Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / Channels / InputQueueChannelAcceptor.cs / 1 / InputQueueChannelAcceptor.cs
//---------------------------------------------------------------------------- // Copyright (c) Microsoft Corporation. All rights reserved. //--------------------------------------------------------------------------- namespace System.ServiceModel.Channels { class InputQueueChannelAcceptor: ChannelAcceptor where TChannel : class, IChannel { InputQueue channelQueue; public InputQueueChannelAcceptor(ChannelManagerBase channelManager) : base(channelManager) { this.channelQueue = new InputQueue (); } public int PendingCount { get { return this.channelQueue.PendingCount; } } public override TChannel AcceptChannel(TimeSpan timeout) { this.ThrowIfNotOpened(); return this.channelQueue.Dequeue(timeout); } public override IAsyncResult BeginAcceptChannel(TimeSpan timeout, AsyncCallback callback, object state) { this.ThrowIfNotOpened(); return this.channelQueue.BeginDequeue(timeout, callback, state); } public void Dispatch() { this.channelQueue.Dispatch(); } public override TChannel EndAcceptChannel(IAsyncResult result) { return this.channelQueue.EndDequeue(result); } public void EnqueueAndDispatch(TChannel channel) { this.channelQueue.EnqueueAndDispatch(channel); } public void EnqueueAndDispatch(TChannel channel, ItemDequeuedCallback dequeuedCallback) { this.channelQueue.EnqueueAndDispatch(channel, dequeuedCallback); } public bool EnqueueWithoutDispatch(TChannel channel, ItemDequeuedCallback dequeuedCallback) { return this.channelQueue.EnqueueWithoutDispatch(channel, dequeuedCallback); } public virtual bool EnqueueWithoutDispatch(Exception exception, ItemDequeuedCallback dequeuedCallback) { return this.channelQueue.EnqueueWithoutDispatch(exception, dequeuedCallback); } public void EnqueueAndDispatch(TChannel channel, ItemDequeuedCallback dequeuedCallback, bool canDispatchOnThisThread) { this.channelQueue.EnqueueAndDispatch(channel, dequeuedCallback, canDispatchOnThisThread); } public virtual void EnqueueAndDispatch(Exception exception, ItemDequeuedCallback dequeuedCallback, bool canDispatchOnThisThread) { this.channelQueue.EnqueueAndDispatch(exception, dequeuedCallback, canDispatchOnThisThread); } public void FaultQueue() { this.Fault(); } protected override void OnClosed() { base.OnClosed(); this.channelQueue.Dispose(); } protected override void OnFaulted() { this.channelQueue.Shutdown(this.ChannelManager); base.OnFaulted(); } public override bool WaitForChannel(TimeSpan timeout) { this.ThrowIfNotOpened(); return this.channelQueue.WaitForItem(timeout); } public override IAsyncResult BeginWaitForChannel(TimeSpan timeout, AsyncCallback callback, object state) { this.ThrowIfNotOpened(); return this.channelQueue.BeginWaitForItem(timeout, callback, state); } public override bool EndWaitForChannel(IAsyncResult result) { return this.channelQueue.EndWaitForItem(result); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
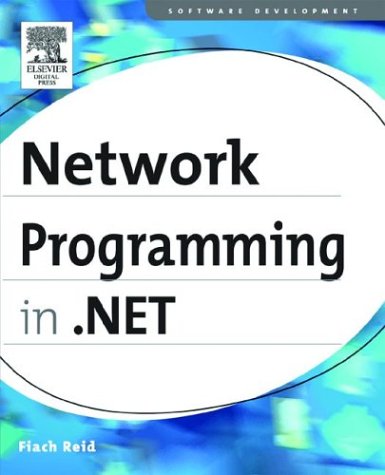
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- BigInt.cs
- WindowsTooltip.cs
- StringUtil.cs
- HwndSourceParameters.cs
- CustomAssemblyResolver.cs
- ConfigurationLocationCollection.cs
- CompoundFileIOPermission.cs
- CellTreeSimplifier.cs
- SqlIdentifier.cs
- ITreeGenerator.cs
- WebPartDisplayModeCancelEventArgs.cs
- DeploymentSectionCache.cs
- ToolStripItemEventArgs.cs
- ConfigurationException.cs
- PeerCollaborationPermission.cs
- RemotingClientProxy.cs
- DrawListViewColumnHeaderEventArgs.cs
- PictureBox.cs
- ExceptionHandlerDesigner.cs
- GlyphCollection.cs
- InitializationEventAttribute.cs
- SetIterators.cs
- HtmlTableRow.cs
- AstNode.cs
- Multiply.cs
- ConfigurationElement.cs
- HMACRIPEMD160.cs
- ObjectTag.cs
- TripleDES.cs
- ToolStripRenderer.cs
- FtpWebRequest.cs
- DataGridTextBox.cs
- HandledEventArgs.cs
- Socket.cs
- ActivityPropertyReference.cs
- GPRECTF.cs
- CurrentChangingEventArgs.cs
- ColorIndependentAnimationStorage.cs
- ResourceCategoryAttribute.cs
- TabControl.cs
- MergablePropertyAttribute.cs
- _SingleItemRequestCache.cs
- SessionStateUtil.cs
- OracleSqlParser.cs
- ScriptingSectionGroup.cs
- CodeExporter.cs
- DispatcherObject.cs
- MediaContext.cs
- TextTreeInsertUndoUnit.cs
- CapabilitiesUse.cs
- MouseButton.cs
- Lease.cs
- ApplicationProxyInternal.cs
- IndependentlyAnimatedPropertyMetadata.cs
- Monitor.cs
- FormatVersion.cs
- ParseElementCollection.cs
- MethodExpression.cs
- NCryptNative.cs
- SequentialUshortCollection.cs
- DetailsViewDeletedEventArgs.cs
- LateBoundBitmapDecoder.cs
- ToolboxComponentsCreatingEventArgs.cs
- UnmanagedMarshal.cs
- OptimizerPatterns.cs
- Evidence.cs
- DummyDataSource.cs
- EventPropertyMap.cs
- ResourceContainer.cs
- XPathAxisIterator.cs
- DataGridViewTextBoxColumn.cs
- SqlCacheDependencyDatabase.cs
- CompensatableTransactionScopeActivity.cs
- TableLayoutSettingsTypeConverter.cs
- DateTimeStorage.cs
- XPathDocument.cs
- FlowDocumentPageViewerAutomationPeer.cs
- SynchronizationContext.cs
- SrgsElementFactory.cs
- MouseButton.cs
- SuppressIldasmAttribute.cs
- TypeNameParser.cs
- PageContent.cs
- AnnotationHelper.cs
- SQLSingle.cs
- TokenBasedSet.cs
- SymmetricKeyWrap.cs
- WizardStepCollectionEditor.cs
- AppDomainShutdownMonitor.cs
- ClickablePoint.cs
- MetafileHeader.cs
- EventManager.cs
- LocationReference.cs
- WebPartDisplayModeEventArgs.cs
- SqlDataSourceEnumerator.cs
- ComplusTypeValidator.cs
- MultipleFilterMatchesException.cs
- DesignerSerializerAttribute.cs
- XPathNodePointer.cs
- DiagnosticsConfiguration.cs