Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / xsp / System / Web / UI / WebControls / ValidationSummary.cs / 5 / ValidationSummary.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls { using System.ComponentModel; using System.Drawing; using System.Globalization; using System.Web; using System.Web.UI.HtmlControls; using System.Security.Permissions; using System.Web.Util; ////// [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] [Designer("System.Web.UI.Design.WebControls.PreviewControlDesigner, " + AssemblyRef.SystemDesign)] public class ValidationSummary : WebControl { private const String breakTag = "b"; private bool renderUplevel; ///Displays a summary of all validation errors of /// a page in a list, bulletted list, or single paragraph format. The errors can be displayed inline /// and/or in a popup message box. ////// public ValidationSummary() : base(HtmlTextWriterTag.Div) { renderUplevel = false; // Red by default ForeColor = Color.Red; } ///Initializes a new instance of the ///class. /// [ WebCategory("Appearance"), DefaultValue(ValidationSummaryDisplayMode.BulletList), WebSysDescription(SR.ValidationSummary_DisplayMode) ] public ValidationSummaryDisplayMode DisplayMode { get { object o = ViewState["DisplayMode"]; return((o == null) ? ValidationSummaryDisplayMode.BulletList : (ValidationSummaryDisplayMode)o); } set { if (value < ValidationSummaryDisplayMode.List || value > ValidationSummaryDisplayMode.SingleParagraph) { throw new ArgumentOutOfRangeException("value"); } ViewState["DisplayMode"] = value; } } ///Gets or sets the display mode of the validation summary. ////// [ WebCategory("Behavior"), Themeable(false), DefaultValue(true), WebSysDescription(SR.ValidationSummary_EnableClientScript) ] public bool EnableClientScript { get { object o = ViewState["EnableClientScript"]; return((o == null) ? true : (bool)o); } set { ViewState["EnableClientScript"] = value; } } ///[To be supplied.] ////// [ DefaultValue(typeof(Color), "Red") ] public override Color ForeColor { get { return base.ForeColor; } set { base.ForeColor = value; } } ///Gets or sets the foreground color /// (typically the color of the text) of the control. ////// [ Localizable(true), WebCategory("Appearance"), DefaultValue(""), WebSysDescription(SR.ValidationSummary_HeaderText) ] public string HeaderText { get { object o = ViewState["HeaderText"]; return((o == null) ? String.Empty : (string)o); } set { ViewState["HeaderText"] = value; } } ///Gets or sets the header text to be displayed at the top /// of the summary. ////// [ WebCategory("Behavior"), DefaultValue(false), WebSysDescription(SR.ValidationSummary_ShowMessageBox) ] public bool ShowMessageBox { get { object o = ViewState["ShowMessageBox"]; return((o == null) ? false : (bool)o); } set { ViewState["ShowMessageBox"] = value; } } ///Gets or sets a value indicating whether the validation /// summary is to be displayed in a pop-up message box. ////// [ WebCategory("Behavior"), DefaultValue(true), WebSysDescription(SR.ValidationSummary_ShowSummary) ] public bool ShowSummary { get { object o = ViewState["ShowSummary"]; return((o == null) ? true : (bool)o); } set { ViewState["ShowSummary"] = value; } } [ WebCategory("Behavior"), Themeable(false), DefaultValue(""), WebSysDescription(SR.ValidationSummary_ValidationGroup) ] public virtual string ValidationGroup { get { string s = (string)ViewState["ValidationGroup"]; return((s == null) ? string.Empty : s); } set { ViewState["ValidationGroup"] = value; } } ///Gets or sets a value indicating whether the validation /// summary is to be displayed inline. ////// /// AddAttributesToRender method. /// protected override void AddAttributesToRender(HtmlTextWriter writer) { if (renderUplevel) { // We always want validation cotnrols to have an id on the client EnsureID(); string id = ClientID; // DevDiv 33149: A backward compat. switch for Everett rendering HtmlTextWriter expandoAttributeWriter = (EnableLegacyRendering) ? writer : null; if (HeaderText.Length > 0 ) { BaseValidator.AddExpandoAttribute(this, expandoAttributeWriter, id, "headertext", HeaderText, true); } if (ShowMessageBox) { BaseValidator.AddExpandoAttribute(this, expandoAttributeWriter, id, "showmessagebox", "True", false); } if (!ShowSummary) { BaseValidator.AddExpandoAttribute(this, expandoAttributeWriter, id, "showsummary", "False", false); } if (DisplayMode != ValidationSummaryDisplayMode.BulletList) { BaseValidator.AddExpandoAttribute(this, expandoAttributeWriter, id, "displaymode", PropertyConverter.EnumToString(typeof(ValidationSummaryDisplayMode), DisplayMode), false); } if (ValidationGroup.Length > 0) { BaseValidator.AddExpandoAttribute(this, expandoAttributeWriter, id, "validationGroup", ValidationGroup, true); } } base.AddAttributesToRender(writer); } internal String[] GetErrorMessages(out bool inError) { // Fetch errors from the Page String [] errorDescriptions = null; inError = false; // see if we are in error and how many messages there are int messages = 0; ValidatorCollection validators = Page.GetValidators(ValidationGroup); for (int i = 0; i < validators.Count; i++) { IValidator val = validators[i]; if (!val.IsValid) { inError = true; if (val.ErrorMessage.Length != 0) { messages++; } } } if (messages != 0) { // get the messages; errorDescriptions = new string [messages]; int iMessage = 0; for (int i = 0; i < validators.Count; i++) { IValidator val = validators[i]; if (!val.IsValid && val.ErrorMessage != null && val.ErrorMessage.Length != 0) { errorDescriptions[iMessage] = string.Copy(val.ErrorMessage); iMessage++; } } Debug.Assert(messages == iMessage, "Not all messages were found!"); } return errorDescriptions; } ////// /// PreRender method. /// protected internal override void OnPreRender(EventArgs e) { base.OnPreRender(e); // Act like invisible if disabled if (!Enabled) { return; } // work out uplevelness now Page page = Page; if (page != null && page.RequestInternal != null) { renderUplevel = (EnableClientScript && page.Request.Browser.W3CDomVersion.Major >= 1 && page.Request.Browser.EcmaScriptVersion.CompareTo(new Version(1, 2)) >= 0); } if (renderUplevel) { const string arrayName = "Page_ValidationSummaries"; string element = "document.getElementById(\"" + ClientID + "\")"; // Cannot use the overloads of Register* that take a Control, since these methods only work with AJAX 3.5, // and we need to support Validators in AJAX 1.0 (Windows OS Bugs 2015831). if (!Page.IsPartialRenderingSupported) { Page.ClientScript.RegisterArrayDeclaration(arrayName, element); } else { ValidatorCompatibilityHelper.RegisterArrayDeclaration(this, arrayName, element); // Register a dispose script to make sure we clean up the page if we get destroyed // during an async postback. // We should technically use the ScriptManager.RegisterDispose() method here, but the original implementation // of Validators in AJAX 1.0 manually attached a dispose expando. We added this code back in the product // late in the Orcas cycle, and we didn't want to take the risk of using RegisterDispose() instead. // (Windows OS Bugs 2015831) ValidatorCompatibilityHelper.RegisterStartupScript(this, typeof(ValidationSummary), ClientID + "_DisposeScript", String.Format( CultureInfo.InvariantCulture, @" document.getElementById('{0}').dispose = function() {{ Array.remove({1}, document.getElementById('{0}')); }} ", ClientID, arrayName), true); } } } ////// /// Render method. /// protected internal override void Render(HtmlTextWriter writer) { string [] errorDescriptions; bool displayContents; if (DesignMode) { // Dummy Error state errorDescriptions = new string [] { SR.GetString(SR.ValSummary_error_message_1), SR.GetString(SR.ValSummary_error_message_2), }; displayContents = true; renderUplevel = false; } else { // Act like invisible if disabled if (!Enabled) { return; } bool inError; errorDescriptions = GetErrorMessages(out inError); displayContents = (ShowSummary && inError); // Make sure tags are hidden if there are no contents if (!displayContents && renderUplevel) { Style["display"] = "none"; } } // Make sure we are in a form tag with runat=server. if (Page != null) { Page.VerifyRenderingInServerForm(this); } bool displayTags = renderUplevel ? true : displayContents; if (displayTags) { RenderBeginTag(writer); } if (displayContents) { string headerSep; string first; string pre; string post; string final; switch (DisplayMode) { case ValidationSummaryDisplayMode.List: headerSep = breakTag; first = String.Empty; pre = String.Empty; post = breakTag; final = String.Empty; break; case ValidationSummaryDisplayMode.BulletList: headerSep = String.Empty; first = "
- ";
pre = "
- "; post = " "; final = "
- ";
pre = "
- "; post = " "; final = "
Link Menu
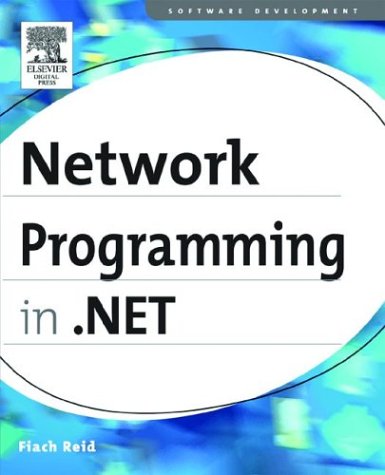
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ErrorWrapper.cs
- Misc.cs
- BindingElement.cs
- ToolBar.cs
- BuildManagerHost.cs
- IIS7WorkerRequest.cs
- EditorZoneBase.cs
- StorageComplexTypeMapping.cs
- Component.cs
- SpellerHighlightLayer.cs
- MaskDescriptors.cs
- UserControlBuildProvider.cs
- SecurityTokenValidationException.cs
- HttpResponseWrapper.cs
- QueryContinueDragEvent.cs
- DetailsViewRow.cs
- RegexNode.cs
- CategoryEditor.cs
- NGCUIElementCollectionSerializerAsync.cs
- LogExtentCollection.cs
- ExtendedProperty.cs
- ConditionValidator.cs
- WhitespaceRuleLookup.cs
- XmlAttributeAttribute.cs
- HostingEnvironmentSection.cs
- DiscoveryEndpointValidator.cs
- Vector3dCollection.cs
- CommandID.cs
- CreateUserWizardStep.cs
- InstanceValue.cs
- Floater.cs
- HttpResponse.cs
- ItemsControl.cs
- controlskin.cs
- VisualStyleRenderer.cs
- SQLStringStorage.cs
- XmlEncodedRawTextWriter.cs
- ChannelSinkStacks.cs
- MutexSecurity.cs
- WebPartHelpVerb.cs
- InfoCardRSAPKCS1SignatureFormatter.cs
- XmlRootAttribute.cs
- WebSysDisplayNameAttribute.cs
- ClientCultureInfo.cs
- URLMembershipCondition.cs
- ToolStripSplitButton.cs
- FolderLevelBuildProviderCollection.cs
- DataSourceXmlSubItemAttribute.cs
- DoubleAnimationUsingKeyFrames.cs
- KeySplineConverter.cs
- SemanticKeyElement.cs
- BinaryCommonClasses.cs
- SqlMethodAttribute.cs
- URLMembershipCondition.cs
- DatatypeImplementation.cs
- DataBoundLiteralControl.cs
- ListBox.cs
- SoapFormatter.cs
- GlyphShapingProperties.cs
- TimeZone.cs
- Application.cs
- StrokeRenderer.cs
- DataServiceQuery.cs
- TextRunTypographyProperties.cs
- FixedSOMPageConstructor.cs
- PropertyGridView.cs
- OrderedDictionary.cs
- SchemaMerger.cs
- SubclassTypeValidator.cs
- XNameTypeConverter.cs
- Publisher.cs
- IssuedTokenServiceCredential.cs
- StructuralType.cs
- DataGridItemEventArgs.cs
- WebScriptMetadataMessageEncodingBindingElement.cs
- WindowsTokenRoleProvider.cs
- ValidationErrorCollection.cs
- ColumnResizeUndoUnit.cs
- BamlRecordHelper.cs
- ConfigPathUtility.cs
- ResponseBodyWriter.cs
- DesignerOptionService.cs
- BehaviorEditorPart.cs
- ZipIOLocalFileBlock.cs
- SqlExpander.cs
- MergeFilterQuery.cs
- AttributeInfo.cs
- BlockUIContainer.cs
- PeerTransportElement.cs
- ContainerTracking.cs
- Validator.cs
- VolatileResourceManager.cs
- SocketPermission.cs
- UIElementIsland.cs
- BaseDataListActionList.cs
- TripleDESCryptoServiceProvider.cs
- Transaction.cs
- HyperLinkField.cs
- ViewCellSlot.cs
- FieldNameLookup.cs