Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / infocard / Service / managed / Microsoft / InfoCards / UIAgentMonitor.cs / 1 / UIAgentMonitor.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- // // Presharp uses the c# pragma mechanism to supress its warnings. // These are not recognised by the base compiler so we need to explictly // disable the following warnings. See http://winweb/cse/Tools/PREsharp/userguide/default.asp // for details. // #pragma warning disable 1634, 1691 // unknown message, unknown pragma namespace Microsoft.InfoCards { using System; using System.Collections.Generic; using IDT = Microsoft.InfoCards.Diagnostics.InfoCardTrace; // // Summary // This class is used to make certain that only one user call and only one client per TS session can // use the infocard system. // class UIAgentMonitor { static UIAgentMonitor s_singleton = null; static object s_syncRoot = new object(); // // Dictionary of currently running users. // Dictionarym_currentCallingUsers; // user name to request dictionary. Dictionary m_currentTSSessions; // tssession to request dictionary. private UIAgentMonitor() { m_currentCallingUsers = new Dictionary (); m_currentTSSessions = new Dictionary (); } // // Summary // Returns the singleton instance of the monitor, creating it if necessary. // static public UIAgentMonitor Instance() { // // Has it been created yet? // if ( null == s_singleton ) { // // Doesn't look like it try for the lock. // lock ( s_syncRoot ) { // // Was this the first thread to get the lock? // if ( null == s_singleton ) { // // Yes, this thread needs to create the monitor. // s_singleton = new UIAgentMonitor(); } } } return s_singleton; } // // Summary // Adds a new UIAgentMonitorHandle to the collection if the user and tssession associated with the request // are not already occupied. If they are then this method throws the ServiceBusyException. // // Parameters // request - The request to add. // public void AddNewClient( UIAgentMonitorHandle handle ) { lock ( s_syncRoot ) { string user = handle.UserName; int tssession = handle.TsSessionId; if ( m_currentCallingUsers.ContainsKey( user ) ) { throw IDT.ThrowHelperError( new ServiceBusyException( SR.GetString( SR.ServiceInUseOnAnotherSession ) ) ); } if ( m_currentTSSessions.ContainsKey( tssession ) ) { throw IDT.ThrowHelperError( new ServiceBusyException( SR.GetString( SR.ServiceInUseOnAnotherSession ) ) ); } m_currentCallingUsers.Add( user, handle ); try { m_currentTSSessions.Add( tssession, handle ); } catch( Exception ) { m_currentCallingUsers.Remove( user ); throw; } } } // // Summary // Removes a UIAgentMonitorHandle from the collection if this specific request is in the collection. // // Parameters // request - the request to remove. // public void RemoveClient( UIAgentMonitorHandle handle ) { lock ( s_syncRoot ) { string user = handle.UserName; int tssession = handle.TsSessionId; if ( ( !string.IsNullOrEmpty( user ) ) && ( m_currentCallingUsers.ContainsKey( user ) ) && ( handle == m_currentCallingUsers[ user ] ) ) { m_currentCallingUsers.Remove( user ); } if ( ( m_currentTSSessions.ContainsKey( tssession ) ) && ( handle == m_currentTSSessions[ tssession ] ) ) { m_currentTSSessions.Remove( tssession ); } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
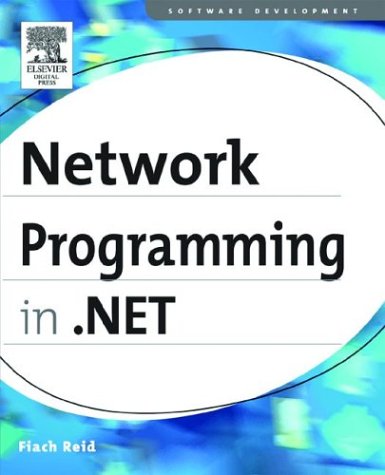
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- QueryOperationResponseOfT.cs
- CommandSet.cs
- LoginStatusDesigner.cs
- COM2IManagedPerPropertyBrowsingHandler.cs
- QuaternionValueSerializer.cs
- SHA384.cs
- ToolStrip.cs
- HttpMethodConstraint.cs
- GrammarBuilderRuleRef.cs
- DataServiceEntityAttribute.cs
- TableStyle.cs
- NameTable.cs
- ChooseAction.cs
- ApplicationException.cs
- BaseProcessProtocolHandler.cs
- ExtensionFile.cs
- StylusCaptureWithinProperty.cs
- CommonObjectSecurity.cs
- SymbolEqualComparer.cs
- Block.cs
- AutomationPattern.cs
- GenericWebPart.cs
- AuthenticationModulesSection.cs
- WebPartManagerInternals.cs
- WebRequest.cs
- Command.cs
- SchemaInfo.cs
- WebPartAddingEventArgs.cs
- TextDecorationCollectionConverter.cs
- VirtualPathUtility.cs
- EndEvent.cs
- SpeakCompletedEventArgs.cs
- MenuItemStyle.cs
- HttpMethodConstraint.cs
- TextElementEditingBehaviorAttribute.cs
- UniformGrid.cs
- ToolStripDropDownDesigner.cs
- Label.cs
- RoleManagerModule.cs
- FrameworkElementFactoryMarkupObject.cs
- ProtocolException.cs
- GenericsNotImplementedException.cs
- ThreadStateException.cs
- XmlCustomFormatter.cs
- Int32Collection.cs
- IdentityNotMappedException.cs
- ITreeGenerator.cs
- XPathNodeHelper.cs
- TemplateBindingExpression.cs
- TextRunCache.cs
- InternalControlCollection.cs
- CallSiteHelpers.cs
- ConfigurationLocationCollection.cs
- ObjectListFieldsPage.cs
- Menu.cs
- ThreadExceptionDialog.cs
- ClipboardData.cs
- XmlDataDocument.cs
- SoapParser.cs
- PassportAuthentication.cs
- ConstNode.cs
- ComponentDispatcherThread.cs
- DataGridTableStyleMappingNameEditor.cs
- FileUtil.cs
- DataSourceSerializationException.cs
- HttpServerChannel.cs
- RedirectionProxy.cs
- AppDomainProtocolHandler.cs
- HttpRuntimeSection.cs
- Viewport3DAutomationPeer.cs
- ExecutedRoutedEventArgs.cs
- FigureHelper.cs
- SvcMapFileSerializer.cs
- ToolStripItem.cs
- MSG.cs
- SplitContainer.cs
- WindowsFont.cs
- AssemblySettingAttributes.cs
- AtomMaterializerLog.cs
- SystemEvents.cs
- PageHandlerFactory.cs
- XmlSyndicationContent.cs
- SqlDataSourceConnectionPanel.cs
- LassoSelectionBehavior.cs
- IntranetCredentialPolicy.cs
- SerTrace.cs
- List.cs
- GridItemPattern.cs
- DataControlLinkButton.cs
- OpenTypeCommon.cs
- DataGridViewCheckBoxColumn.cs
- TrackingMemoryStream.cs
- Crypto.cs
- RegisteredDisposeScript.cs
- ResourceWriter.cs
- MemoryStream.cs
- ZipIOCentralDirectoryDigitalSignature.cs
- UnauthorizedAccessException.cs
- AssertSection.cs
- wgx_exports.cs