitXor: case SqlNodeType.Concat: case SqlNodeType.Coalesce: return false; default: throw Error.UnexpectedNode(nodeType); } } ////// Determines whether this operator is a binary comparison operator (i.e. >, =>, ==, etc) /// [SuppressMessage("Microsoft.Maintainability", "CA1502:AvoidExcessiveComplexity", Justification = "These issues are related to our use of if-then and case statements for node types, which adds to the complexity count however when reviewed they are easy to navigate and understand.")] internal static bool IsComparisonOperator(this SqlNodeType nodeType) { switch (nodeType) { case SqlNodeType.GE: case SqlNodeType.GT: case SqlNodeType.LE: case SqlNodeType.LT: case SqlNodeType.EQ: case SqlNodeType.NE: case SqlNodeType.EQ2V: case SqlNodeType.NE2V: return true; case SqlNodeType.And: case SqlNodeType.Or: case SqlNodeType.Add: case SqlNodeType.Sub: case SqlNodeType.Mul: case SqlNodeType.Div: case SqlNodeType.Mod: case SqlNodeType.BitAnd: case SqlNodeType.BitOr: case SqlNodeType.BitXor: case SqlNodeType.Concat: case SqlNodeType.Coalesce: return false; default: throw Error.UnexpectedNode(nodeType); } } ////// Determines whether the given binary operator node type returns a value that /// is a predicate boolean. /// [SuppressMessage("Microsoft.Maintainability", "CA1502:AvoidExcessiveComplexity", Justification="These issues are related to our use of if-then and case statements for node types, which adds to the complexity count however when reviewed they are easy to navigate and understand.")] internal static bool IsBinaryOperatorExpectingPredicateOperands(this SqlNodeType nodeType) { switch (nodeType) { case SqlNodeType.And: case SqlNodeType.Or: return true; case SqlNodeType.EQ: case SqlNodeType.EQ2V: case SqlNodeType.GE: case SqlNodeType.GT: case SqlNodeType.LE: case SqlNodeType.LT: case SqlNodeType.NE: case SqlNodeType.NE2V: case SqlNodeType.Add: case SqlNodeType.Sub: case SqlNodeType.Mul: case SqlNodeType.Div: case SqlNodeType.Mod: case SqlNodeType.BitAnd: case SqlNodeType.BitOr: case SqlNodeType.BitXor: case SqlNodeType.Concat: case SqlNodeType.Coalesce: return false; default: throw Error.UnexpectedNode(nodeType); } } ////// Determines whether the given node requires support on the client for evaluation. /// For example, LINK nodes may be delay-executed only when the user requests the result. /// internal static bool IsClientAidedExpression(this SqlExpression expr) { switch (expr.NodeType) { case SqlNodeType.Link: case SqlNodeType.Element: case SqlNodeType.Multiset: case SqlNodeType.ClientQuery: case SqlNodeType.TypeCase: case SqlNodeType.New: return true; default: return false; }; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System; using System.Collections.Generic; using System.Text; using System.Diagnostics.CodeAnalysis; namespace System.Data.Linq.SqlClient { internal static class SqlNodeTypeOperators { ////// Determines whether the given unary operator node type returns a value that /// is predicate. /// [SuppressMessage("Microsoft.Maintainability", "CA1502:AvoidExcessiveComplexity", Justification="These issues are related to our use of if-then and case statements for node types, which adds to the complexity count however when reviewed they are easy to navigate and understand.")] internal static bool IsPredicateUnaryOperator(this SqlNodeType nodeType) { switch (nodeType) { case SqlNodeType.Not: case SqlNodeType.Not2V: case SqlNodeType.IsNull: case SqlNodeType.IsNotNull: return true; case SqlNodeType.Negate: case SqlNodeType.BitNot: case SqlNodeType.Count: case SqlNodeType.LongCount: case SqlNodeType.Max: case SqlNodeType.Min: case SqlNodeType.Sum: case SqlNodeType.Avg: case SqlNodeType.Stddev: case SqlNodeType.Covar: case SqlNodeType.Convert: case SqlNodeType.Cast: case SqlNodeType.ValueOf: case SqlNodeType.OuterJoinedValue: case SqlNodeType.ClrLength: return false; default: throw Error.UnexpectedNode(nodeType); } } ////// Determines whether the given unary operator expects a predicate as input. /// [SuppressMessage("Microsoft.Maintainability", "CA1502:AvoidExcessiveComplexity", Justification="These issues are related to our use of if-then and case statements for node types, which adds to the complexity count however when reviewed they are easy to navigate and understand.")] internal static bool IsUnaryOperatorExpectingPredicateOperand(this SqlNodeType nodeType) { switch (nodeType) { case SqlNodeType.Not: case SqlNodeType.Not2V: return true; case SqlNodeType.IsNull: case SqlNodeType.IsNotNull: case SqlNodeType.Negate: case SqlNodeType.BitNot: case SqlNodeType.Count: case SqlNodeType.LongCount: case SqlNodeType.Max: case SqlNodeType.Min: case SqlNodeType.Sum: case SqlNodeType.Avg: case SqlNodeType.Stddev: case SqlNodeType.Covar: case SqlNodeType.Convert: case SqlNodeType.Cast: case SqlNodeType.ValueOf: case SqlNodeType.OuterJoinedValue: case SqlNodeType.ClrLength: return false; default: throw Error.UnexpectedNode(nodeType); } } ////// Determines whether the given binary operator node type returns a value that /// is a predicate boolean. /// [SuppressMessage("Microsoft.Maintainability", "CA1502:AvoidExcessiveComplexity", Justification="These issues are related to our use of if-then and case statements for node types, which adds to the complexity count however when reviewed they are easy to navigate and understand.")] internal static bool IsPredicateBinaryOperator(this SqlNodeType nodeType) { switch (nodeType) { case SqlNodeType.GE: case SqlNodeType.GT: case SqlNodeType.LE: case SqlNodeType.LT: case SqlNodeType.EQ: case SqlNodeType.NE: case SqlNodeType.EQ2V: case SqlNodeType.NE2V: case SqlNodeType.And: case SqlNodeType.Or: return true; case SqlNodeType.Add: case SqlNodeType.Sub: case SqlNodeType.Mul: case SqlNodeType.Div: case SqlNodeType.Mod: case SqlNodeType.BitAnd: case SqlNodeType.BitOr: case SqlNodeType.BitXor: case SqlNodeType.Concat: case SqlNodeType.Coalesce: return false; default: throw Error.UnexpectedNode(nodeType); } } ////// Determines whether this operator is a binary comparison operator (i.e. >, =>, ==, etc) /// [SuppressMessage("Microsoft.Maintainability", "CA1502:AvoidExcessiveComplexity", Justification = "These issues are related to our use of if-then and case statements for node types, which adds to the complexity count however when reviewed they are easy to navigate and understand.")] internal static bool IsComparisonOperator(this SqlNodeType nodeType) { switch (nodeType) { case SqlNodeType.GE: case SqlNodeType.GT: case SqlNodeType.LE: case SqlNodeType.LT: case SqlNodeType.EQ: case SqlNodeType.NE: case SqlNodeType.EQ2V: case SqlNodeType.NE2V: return true; case SqlNodeType.And: case SqlNodeType.Or: case SqlNodeType.Add: case SqlNodeType.Sub: case SqlNodeType.Mul: case SqlNodeType.Div: case SqlNodeType.Mod: case SqlNodeType.BitAnd: case SqlNodeType.BitOr: case SqlNodeType.BitXor: case SqlNodeType.Concat: case SqlNodeType.Coalesce: return false; default: throw Error.UnexpectedNode(nodeType); } } ////// Determines whether the given binary operator node type returns a value that /// is a predicate boolean. /// [SuppressMessage("Microsoft.Maintainability", "CA1502:AvoidExcessiveComplexity", Justification="These issues are related to our use of if-then and case statements for node types, which adds to the complexity count however when reviewed they are easy to navigate and understand.")] internal static bool IsBinaryOperatorExpectingPredicateOperands(this SqlNodeType nodeType) { switch (nodeType) { case SqlNodeType.And: case SqlNodeType.Or: return true; case SqlNodeType.EQ: case SqlNodeType.EQ2V: case SqlNodeType.GE: case SqlNodeType.GT: case SqlNodeType.LE: case SqlNodeType.LT: case SqlNodeType.NE: case SqlNodeType.NE2V: case SqlNodeType.Add: case SqlNodeType.Sub: case SqlNodeType.Mul: case SqlNodeType.Div: case SqlNodeType.Mod: case SqlNodeType.BitAnd: case SqlNodeType.BitOr: case SqlNodeType.BitXor: case SqlNodeType.Concat: case SqlNodeType.Coalesce: return false; default: throw Error.UnexpectedNode(nodeType); } } ////// Determines whether the given node requires support on the client for evaluation. /// For example, LINK nodes may be delay-executed only when the user requests the result. /// internal static bool IsClientAidedExpression(this SqlExpression expr) { switch (expr.NodeType) { case SqlNodeType.Link: case SqlNodeType.Element: case SqlNodeType.Multiset: case SqlNodeType.ClientQuery: case SqlNodeType.TypeCase: case SqlNodeType.New: return true; default: return false; }; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
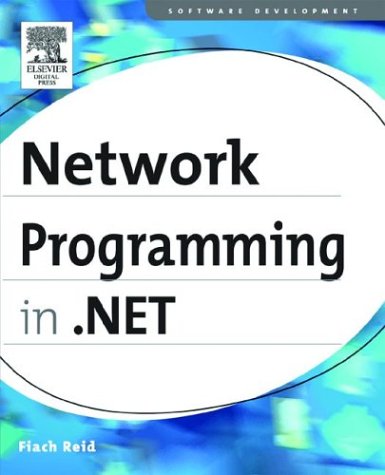
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- AvTrace.cs
- BinaryParser.cs
- Int32CollectionValueSerializer.cs
- WhiteSpaceTrimStringConverter.cs
- FixedSOMContainer.cs
- QuotedStringWriteStateInfo.cs
- CharacterHit.cs
- XmlCountingReader.cs
- DoubleAnimationUsingPath.cs
- RootContext.cs
- RowUpdatingEventArgs.cs
- XmlChoiceIdentifierAttribute.cs
- JulianCalendar.cs
- StorageRoot.cs
- ActivitySurrogate.cs
- ValueQuery.cs
- DataReceivedEventArgs.cs
- BufferedOutputStream.cs
- ParseNumbers.cs
- LicenseProviderAttribute.cs
- EventSetter.cs
- FixedElement.cs
- AccessedThroughPropertyAttribute.cs
- ApplicationException.cs
- PlaceHolder.cs
- PageWrapper.cs
- DoWorkEventArgs.cs
- SimpleApplicationHost.cs
- Codec.cs
- InfiniteTimeSpanConverter.cs
- GenericRootAutomationPeer.cs
- SimpleTextLine.cs
- DataGridViewRowsRemovedEventArgs.cs
- DynamicRouteExpression.cs
- XmlSerializationGeneratedCode.cs
- EntityDataSourceDataSelection.cs
- TextEffect.cs
- oledbmetadatacollectionnames.cs
- BindableAttribute.cs
- QilXmlWriter.cs
- ZipFileInfoCollection.cs
- ProcessProtocolHandler.cs
- XmlDomTextWriter.cs
- MemoryMappedViewStream.cs
- PrimaryKeyTypeConverter.cs
- Domain.cs
- TransportOutputChannel.cs
- ResponseStream.cs
- DiscoveryClientReferences.cs
- MdImport.cs
- SmtpReplyReaderFactory.cs
- Permission.cs
- SerializationHelper.cs
- TraceInternal.cs
- CommonGetThemePartSize.cs
- Point3DCollectionConverter.cs
- BrushConverter.cs
- ConnectionManagementElementCollection.cs
- StaticFileHandler.cs
- Options.cs
- XmlNamespaceDeclarationsAttribute.cs
- ActivityExecutorOperation.cs
- FormatterConverter.cs
- LongValidatorAttribute.cs
- XmlCharCheckingReader.cs
- Accessible.cs
- VirtualizingPanel.cs
- KeyMatchBuilder.cs
- Utils.cs
- CodeMethodInvokeExpression.cs
- InstallerTypeAttribute.cs
- FillErrorEventArgs.cs
- OutputScope.cs
- OleDbConnectionPoolGroupProviderInfo.cs
- StreamWriter.cs
- PanelStyle.cs
- TypeInfo.cs
- ManagementOperationWatcher.cs
- ElementProxy.cs
- PartialCachingAttribute.cs
- Label.cs
- IntegerCollectionEditor.cs
- PageDeviceFont.cs
- MessageAction.cs
- SyndicationDeserializer.cs
- OutputWindow.cs
- DataGridViewIntLinkedList.cs
- ToolStripItemImageRenderEventArgs.cs
- HandlerBase.cs
- DataGridViewButtonCell.cs
- _CacheStreams.cs
- TextDpi.cs
- IssuanceLicense.cs
- LineGeometry.cs
- GroupBox.cs
- ArrangedElementCollection.cs
- GeneralTransform.cs
- LeftCellWrapper.cs
- ComponentChangedEvent.cs
- GeometryModel3D.cs