Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / Tools / xws_reg / System / ServiceModel / Install / ServiceManagerHandle.cs / 1 / ServiceManagerHandle.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.ServiceModel.Install { using Microsoft.Win32.SafeHandles; using System; using System.ComponentModel; using System.Diagnostics; using System.Runtime.ConstrainedExecution; using System.Runtime.InteropServices; using System.Security.AccessControl; using System.Text; internal class ServiceManagerHandle : SafeHandleZeroOrMinusOneIsInvalid { internal ServiceManagerHandle() : base(true) { } internal ServiceHandle CreateService(string serviceName, string displayName, int serviceType, int startType, int errorControl, string binaryPathName, string dependencies, string serviceStartName) { const int desiredAccess = NativeMethods.SERVICE_CHANGE_CONFIG | // setting the description NativeMethods.SERVICE_START | // setting the failure actions NativeMethods.WRITE_DAC | // setting the DACL NativeMethods.ACCESS_SYSTEM_SECURITY; // setting the SACL #pragma warning suppress 56523 // [....]; Win32Exception default constructor calls Marshal.GetLastWin32Error() internally. ServiceHandle retVal = NativeMethods.CreateService(this, serviceName, displayName, desiredAccess, serviceType, startType, errorControl, binaryPathName, null, IntPtr.Zero, dependencies, serviceStartName, null); if ((retVal == null) || retVal.IsInvalid) { throw new Win32Exception(); } return retVal; } internal ServiceHandle OpenService(string serviceName, int desiredAccess) { #pragma warning suppress 56523 // [....]; Win32Exception default constructor calls Marshal.GetLastWin32Error() internally. ServiceHandle retVal = NativeMethods.OpenService(this, serviceName, desiredAccess); if ((null == retVal) || retVal.IsInvalid) { throw new Win32Exception(); } return retVal; } internal static ServiceManagerHandle OpenServiceManager() { #pragma warning suppress 56523 // [....]; Win32Exception default constructor calls Marshal.GetLastWin32Error() internally. ServiceManagerHandle retVal = NativeMethods.OpenSCManager(null, null, NativeMethods.SC_MANAGER_ALL_ACCESS); if ((null == retVal) || retVal.IsInvalid) { throw new Win32Exception(); } return retVal; } protected override bool ReleaseHandle() { #pragma warning suppress 56523 // [....]; Win32Exception default constructor calls Marshal.GetLastWin32Error() internally. bool retVal = NativeMethods.CloseServiceHandle(handle); if (!retVal) { throw new Win32Exception(); } return retVal; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
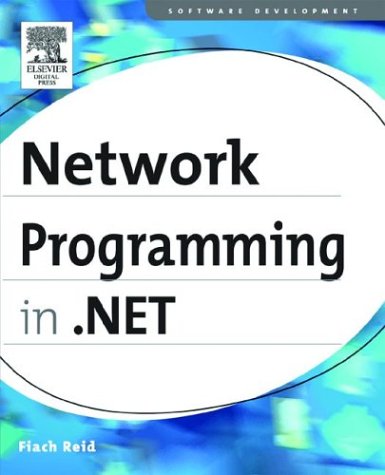
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- FixUp.cs
- _NestedSingleAsyncResult.cs
- Profiler.cs
- FormCollection.cs
- CallbackTimeoutsElement.cs
- IDQuery.cs
- PointLightBase.cs
- TemplatePagerField.cs
- FragmentQuery.cs
- DocumentSchemaValidator.cs
- EncoderBestFitFallback.cs
- WebContentFormatHelper.cs
- DataGridLinkButton.cs
- OneWayBindingElementImporter.cs
- BaseValidatorDesigner.cs
- InvalidCastException.cs
- Point.cs
- RawUIStateInputReport.cs
- InlineObject.cs
- FileIOPermission.cs
- XPathNavigatorException.cs
- QilReference.cs
- ThreadInterruptedException.cs
- BuildResult.cs
- DbResourceAllocator.cs
- ResourcePermissionBaseEntry.cs
- VersionConverter.cs
- TextElementEnumerator.cs
- ProxyManager.cs
- TdsEnums.cs
- XmlnsCache.cs
- MasterPageBuildProvider.cs
- VariableReference.cs
- SmtpCommands.cs
- DocumentXmlWriter.cs
- CTreeGenerator.cs
- ISAPIApplicationHost.cs
- InputLanguage.cs
- WebPartDescription.cs
- SQLBoolean.cs
- hebrewshape.cs
- _SSPIWrapper.cs
- FontStretch.cs
- InfoCardService.cs
- PackageProperties.cs
- PrivateFontCollection.cs
- MimeMultiPart.cs
- ComplexObject.cs
- StringInfo.cs
- DomNameTable.cs
- CollectionEditor.cs
- ManagedFilter.cs
- ChtmlTextWriter.cs
- __Filters.cs
- RangeValuePattern.cs
- ContextMenu.cs
- ThousandthOfEmRealDoubles.cs
- UnsafeNativeMethods.cs
- HashHelper.cs
- Evidence.cs
- SystemNetworkInterface.cs
- DataGridViewCellParsingEventArgs.cs
- AccessibilityApplicationManager.cs
- HijriCalendar.cs
- ChannelServices.cs
- XmlEntity.cs
- _CookieModule.cs
- TypeDependencyAttribute.cs
- HttpEncoderUtility.cs
- SqlRowUpdatedEvent.cs
- XmlHierarchicalEnumerable.cs
- Encoder.cs
- StateRuntime.cs
- CodeExpressionStatement.cs
- WsatServiceCertificate.cs
- LayoutUtils.cs
- NullableDoubleSumAggregationOperator.cs
- ComEventsInfo.cs
- BoolExpr.cs
- DataContext.cs
- ModuleBuilder.cs
- AssociationTypeEmitter.cs
- TreeNodeSelectionProcessor.cs
- CharConverter.cs
- FixedFlowMap.cs
- MenuAdapter.cs
- DummyDataSource.cs
- AbstractDataSvcMapFileLoader.cs
- XmlParserContext.cs
- OwnerDrawPropertyBag.cs
- AssemblyInfo.cs
- SmiRecordBuffer.cs
- WebControlAdapter.cs
- CellLabel.cs
- WebBrowserContainer.cs
- PersistChildrenAttribute.cs
- OracleColumn.cs
- MailDefinitionBodyFileNameEditor.cs
- NativeMethods.cs
- RIPEMD160Managed.cs