Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / DataOracleClient / System / Data / OracleClient / OciLobLocator.cs / 1 / OciLobLocator.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace System.Data.OracleClient { using System; using System.Data.Common; using System.Data.SqlTypes; using System.Diagnostics; using System.Globalization; using System.IO; using System.Runtime.ConstrainedExecution; using System.Runtime.InteropServices; using System.Text; using System.Threading; sealed internal class OciLobLocator { private OracleConnection _connection; // the connection the lob is attached to private int _connectionCloseCount; // The close count of the connection; used to decide if we're zombied private OracleType _lobType; // the underlying data type of the LOB locator private OciHandle _descriptor; // the lob/file descriptor. private int _cloneCount; // the number of clones of this object -- used to know when we can close the lob/bfile. private int _openMode; // zero when not opened, otherwise, it's the value of OracleLobOpenMode internal OciLobLocator(OracleConnection connection, OracleType lobType) { _connection = connection; _connectionCloseCount = connection.CloseCount; _lobType = lobType; _cloneCount = 1; switch (lobType) { case OracleType.Blob: case OracleType.Clob: case OracleType.NClob: _descriptor = new OciLobDescriptor(connection.ServiceContextHandle); break; case OracleType.BFile: _descriptor = new OciFileDescriptor(connection.ServiceContextHandle); break; default: Debug.Assert(false, "Invalid lobType"); break; } } internal OracleConnection Connection { get { return _connection; } } internal bool ConnectionIsClosed { // returns TRUE when the parent connection object has been closed get { return (null == _connection) || (_connectionCloseCount != _connection.CloseCount); } } internal OciErrorHandle ErrorHandle { // Every OCI call needs an error handle, so make it available // internally. get { return Connection.ErrorHandle; } } internal OciHandle Descriptor { [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] get { return _descriptor; } } public OracleType LobType { get { return _lobType; } } internal OciServiceContextHandle ServiceContextHandle { // You need to provide the service context handle to things like the // OCI execute call so a statement handle can be associated with a // connection. Better make it available internally, then. get { return Connection.ServiceContextHandle; } } internal OciLobLocator Clone() { Interlocked.Increment(ref _cloneCount); return this; } internal void Dispose() { int cloneCount = Interlocked.Decrement(ref _cloneCount); if (0 == cloneCount) { if (0 != _openMode && !ConnectionIsClosed) { ForceClose(); } OciHandle.SafeDispose(ref _descriptor); GC.KeepAlive(this); _connection = null; } } internal void ForceClose() { if (0 != _openMode) { int rc = TracedNativeMethods.OCILobClose( ServiceContextHandle, ErrorHandle, Descriptor ); if (0 != rc) { Connection.CheckError(ErrorHandle, rc); } _openMode = 0; } } internal void ForceOpen() { if (0 != _openMode) { int rc = TracedNativeMethods.OCILobOpen( ServiceContextHandle, ErrorHandle, Descriptor, (byte)_openMode ); if (0 != rc) { _openMode = 0; // failure means we didn't really open it. Connection.CheckError(ErrorHandle, rc); } } } internal void Open (OracleLobOpenMode mode) { OracleLobOpenMode openMode = (OracleLobOpenMode)Interlocked.CompareExchange(ref _openMode, (int)mode, 0); if (0 == openMode) { ForceOpen(); } else if (mode != openMode) { throw ADP.CannotOpenLobWithDifferentMode(mode, openMode); } } internal static void SafeDispose(ref OciLobLocator locator) { // Safely disposes of the handle (even if it is already null) and // then nulls it out. if (null != locator) { locator.Dispose(); } locator = null; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace System.Data.OracleClient { using System; using System.Data.Common; using System.Data.SqlTypes; using System.Diagnostics; using System.Globalization; using System.IO; using System.Runtime.ConstrainedExecution; using System.Runtime.InteropServices; using System.Text; using System.Threading; sealed internal class OciLobLocator { private OracleConnection _connection; // the connection the lob is attached to private int _connectionCloseCount; // The close count of the connection; used to decide if we're zombied private OracleType _lobType; // the underlying data type of the LOB locator private OciHandle _descriptor; // the lob/file descriptor. private int _cloneCount; // the number of clones of this object -- used to know when we can close the lob/bfile. private int _openMode; // zero when not opened, otherwise, it's the value of OracleLobOpenMode internal OciLobLocator(OracleConnection connection, OracleType lobType) { _connection = connection; _connectionCloseCount = connection.CloseCount; _lobType = lobType; _cloneCount = 1; switch (lobType) { case OracleType.Blob: case OracleType.Clob: case OracleType.NClob: _descriptor = new OciLobDescriptor(connection.ServiceContextHandle); break; case OracleType.BFile: _descriptor = new OciFileDescriptor(connection.ServiceContextHandle); break; default: Debug.Assert(false, "Invalid lobType"); break; } } internal OracleConnection Connection { get { return _connection; } } internal bool ConnectionIsClosed { // returns TRUE when the parent connection object has been closed get { return (null == _connection) || (_connectionCloseCount != _connection.CloseCount); } } internal OciErrorHandle ErrorHandle { // Every OCI call needs an error handle, so make it available // internally. get { return Connection.ErrorHandle; } } internal OciHandle Descriptor { [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] get { return _descriptor; } } public OracleType LobType { get { return _lobType; } } internal OciServiceContextHandle ServiceContextHandle { // You need to provide the service context handle to things like the // OCI execute call so a statement handle can be associated with a // connection. Better make it available internally, then. get { return Connection.ServiceContextHandle; } } internal OciLobLocator Clone() { Interlocked.Increment(ref _cloneCount); return this; } internal void Dispose() { int cloneCount = Interlocked.Decrement(ref _cloneCount); if (0 == cloneCount) { if (0 != _openMode && !ConnectionIsClosed) { ForceClose(); } OciHandle.SafeDispose(ref _descriptor); GC.KeepAlive(this); _connection = null; } } internal void ForceClose() { if (0 != _openMode) { int rc = TracedNativeMethods.OCILobClose( ServiceContextHandle, ErrorHandle, Descriptor ); if (0 != rc) { Connection.CheckError(ErrorHandle, rc); } _openMode = 0; } } internal void ForceOpen() { if (0 != _openMode) { int rc = TracedNativeMethods.OCILobOpen( ServiceContextHandle, ErrorHandle, Descriptor, (byte)_openMode ); if (0 != rc) { _openMode = 0; // failure means we didn't really open it. Connection.CheckError(ErrorHandle, rc); } } } internal void Open (OracleLobOpenMode mode) { OracleLobOpenMode openMode = (OracleLobOpenMode)Interlocked.CompareExchange(ref _openMode, (int)mode, 0); if (0 == openMode) { ForceOpen(); } else if (mode != openMode) { throw ADP.CannotOpenLobWithDifferentMode(mode, openMode); } } internal static void SafeDispose(ref OciLobLocator locator) { // Safely disposes of the handle (even if it is already null) and // then nulls it out. if (null != locator) { locator.Dispose(); } locator = null; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
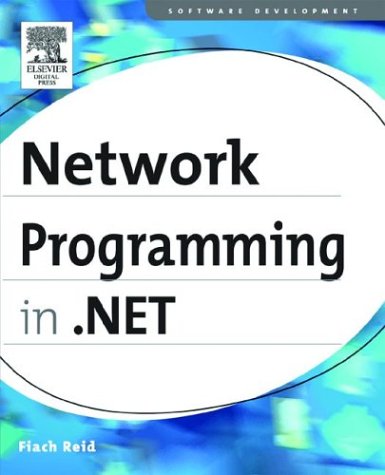
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- GenericTextProperties.cs
- TextParagraphView.cs
- Storyboard.cs
- TypeToken.cs
- DbConnectionPoolCounters.cs
- ObservableCollectionDefaultValueFactory.cs
- TextBox.cs
- EntitySetDataBindingList.cs
- TableLayoutPanelCellPosition.cs
- PermissionSetTriple.cs
- behaviorssection.cs
- VisualBasicImportReference.cs
- ApplicationHost.cs
- AttachInfo.cs
- arabicshape.cs
- Regex.cs
- DataSourceControlBuilder.cs
- QilStrConcat.cs
- EntityDataReader.cs
- Clock.cs
- DataGridViewButtonCell.cs
- WCFBuildProvider.cs
- DelegatingTypeDescriptionProvider.cs
- VirtualPathProvider.cs
- RichTextBoxDesigner.cs
- XmlILAnnotation.cs
- SortDescription.cs
- OdbcConnectionOpen.cs
- NonClientArea.cs
- _HeaderInfoTable.cs
- RunClient.cs
- WeakHashtable.cs
- MultiView.cs
- ObjectToken.cs
- PeerNameResolver.cs
- SqlException.cs
- TextSelectionHelper.cs
- AsyncOperation.cs
- EncryptRequest.cs
- Brush.cs
- SplayTreeNode.cs
- SettingsBase.cs
- NodeLabelEditEvent.cs
- DependencyPropertyKind.cs
- itemelement.cs
- CustomTypeDescriptor.cs
- PeerPresenceInfo.cs
- ResourceSet.cs
- CustomActivityDesigner.cs
- RtfControlWordInfo.cs
- RbTree.cs
- IPCCacheManager.cs
- WebHttpDispatchOperationSelector.cs
- ContextDataSourceContextData.cs
- SynchronizationContextHelper.cs
- SortExpressionBuilder.cs
- FusionWrap.cs
- GeneratedView.cs
- DataRowComparer.cs
- XmlUtf8RawTextWriter.cs
- FactoryGenerator.cs
- TrackPoint.cs
- DataViewListener.cs
- EventDescriptorCollection.cs
- LogSwitch.cs
- DataGridCaption.cs
- hresults.cs
- ApplicationSecurityManager.cs
- ToolStripSettings.cs
- RelationshipFixer.cs
- ProcessHostServerConfig.cs
- CreateDataSourceDialog.cs
- XmlWriter.cs
- HttpChannelBindingToken.cs
- TcpClientChannel.cs
- FontStretch.cs
- ButtonColumn.cs
- XPathItem.cs
- CmsInterop.cs
- IssuedTokenParametersElement.cs
- EventPropertyMap.cs
- AttributedMetaModel.cs
- DropDownButton.cs
- LogAppendAsyncResult.cs
- DependencyPropertyValueSerializer.cs
- ConfigXmlCDataSection.cs
- StyleCollectionEditor.cs
- XmlSerializerAssemblyAttribute.cs
- ListItemParagraph.cs
- BasicCellRelation.cs
- RawStylusInputCustomDataList.cs
- FixedHighlight.cs
- InvalidComObjectException.cs
- ReferentialConstraint.cs
- PathGeometry.cs
- EventToken.cs
- Point3DAnimationBase.cs
- SoapAttributes.cs
- SqlRowUpdatingEvent.cs
- TableRowCollection.cs