Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / Dispatcher / ProxyOperationRuntime.cs / 3 / ProxyOperationRuntime.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.ServiceModel.Dispatcher { using System; using System.ServiceModel.Channels; using System.ServiceModel; using System.ServiceModel.Description; using System.Collections.ObjectModel; using System.Collections.Generic; using System.Reflection; using System.Runtime.Remoting.Proxies; using System.Runtime.Remoting; using System.Runtime.Remoting.Messaging; using System.ServiceModel.Diagnostics; using System.Security; class ProxyOperationRuntime { static internal readonly ParameterInfo[] NoParams = new ParameterInfo[0]; static internal readonly object[] EmptyArray = new object[0]; readonly IClientMessageFormatter formatter; readonly bool isInitiating; readonly bool isOneWay; readonly bool isTerminating; readonly string name; readonly IParameterInspector[] parameterInspectors; readonly IClientFaultFormatter faultFormatter; readonly ImmutableClientRuntime parent; bool serializeRequest; bool deserializeReply; string action; string replyAction; MethodInfo beginMethod; MethodInfo syncMethod; ParameterInfo[] inParams; ParameterInfo[] outParams; ParameterInfo[] endOutParams; ParameterInfo returnParam; internal ProxyOperationRuntime(ClientOperation operation, ImmutableClientRuntime parent) { if (operation == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("operation"); if (parent == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("parent"); this.parent = parent; this.formatter = operation.Formatter; this.isInitiating = operation.IsInitiating; this.isOneWay = operation.IsOneWay; this.isTerminating = operation.IsTerminating; this.name = operation.Name; this.parameterInspectors = EmptyArray.ToArray(operation.ParameterInspectors); this.faultFormatter = operation.FaultFormatter; this.serializeRequest = operation.SerializeRequest; this.deserializeReply = operation.DeserializeReply; this.action = operation.Action; this.replyAction = operation.ReplyAction; this.beginMethod = operation.BeginMethod; this.syncMethod = operation.SyncMethod; if (this.beginMethod != null) { this.inParams = ServiceReflector.GetInputParameters(this.beginMethod, true); if (this.syncMethod != null) { this.outParams = ServiceReflector.GetOutputParameters(this.syncMethod, false); } else { this.outParams = NoParams; } this.endOutParams = ServiceReflector.GetOutputParameters(operation.EndMethod, true); this.returnParam = operation.EndMethod.ReturnParameter; } else if (this.syncMethod != null) { this.inParams = ServiceReflector.GetInputParameters(this.syncMethod, false); this.outParams = ServiceReflector.GetOutputParameters(this.syncMethod, false); this.returnParam = this.syncMethod.ReturnParameter; } if (this.formatter == null && (serializeRequest || deserializeReply)) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new InvalidOperationException(SR.GetString(SR.ClientRuntimeRequiresFormatter0, this.name))); } } internal string Action { get { return this.action; } } internal IClientFaultFormatter FaultFormatter { get { return this.faultFormatter; } } internal bool IsInitiating { get { return this.isInitiating; } } internal bool IsOneWay { get { return this.isOneWay; } } internal bool IsTerminating { get { return this.isTerminating; } } internal string Name { get { return this.name; } } internal ImmutableClientRuntime Parent { get { return this.parent; } } internal string ReplyAction { get { return this.replyAction; } } internal bool DeserializeReply { get { return this.deserializeReply; } } internal bool SerializeRequest { get { return this.serializeRequest; } } internal void AfterReply(ref ProxyRpc rpc) { if (!this.isOneWay) { Message reply = rpc.Reply; if (this.deserializeReply) { rpc.ReturnValue = this.formatter.DeserializeReply(reply, rpc.OutputParameters); } else { rpc.ReturnValue = reply; } int offset = this.parent.ParameterInspectorCorrelationOffset; try { for (int i=parameterInspectors.Length-1; i>=0; i--) { this.parameterInspectors[i].AfterCall(this.name, rpc.OutputParameters, rpc.ReturnValue, rpc.Correlation[offset+i]); } } catch (Exception e) { if (DiagnosticUtility.IsFatal(e)) { throw; } if (ErrorBehavior.ShouldRethrowClientSideExceptionAsIs(e)) { throw; } throw DiagnosticUtility.ExceptionUtility.ThrowHelperCallback(e); } if (parent.ValidateMustUnderstand) { Collection headersNotUnderstood = reply.Headers.GetHeadersNotUnderstood(); if (headersNotUnderstood != null && headersNotUnderstood.Count > 0) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ProtocolException(SR.GetString(SR.SFxHeaderNotUnderstood, headersNotUnderstood[0].Name, headersNotUnderstood[0].Namespace))); } } } } internal void BeforeRequest(ref ProxyRpc rpc) { int offset = this.parent.ParameterInspectorCorrelationOffset; try { for (int i=0; i
Link Menu
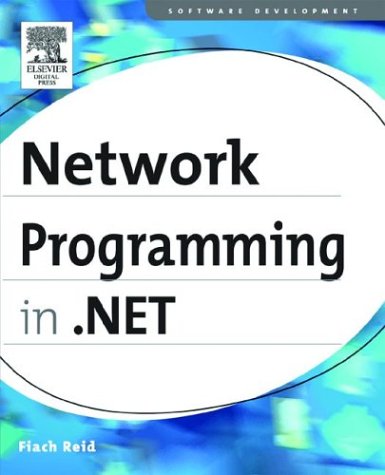
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- AssociationSetMetadata.cs
- smtpconnection.cs
- MILUtilities.cs
- EventManager.cs
- SelfIssuedSamlTokenFactory.cs
- FormatConvertedBitmap.cs
- ExclusiveCanonicalizationTransform.cs
- IntSecurity.cs
- OleDbConnectionInternal.cs
- PhysicalFontFamily.cs
- UnitControl.cs
- Adorner.cs
- WCFBuildProvider.cs
- CanonicalXml.cs
- ClonableStack.cs
- SpotLight.cs
- XmlSecureResolver.cs
- AuthenticationException.cs
- BaseCodePageEncoding.cs
- PiiTraceSource.cs
- ScrollProperties.cs
- SchemaElementLookUpTable.cs
- InternalBase.cs
- SizeAnimation.cs
- CollectionContainer.cs
- EntitySqlException.cs
- TaskFormBase.cs
- GroupLabel.cs
- InternalUserCancelledException.cs
- UpDownEvent.cs
- GridViewRowPresenter.cs
- FormsAuthenticationTicket.cs
- CancelEventArgs.cs
- XmlSchemaAttribute.cs
- MonthCalendarDesigner.cs
- NodeInfo.cs
- ThreadStateException.cs
- JsonServiceDocumentSerializer.cs
- PartialCachingAttribute.cs
- _NetRes.cs
- OdbcCommand.cs
- ResourceSet.cs
- NonClientArea.cs
- IMembershipProvider.cs
- Int32Collection.cs
- DetailsViewModeEventArgs.cs
- XMLSchema.cs
- AssemblyInfo.cs
- metadatamappinghashervisitor.cs
- XmlEntityReference.cs
- DataGridViewRowDividerDoubleClickEventArgs.cs
- UnsafeNativeMethods.cs
- HttpRawResponse.cs
- ListViewItem.cs
- ToolbarAUtomationPeer.cs
- JsonFormatReaderGenerator.cs
- DefaultValueTypeConverter.cs
- AsyncParams.cs
- ByteStack.cs
- lengthconverter.cs
- BooleanAnimationBase.cs
- GroupDescription.cs
- CodeTypeMemberCollection.cs
- TextDecorationCollectionConverter.cs
- ZipIOModeEnforcingStream.cs
- InfoCardAsymmetricCrypto.cs
- DictionarySectionHandler.cs
- Viewport2DVisual3D.cs
- WeakEventManager.cs
- HostingEnvironmentSection.cs
- Cursors.cs
- RtfToXamlLexer.cs
- StandardOleMarshalObject.cs
- AsmxEndpointPickerExtension.cs
- TypeDescriptor.cs
- IconConverter.cs
- DateTimeOffsetConverter.cs
- PeerNearMe.cs
- CrossContextChannel.cs
- ExpressionTextBoxAutomationPeer.cs
- Rights.cs
- Converter.cs
- SetStateEventArgs.cs
- RichTextBoxAutomationPeer.cs
- RelationshipConstraintValidator.cs
- DataServiceStreamResponse.cs
- State.cs
- UInt32.cs
- Vector3D.cs
- ListViewDesigner.cs
- TextElementCollectionHelper.cs
- DynamicPropertyHolder.cs
- CodeMethodInvokeExpression.cs
- DbBuffer.cs
- DataColumnMapping.cs
- HostProtectionException.cs
- DnsPermission.cs
- StreamWithDictionary.cs
- DataBoundControl.cs
- HtmlContainerControl.cs