Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / infocard / Service / managed / Microsoft / InfoCards / UIAgentRequest.cs / 1 / UIAgentRequest.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- // // Presharp uses the c# pragma mechanism to supress its warnings. // These are not recognised by the base compiler so we need to explictly // disable the following warnings. See http://winweb/cse/Tools/PREsharp/userguide/default.asp // for details. // #pragma warning disable 1634, 1691 // unknown message, unknown pragma namespace Microsoft.InfoCards { using System; using System.Collections.Generic; using System.ComponentModel; using System.Diagnostics; using System.Security; //SecurityException using IDT = Microsoft.InfoCards.Diagnostics.InfoCardTrace; using System.Security.Principal; //WindowsIdentity using Microsoft.Win32.SafeHandles; //SafeWaitHandle using System.IO; //Stream using System.Runtime.InteropServices; // // Summary: Base class to represent UI Agent RPC requests // internal abstract class UIAgentRequest : Request { // Holds the parent request that this UI Agent RPC call was initiated by ClientUIRequest m_parentRequest; // // Summary: Constructor to initialize member variables // public UIAgentRequest( IntPtr rpcHandle, Stream inArgs, Stream outArgs, ClientUIRequest parent ) : this( rpcHandle, inArgs, outArgs, parent, ExceptionList.Empty ) { } // // Summary: Constructor to initialize member variables // public UIAgentRequest( IntPtr rpcHandle, Stream inArgs, Stream outArgs, ClientUIRequest parent, ExceptionList exceptionList ) : base( rpcHandle, inArgs, outArgs, exceptionList ) { m_parentRequest = parent; } public ClientUIRequest ParentRequest { get { return m_parentRequest; } } protected InfoCardUIAgent UIAgent { get { if( null != ParentRequest ) { return ParentRequest.UIAgent; } return null; } } // // Summary: // Called by native RPC code in order to pre-authorize the incoming UI Agent. // // Remarks: // If this method returns a non zero result then the ui agent will be denied access to any // of the UI Agent RPC interface functions. // // Parameters: // rpcIfHandle - an RPC interface handle. // context - an RPC context handle. // static public uint Authorize( IntPtr rpcIfHandle, IntPtr context ) { uint err = NativeMethods.ERROR_ACCESS_DENIED; if( IntPtr.Zero == rpcIfHandle ) { throw IDT.ThrowHelperArgumentNull( "rpcIfHandle" ); } if( IntPtr.Zero == context ) { throw IDT.ThrowHelperArgumentNull( "context" ); } try { WindowsIdentity id = Utility.GetWindowsIdentity( context ); try { // // The calling process should be in our list of PID's // created and additionally, the PID should have the // matching trusted user SID associated with that PID. // uint pid = Utility.GetRpcClientPid( context ); IDT.TraceDebug( "Incoming process id is {0}", pid); InfoCardUIAgent icUIAgent = InfoCardUIAgent.FindByPid( pid ); if ( null == icUIAgent ) { throw IDT.ThrowHelperError( new SecurityException() ); } // // Verify the trusted user SID. // NativeMcppMethods.CheckSIDAgainstCurrentRpcUser( icUIAgent.TrustedUserSid ); // // The client passed all checks, let them in. // err = 0; } finally { id.Dispose(); } } catch ( SecurityException ) { IDT.Assert( NativeMethods.ERROR_ACCESS_DENIED == err, "Unexpected value for err!" ); } #pragma warning suppress 56500 catch ( Exception e ) { InfoCardService.Crash( e ); } #pragma warning restore 56500 return err; } // // Summary: // Called by native code in order to get the GetWorkCallback delegate for this instance of the uiagent. // // Parameters: // processId - The pid of the calling process. // nativeEventHandle - A native event handle that this class can use to get the attention of the // UI Agent process. // callback - The GetWorkCallback delegate to be returned. // static public void BindToService( IntPtr uiagentRpcHandle, IntPtr nativeDesktopName, int cbUserSid, IntPtr pUserSid, int cbTrustedSid, IntPtr pTrustedSid, out IntPtr nativeStartEventHandle, out IntPtr nativeCompleteEventHandle, out RpcUIAgentGetWorkCallback callback ) { string desktopName = Marshal.PtrToStringUni( nativeDesktopName ); callback = null; nativeStartEventHandle = IntPtr.Zero; nativeCompleteEventHandle = IntPtr.Zero; using( WindowsIdentity identity = Utility.GetWindowsIdentity( uiagentRpcHandle ) ) { WindowsImpersonationContext impersonationContext = identity.Impersonate(); try { uint processId; int err = (int)NativeMethods.I_RpcBindingInqLocalClientPID( uiagentRpcHandle, out processId ); if( 0 != err ) { throw IDT.ThrowHelperError( new CommunicationException( SR.GetString( SR.FailedToBindToService ) ) ); } InfoCardUIAgent uiagent = InfoCardUIAgent.FindByPid( processId ); if( null != uiagent ) { // // bind to the UIAgent. // SafeWaitHandle hStartEvent; SafeWaitHandle hCompleteEvent; SecurityIdentifier trustedSid; callback = uiagent.Bind( desktopName, out trustedSid, out hStartEvent, out hCompleteEvent ); // // Copy the sids to the output buffer. // byte[] buffer = new byte[ Math.Max( cbUserSid, cbTrustedSid ) ]; identity.User.GetBinaryForm( buffer, 0 ); Marshal.Copy( buffer, 0, pUserSid, cbUserSid ); trustedSid.GetBinaryForm( buffer, 0 ); Marshal.Copy( buffer, 0, pTrustedSid, cbTrustedSid ); // // Capture the remove event handle. // nativeStartEventHandle = hStartEvent.DangerousGetHandle(); nativeCompleteEventHandle = hCompleteEvent.DangerousGetHandle(); } } finally { impersonationContext.Undo(); } } } public override WindowsIdentity RequestorIdentity { get{ return ParentRequest.RequestorIdentity; } } protected override void OnInitializeAsSystem() { try { // // Do an access check to make certain that the incoming user has the correct // truster user sid. // NativeMcppMethods.CheckSIDAgainstCurrentRpcUser( ParentRequest.UIAgentLogonSid ); } catch( Win32Exception e ) { throw IDT.ThrowHelperError( new InfoCardArgumentException( SR.GetString( SR.UnableToAuthenticateUIAgent ), e ) ); } catch( SecurityException e ) { throw IDT.ThrowHelperError( new InfoCardArgumentException( SR.GetString( SR.UnableToAuthenticateUIAgent ), e ) ); } } // // Summary: // Retrive policy if parentrequest is of GetTokenRequest. Return null otherwise // protected InfoCardPolicy GetPolicy() { GetTokenRequest gtr = ParentRequest as GetTokenRequest; if ( gtr == null ) { return null; } else { return gtr.Policy; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
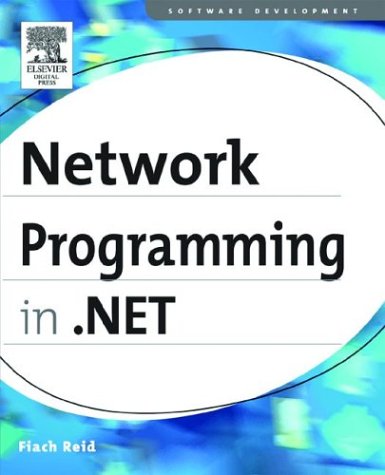
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HMACRIPEMD160.cs
- Application.cs
- NativeMsmqMessage.cs
- Color.cs
- RouteUrlExpressionBuilder.cs
- DataGridTextColumn.cs
- KeyEventArgs.cs
- GeneralTransform3DTo2D.cs
- FileDialog.cs
- OletxEnlistment.cs
- OutOfMemoryException.cs
- ApplyTemplatesAction.cs
- TextEditorSpelling.cs
- ViewService.cs
- SymLanguageVendor.cs
- DrawTreeNodeEventArgs.cs
- Pair.cs
- LocationSectionRecord.cs
- DataGridPageChangedEventArgs.cs
- RuleSettingsCollection.cs
- AssemblyAssociatedContentFileAttribute.cs
- SchemaNamespaceManager.cs
- DesignTimeVisibleAttribute.cs
- TableItemStyle.cs
- AssociationSetMetadata.cs
- SpeakInfo.cs
- PtsCache.cs
- CodePropertyReferenceExpression.cs
- HtmlShim.cs
- LoginName.cs
- DeploymentSection.cs
- StringCollectionEditor.cs
- ThreadPool.cs
- MatrixAnimationUsingPath.cs
- SmiMetaDataProperty.cs
- MethodImplAttribute.cs
- ContentPropertyAttribute.cs
- CodeThrowExceptionStatement.cs
- FamilyMap.cs
- DiagnosticsConfiguration.cs
- OutputCacheSection.cs
- FormViewUpdatedEventArgs.cs
- RequiredAttributeAttribute.cs
- EventLogTraceListener.cs
- WindowsFormsHost.cs
- SecurityDescriptor.cs
- OpenTypeLayout.cs
- CaseInsensitiveComparer.cs
- AbstractSvcMapFileLoader.cs
- OleDbParameter.cs
- DrawingBrush.cs
- HttpCookieCollection.cs
- FixedLineResult.cs
- SqlBulkCopyColumnMappingCollection.cs
- TextParagraphCache.cs
- BinaryExpressionHelper.cs
- SplineKeyFrames.cs
- BaseAddressPrefixFilterElementCollection.cs
- DynamicILGenerator.cs
- ResourceManager.cs
- loginstatus.cs
- TableItemProviderWrapper.cs
- RSACryptoServiceProvider.cs
- FunctionDescription.cs
- ModuleBuilderData.cs
- TypeInfo.cs
- Component.cs
- ConsoleKeyInfo.cs
- QueryExecutionOption.cs
- DataGridColumnEventArgs.cs
- Schema.cs
- PageBreakRecord.cs
- TdsParserSessionPool.cs
- EditorZone.cs
- SqlCrossApplyToCrossJoin.cs
- FrameworkElementAutomationPeer.cs
- ObjectListCommandCollection.cs
- BitmapEffectGeneralTransform.cs
- ManagementOptions.cs
- WhereQueryOperator.cs
- CompModHelpers.cs
- TextDecorationUnitValidation.cs
- StartUpEventArgs.cs
- MsmqMessageProperty.cs
- DbProviderFactory.cs
- SemanticResolver.cs
- TreeNodeStyleCollection.cs
- WmlMobileTextWriter.cs
- FontInfo.cs
- HyperLink.cs
- TextEffect.cs
- Propagator.Evaluator.cs
- ObjectDataSourceFilteringEventArgs.cs
- WizardPanel.cs
- Debug.cs
- DurableInstanceProvider.cs
- SequenceDesigner.cs
- StorageInfo.cs
- StaticDataManager.cs
- AttributeInfo.cs