Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / xsp / System / Web / Compilation / XsdBuildProvider.cs / 1601920 / XsdBuildProvider.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Compilation { using System; using System.IO; using System.Data; using System.Data.Design; using System.Globalization; using System.Xml; using System.Xml.Schema; using System.Reflection; using System.CodeDom; using System.CodeDom.Compiler; using System.Web.Hosting; using System.Web.Configuration; using System.Collections; using Util=System.Web.UI.Util; #if !FEATURE_PAL // FEATURE_PAL does not support System.Data.Design using TypedDataSetGenerator=System.Data.Design.TypedDataSetGenerator; #endif // !FEATURE_PAL [BuildProviderAppliesTo(BuildProviderAppliesTo.Code)] internal class XsdBuildProvider: BuildProvider { public override void GenerateCode(AssemblyBuilder assemblyBuilder) { #if !FEATURE_PAL // FEATURE_PAL does not support System.Data.Design // Get the namespace that we will use string ns = Util.GetNamespaceFromVirtualPath(VirtualPathObject); // We need to use XmlDocument to parse the xsd file is order to open it with the // correct encoding (VSWhidbey 566286) XmlDocument doc = new XmlDocument(); using (Stream stream = OpenStream()) { doc.Load(stream); } String content = doc.OuterXml; // Generate a CodeCompileUnit from the dataset CodeCompileUnit codeCompileUnit = new CodeCompileUnit(); CodeNamespace codeNamespace = new CodeNamespace(ns); codeCompileUnit.Namespaces.Add(codeNamespace); // Devdiv 18365, Dev10 bug 444516 // Call a different Generate method if compiler version is v3.5 or above bool isVer35OrAbove = CompilationUtil.IsCompilerVersion35OrAbove(assemblyBuilder.CodeDomProvider.GetType()); if (isVer35OrAbove) { TypedDataSetGenerator.GenerateOption generateOptions = TypedDataSetGenerator.GenerateOption.None; generateOptions |= TypedDataSetGenerator.GenerateOption.HierarchicalUpdate; generateOptions |= TypedDataSetGenerator.GenerateOption.LinqOverTypedDatasets; Hashtable customDBProviders = null; TypedDataSetGenerator.Generate(content, codeCompileUnit, codeNamespace, assemblyBuilder.CodeDomProvider, customDBProviders, generateOptions); } else { TypedDataSetGenerator.Generate(content, codeCompileUnit, codeNamespace, assemblyBuilder.CodeDomProvider); } // Add all the assembly references needed by the generated code if (TypedDataSetGenerator.ReferencedAssemblies != null) { var isVer35 = CompilationUtil.IsCompilerVersion35(assemblyBuilder.CodeDomProvider.GetType()); foreach (Assembly a in TypedDataSetGenerator.ReferencedAssemblies) { if (isVer35) { var aName = a.GetName(); if (aName.Name == "System.Data.DataSetExtensions") { // Dev10 Bug 861688 - We need to specify v3.5 version so that the build system knows to use the v3.5 version // because the loaded assembly here is always v4.0 aName.Version = new Version(3, 5, 0, 0); CompilationSection.RecordAssembly(aName.FullName, a); } } assemblyBuilder.AddAssemblyReference(a); } } // Add the CodeCompileUnit to the compilation assemblyBuilder.AddCodeCompileUnit(this, codeCompileUnit); #else // !FEATURE_PAL throw new NotImplementedException("System.Data.Design - ROTORTODO"); #endif // !FEATURE_PAL } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Compilation { using System; using System.IO; using System.Data; using System.Data.Design; using System.Globalization; using System.Xml; using System.Xml.Schema; using System.Reflection; using System.CodeDom; using System.CodeDom.Compiler; using System.Web.Hosting; using System.Web.Configuration; using System.Collections; using Util=System.Web.UI.Util; #if !FEATURE_PAL // FEATURE_PAL does not support System.Data.Design using TypedDataSetGenerator=System.Data.Design.TypedDataSetGenerator; #endif // !FEATURE_PAL [BuildProviderAppliesTo(BuildProviderAppliesTo.Code)] internal class XsdBuildProvider: BuildProvider { public override void GenerateCode(AssemblyBuilder assemblyBuilder) { #if !FEATURE_PAL // FEATURE_PAL does not support System.Data.Design // Get the namespace that we will use string ns = Util.GetNamespaceFromVirtualPath(VirtualPathObject); // We need to use XmlDocument to parse the xsd file is order to open it with the // correct encoding (VSWhidbey 566286) XmlDocument doc = new XmlDocument(); using (Stream stream = OpenStream()) { doc.Load(stream); } String content = doc.OuterXml; // Generate a CodeCompileUnit from the dataset CodeCompileUnit codeCompileUnit = new CodeCompileUnit(); CodeNamespace codeNamespace = new CodeNamespace(ns); codeCompileUnit.Namespaces.Add(codeNamespace); // Devdiv 18365, Dev10 bug 444516 // Call a different Generate method if compiler version is v3.5 or above bool isVer35OrAbove = CompilationUtil.IsCompilerVersion35OrAbove(assemblyBuilder.CodeDomProvider.GetType()); if (isVer35OrAbove) { TypedDataSetGenerator.GenerateOption generateOptions = TypedDataSetGenerator.GenerateOption.None; generateOptions |= TypedDataSetGenerator.GenerateOption.HierarchicalUpdate; generateOptions |= TypedDataSetGenerator.GenerateOption.LinqOverTypedDatasets; Hashtable customDBProviders = null; TypedDataSetGenerator.Generate(content, codeCompileUnit, codeNamespace, assemblyBuilder.CodeDomProvider, customDBProviders, generateOptions); } else { TypedDataSetGenerator.Generate(content, codeCompileUnit, codeNamespace, assemblyBuilder.CodeDomProvider); } // Add all the assembly references needed by the generated code if (TypedDataSetGenerator.ReferencedAssemblies != null) { var isVer35 = CompilationUtil.IsCompilerVersion35(assemblyBuilder.CodeDomProvider.GetType()); foreach (Assembly a in TypedDataSetGenerator.ReferencedAssemblies) { if (isVer35) { var aName = a.GetName(); if (aName.Name == "System.Data.DataSetExtensions") { // Dev10 Bug 861688 - We need to specify v3.5 version so that the build system knows to use the v3.5 version // because the loaded assembly here is always v4.0 aName.Version = new Version(3, 5, 0, 0); CompilationSection.RecordAssembly(aName.FullName, a); } } assemblyBuilder.AddAssemblyReference(a); } } // Add the CodeCompileUnit to the compilation assemblyBuilder.AddCodeCompileUnit(this, codeCompileUnit); #else // !FEATURE_PAL throw new NotImplementedException("System.Data.Design - ROTORTODO"); #endif // !FEATURE_PAL } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
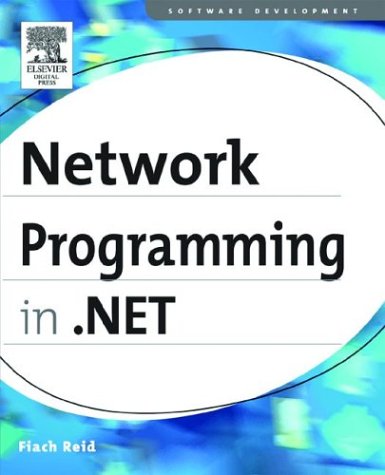
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- BufferedGraphicsContext.cs
- FontConverter.cs
- ValueUtilsSmi.cs
- BitmapEffectDrawing.cs
- ReadOnlyDictionary.cs
- TransformerTypeCollection.cs
- QilInvokeEarlyBound.cs
- EpmContentDeSerializer.cs
- QilValidationVisitor.cs
- HandlerMappingMemo.cs
- CompiledRegexRunner.cs
- ToolStripSystemRenderer.cs
- XmlSchemaType.cs
- ObjectAnimationUsingKeyFrames.cs
- BoolExpressionVisitors.cs
- Matrix.cs
- ValidatedControlConverter.cs
- _ChunkParse.cs
- LogLogRecord.cs
- ProcessProtocolHandler.cs
- ObjectDataSourceView.cs
- RSAOAEPKeyExchangeFormatter.cs
- DataViewManager.cs
- ScriptingJsonSerializationSection.cs
- SafeUserTokenHandle.cs
- SelectionRange.cs
- RemoteWebConfigurationHostStream.cs
- SqlDataSourceView.cs
- FixedSOMTableCell.cs
- TcpClientSocketManager.cs
- SqlDataSourceStatusEventArgs.cs
- ArgumentOutOfRangeException.cs
- Send.cs
- OverflowException.cs
- PageStatePersister.cs
- DataGridDesigner.cs
- MulticastDelegate.cs
- DynamicValidatorEventArgs.cs
- SAPIEngineTypes.cs
- Matrix3D.cs
- PnrpPeerResolverBindingElement.cs
- SqlPersistenceProviderFactory.cs
- xmlfixedPageInfo.cs
- DoWhile.cs
- DependencyPropertyKind.cs
- CompilerInfo.cs
- MulticastDelegate.cs
- ComPlusTraceRecord.cs
- DragDeltaEventArgs.cs
- ConditionalWeakTable.cs
- RuntimeCompatibilityAttribute.cs
- EncryptedXml.cs
- PenThread.cs
- SafeProcessHandle.cs
- OracleInternalConnection.cs
- QueryOutputWriterV1.cs
- Run.cs
- LoginUtil.cs
- StsCommunicationException.cs
- FontEmbeddingManager.cs
- SubpageParagraph.cs
- CacheDependency.cs
- StaticDataManager.cs
- QilReplaceVisitor.cs
- TransformerInfoCollection.cs
- ResourceAttributes.cs
- EventLogEntry.cs
- NoneExcludedImageIndexConverter.cs
- Transform3DGroup.cs
- ViewManagerAttribute.cs
- WindowsGraphicsCacheManager.cs
- ComPlusTypeLoader.cs
- SmtpFailedRecipientsException.cs
- Int32.cs
- FlowLayoutPanel.cs
- iisPickupDirectory.cs
- externdll.cs
- RegistrySecurity.cs
- InternalResources.cs
- Speller.cs
- CompositeDataBoundControl.cs
- XPathNode.cs
- ThicknessConverter.cs
- DataBoundLiteralControl.cs
- ToolstripProfessionalRenderer.cs
- AnnouncementEndpointElement.cs
- RectangleF.cs
- CustomErrorsSectionWrapper.cs
- SvcFileManager.cs
- WebContext.cs
- WinEventHandler.cs
- XmlValueConverter.cs
- PropertyGeneratedEventArgs.cs
- ControlPaint.cs
- XPathMultyIterator.cs
- ReflectionUtil.cs
- EventsTab.cs
- ObjectConverter.cs
- SystemFonts.cs
- ProjectionAnalyzer.cs