Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Core / System / Security / Cryptography / X509Certificates / TimestampInformation.cs / 1305376 / TimestampInformation.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== using System; using System.Diagnostics; using System.Security.Cryptography; using System.Security.Permissions; namespace System.Security.Cryptography.X509Certificates { ////// Details about the timestamp applied to a manifest's Authenticode signature /// [System.Security.Permissions.HostProtection(MayLeakOnAbort = true)] public sealed class TimestampInformation { private CapiNative.AlgorithmId m_hashAlgorithmId; private DateTime m_timestamp; private X509Chain m_timestampChain; private SignatureVerificationResult m_verificationResult; private X509Certificate2 m_timestamper; //// [System.Security.SecurityCritical] internal TimestampInformation(X509Native.AXL_AUTHENTICODE_TIMESTAMPER_INFO timestamper) { m_hashAlgorithmId = timestamper.algHash; m_verificationResult = (SignatureVerificationResult)timestamper.dwError; ulong filetime = ((ulong)((uint)timestamper.ftTimestamp.dwHighDateTime) << 32) | (ulong)((uint)timestamper.ftTimestamp.dwLowDateTime); m_timestamp = DateTime.FromFileTimeUtc((long)filetime); if (timestamper.pChainContext != IntPtr.Zero) { m_timestampChain = new X509Chain(timestamper.pChainContext); } } internal TimestampInformation(SignatureVerificationResult error) { Debug.Assert(error != SignatureVerificationResult.Valid, "error != SignatureVerificationResult.Valid"); m_verificationResult = error; } ///// /// Hash algorithm the timestamp signature was calculated with /// public string HashAlgorithm { get { return CapiNative.GetAlgorithmName(m_hashAlgorithmId); } } ////// HRESULT from verifying the timestamp /// public int HResult { get { return CapiNative.HResultForVerificationResult(m_verificationResult); } } ////// Is the signature of the timestamp valid /// public bool IsValid { get { // Timestamp signatures are valid only if they were created by a trusted chain return VerificationResult == SignatureVerificationResult.Valid || VerificationResult == SignatureVerificationResult.CertificateNotExplicitlyTrusted; } } ////// Chain of certificates used to verify the timestamp /// public X509Chain SignatureChain { [StorePermission(SecurityAction.Demand, OpenStore = true, EnumerateCertificates = true)] get { return m_timestampChain; } } ////// Certificate that signed the timestamp /// public X509Certificate2 SigningCertificate { [StorePermission(SecurityAction.Demand, OpenStore = true, EnumerateCertificates = true)] get { if (m_timestamper == null && SignatureChain != null) { m_timestamper = SignatureChain.ChainElements[0].Certificate; } return m_timestamper; } } ////// When the timestamp was applied, expressed in local time /// public DateTime Timestamp { get { return m_timestamp.ToLocalTime(); } } ////// Result of verifying the timestamp signature /// public SignatureVerificationResult VerificationResult { get { return m_verificationResult; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== using System; using System.Diagnostics; using System.Security.Cryptography; using System.Security.Permissions; namespace System.Security.Cryptography.X509Certificates { ////// Details about the timestamp applied to a manifest's Authenticode signature /// [System.Security.Permissions.HostProtection(MayLeakOnAbort = true)] public sealed class TimestampInformation { private CapiNative.AlgorithmId m_hashAlgorithmId; private DateTime m_timestamp; private X509Chain m_timestampChain; private SignatureVerificationResult m_verificationResult; private X509Certificate2 m_timestamper; //// [System.Security.SecurityCritical] internal TimestampInformation(X509Native.AXL_AUTHENTICODE_TIMESTAMPER_INFO timestamper) { m_hashAlgorithmId = timestamper.algHash; m_verificationResult = (SignatureVerificationResult)timestamper.dwError; ulong filetime = ((ulong)((uint)timestamper.ftTimestamp.dwHighDateTime) << 32) | (ulong)((uint)timestamper.ftTimestamp.dwLowDateTime); m_timestamp = DateTime.FromFileTimeUtc((long)filetime); if (timestamper.pChainContext != IntPtr.Zero) { m_timestampChain = new X509Chain(timestamper.pChainContext); } } internal TimestampInformation(SignatureVerificationResult error) { Debug.Assert(error != SignatureVerificationResult.Valid, "error != SignatureVerificationResult.Valid"); m_verificationResult = error; } ///// /// Hash algorithm the timestamp signature was calculated with /// public string HashAlgorithm { get { return CapiNative.GetAlgorithmName(m_hashAlgorithmId); } } ////// HRESULT from verifying the timestamp /// public int HResult { get { return CapiNative.HResultForVerificationResult(m_verificationResult); } } ////// Is the signature of the timestamp valid /// public bool IsValid { get { // Timestamp signatures are valid only if they were created by a trusted chain return VerificationResult == SignatureVerificationResult.Valid || VerificationResult == SignatureVerificationResult.CertificateNotExplicitlyTrusted; } } ////// Chain of certificates used to verify the timestamp /// public X509Chain SignatureChain { [StorePermission(SecurityAction.Demand, OpenStore = true, EnumerateCertificates = true)] get { return m_timestampChain; } } ////// Certificate that signed the timestamp /// public X509Certificate2 SigningCertificate { [StorePermission(SecurityAction.Demand, OpenStore = true, EnumerateCertificates = true)] get { if (m_timestamper == null && SignatureChain != null) { m_timestamper = SignatureChain.ChainElements[0].Certificate; } return m_timestamper; } } ////// When the timestamp was applied, expressed in local time /// public DateTime Timestamp { get { return m_timestamp.ToLocalTime(); } } ////// Result of verifying the timestamp signature /// public SignatureVerificationResult VerificationResult { get { return m_verificationResult; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
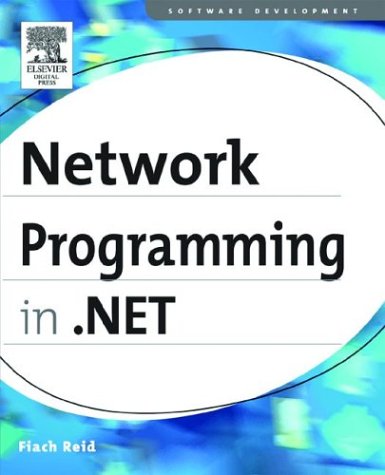
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ProxyWebPartConnectionCollection.cs
- ParseNumbers.cs
- CultureTableRecord.cs
- DrawTreeNodeEventArgs.cs
- SettingsProviderCollection.cs
- ProxyElement.cs
- RootBrowserWindowAutomationPeer.cs
- SystemIPv6InterfaceProperties.cs
- DataServiceRequestOfT.cs
- Helper.cs
- RichTextBoxAutomationPeer.cs
- SiteMapSection.cs
- DotExpr.cs
- DbTransaction.cs
- SqlClientFactory.cs
- FixedSOMImage.cs
- TemplateContent.cs
- OrderedHashRepartitionStream.cs
- ElementFactory.cs
- EditorResources.cs
- _ScatterGatherBuffers.cs
- ListControlConvertEventArgs.cs
- _SslStream.cs
- WindowsPrincipal.cs
- MenuBase.cs
- SliderAutomationPeer.cs
- OperatingSystemVersionCheck.cs
- SpotLight.cs
- ResourcePart.cs
- GenericArgumentsUpdater.cs
- SmtpCommands.cs
- Matrix3DValueSerializer.cs
- glyphs.cs
- BamlBinaryWriter.cs
- SoapAttributes.cs
- RegexTree.cs
- XsltLoader.cs
- BindMarkupExtensionSerializer.cs
- MemoryPressure.cs
- ToolboxCategoryItems.cs
- CommandValueSerializer.cs
- DataListCommandEventArgs.cs
- QilInvoke.cs
- DecimalConverter.cs
- DataGridHeaderBorder.cs
- DataGridViewCellContextMenuStripNeededEventArgs.cs
- PieceDirectory.cs
- IOException.cs
- PeerObject.cs
- SelectionProcessor.cs
- Matrix3DStack.cs
- RequestUriProcessor.cs
- AsmxEndpointPickerExtension.cs
- StandardCommands.cs
- SqlFileStream.cs
- Panel.cs
- TextRangeAdaptor.cs
- BulletedListEventArgs.cs
- InstanceDataCollectionCollection.cs
- MSAANativeProvider.cs
- DataGridLinkButton.cs
- LayoutDump.cs
- PreProcessor.cs
- DataStreams.cs
- CodeMemberEvent.cs
- HighlightVisual.cs
- SecureStringHasher.cs
- AspNetHostingPermission.cs
- XPathNodeInfoAtom.cs
- IPAddress.cs
- VisualProxy.cs
- PatternMatcher.cs
- InnerItemCollectionView.cs
- SeekStoryboard.cs
- PolyLineSegmentFigureLogic.cs
- Automation.cs
- PrinterUnitConvert.cs
- Point.cs
- DataGrid.cs
- LazyTextWriterCreator.cs
- ModifiableIteratorCollection.cs
- XmlDataCollection.cs
- Timeline.cs
- XmlSerializationGeneratedCode.cs
- HwndHost.cs
- ExtensionDataObject.cs
- ItemCollection.cs
- ProfilePropertySettingsCollection.cs
- DiscoveryEndpointValidator.cs
- InfoCardUIAgent.cs
- SessionParameter.cs
- XmlNavigatorStack.cs
- AlphabeticalEnumConverter.cs
- C14NUtil.cs
- HtmlElement.cs
- FixedDocument.cs
- WebPartMenuStyle.cs
- WsdlWriter.cs
- Matrix3DStack.cs
- DateTimeHelper.cs