Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / xsp / System / Web / UI / WebControls / CompositeDataBoundControl.cs / 1 / CompositeDataBoundControl.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls { using System; using System.Collections; using System.Security.Permissions; [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public abstract class CompositeDataBoundControl : DataBoundControl, INamingContainer { internal const string ItemCountViewStateKey = "_!ItemCount"; public override ControlCollection Controls { get { EnsureChildControls(); return base.Controls; } } ////// Overriden by DataBoundControl to determine if the control should /// recreate its control hierarchy based on values in view state. /// If the control hierarchy should be created, i.e. view state does /// exist, it calls CreateChildControls with a dummy (empty) data source /// which is usable for enumeration purposes only. /// protected internal override void CreateChildControls() { Controls.Clear(); object controlCount = ViewState[ItemCountViewStateKey]; if (controlCount == null && RequiresDataBinding) { EnsureDataBound(); } if (controlCount != null && ((int)controlCount) != -1) { DummyDataSource dummyDataSource = new DummyDataSource((int)controlCount); CreateChildControls(dummyDataSource, false); ClearChildViewState(); } } ////// Performs the work of creating the control hierarchy based on a data source. /// When dataBinding is true, the specified data source contains real /// data, and the data is supposed to be pushed into the UI. /// When dataBinding is false, the specified data source is a dummy data /// source, that allows enumerating the right number of items, but the items /// themselves are null and do not contain data. In this case, the recreated /// control hierarchy reinitializes its state from view state. /// It enables a DataBoundControl to encapsulate the logic of creating its /// control hierarchy in both modes into a single code path. /// /// /// The data source to be used to enumerate items. /// /// /// Whether the method has been called from DataBind or not. /// ////// The number of items created based on the data source. Put another way, its /// the number of items enumerated from the data source. /// protected abstract int CreateChildControls(IEnumerable dataSource, bool dataBinding); ////// Overriden by DataBoundControl to use its properties to determine the real /// data source that the control should bind to. It then clears the existing /// control hierarchy, and calls createChildControls to create a new control /// hierarchy based on the resolved data source. /// The implementation resolves various data source related properties to /// arrive at the appropriate IEnumerable implementation to use as the real /// data source. /// When resolving data sources, the DataSourceControlID takes highest precedence. /// In this mode, DataMember is used to access the appropriate list from the /// DataControl. /// If DataSourceControlID is not set, the value of the DataSource property is used. /// In this second alternative, DataMember is used to extract the appropriate /// list if the control has been handed an IListSource as a data source. /// protected internal override void PerformDataBinding(IEnumerable data) { base.PerformDataBinding(data); Controls.Clear(); ClearChildViewState(); TrackViewState(); int controlCount = CreateChildControls(data, true); ChildControlsCreated = true; ViewState[ItemCountViewStateKey] = controlCount; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls { using System; using System.Collections; using System.Security.Permissions; [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public abstract class CompositeDataBoundControl : DataBoundControl, INamingContainer { internal const string ItemCountViewStateKey = "_!ItemCount"; public override ControlCollection Controls { get { EnsureChildControls(); return base.Controls; } } ////// Overriden by DataBoundControl to determine if the control should /// recreate its control hierarchy based on values in view state. /// If the control hierarchy should be created, i.e. view state does /// exist, it calls CreateChildControls with a dummy (empty) data source /// which is usable for enumeration purposes only. /// protected internal override void CreateChildControls() { Controls.Clear(); object controlCount = ViewState[ItemCountViewStateKey]; if (controlCount == null && RequiresDataBinding) { EnsureDataBound(); } if (controlCount != null && ((int)controlCount) != -1) { DummyDataSource dummyDataSource = new DummyDataSource((int)controlCount); CreateChildControls(dummyDataSource, false); ClearChildViewState(); } } ////// Performs the work of creating the control hierarchy based on a data source. /// When dataBinding is true, the specified data source contains real /// data, and the data is supposed to be pushed into the UI. /// When dataBinding is false, the specified data source is a dummy data /// source, that allows enumerating the right number of items, but the items /// themselves are null and do not contain data. In this case, the recreated /// control hierarchy reinitializes its state from view state. /// It enables a DataBoundControl to encapsulate the logic of creating its /// control hierarchy in both modes into a single code path. /// /// /// The data source to be used to enumerate items. /// /// /// Whether the method has been called from DataBind or not. /// ////// The number of items created based on the data source. Put another way, its /// the number of items enumerated from the data source. /// protected abstract int CreateChildControls(IEnumerable dataSource, bool dataBinding); ////// Overriden by DataBoundControl to use its properties to determine the real /// data source that the control should bind to. It then clears the existing /// control hierarchy, and calls createChildControls to create a new control /// hierarchy based on the resolved data source. /// The implementation resolves various data source related properties to /// arrive at the appropriate IEnumerable implementation to use as the real /// data source. /// When resolving data sources, the DataSourceControlID takes highest precedence. /// In this mode, DataMember is used to access the appropriate list from the /// DataControl. /// If DataSourceControlID is not set, the value of the DataSource property is used. /// In this second alternative, DataMember is used to extract the appropriate /// list if the control has been handed an IListSource as a data source. /// protected internal override void PerformDataBinding(IEnumerable data) { base.PerformDataBinding(data); Controls.Clear(); ClearChildViewState(); TrackViewState(); int controlCount = CreateChildControls(data, true); ChildControlsCreated = true; ViewState[ItemCountViewStateKey] = controlCount; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
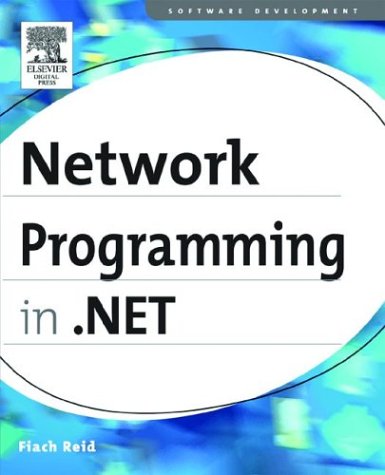
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- UriExt.cs
- CaseStatement.cs
- AsnEncodedData.cs
- ContextMenuStrip.cs
- ObjectDataSourceMethodEventArgs.cs
- DataTrigger.cs
- SemaphoreFullException.cs
- validation.cs
- WeakHashtable.cs
- DataGridViewCellStyleChangedEventArgs.cs
- HttpModule.cs
- BevelBitmapEffect.cs
- GenericTypeParameterBuilder.cs
- ExpressionLexer.cs
- ListItemViewAttribute.cs
- ProfileEventArgs.cs
- AuthenticationSection.cs
- CallContext.cs
- FormsAuthenticationConfiguration.cs
- GenericAuthenticationEventArgs.cs
- BoundColumn.cs
- TokenizerHelper.cs
- InputReferenceExpression.cs
- RelationshipConverter.cs
- UInt16Converter.cs
- ImageSource.cs
- Point3DValueSerializer.cs
- SrgsElementFactoryCompiler.cs
- cookie.cs
- TrackingQueryElement.cs
- ImpersonateTokenRef.cs
- PageBreakRecord.cs
- FieldCollectionEditor.cs
- SafeArrayTypeMismatchException.cs
- DesignerSerializationOptionsAttribute.cs
- BatchWriter.cs
- TagPrefixCollection.cs
- UTF8Encoding.cs
- AppModelKnownContentFactory.cs
- _SpnDictionary.cs
- Content.cs
- HtmlAnchor.cs
- FormsAuthenticationCredentials.cs
- LinkGrep.cs
- XsdBuildProvider.cs
- ListViewGroup.cs
- SqlComparer.cs
- SafeSecurityHandles.cs
- _IPv4Address.cs
- DbProviderSpecificTypePropertyAttribute.cs
- GridEntryCollection.cs
- MatrixTransform3D.cs
- Stackframe.cs
- ProfileBuildProvider.cs
- FillBehavior.cs
- NamespaceListProperty.cs
- ClientTargetSection.cs
- UrlPath.cs
- DefaultBindingPropertyAttribute.cs
- ZipFileInfo.cs
- FlagsAttribute.cs
- Positioning.cs
- datacache.cs
- FrameworkReadOnlyPropertyMetadata.cs
- HtmlTableCell.cs
- TextTreeDeleteContentUndoUnit.cs
- PtsHost.cs
- ThreadSafeList.cs
- LinqExpressionNormalizer.cs
- XmlILCommand.cs
- OleDbRowUpdatedEvent.cs
- _ConnectionGroup.cs
- MTConfigUtil.cs
- StateMachineTimers.cs
- EnumMember.cs
- MenuStrip.cs
- BitmapCacheBrush.cs
- QilLiteral.cs
- PropertyStore.cs
- PersonalizationDictionary.cs
- ExcCanonicalXml.cs
- Cursor.cs
- EditableRegion.cs
- DataStorage.cs
- HttpConfigurationSystem.cs
- CommandEventArgs.cs
- XmlQueryRuntime.cs
- COM2PictureConverter.cs
- OdbcTransaction.cs
- GlobalizationSection.cs
- MergablePropertyAttribute.cs
- DisplayInformation.cs
- Int64AnimationUsingKeyFrames.cs
- DataControlCommands.cs
- XmlDocumentSurrogate.cs
- CachedPathData.cs
- Environment.cs
- SourceFilter.cs
- Pair.cs
- Delegate.cs