Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / NetFx35 / System.ServiceModel.Web / System / ServiceModel / Dispatcher / HttpUnhandledOperationInvoker.cs / 1 / HttpUnhandledOperationInvoker.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- #pragma warning disable 1634, 1691 namespace System.ServiceModel.Dispatcher { using System; using System.Globalization; using System.Xml; using System.Net; using System.ServiceModel; using System.ServiceModel.Channels; using System.ServiceModel.Description; using System.ServiceModel.Web; using System.Diagnostics.CodeAnalysis; using System.Diagnostics; using System.ServiceModel.Diagnostics; internal class HttpUnhandledOperationInvoker : IOperationInvoker { const string HtmlContentType = "text/html; charset=UTF-8"; public bool IsSynchronous { get { return true; } } public object[] AllocateInputs() { return new object[1]; } [SuppressMessage("Reliability", "Reliability104:CaughtAndHandledExceptionsRule", Justification = "The exception is thrown for tracing purposes")] public object Invoke(object instance, object[] inputs, out object[] outputs) { Message message = inputs[0] as Message; #pragma warning disable 56506 // [....], message.Properties is never null if (message == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new InvalidOperationException( SR2.GetString(SR2.HttpUnhandledOperationInvokerCalledWithoutMessage))); } // We might be here because we desire a redirect... Uri newLocation = null; Uri to = message.Headers.To; if (message.Properties.ContainsKey(WebHttpDispatchOperationSelector.RedirectPropertyName)) { newLocation = message.Properties[WebHttpDispatchOperationSelector.RedirectPropertyName] as Uri; } if (newLocation != null && to != null) { // ...redirect Message redirectResult = new HttpTransferRedirectMessage(to, newLocation); HttpResponseMessageProperty redirectResp = new HttpResponseMessageProperty(); redirectResp.StatusCode = System.Net.HttpStatusCode.TemporaryRedirect; redirectResp.Headers.Add(HttpResponseHeader.Location, newLocation.AbsoluteUri); redirectResp.Headers.Add(HttpResponseHeader.ContentType, HtmlContentType); #pragma warning disable 56506 // [....], message.Properties is never null redirectResult.Properties.Add(HttpResponseMessageProperty.Name, redirectResp); #pragma warning enable 56506 outputs = null; // Note that no exception is thrown along this path, even if the debugger is attached if (DiagnosticUtility.ShouldTraceInformation) { DiagnosticUtility.DiagnosticTrace.TraceEvent(TraceEventType.Information, TraceCode.WebRequestRedirect, SR2.GetString(SR2.TraceCodeWebRequestRedirect, to, newLocation)); } return redirectResult; } // otherwise we are here to issue either a 404 or a 405 bool uriMatched = false; if (message.Properties.ContainsKey(WebHttpDispatchOperationSelector.HttpOperationSelectorUriMatchedPropertyName)) { uriMatched = (bool) message.Properties[WebHttpDispatchOperationSelector.HttpOperationSelectorUriMatchedPropertyName]; } #pragma warning enable 56506 Message result = new HttpTransferHelpPageMessage(uriMatched); HttpResponseMessageProperty resp = new HttpResponseMessageProperty(); if (uriMatched) { resp.StatusCode = System.Net.HttpStatusCode.MethodNotAllowed; } else { resp.StatusCode = System.Net.HttpStatusCode.NotFound; } try { if (resp.StatusCode == HttpStatusCode.NotFound) { if (Debugger.IsAttached) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperWarning(new InvalidOperationException(SR2.GetString(SR2.WebRequestDidNotMatchOperation, OperationContext.Current.IncomingMessageHeaders.To))); } else { if (DiagnosticUtility.ShouldTraceWarning) { DiagnosticUtility.ExceptionUtility.TraceHandledException(new InvalidOperationException(SR2.GetString(SR2.WebRequestDidNotMatchOperation, OperationContext.Current.IncomingMessageHeaders.To)), TraceEventType.Warning); } } } else { if (Debugger.IsAttached) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperWarning(new InvalidOperationException(SR2.GetString(SR2.WebRequestDidNotMatchMethod, WebOperationContext.Current.IncomingRequest.Method, OperationContext.Current.IncomingMessageHeaders.To))); } else { if (DiagnosticUtility.ShouldTraceWarning) { DiagnosticUtility.ExceptionUtility.TraceHandledException(new InvalidOperationException(SR2.GetString(SR2.WebRequestDidNotMatchMethod, WebOperationContext.Current.IncomingRequest.Method, OperationContext.Current.IncomingMessageHeaders.To)), TraceEventType.Warning); } } } } catch (InvalidOperationException) { // catch the exception - its only used for tracing } resp.Headers.Add(HttpResponseHeader.ContentType, HtmlContentType); #pragma warning disable 56506 // [....], message.Properties is never null result.Properties.Add(HttpResponseMessageProperty.Name, resp); #pragma warning enable 56506 outputs = null; return result; } public IAsyncResult InvokeBegin(object instance, object[] inputs, AsyncCallback callback, object state) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new NotSupportedException()); } public object InvokeEnd(object instance, out object[] outputs, IAsyncResult result) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new NotSupportedException()); } class HttpTransferHelpPageMessage : ContentOnlyMessage { bool methodNotAllowed; // true if this is a 405 public HttpTransferHelpPageMessage() : base() { this.methodNotAllowed = false; } public HttpTransferHelpPageMessage(bool methodNotAllowed) : base() { this.methodNotAllowed = methodNotAllowed; } protected override void OnWriteBodyContents(XmlDictionaryWriter writer) { writer.WriteStartElement("HTML"); writer.WriteStartElement("HEAD"); writer.WriteRaw(String.Format(CultureInfo.InvariantCulture, @"{0}{1} ", SR2.GetString(SR2.HelpPageLayout), SR2.GetString(SR2.HelpPageTitleText))); writer.WriteEndElement(); //HEAD writer.WriteRaw(String.Format(CultureInfo.InvariantCulture, @"", SR2.GetString(SR2.HelpPageTitleText), this.methodNotAllowed ? SR2.GetString(SR2.HelpPageMethodNotAllowedText) : SR2.GetString(SR2.HelpPageText))); writer.WriteEndElement(); //HTML } } class HttpTransferRedirectMessage : ContentOnlyMessage { Uri newLocation; Uri originalTo; public HttpTransferRedirectMessage(Uri originalTo, Uri newLocation) : base() { this.originalTo = originalTo; this.newLocation = newLocation; } protected override void OnWriteBodyContents(XmlDictionaryWriter writer) { writer.WriteStartElement("HTML"); writer.WriteStartElement("HEAD"); writer.WriteRaw(String.Format(CultureInfo.InvariantCulture, @"{0}{0}
{1}
{1} ", SR2.GetString(SR2.HelpPageLayout), SR2.GetString(SR2.HelpPageTitleText))); writer.WriteEndElement(); //HEAD writer.WriteRaw(String.Format(CultureInfo.InvariantCulture, @"", SR2.GetString(SR2.HelpPageTitleText), SR2.GetString(SR2.RedirectPageText, this.originalTo.AbsoluteUri, this.newLocation.AbsoluteUri))); writer.WriteEndElement(); //HTML } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.{0}
{1}
Link Menu
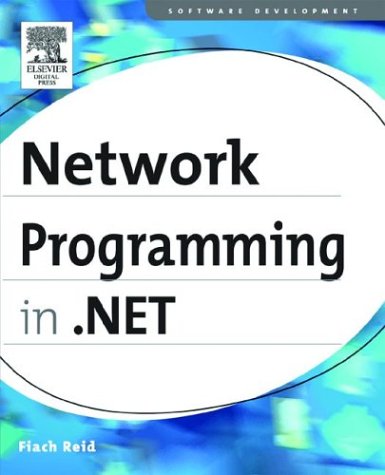
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DesignerDataTable.cs
- TrackingQuery.cs
- ToolStripEditorManager.cs
- RotateTransform3D.cs
- InsufficientExecutionStackException.cs
- DrawingGroup.cs
- AliasedSlot.cs
- GroupBox.cs
- IgnoreFlushAndCloseStream.cs
- TypedReference.cs
- BroadcastEventHelper.cs
- EntityDataSourceChangedEventArgs.cs
- CursorConverter.cs
- WsrmTraceRecord.cs
- CodeGenerator.cs
- AdapterUtil.cs
- MLangCodePageEncoding.cs
- FullTrustAssemblyCollection.cs
- TextBoxDesigner.cs
- LinqTreeNodeEvaluator.cs
- SerialPort.cs
- ServiceObjectContainer.cs
- MarkupCompilePass1.cs
- SplineKeyFrames.cs
- TableLayoutColumnStyleCollection.cs
- Configuration.cs
- RoleBoolean.cs
- CommandDesigner.cs
- _ListenerResponseStream.cs
- EllipseGeometry.cs
- TableRowCollection.cs
- ListViewAutomationPeer.cs
- SevenBitStream.cs
- XamlPoint3DCollectionSerializer.cs
- MarkupProperty.cs
- RelationshipNavigation.cs
- AmbientLight.cs
- MemberDomainMap.cs
- MessageEncodingBindingElementImporter.cs
- TransactionFlowBindingElementImporter.cs
- PinnedBufferMemoryStream.cs
- WriteStateInfoBase.cs
- StylusPointDescription.cs
- StorageComplexTypeMapping.cs
- HwndProxyElementProvider.cs
- MemberMaps.cs
- ProfileService.cs
- WhitespaceRule.cs
- HttpProfileGroupBase.cs
- EmissiveMaterial.cs
- ISCIIEncoding.cs
- columnmapfactory.cs
- EdmItemError.cs
- KeysConverter.cs
- VectorCollectionConverter.cs
- WebHttpEndpoint.cs
- MimeMapping.cs
- EventLogPermissionEntry.cs
- DecoderReplacementFallback.cs
- Path.cs
- CodeCatchClauseCollection.cs
- CancelEventArgs.cs
- LabelEditEvent.cs
- HttpRuntimeSection.cs
- ReachSerializer.cs
- UrlEncodedParameterWriter.cs
- SortedList.cs
- DesignerCapabilities.cs
- TextTreeTextBlock.cs
- TextRangeEditTables.cs
- ExpanderAutomationPeer.cs
- TreeViewHitTestInfo.cs
- XmlSchemaImport.cs
- SmiRecordBuffer.cs
- WebPartMinimizeVerb.cs
- EarlyBoundInfo.cs
- CorrelationResolver.cs
- CodeCastExpression.cs
- ExpressionBuilderCollection.cs
- CurrencyManager.cs
- SqlNotificationEventArgs.cs
- DataGridSortingEventArgs.cs
- BaseParagraph.cs
- BridgeDataReader.cs
- TdsParserHelperClasses.cs
- StringPropertyBuilder.cs
- IntegerFacetDescriptionElement.cs
- Html32TextWriter.cs
- WSHttpBindingCollectionElement.cs
- DynamicQueryableWrapper.cs
- ControlLocalizer.cs
- QilReference.cs
- BuildDependencySet.cs
- PersonalizationProviderHelper.cs
- ADMembershipUser.cs
- TypeSource.cs
- GlobalizationSection.cs
- xmlfixedPageInfo.cs
- DataGridViewControlCollection.cs
- PersonalizablePropertyEntry.cs